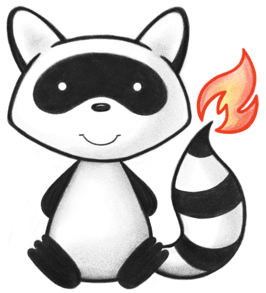
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Attachment10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Instant10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 010import org.hl7.fhir.dstu3.model.DiagnosticReport; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.exceptions.FHIRException; 013 014public class DiagnosticReport10_30 { 015 016 public static org.hl7.fhir.dstu2.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.dstu3.model.DiagnosticReport src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.dstu2.model.DiagnosticReport tgt = new org.hl7.fhir.dstu2.model.DiagnosticReport(); 020 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 025 if (src.hasCategory()) 026 tgt.setCategory(CodeableConcept10_30.convertCodeableConcept(src.getCategory())); 027 if (src.hasCode()) 028 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 029 if (src.hasSubject()) 030 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 031 if (src.hasContext()) 032 tgt.setEncounter(Reference10_30.convertReference(src.getContext())); 033 if (src.hasEffective()) 034 tgt.setEffective(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getEffective())); 035 if (src.hasIssuedElement()) 036 tgt.setIssuedElement(Instant10_30.convertInstant(src.getIssuedElement())); 037 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) tgt.addRequest(Reference10_30.convertReference(t)); 038 for (org.hl7.fhir.dstu3.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference10_30.convertReference(t)); 039 for (org.hl7.fhir.dstu3.model.Reference t : src.getResult()) tgt.addResult(Reference10_30.convertReference(t)); 040 for (org.hl7.fhir.dstu3.model.Reference t : src.getImagingStudy()) 041 tgt.addImagingStudy(Reference10_30.convertReference(t)); 042 for (org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent t : src.getImage()) 043 tgt.addImage(convertDiagnosticReportImageComponent(t)); 044 if (src.hasConclusionElement()) 045 tgt.setConclusionElement(String10_30.convertString(src.getConclusionElement())); 046 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCodedDiagnosis()) 047 tgt.addCodedDiagnosis(CodeableConcept10_30.convertCodeableConcept(t)); 048 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPresentedForm()) 049 tgt.addPresentedForm(Attachment10_30.convertAttachment(t)); 050 return tgt; 051 } 052 053 public static org.hl7.fhir.dstu3.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.dstu2.model.DiagnosticReport src) throws FHIRException { 054 if (src == null || src.isEmpty()) 055 return null; 056 org.hl7.fhir.dstu3.model.DiagnosticReport tgt = new org.hl7.fhir.dstu3.model.DiagnosticReport(); 057 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 058 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 059 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 060 if (src.hasStatus()) 061 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 062 if (src.hasCategory()) 063 tgt.setCategory(CodeableConcept10_30.convertCodeableConcept(src.getCategory())); 064 if (src.hasCode()) 065 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 066 if (src.hasSubject()) 067 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 068 if (src.hasEncounter()) 069 tgt.setContext(Reference10_30.convertReference(src.getEncounter())); 070 if (src.hasEffective()) 071 tgt.setEffective(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getEffective())); 072 if (src.hasIssuedElement()) 073 tgt.setIssuedElement(Instant10_30.convertInstant(src.getIssuedElement())); 074 for (org.hl7.fhir.dstu2.model.Reference t : src.getRequest()) tgt.addBasedOn(Reference10_30.convertReference(t)); 075 for (org.hl7.fhir.dstu2.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference10_30.convertReference(t)); 076 for (org.hl7.fhir.dstu2.model.Reference t : src.getResult()) tgt.addResult(Reference10_30.convertReference(t)); 077 for (org.hl7.fhir.dstu2.model.Reference t : src.getImagingStudy()) 078 tgt.addImagingStudy(Reference10_30.convertReference(t)); 079 for (org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent t : src.getImage()) 080 tgt.addImage(convertDiagnosticReportImageComponent(t)); 081 if (src.hasConclusionElement()) 082 tgt.setConclusionElement(String10_30.convertString(src.getConclusionElement())); 083 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCodedDiagnosis()) 084 tgt.addCodedDiagnosis(CodeableConcept10_30.convertCodeableConcept(t)); 085 for (org.hl7.fhir.dstu2.model.Attachment t : src.getPresentedForm()) 086 tgt.addPresentedForm(Attachment10_30.convertAttachment(t)); 087 return tgt; 088 } 089 090 public static org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent convertDiagnosticReportImageComponent(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent src) throws FHIRException { 091 if (src == null || src.isEmpty()) 092 return null; 093 org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent tgt = new org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent(); 094 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 095 if (src.hasCommentElement()) 096 tgt.setCommentElement(String10_30.convertString(src.getCommentElement())); 097 if (src.hasLink()) 098 tgt.setLink(Reference10_30.convertReference(src.getLink())); 099 return tgt; 100 } 101 102 public static org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent convertDiagnosticReportImageComponent(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent src) throws FHIRException { 103 if (src == null || src.isEmpty()) 104 return null; 105 org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent tgt = new org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent(); 106 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 107 if (src.hasCommentElement()) 108 tgt.setCommentElement(String10_30.convertString(src.getCommentElement())); 109 if (src.hasLink()) 110 tgt.setLink(Reference10_30.convertReference(src.getLink())); 111 return tgt; 112 } 113 114 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 115 if (src == null || src.isEmpty()) 116 return null; 117 Enumeration<DiagnosticReport.DiagnosticReportStatus> tgt = new Enumeration<>(new DiagnosticReport.DiagnosticReportStatusEnumFactory()); 118 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 119 if (src.getValue() == null) { 120 tgt.setValue(null); 121 } else { 122 switch (src.getValue()) { 123 case REGISTERED: 124 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.REGISTERED); 125 break; 126 case PARTIAL: 127 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PARTIAL); 128 break; 129 case FINAL: 130 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.FINAL); 131 break; 132 case CORRECTED: 133 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CORRECTED); 134 break; 135 case APPENDED: 136 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.APPENDED); 137 break; 138 case CANCELLED: 139 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CANCELLED); 140 break; 141 case ENTEREDINERROR: 142 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 143 break; 144 default: 145 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.NULL); 146 break; 147 } 148 } 149 return tgt; 150 } 151 152 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 153 if (src == null || src.isEmpty()) 154 return null; 155 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatusEnumFactory()); 156 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 157 if (src.getValue() == null) { 158 tgt.setValue(null); 159 } else { 160 switch (src.getValue()) { 161 case REGISTERED: 162 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.REGISTERED); 163 break; 164 case PARTIAL: 165 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.PARTIAL); 166 break; 167 case FINAL: 168 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.FINAL); 169 break; 170 case CORRECTED: 171 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.CORRECTED); 172 break; 173 case APPENDED: 174 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.APPENDED); 175 break; 176 case CANCELLED: 177 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.CANCELLED); 178 break; 179 case ENTEREDINERROR: 180 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 181 break; 182 default: 183 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.NULL); 184 break; 185 } 186 } 187 return tgt; 188 } 189}