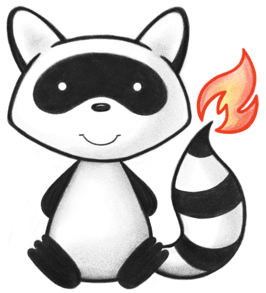
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Attachment10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Coding10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Period10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Instant10_30; 012import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 013import org.hl7.fhir.dstu2.model.CodeableConcept; 014import org.hl7.fhir.dstu2.model.DocumentReference; 015import org.hl7.fhir.dstu2.model.Enumeration; 016import org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus; 017import org.hl7.fhir.dstu3.model.Enumerations; 018import org.hl7.fhir.exceptions.FHIRException; 019 020public class DocumentReference10_30 { 021 022 static public ReferredDocumentStatus convertDocStatus(CodeableConcept cc) { 023 if (CodeableConcept10_30.hasConcept(cc, "http://hl7.org/fhir/composition-status", "preliminary")) 024 return ReferredDocumentStatus.PRELIMINARY; 025 if (CodeableConcept10_30.hasConcept(cc, "http://hl7.org/fhir/composition-status", "final")) 026 return ReferredDocumentStatus.FINAL; 027 if (CodeableConcept10_30.hasConcept(cc, "http://hl7.org/fhir/composition-status", "amended")) 028 return ReferredDocumentStatus.AMENDED; 029 if (CodeableConcept10_30.hasConcept(cc, "http://hl7.org/fhir/composition-status", "entered-in-error")) 030 return ReferredDocumentStatus.ENTEREDINERROR; 031 return null; 032 } 033 034 static public CodeableConcept convertDocStatus(ReferredDocumentStatus docStatus) { 035 CodeableConcept cc = new CodeableConcept(); 036 switch (docStatus) { 037 case AMENDED: 038 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("amended"); 039 break; 040 case ENTEREDINERROR: 041 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("entered-in-error"); 042 break; 043 case FINAL: 044 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("final"); 045 break; 046 case PRELIMINARY: 047 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("preliminary"); 048 break; 049 default: 050 return null; 051 } 052 return cc; 053 } 054 055 public static org.hl7.fhir.dstu3.model.DocumentReference convertDocumentReference(org.hl7.fhir.dstu2.model.DocumentReference src) throws FHIRException { 056 if (src == null || src.isEmpty()) 057 return null; 058 org.hl7.fhir.dstu3.model.DocumentReference tgt = new org.hl7.fhir.dstu3.model.DocumentReference(); 059 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 060 if (src.hasMasterIdentifier()) 061 tgt.setMasterIdentifier(Identifier10_30.convertIdentifier(src.getMasterIdentifier())); 062 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 063 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 064 if (src.hasSubject()) 065 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 066 if (src.hasType()) 067 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 068 if (src.hasClass_()) 069 tgt.setClass_(CodeableConcept10_30.convertCodeableConcept(src.getClass_())); 070 for (org.hl7.fhir.dstu2.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference10_30.convertReference(t)); 071 if (src.hasCustodian()) 072 tgt.setCustodian(Reference10_30.convertReference(src.getCustodian())); 073 if (src.hasAuthenticator()) 074 tgt.setAuthenticator(Reference10_30.convertReference(src.getAuthenticator())); 075 if (src.hasCreatedElement()) 076 tgt.setCreatedElement(DateTime10_30.convertDateTime(src.getCreatedElement())); 077 if (src.hasIndexedElement()) 078 tgt.setIndexedElement(Instant10_30.convertInstant(src.getIndexedElement())); 079 if (src.hasStatus()) 080 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 081 if (src.hasDocStatus()) 082 tgt.setDocStatus(convertDocStatus(src.getDocStatus())); 083 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 084 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 085 if (src.hasDescriptionElement()) 086 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 087 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getSecurityLabel()) 088 tgt.addSecurityLabel(CodeableConcept10_30.convertCodeableConcept(t)); 089 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 090 tgt.addContent(convertDocumentReferenceContentComponent(t)); 091 if (src.hasContext()) 092 tgt.setContext(convertDocumentReferenceContextComponent(src.getContext())); 093 return tgt; 094 } 095 096 public static org.hl7.fhir.dstu2.model.DocumentReference convertDocumentReference(org.hl7.fhir.dstu3.model.DocumentReference src) throws FHIRException { 097 if (src == null || src.isEmpty()) 098 return null; 099 org.hl7.fhir.dstu2.model.DocumentReference tgt = new org.hl7.fhir.dstu2.model.DocumentReference(); 100 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 101 if (src.hasMasterIdentifier()) 102 tgt.setMasterIdentifier(Identifier10_30.convertIdentifier(src.getMasterIdentifier())); 103 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 104 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 105 if (src.hasSubject()) 106 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 107 if (src.hasType()) 108 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 109 if (src.hasClass_()) 110 tgt.setClass_(CodeableConcept10_30.convertCodeableConcept(src.getClass_())); 111 for (org.hl7.fhir.dstu3.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference10_30.convertReference(t)); 112 if (src.hasCustodian()) 113 tgt.setCustodian(Reference10_30.convertReference(src.getCustodian())); 114 if (src.hasAuthenticator()) 115 tgt.setAuthenticator(Reference10_30.convertReference(src.getAuthenticator())); 116 if (src.hasCreatedElement()) 117 tgt.setCreatedElement(DateTime10_30.convertDateTime(src.getCreatedElement())); 118 if (src.hasIndexedElement()) 119 tgt.setIndexedElement(Instant10_30.convertInstant(src.getIndexedElement())); 120 if (src.hasStatus()) 121 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 122 if (src.hasDocStatus()) 123 tgt.setDocStatus(convertDocStatus(src.getDocStatus())); 124 for (org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 125 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 126 if (src.hasDescriptionElement()) 127 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 128 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSecurityLabel()) 129 tgt.addSecurityLabel(CodeableConcept10_30.convertCodeableConcept(t)); 130 for (org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 131 tgt.addContent(convertDocumentReferenceContentComponent(t)); 132 if (src.hasContext()) 133 tgt.setContext(convertDocumentReferenceContextComponent(src.getContext())); 134 return tgt; 135 } 136 137 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 138 if (src == null || src.isEmpty()) 139 return null; 140 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent(); 141 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 142 if (src.hasAttachment()) 143 tgt.setAttachment(Attachment10_30.convertAttachment(src.getAttachment())); 144 if (src.hasFormat()) 145 tgt.addFormat(Coding10_30.convertCoding(src.getFormat())); 146 return tgt; 147 } 148 149 public static org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 150 if (src == null || src.isEmpty()) 151 return null; 152 org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent(); 153 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 154 if (src.hasAttachment()) 155 tgt.setAttachment(Attachment10_30.convertAttachment(src.getAttachment())); 156 for (org.hl7.fhir.dstu2.model.Coding t : src.getFormat()) tgt.setFormat(Coding10_30.convertCoding(t)); 157 return tgt; 158 } 159 160 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent convertDocumentReferenceContextComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextComponent src) throws FHIRException { 161 if (src == null || src.isEmpty()) 162 return null; 163 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent(); 164 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 165 if (src.hasEncounter()) 166 tgt.setEncounter(Reference10_30.convertReference(src.getEncounter())); 167 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getEvent()) 168 tgt.addEvent(CodeableConcept10_30.convertCodeableConcept(t)); 169 if (src.hasPeriod()) 170 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 171 if (src.hasFacilityType()) 172 tgt.setFacilityType(CodeableConcept10_30.convertCodeableConcept(src.getFacilityType())); 173 if (src.hasPracticeSetting()) 174 tgt.setPracticeSetting(CodeableConcept10_30.convertCodeableConcept(src.getPracticeSetting())); 175 if (src.hasSourcePatientInfo()) 176 tgt.setSourcePatientInfo(Reference10_30.convertReference(src.getSourcePatientInfo())); 177 for (org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent t : src.getRelated()) 178 tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 179 return tgt; 180 } 181 182 public static org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextComponent convertDocumentReferenceContextComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent src) throws FHIRException { 183 if (src == null || src.isEmpty()) 184 return null; 185 org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextComponent tgt = new org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextComponent(); 186 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 187 if (src.hasEncounter()) 188 tgt.setEncounter(Reference10_30.convertReference(src.getEncounter())); 189 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getEvent()) 190 tgt.addEvent(CodeableConcept10_30.convertCodeableConcept(t)); 191 if (src.hasPeriod()) 192 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 193 if (src.hasFacilityType()) 194 tgt.setFacilityType(CodeableConcept10_30.convertCodeableConcept(src.getFacilityType())); 195 if (src.hasPracticeSetting()) 196 tgt.setPracticeSetting(CodeableConcept10_30.convertCodeableConcept(src.getPracticeSetting())); 197 if (src.hasSourcePatientInfo()) 198 tgt.setSourcePatientInfo(Reference10_30.convertReference(src.getSourcePatientInfo())); 199 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent t : src.getRelated()) 200 tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent src) throws FHIRException { 205 if (src == null || src.isEmpty()) 206 return null; 207 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent(); 208 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 209 if (src.hasIdentifier()) 210 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 211 if (src.hasRef()) 212 tgt.setRef(Reference10_30.convertReference(src.getRef())); 213 return tgt; 214 } 215 216 public static org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent src) throws FHIRException { 217 if (src == null || src.isEmpty()) 218 return null; 219 org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent tgt = new org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent(); 220 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 221 if (src.hasIdentifier()) 222 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 223 if (src.hasRef()) 224 tgt.setRef(Reference10_30.convertReference(src.getRef())); 225 return tgt; 226 } 227 228 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 229 if (src == null || src.isEmpty()) 230 return null; 231 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent(); 232 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 233 if (src.hasCode()) 234 tgt.setCodeElement(convertDocumentRelationshipType(src.getCodeElement())); 235 if (src.hasTarget()) 236 tgt.setTarget(Reference10_30.convertReference(src.getTarget())); 237 return tgt; 238 } 239 240 public static org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 241 if (src == null || src.isEmpty()) 242 return null; 243 org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent(); 244 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 245 if (src.hasCode()) 246 tgt.setCodeElement(convertDocumentRelationshipType(src.getCodeElement())); 247 if (src.hasTarget()) 248 tgt.setTarget(Reference10_30.convertReference(src.getTarget())); 249 return tgt; 250 } 251 252 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType> src) throws FHIRException { 253 if (src == null || src.isEmpty()) 254 return null; 255 Enumeration<DocumentReference.DocumentRelationshipType> tgt = new Enumeration<>(new DocumentReference.DocumentRelationshipTypeEnumFactory()); 256 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 257 if (src.getValue() == null) { 258 tgt.setValue(null); 259 } else { 260 switch (src.getValue()) { 261 case REPLACES: 262 tgt.setValue(DocumentReference.DocumentRelationshipType.REPLACES); 263 break; 264 case TRANSFORMS: 265 tgt.setValue(DocumentReference.DocumentRelationshipType.TRANSFORMS); 266 break; 267 case SIGNS: 268 tgt.setValue(DocumentReference.DocumentRelationshipType.SIGNS); 269 break; 270 case APPENDS: 271 tgt.setValue(DocumentReference.DocumentRelationshipType.APPENDS); 272 break; 273 default: 274 tgt.setValue(DocumentReference.DocumentRelationshipType.NULL); 275 break; 276 } 277 } 278 return tgt; 279 } 280 281 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> src) throws FHIRException { 282 if (src == null || src.isEmpty()) 283 return null; 284 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipTypeEnumFactory()); 285 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 286 if (src.getValue() == null) { 287 tgt.setValue(null); 288 } else { 289 switch (src.getValue()) { 290 case REPLACES: 291 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.REPLACES); 292 break; 293 case TRANSFORMS: 294 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.TRANSFORMS); 295 break; 296 case SIGNS: 297 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.SIGNS); 298 break; 299 case APPENDS: 300 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.APPENDS); 301 break; 302 default: 303 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.NULL); 304 break; 305 } 306 } 307 return tgt; 308 } 309 310 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 311 if (src == null || src.isEmpty()) return null; 312 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatusEnumFactory()); 313 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 314 if (src.getValue() == null) { 315 tgt.setValue(null); 316} else { 317 switch(src.getValue()) { 318 case CURRENT: 319 tgt.setValue(Enumerations.DocumentReferenceStatus.CURRENT); 320 break; 321 case SUPERSEDED: 322 tgt.setValue(Enumerations.DocumentReferenceStatus.SUPERSEDED); 323 break; 324 case ENTEREDINERROR: 325 tgt.setValue(Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 326 break; 327 default: 328 tgt.setValue(Enumerations.DocumentReferenceStatus.NULL); 329 break; 330 } 331} 332 return tgt; 333 } 334 335 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 336 if (src == null || src.isEmpty()) return null; 337 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatusEnumFactory()); 338 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 339 if (src.getValue() == null) { 340 tgt.setValue(null); 341} else { 342 switch(src.getValue()) { 343 case CURRENT: 344 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.CURRENT); 345 break; 346 case SUPERSEDED: 347 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.SUPERSEDED); 348 break; 349 case ENTEREDINERROR: 350 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 351 break; 352 default: 353 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.NULL); 354 break; 355 } 356} 357 return tgt; 358 } 359}