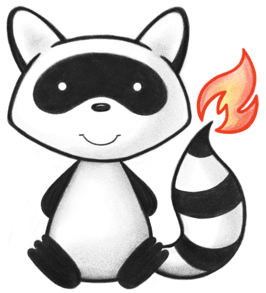
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class EnrollmentResponse10_30 { 011 012 public static org.hl7.fhir.dstu3.model.EnrollmentResponse convertEnrollmentResponse(org.hl7.fhir.dstu2.model.EnrollmentResponse src) throws FHIRException { 013 if (src == null || src.isEmpty()) 014 return null; 015 org.hl7.fhir.dstu3.model.EnrollmentResponse tgt = new org.hl7.fhir.dstu3.model.EnrollmentResponse(); 016 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 017 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 018 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 019 if (src.hasRequest()) 020 tgt.setRequest(Reference10_30.convertReference(src.getRequest())); 021 if (src.hasDispositionElement()) 022 tgt.setDispositionElement(String10_30.convertString(src.getDispositionElement())); 023 if (src.hasCreatedElement()) 024 tgt.setCreatedElement(DateTime10_30.convertDateTime(src.getCreatedElement())); 025 if (src.hasOrganization()) 026 tgt.setOrganization(Reference10_30.convertReference(src.getOrganization())); 027 if (src.hasRequestProvider()) 028 tgt.setRequestProvider(Reference10_30.convertReference(src.getRequestProvider())); 029 if (src.hasRequestOrganization()) 030 tgt.setRequestOrganization(Reference10_30.convertReference(src.getRequestOrganization())); 031 return tgt; 032 } 033 034 public static org.hl7.fhir.dstu2.model.EnrollmentResponse convertEnrollmentResponse(org.hl7.fhir.dstu3.model.EnrollmentResponse src) throws FHIRException { 035 if (src == null || src.isEmpty()) 036 return null; 037 org.hl7.fhir.dstu2.model.EnrollmentResponse tgt = new org.hl7.fhir.dstu2.model.EnrollmentResponse(); 038 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 039 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 040 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 041 if (src.hasDispositionElement()) 042 tgt.setDispositionElement(String10_30.convertString(src.getDispositionElement())); 043 if (src.hasCreatedElement()) 044 tgt.setCreatedElement(DateTime10_30.convertDateTime(src.getCreatedElement())); 045 return tgt; 046 } 047}