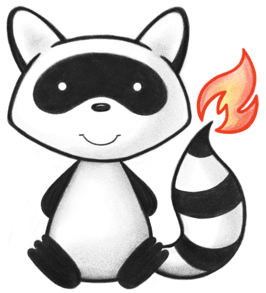
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.exceptions.FHIRException; 005 006public class Enumerations10_30 { 007 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus> convertConformanceResourceStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> src) throws FHIRException { 008 if (src == null || src.isEmpty()) return null; 009 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory()); 010 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 011 if (src.getValue() == null) { 012 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus.NULL); 013 } else { 014 switch (src.getValue()) { 015 case DRAFT: 016 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus.DRAFT); 017 break; 018 case ACTIVE: 019 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus.ACTIVE); 020 break; 021 case RETIRED: 022 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus.RETIRED); 023 break; 024 default: 025 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus.NULL); 026 break; 027 } 028 } 029 return tgt; 030 } 031 032 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> convertConformanceResourceStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus> src) throws FHIRException { 033 if (src == null || src.isEmpty()) return null; 034 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory()); 035 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 036 if (src.getValue() == null) { 037 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.NULL); 038 } else { 039 switch (src.getValue()) { 040 case DRAFT: 041 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.DRAFT); 042 break; 043 case ACTIVE: 044 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.ACTIVE); 045 break; 046 case RETIRED: 047 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.RETIRED); 048 break; 049 default: 050 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.NULL); 051 break; 052 } 053 } 054 return tgt; 055 } 056 057 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> src) throws FHIRException { 058 if (src == null || src.isEmpty()) return null; 059 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGenderEnumFactory()); 060 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 061 if (src.getValue() == null) { 062 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender.NULL); 063 } else { 064 switch (src.getValue()) { 065 case MALE: 066 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender.MALE); 067 break; 068 case FEMALE: 069 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender.FEMALE); 070 break; 071 case OTHER: 072 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender.OTHER); 073 break; 074 case UNKNOWN: 075 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender.UNKNOWN); 076 break; 077 default: 078 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender.NULL); 079 break; 080 } 081 } 082 return tgt; 083 } 084 085 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender> src) throws FHIRException { 086 if (src == null || src.isEmpty()) return null; 087 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory()); 088 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 089 if (src.getValue() == null) { 090 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.NULL); 091 } else { 092 switch (src.getValue()) { 093 case MALE: 094 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.MALE); 095 break; 096 case FEMALE: 097 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.FEMALE); 098 break; 099 case OTHER: 100 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.OTHER); 101 break; 102 case UNKNOWN: 103 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.UNKNOWN); 104 break; 105 default: 106 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.NULL); 107 break; 108 } 109 } 110 return tgt; 111 } 112}