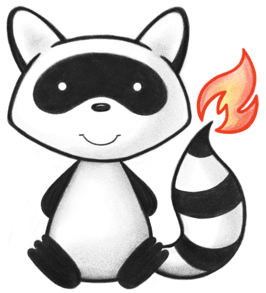
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 009import org.hl7.fhir.dstu3.model.Enumeration; 010import org.hl7.fhir.dstu3.model.FamilyMemberHistory; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class FamilyMemberHistory10_30 { 014 015 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.FamilyMemberHistory.FamilyHistoryStatus> convertFamilyHistoryStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus> src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 Enumeration<FamilyMemberHistory.FamilyHistoryStatus> tgt = new Enumeration<>(new FamilyMemberHistory.FamilyHistoryStatusEnumFactory()); 019 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 020 if (src.getValue() == null) { 021 tgt.setValue(null); 022 } else { 023 switch (src.getValue()) { 024 case PARTIAL: 025 tgt.setValue(FamilyMemberHistory.FamilyHistoryStatus.PARTIAL); 026 break; 027 case COMPLETED: 028 tgt.setValue(FamilyMemberHistory.FamilyHistoryStatus.COMPLETED); 029 break; 030 case ENTEREDINERROR: 031 tgt.setValue(FamilyMemberHistory.FamilyHistoryStatus.ENTEREDINERROR); 032 break; 033 case HEALTHUNKNOWN: 034 tgt.setValue(FamilyMemberHistory.FamilyHistoryStatus.HEALTHUNKNOWN); 035 break; 036 default: 037 tgt.setValue(FamilyMemberHistory.FamilyHistoryStatus.NULL); 038 break; 039 } 040 } 041 return tgt; 042 } 043 044 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus> convertFamilyHistoryStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.FamilyMemberHistory.FamilyHistoryStatus> src) throws FHIRException { 045 if (src == null || src.isEmpty()) 046 return null; 047 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatusEnumFactory()); 048 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 049 if (src.getValue() == null) { 050 tgt.setValue(null); 051 } else { 052 switch (src.getValue()) { 053 case PARTIAL: 054 tgt.setValue(org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus.PARTIAL); 055 break; 056 case COMPLETED: 057 tgt.setValue(org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus.COMPLETED); 058 break; 059 case ENTEREDINERROR: 060 tgt.setValue(org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus.ENTEREDINERROR); 061 break; 062 case HEALTHUNKNOWN: 063 tgt.setValue(org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus.HEALTHUNKNOWN); 064 break; 065 default: 066 tgt.setValue(org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyHistoryStatus.NULL); 067 break; 068 } 069 } 070 return tgt; 071 } 072 073 public static org.hl7.fhir.dstu3.model.FamilyMemberHistory convertFamilyMemberHistory(org.hl7.fhir.dstu2.model.FamilyMemberHistory src) throws FHIRException { 074 if (src == null || src.isEmpty()) 075 return null; 076 org.hl7.fhir.dstu3.model.FamilyMemberHistory tgt = new org.hl7.fhir.dstu3.model.FamilyMemberHistory(); 077 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 078 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 079 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 080 if (src.hasPatient()) 081 tgt.setPatient(Reference10_30.convertReference(src.getPatient())); 082 if (src.hasDate()) 083 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 084 if (src.hasStatus()) 085 tgt.setStatusElement(convertFamilyHistoryStatus(src.getStatusElement())); 086 if (src.hasNameElement()) 087 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 088 if (src.hasRelationship()) 089 tgt.setRelationship(CodeableConcept10_30.convertCodeableConcept(src.getRelationship())); 090 if (src.hasGender()) 091 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 092 if (src.hasBorn()) 093 tgt.setBorn(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getBorn())); 094 if (src.hasAge()) 095 tgt.setAge(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getAge())); 096 if (src.hasDeceased()) 097 tgt.setDeceased(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getDeceased())); 098 for (org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent t : src.getCondition()) 099 tgt.addCondition(convertFamilyMemberHistoryConditionComponent(t)); 100 return tgt; 101 } 102 103 public static org.hl7.fhir.dstu2.model.FamilyMemberHistory convertFamilyMemberHistory(org.hl7.fhir.dstu3.model.FamilyMemberHistory src) throws FHIRException { 104 if (src == null || src.isEmpty()) 105 return null; 106 org.hl7.fhir.dstu2.model.FamilyMemberHistory tgt = new org.hl7.fhir.dstu2.model.FamilyMemberHistory(); 107 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 108 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 109 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 110 if (src.hasPatient()) 111 tgt.setPatient(Reference10_30.convertReference(src.getPatient())); 112 if (src.hasDate()) 113 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 114 if (src.hasStatus()) 115 tgt.setStatusElement(convertFamilyHistoryStatus(src.getStatusElement())); 116 if (src.hasNameElement()) 117 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 118 if (src.hasRelationship()) 119 tgt.setRelationship(CodeableConcept10_30.convertCodeableConcept(src.getRelationship())); 120 if (src.hasGender()) 121 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 122 if (src.hasBorn()) 123 tgt.setBorn(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getBorn())); 124 if (src.hasAge()) 125 tgt.setAge(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getAge())); 126 if (src.hasDeceased()) 127 tgt.setDeceased(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getDeceased())); 128 for (org.hl7.fhir.dstu3.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent t : src.getCondition()) 129 tgt.addCondition(convertFamilyMemberHistoryConditionComponent(t)); 130 return tgt; 131 } 132 133 public static org.hl7.fhir.dstu3.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent convertFamilyMemberHistoryConditionComponent(org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 org.hl7.fhir.dstu3.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent tgt = new org.hl7.fhir.dstu3.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent(); 137 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 138 if (src.hasCode()) 139 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 140 if (src.hasOutcome()) 141 tgt.setOutcome(CodeableConcept10_30.convertCodeableConcept(src.getOutcome())); 142 if (src.hasOnset()) 143 tgt.setOnset(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getOnset())); 144 return tgt; 145 } 146 147 public static org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent convertFamilyMemberHistoryConditionComponent(org.hl7.fhir.dstu3.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent src) throws FHIRException { 148 if (src == null || src.isEmpty()) 149 return null; 150 org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent tgt = new org.hl7.fhir.dstu2.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent(); 151 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 152 if (src.hasCode()) 153 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 154 if (src.hasOutcome()) 155 tgt.setOutcome(CodeableConcept10_30.convertCodeableConcept(src.getOutcome())); 156 if (src.hasOnset()) 157 tgt.setOnset(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getOnset())); 158 return tgt; 159 } 160}