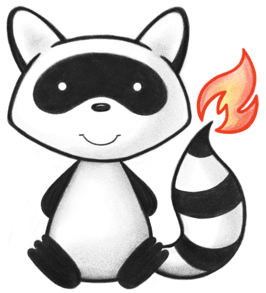
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Period10_30; 008import org.hl7.fhir.dstu3.model.Enumeration; 009import org.hl7.fhir.dstu3.model.Flag; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Flag10_30 { 013 014 public static org.hl7.fhir.dstu3.model.Flag convertFlag(org.hl7.fhir.dstu2.model.Flag src) throws FHIRException { 015 if (src == null || src.isEmpty()) 016 return null; 017 org.hl7.fhir.dstu3.model.Flag tgt = new org.hl7.fhir.dstu3.model.Flag(); 018 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 021 if (src.hasCategory()) 022 tgt.setCategory(CodeableConcept10_30.convertCodeableConcept(src.getCategory())); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertFlagStatus(src.getStatusElement())); 025 if (src.hasPeriod()) 026 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 027 if (src.hasSubject()) 028 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 029 if (src.hasEncounter()) 030 tgt.setEncounter(Reference10_30.convertReference(src.getEncounter())); 031 if (src.hasAuthor()) 032 tgt.setAuthor(Reference10_30.convertReference(src.getAuthor())); 033 if (src.hasCode()) 034 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 035 return tgt; 036 } 037 038 public static org.hl7.fhir.dstu2.model.Flag convertFlag(org.hl7.fhir.dstu3.model.Flag src) throws FHIRException { 039 if (src == null || src.isEmpty()) 040 return null; 041 org.hl7.fhir.dstu2.model.Flag tgt = new org.hl7.fhir.dstu2.model.Flag(); 042 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 043 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 044 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 045 if (src.hasCategory()) 046 tgt.setCategory(CodeableConcept10_30.convertCodeableConcept(src.getCategory())); 047 if (src.hasStatus()) 048 tgt.setStatusElement(convertFlagStatus(src.getStatusElement())); 049 if (src.hasPeriod()) 050 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 051 if (src.hasSubject()) 052 tgt.setSubject(Reference10_30.convertReference(src.getSubject())); 053 if (src.hasEncounter()) 054 tgt.setEncounter(Reference10_30.convertReference(src.getEncounter())); 055 if (src.hasAuthor()) 056 tgt.setAuthor(Reference10_30.convertReference(src.getAuthor())); 057 if (src.hasCode()) 058 tgt.setCode(CodeableConcept10_30.convertCodeableConcept(src.getCode())); 059 return tgt; 060 } 061 062 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Flag.FlagStatus> convertFlagStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Flag.FlagStatus> src) throws FHIRException { 063 if (src == null || src.isEmpty()) 064 return null; 065 Enumeration<Flag.FlagStatus> tgt = new Enumeration<>(new Flag.FlagStatusEnumFactory()); 066 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 067 if (src.getValue() == null) { 068 tgt.setValue(null); 069 } else { 070 switch (src.getValue()) { 071 case ACTIVE: 072 tgt.setValue(Flag.FlagStatus.ACTIVE); 073 break; 074 case INACTIVE: 075 tgt.setValue(Flag.FlagStatus.INACTIVE); 076 break; 077 case ENTEREDINERROR: 078 tgt.setValue(Flag.FlagStatus.ENTEREDINERROR); 079 break; 080 default: 081 tgt.setValue(Flag.FlagStatus.NULL); 082 break; 083 } 084 } 085 return tgt; 086 } 087 088 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Flag.FlagStatus> convertFlagStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Flag.FlagStatus> src) throws FHIRException { 089 if (src == null || src.isEmpty()) 090 return null; 091 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Flag.FlagStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Flag.FlagStatusEnumFactory()); 092 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 093 if (src.getValue() == null) { 094 tgt.setValue(null); 095 } else { 096 switch (src.getValue()) { 097 case ACTIVE: 098 tgt.setValue(org.hl7.fhir.dstu2.model.Flag.FlagStatus.ACTIVE); 099 break; 100 case INACTIVE: 101 tgt.setValue(org.hl7.fhir.dstu2.model.Flag.FlagStatus.INACTIVE); 102 break; 103 case ENTEREDINERROR: 104 tgt.setValue(org.hl7.fhir.dstu2.model.Flag.FlagStatus.ENTEREDINERROR); 105 break; 106 default: 107 tgt.setValue(org.hl7.fhir.dstu2.model.Flag.FlagStatus.NULL); 108 break; 109 } 110 } 111 return tgt; 112 } 113}