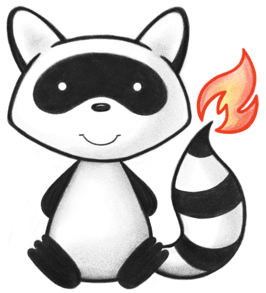
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Address10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Decimal10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 011import org.hl7.fhir.dstu2.model.Enumeration; 012import org.hl7.fhir.dstu2.model.Location; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class Location10_30 { 016 017 public static org.hl7.fhir.dstu2.model.Location convertLocation(org.hl7.fhir.dstu3.model.Location src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.dstu2.model.Location tgt = new org.hl7.fhir.dstu2.model.Location(); 021 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 024 if (src.hasStatus()) 025 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 026 if (src.hasNameElement()) 027 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 028 if (src.hasDescriptionElement()) 029 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 030 if (src.hasMode()) 031 tgt.setModeElement(convertLocationMode(src.getModeElement())); 032 if (src.hasType()) 033 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 034 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 035 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 036 if (src.hasAddress()) 037 tgt.setAddress(Address10_30.convertAddress(src.getAddress())); 038 if (src.hasPhysicalType()) 039 tgt.setPhysicalType(CodeableConcept10_30.convertCodeableConcept(src.getPhysicalType())); 040 if (src.hasPosition()) 041 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 042 if (src.hasManagingOrganization()) 043 tgt.setManagingOrganization(Reference10_30.convertReference(src.getManagingOrganization())); 044 if (src.hasPartOf()) 045 tgt.setPartOf(Reference10_30.convertReference(src.getPartOf())); 046 return tgt; 047 } 048 049 public static org.hl7.fhir.dstu3.model.Location convertLocation(org.hl7.fhir.dstu2.model.Location src) throws FHIRException { 050 if (src == null || src.isEmpty()) 051 return null; 052 org.hl7.fhir.dstu3.model.Location tgt = new org.hl7.fhir.dstu3.model.Location(); 053 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 056 if (src.hasStatus()) 057 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 058 if (src.hasNameElement()) 059 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 060 if (src.hasDescriptionElement()) 061 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 062 if (src.hasMode()) 063 tgt.setModeElement(convertLocationMode(src.getModeElement())); 064 if (src.hasType()) 065 tgt.setType(CodeableConcept10_30.convertCodeableConcept(src.getType())); 066 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 067 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 068 if (src.hasAddress()) 069 tgt.setAddress(Address10_30.convertAddress(src.getAddress())); 070 if (src.hasPhysicalType()) 071 tgt.setPhysicalType(CodeableConcept10_30.convertCodeableConcept(src.getPhysicalType())); 072 if (src.hasPosition()) 073 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 074 if (src.hasManagingOrganization()) 075 tgt.setManagingOrganization(Reference10_30.convertReference(src.getManagingOrganization())); 076 if (src.hasPartOf()) 077 tgt.setPartOf(Reference10_30.convertReference(src.getPartOf())); 078 return tgt; 079 } 080 081 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> src) throws FHIRException { 082 if (src == null || src.isEmpty()) 083 return null; 084 Enumeration<Location.LocationMode> tgt = new Enumeration<>(new Location.LocationModeEnumFactory()); 085 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 086 if (src.getValue() == null) { 087 tgt.setValue(null); 088 } else { 089 switch (src.getValue()) { 090 case INSTANCE: 091 tgt.setValue(Location.LocationMode.INSTANCE); 092 break; 093 case KIND: 094 tgt.setValue(Location.LocationMode.KIND); 095 break; 096 default: 097 tgt.setValue(Location.LocationMode.NULL); 098 break; 099 } 100 } 101 return tgt; 102 } 103 104 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationMode> src) throws FHIRException { 105 if (src == null || src.isEmpty()) 106 return null; 107 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Location.LocationModeEnumFactory()); 108 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 109 if (src.getValue() == null) { 110 tgt.setValue(null); 111 } else { 112 switch (src.getValue()) { 113 case INSTANCE: 114 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationMode.INSTANCE); 115 break; 116 case KIND: 117 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationMode.KIND); 118 break; 119 default: 120 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationMode.NULL); 121 break; 122 } 123 } 124 return tgt; 125 } 126 127 public static org.hl7.fhir.dstu3.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.dstu2.model.Location.LocationPositionComponent src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.dstu3.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.dstu3.model.Location.LocationPositionComponent(); 131 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 132 if (src.hasLongitudeElement()) 133 tgt.setLongitudeElement(Decimal10_30.convertDecimal(src.getLongitudeElement())); 134 if (src.hasLatitudeElement()) 135 tgt.setLatitudeElement(Decimal10_30.convertDecimal(src.getLatitudeElement())); 136 if (src.hasAltitudeElement()) 137 tgt.setAltitudeElement(Decimal10_30.convertDecimal(src.getAltitudeElement())); 138 return tgt; 139 } 140 141 public static org.hl7.fhir.dstu2.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.dstu3.model.Location.LocationPositionComponent src) throws FHIRException { 142 if (src == null || src.isEmpty()) 143 return null; 144 org.hl7.fhir.dstu2.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.dstu2.model.Location.LocationPositionComponent(); 145 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 146 if (src.hasLongitudeElement()) 147 tgt.setLongitudeElement(Decimal10_30.convertDecimal(src.getLongitudeElement())); 148 if (src.hasLatitudeElement()) 149 tgt.setLatitudeElement(Decimal10_30.convertDecimal(src.getLatitudeElement())); 150 if (src.hasAltitudeElement()) 151 tgt.setAltitudeElement(Decimal10_30.convertDecimal(src.getAltitudeElement())); 152 return tgt; 153 } 154 155 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> src) throws FHIRException { 156 if (src == null || src.isEmpty()) 157 return null; 158 Enumeration<Location.LocationStatus> tgt = new Enumeration<>(new Location.LocationStatusEnumFactory()); 159 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 160 if (src.getValue() == null) { 161 tgt.setValue(null); 162 } else { 163 switch (src.getValue()) { 164 case ACTIVE: 165 tgt.setValue(Location.LocationStatus.ACTIVE); 166 break; 167 case SUSPENDED: 168 tgt.setValue(Location.LocationStatus.SUSPENDED); 169 break; 170 case INACTIVE: 171 tgt.setValue(Location.LocationStatus.INACTIVE); 172 break; 173 default: 174 tgt.setValue(Location.LocationStatus.NULL); 175 break; 176 } 177 } 178 return tgt; 179 } 180 181 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationStatus> src) throws FHIRException { 182 if (src == null || src.isEmpty()) 183 return null; 184 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Location.LocationStatusEnumFactory()); 185 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 186 if (src.getValue() == null) { 187 tgt.setValue(null); 188 } else { 189 switch (src.getValue()) { 190 case ACTIVE: 191 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.ACTIVE); 192 break; 193 case SUSPENDED: 194 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.SUSPENDED); 195 break; 196 case INACTIVE: 197 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.INACTIVE); 198 break; 199 default: 200 tgt.setValue(org.hl7.fhir.dstu3.model.Location.LocationStatus.NULL); 201 break; 202 } 203 } 204 return tgt; 205 } 206}