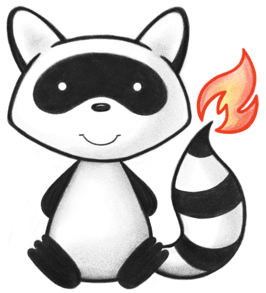
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Ratio10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Timing10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.dstu3.model.MedicationStatement; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class MedicationStatement10_30 { 016 017 public static org.hl7.fhir.dstu3.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.dstu2.model.MedicationStatement src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.dstu3.model.MedicationStatement tgt = new org.hl7.fhir.dstu3.model.MedicationStatement(); 021 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 024 if (src.hasStatus()) 025 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 026 if (src.hasMedication()) 027 tgt.setMedication(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMedication())); 028 if (src.hasPatient()) 029 tgt.setSubject(Reference10_30.convertReference(src.getPatient())); 030 if (src.hasEffective()) 031 tgt.setEffective(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getEffective())); 032 if (src.hasInformationSource()) 033 tgt.setInformationSource(Reference10_30.convertReference(src.getInformationSource())); 034 for (org.hl7.fhir.dstu2.model.Reference t : src.getSupportingInformation()) 035 tgt.addDerivedFrom(Reference10_30.convertReference(t)); 036 if (src.hasDateAsserted()) 037 tgt.setDateAssertedElement(DateTime10_30.convertDateTime(src.getDateAssertedElement())); 038 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReasonNotTaken()) 039 tgt.addReasonNotTaken(CodeableConcept10_30.convertCodeableConcept(t)); 040 if (src.hasNote()) 041 tgt.addNote().setText(src.getNote()); 042 for (org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent t : src.getDosage()) 043 tgt.addDosage(convertMedicationStatementDosageComponent(t)); 044 return tgt; 045 } 046 047 public static org.hl7.fhir.dstu2.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.dstu3.model.MedicationStatement src) throws FHIRException { 048 if (src == null || src.isEmpty()) 049 return null; 050 org.hl7.fhir.dstu2.model.MedicationStatement tgt = new org.hl7.fhir.dstu2.model.MedicationStatement(); 051 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 054 if (src.hasStatus()) 055 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 056 if (src.hasMedication()) 057 tgt.setMedication(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMedication())); 058 if (src.hasSubject()) 059 tgt.setPatient(Reference10_30.convertReference(src.getSubject())); 060 if (src.hasEffective()) 061 tgt.setEffective(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getEffective())); 062 if (src.hasInformationSource()) 063 tgt.setInformationSource(Reference10_30.convertReference(src.getInformationSource())); 064 for (org.hl7.fhir.dstu3.model.Reference t : src.getDerivedFrom()) 065 tgt.addSupportingInformation(Reference10_30.convertReference(t)); 066 if (src.hasDateAsserted()) 067 tgt.setDateAssertedElement(DateTime10_30.convertDateTime(src.getDateAssertedElement())); 068 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonNotTaken()) 069 tgt.addReasonNotTaken(CodeableConcept10_30.convertCodeableConcept(t)); 070 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.setNote(t.getText()); 071 for (org.hl7.fhir.dstu3.model.Dosage t : src.getDosage()) 072 tgt.addDosage(convertMedicationStatementDosageComponent(t)); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.dstu3.model.Dosage convertMedicationStatementDosageComponent(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent src) throws FHIRException { 077 if (src == null || src.isEmpty()) 078 return null; 079 org.hl7.fhir.dstu3.model.Dosage tgt = new org.hl7.fhir.dstu3.model.Dosage(); 080 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 081 if (src.hasTextElement()) 082 tgt.setTextElement(String10_30.convertString(src.getTextElement())); 083 if (src.hasTiming()) 084 tgt.setTiming(Timing10_30.convertTiming(src.getTiming())); 085 if (src.hasAsNeeded()) 086 tgt.setAsNeeded(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getAsNeeded())); 087 if (src.hasSiteCodeableConcept()) 088 tgt.setSite(CodeableConcept10_30.convertCodeableConcept(src.getSiteCodeableConcept())); 089 if (src.hasRoute()) 090 tgt.setRoute(CodeableConcept10_30.convertCodeableConcept(src.getRoute())); 091 if (src.hasMethod()) 092 tgt.setMethod(CodeableConcept10_30.convertCodeableConcept(src.getMethod())); 093 if (src.hasRate()) 094 tgt.setRate(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getRate())); 095 if (src.hasMaxDosePerPeriod()) 096 tgt.setMaxDosePerPeriod(Ratio10_30.convertRatio(src.getMaxDosePerPeriod())); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent convertMedicationStatementDosageComponent(org.hl7.fhir.dstu3.model.Dosage src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent tgt = new org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent(); 104 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 105 if (src.hasTextElement()) 106 tgt.setTextElement(String10_30.convertString(src.getTextElement())); 107 if (src.hasTiming()) 108 tgt.setTiming(Timing10_30.convertTiming(src.getTiming())); 109 if (src.hasAsNeeded()) 110 tgt.setAsNeeded(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getAsNeeded())); 111 if (src.hasSite()) 112 tgt.setSite(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getSite())); 113 if (src.hasRoute()) 114 tgt.setRoute(CodeableConcept10_30.convertCodeableConcept(src.getRoute())); 115 if (src.hasMethod()) 116 tgt.setMethod(CodeableConcept10_30.convertCodeableConcept(src.getMethod())); 117 if (src.hasRate()) 118 tgt.setRate(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getRate())); 119 if (src.hasMaxDosePerPeriod()) 120 tgt.setMaxDosePerPeriod(Ratio10_30.convertRatio(src.getMaxDosePerPeriod())); 121 return tgt; 122 } 123 124 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationStatement.MedicationStatementStatus> convertMedicationStatementStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> src) throws FHIRException { 125 if (src == null || src.isEmpty()) 126 return null; 127 Enumeration<MedicationStatement.MedicationStatementStatus> tgt = new Enumeration<>(new MedicationStatement.MedicationStatementStatusEnumFactory()); 128 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 129 if (src.getValue() == null) { 130 tgt.setValue(null); 131 } else { 132 switch (src.getValue()) { 133 case ACTIVE: 134 tgt.setValue(MedicationStatement.MedicationStatementStatus.ACTIVE); 135 break; 136 case COMPLETED: 137 tgt.setValue(MedicationStatement.MedicationStatementStatus.COMPLETED); 138 break; 139 case ENTEREDINERROR: 140 tgt.setValue(MedicationStatement.MedicationStatementStatus.ENTEREDINERROR); 141 break; 142 case INTENDED: 143 tgt.setValue(MedicationStatement.MedicationStatementStatus.INTENDED); 144 break; 145 default: 146 tgt.setValue(MedicationStatement.MedicationStatementStatus.NULL); 147 break; 148 } 149 } 150 return tgt; 151 } 152 153 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> convertMedicationStatementStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationStatement.MedicationStatementStatus> src) throws FHIRException { 154 if (src == null || src.isEmpty()) 155 return null; 156 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatusEnumFactory()); 157 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 158 if (src.getValue() == null) { 159 tgt.setValue(null); 160 } else { 161 switch (src.getValue()) { 162 case ACTIVE: 163 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.ACTIVE); 164 break; 165 case COMPLETED: 166 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.COMPLETED); 167 break; 168 case ENTEREDINERROR: 169 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.ENTEREDINERROR); 170 break; 171 case INTENDED: 172 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.INTENDED); 173 break; 174 default: 175 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.NULL); 176 break; 177 } 178 } 179 return tgt; 180 } 181}