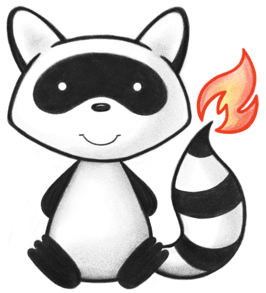
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Address10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Attachment10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.HumanName10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Period10_30; 012import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 013import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Date10_30; 014import org.hl7.fhir.dstu2.model.Enumeration; 015import org.hl7.fhir.dstu2.model.Patient; 016import org.hl7.fhir.exceptions.FHIRException; 017 018public class Patient10_30 { 019 020 public static org.hl7.fhir.dstu3.model.Patient.AnimalComponent convertAnimalComponent(org.hl7.fhir.dstu2.model.Patient.AnimalComponent src) throws FHIRException { 021 if (src == null || src.isEmpty()) 022 return null; 023 org.hl7.fhir.dstu3.model.Patient.AnimalComponent tgt = new org.hl7.fhir.dstu3.model.Patient.AnimalComponent(); 024 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 025 if (src.hasSpecies()) 026 tgt.setSpecies(CodeableConcept10_30.convertCodeableConcept(src.getSpecies())); 027 if (src.hasBreed()) 028 tgt.setBreed(CodeableConcept10_30.convertCodeableConcept(src.getBreed())); 029 if (src.hasGenderStatus()) 030 tgt.setGenderStatus(CodeableConcept10_30.convertCodeableConcept(src.getGenderStatus())); 031 return tgt; 032 } 033 034 public static org.hl7.fhir.dstu2.model.Patient.AnimalComponent convertAnimalComponent(org.hl7.fhir.dstu3.model.Patient.AnimalComponent src) throws FHIRException { 035 if (src == null || src.isEmpty()) 036 return null; 037 org.hl7.fhir.dstu2.model.Patient.AnimalComponent tgt = new org.hl7.fhir.dstu2.model.Patient.AnimalComponent(); 038 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 039 if (src.hasSpecies()) 040 tgt.setSpecies(CodeableConcept10_30.convertCodeableConcept(src.getSpecies())); 041 if (src.hasBreed()) 042 tgt.setBreed(CodeableConcept10_30.convertCodeableConcept(src.getBreed())); 043 if (src.hasGenderStatus()) 044 tgt.setGenderStatus(CodeableConcept10_30.convertCodeableConcept(src.getGenderStatus())); 045 return tgt; 046 } 047 048 public static org.hl7.fhir.dstu3.model.Patient.ContactComponent convertContactComponent(org.hl7.fhir.dstu2.model.Patient.ContactComponent src) throws FHIRException { 049 if (src == null || src.isEmpty()) 050 return null; 051 org.hl7.fhir.dstu3.model.Patient.ContactComponent tgt = new org.hl7.fhir.dstu3.model.Patient.ContactComponent(); 052 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 053 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getRelationship()) 054 tgt.addRelationship(CodeableConcept10_30.convertCodeableConcept(t)); 055 if (src.hasName()) 056 tgt.setName(HumanName10_30.convertHumanName(src.getName())); 057 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 058 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 059 if (src.hasAddress()) 060 tgt.setAddress(Address10_30.convertAddress(src.getAddress())); 061 if (src.hasGender()) 062 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 063 if (src.hasOrganization()) 064 tgt.setOrganization(Reference10_30.convertReference(src.getOrganization())); 065 if (src.hasPeriod()) 066 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.dstu2.model.Patient.ContactComponent convertContactComponent(org.hl7.fhir.dstu3.model.Patient.ContactComponent src) throws FHIRException { 071 if (src == null || src.isEmpty()) 072 return null; 073 org.hl7.fhir.dstu2.model.Patient.ContactComponent tgt = new org.hl7.fhir.dstu2.model.Patient.ContactComponent(); 074 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 075 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getRelationship()) 076 tgt.addRelationship(CodeableConcept10_30.convertCodeableConcept(t)); 077 if (src.hasName()) 078 tgt.setName(HumanName10_30.convertHumanName(src.getName())); 079 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 080 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 081 if (src.hasAddress()) 082 tgt.setAddress(Address10_30.convertAddress(src.getAddress())); 083 if (src.hasGender()) 084 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 085 if (src.hasOrganization()) 086 tgt.setOrganization(Reference10_30.convertReference(src.getOrganization())); 087 if (src.hasPeriod()) 088 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 089 return tgt; 090 } 091 092 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Patient.LinkType> convertLinkType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Patient.LinkType> src) throws FHIRException { 093 if (src == null || src.isEmpty()) 094 return null; 095 Enumeration<Patient.LinkType> tgt = new Enumeration<>(new Patient.LinkTypeEnumFactory()); 096 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 097 if (src.getValue() == null) { 098 tgt.setValue(null); 099 } else { 100 switch (src.getValue()) { 101 case REPLACEDBY: 102 tgt.setValue(Patient.LinkType.REPLACE); 103 break; 104 case REPLACES: 105 tgt.setValue(Patient.LinkType.REPLACE); 106 break; 107 case REFER: 108 tgt.setValue(Patient.LinkType.REFER); 109 break; 110 case SEEALSO: 111 tgt.setValue(Patient.LinkType.SEEALSO); 112 break; 113 default: 114 tgt.setValue(Patient.LinkType.NULL); 115 break; 116 } 117 } 118 return tgt; 119 } 120 121 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Patient.LinkType> convertLinkType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Patient.LinkType> src) throws FHIRException { 122 if (src == null || src.isEmpty()) 123 return null; 124 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Patient.LinkType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Patient.LinkTypeEnumFactory()); 125 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 126 if (src.getValue() == null) { 127 tgt.setValue(null); 128 } else { 129 switch (src.getValue()) { 130 case REPLACE: 131 tgt.setValue(org.hl7.fhir.dstu3.model.Patient.LinkType.REPLACEDBY); 132 break; 133 case REFER: 134 tgt.setValue(org.hl7.fhir.dstu3.model.Patient.LinkType.REFER); 135 break; 136 case SEEALSO: 137 tgt.setValue(org.hl7.fhir.dstu3.model.Patient.LinkType.SEEALSO); 138 break; 139 default: 140 tgt.setValue(org.hl7.fhir.dstu3.model.Patient.LinkType.NULL); 141 break; 142 } 143 } 144 return tgt; 145 } 146 147 public static org.hl7.fhir.dstu3.model.Patient convertPatient(org.hl7.fhir.dstu2.model.Patient src) throws FHIRException { 148 if (src == null || src.isEmpty()) 149 return null; 150 org.hl7.fhir.dstu3.model.Patient tgt = new org.hl7.fhir.dstu3.model.Patient(); 151 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 152 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 153 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 154 if (src.hasActiveElement()) 155 tgt.setActiveElement(Boolean10_30.convertBoolean(src.getActiveElement())); 156 for (org.hl7.fhir.dstu2.model.HumanName t : src.getName()) tgt.addName(HumanName10_30.convertHumanName(t)); 157 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 158 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 159 if (src.hasGender()) 160 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 161 if (src.hasBirthDateElement()) 162 tgt.setBirthDateElement(Date10_30.convertDate(src.getBirthDateElement())); 163 if (src.hasDeceased()) 164 tgt.setDeceased(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getDeceased())); 165 for (org.hl7.fhir.dstu2.model.Address t : src.getAddress()) tgt.addAddress(Address10_30.convertAddress(t)); 166 if (src.hasMaritalStatus()) 167 tgt.setMaritalStatus(CodeableConcept10_30.convertCodeableConcept(src.getMaritalStatus())); 168 if (src.hasMultipleBirth()) 169 tgt.setMultipleBirth(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMultipleBirth())); 170 for (org.hl7.fhir.dstu2.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_30.convertAttachment(t)); 171 for (org.hl7.fhir.dstu2.model.Patient.ContactComponent t : src.getContact()) 172 tgt.addContact(convertContactComponent(t)); 173 if (src.hasAnimal()) 174 tgt.setAnimal(convertAnimalComponent(src.getAnimal())); 175 for (org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent t : src.getCommunication()) 176 tgt.addCommunication(convertPatientCommunicationComponent(t)); 177 for (org.hl7.fhir.dstu2.model.Reference t : src.getCareProvider()) 178 tgt.addGeneralPractitioner(Reference10_30.convertReference(t)); 179 if (src.hasManagingOrganization()) 180 tgt.setManagingOrganization(Reference10_30.convertReference(src.getManagingOrganization())); 181 for (org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent t : src.getLink()) 182 tgt.addLink(convertPatientLinkComponent(t)); 183 return tgt; 184 } 185 186 public static org.hl7.fhir.dstu2.model.Patient convertPatient(org.hl7.fhir.dstu3.model.Patient src) throws FHIRException { 187 if (src == null || src.isEmpty()) 188 return null; 189 org.hl7.fhir.dstu2.model.Patient tgt = new org.hl7.fhir.dstu2.model.Patient(); 190 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 191 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 192 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 193 if (src.hasActiveElement()) 194 tgt.setActiveElement(Boolean10_30.convertBoolean(src.getActiveElement())); 195 for (org.hl7.fhir.dstu3.model.HumanName t : src.getName()) tgt.addName(HumanName10_30.convertHumanName(t)); 196 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 197 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 198 if (src.hasGender()) 199 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 200 if (src.hasBirthDateElement()) 201 tgt.setBirthDateElement(Date10_30.convertDate(src.getBirthDateElement())); 202 if (src.hasDeceased()) 203 tgt.setDeceased(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getDeceased())); 204 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) tgt.addAddress(Address10_30.convertAddress(t)); 205 if (src.hasMaritalStatus()) 206 tgt.setMaritalStatus(CodeableConcept10_30.convertCodeableConcept(src.getMaritalStatus())); 207 if (src.hasMultipleBirth()) 208 tgt.setMultipleBirth(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getMultipleBirth())); 209 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_30.convertAttachment(t)); 210 for (org.hl7.fhir.dstu3.model.Patient.ContactComponent t : src.getContact()) 211 tgt.addContact(convertContactComponent(t)); 212 if (src.hasAnimal()) 213 tgt.setAnimal(convertAnimalComponent(src.getAnimal())); 214 for (org.hl7.fhir.dstu3.model.Patient.PatientCommunicationComponent t : src.getCommunication()) 215 tgt.addCommunication(convertPatientCommunicationComponent(t)); 216 for (org.hl7.fhir.dstu3.model.Reference t : src.getGeneralPractitioner()) 217 tgt.addCareProvider(Reference10_30.convertReference(t)); 218 if (src.hasManagingOrganization()) 219 tgt.setManagingOrganization(Reference10_30.convertReference(src.getManagingOrganization())); 220 for (org.hl7.fhir.dstu3.model.Patient.PatientLinkComponent t : src.getLink()) 221 tgt.addLink(convertPatientLinkComponent(t)); 222 return tgt; 223 } 224 225 public static org.hl7.fhir.dstu3.model.Patient.PatientCommunicationComponent convertPatientCommunicationComponent(org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent src) throws FHIRException { 226 if (src == null || src.isEmpty()) 227 return null; 228 org.hl7.fhir.dstu3.model.Patient.PatientCommunicationComponent tgt = new org.hl7.fhir.dstu3.model.Patient.PatientCommunicationComponent(); 229 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 230 if (src.hasLanguage()) 231 tgt.setLanguage(CodeableConcept10_30.convertCodeableConcept(src.getLanguage())); 232 if (src.hasPreferredElement()) 233 tgt.setPreferredElement(Boolean10_30.convertBoolean(src.getPreferredElement())); 234 return tgt; 235 } 236 237 public static org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent convertPatientCommunicationComponent(org.hl7.fhir.dstu3.model.Patient.PatientCommunicationComponent src) throws FHIRException { 238 if (src == null || src.isEmpty()) 239 return null; 240 org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent tgt = new org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent(); 241 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 242 if (src.hasLanguage()) 243 tgt.setLanguage(CodeableConcept10_30.convertCodeableConcept(src.getLanguage())); 244 if (src.hasPreferredElement()) 245 tgt.setPreferredElement(Boolean10_30.convertBoolean(src.getPreferredElement())); 246 return tgt; 247 } 248 249 public static org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent convertPatientLinkComponent(org.hl7.fhir.dstu3.model.Patient.PatientLinkComponent src) throws FHIRException { 250 if (src == null || src.isEmpty()) 251 return null; 252 org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent tgt = new org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent(); 253 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 254 if (src.hasOther()) 255 tgt.setOther(Reference10_30.convertReference(src.getOther())); 256 if (src.hasType()) 257 tgt.setTypeElement(convertLinkType(src.getTypeElement())); 258 return tgt; 259 } 260 261 public static org.hl7.fhir.dstu3.model.Patient.PatientLinkComponent convertPatientLinkComponent(org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent src) throws FHIRException { 262 if (src == null || src.isEmpty()) 263 return null; 264 org.hl7.fhir.dstu3.model.Patient.PatientLinkComponent tgt = new org.hl7.fhir.dstu3.model.Patient.PatientLinkComponent(); 265 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 266 if (src.hasOther()) 267 tgt.setOther(Reference10_30.convertReference(src.getOther())); 268 if (src.hasType()) 269 tgt.setTypeElement(convertLinkType(src.getTypeElement())); 270 return tgt; 271 } 272}