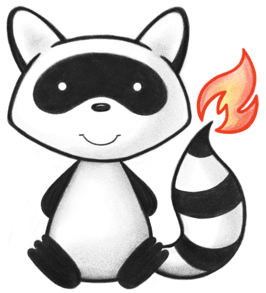
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Integer10_30; 005import org.hl7.fhir.dstu3.model.ProcessRequest; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class ProcessRequest10_30 { 009 public static org.hl7.fhir.dstu3.model.ProcessRequest.ItemsComponent convertItemsComponent(org.hl7.fhir.dstu2.model.ProcessRequest.ItemsComponent src) throws FHIRException { 010 if (src == null || src.isEmpty()) return null; 011 org.hl7.fhir.dstu3.model.ProcessRequest.ItemsComponent tgt = new org.hl7.fhir.dstu3.model.ProcessRequest.ItemsComponent(); 012 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 013 if (src.hasSequenceLinkIdElement()) 014 tgt.setSequenceLinkIdElement(Integer10_30.convertInteger(src.getSequenceLinkIdElement())); 015 return tgt; 016 } 017 018 public static org.hl7.fhir.dstu2.model.ProcessRequest.ItemsComponent convertItemsComponent(org.hl7.fhir.dstu3.model.ProcessRequest.ItemsComponent src) throws FHIRException { 019 if (src == null || src.isEmpty()) return null; 020 org.hl7.fhir.dstu2.model.ProcessRequest.ItemsComponent tgt = new org.hl7.fhir.dstu2.model.ProcessRequest.ItemsComponent(); 021 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 022 if (src.hasSequenceLinkIdElement()) 023 tgt.setSequenceLinkIdElement(Integer10_30.convertInteger(src.getSequenceLinkIdElement())); 024 return tgt; 025 } 026 027 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ProcessRequest.ActionList> convertActionList(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ProcessRequest.ActionList> src) throws FHIRException { 028 if (src == null || src.isEmpty()) return null; 029 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ProcessRequest.ActionList> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ProcessRequest.ActionListEnumFactory()); 030 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 031 if (src.getValue() == null) { 032 tgt.setValue(null); 033} else { 034 switch(src.getValue()) { 035 case CANCEL: 036 tgt.setValue(ProcessRequest.ActionList.CANCEL); 037 break; 038 case POLL: 039 tgt.setValue(ProcessRequest.ActionList.POLL); 040 break; 041 case REPROCESS: 042 tgt.setValue(ProcessRequest.ActionList.REPROCESS); 043 break; 044 case STATUS: 045 tgt.setValue(ProcessRequest.ActionList.STATUS); 046 break; 047 default: 048 tgt.setValue(ProcessRequest.ActionList.NULL); 049 break; 050 } 051} 052 return tgt; 053 } 054 055 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ProcessRequest.ActionList> convertActionList(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ProcessRequest.ActionList> src) throws FHIRException { 056 if (src == null || src.isEmpty()) return null; 057 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ProcessRequest.ActionList> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ProcessRequest.ActionListEnumFactory()); 058 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 059 if (src.getValue() == null) { 060 tgt.setValue(null); 061} else { 062 switch(src.getValue()) { 063 case CANCEL: 064 tgt.setValue(org.hl7.fhir.dstu2.model.ProcessRequest.ActionList.CANCEL); 065 break; 066 case POLL: 067 tgt.setValue(org.hl7.fhir.dstu2.model.ProcessRequest.ActionList.POLL); 068 break; 069 case REPROCESS: 070 tgt.setValue(org.hl7.fhir.dstu2.model.ProcessRequest.ActionList.REPROCESS); 071 break; 072 case STATUS: 073 tgt.setValue(org.hl7.fhir.dstu2.model.ProcessRequest.ActionList.STATUS); 074 break; 075 default: 076 tgt.setValue(org.hl7.fhir.dstu2.model.ProcessRequest.ActionList.NULL); 077 break; 078 } 079} 080 return tgt; 081 } 082}