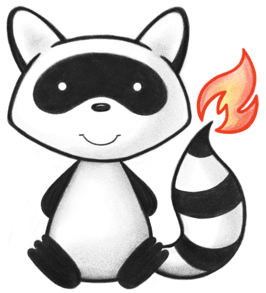
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Coding10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 011import org.hl7.fhir.dstu2.model.Enumeration; 012import org.hl7.fhir.dstu2.model.Questionnaire; 013import org.hl7.fhir.dstu3.model.ContactDetail; 014import org.hl7.fhir.dstu3.model.Enumerations; 015import org.hl7.fhir.exceptions.FHIRException; 016 017public class Questionnaire10_30 { 018 019 public static org.hl7.fhir.dstu2.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu3.model.Questionnaire src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 org.hl7.fhir.dstu2.model.Questionnaire tgt = new org.hl7.fhir.dstu2.model.Questionnaire(); 023 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 024 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 025 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 026 if (src.hasVersionElement()) 027 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 028 if (src.hasStatus()) 029 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 030 if (src.hasDate()) 031 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 032 if (src.hasPublisherElement()) 033 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 034 for (ContactDetail t : src.getContact()) 035 for (org.hl7.fhir.dstu3.model.ContactPoint t1 : t.getTelecom()) 036 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t1)); 037 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent root = tgt.getGroup(); 038 root.setTitle(src.getTitle()); 039 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) { 040 root.addConcept(Coding10_30.convertCoding(t)); 041 } 042 for (org.hl7.fhir.dstu3.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 043 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 044 if (t.getType() == org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.GROUP) 045 root.addGroup(convertQuestionnaireGroupComponent(t)); 046 else 047 root.addQuestion(convertQuestionnaireQuestionComponent(t)); 048 return tgt; 049 } 050 051 public static org.hl7.fhir.dstu3.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu2.model.Questionnaire src) throws FHIRException { 052 if (src == null || src.isEmpty()) 053 return null; 054 org.hl7.fhir.dstu3.model.Questionnaire tgt = new org.hl7.fhir.dstu3.model.Questionnaire(); 055 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 056 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 057 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 058 if (src.hasVersionElement()) 059 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 060 if (src.hasStatus()) 061 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 062 if (src.hasDate()) 063 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 064 if (src.hasPublisherElement()) 065 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 066 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 067 tgt.addContact(convertQuestionnaireContactComponent(t)); 068 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent root = src.getGroup(); 069 tgt.setTitle(root.getTitle()); 070 for (org.hl7.fhir.dstu2.model.Coding t : root.getConcept()) tgt.addCode(Coding10_30.convertCoding(t)); 071 for (org.hl7.fhir.dstu2.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 072 tgt.addItem(convertQuestionnaireGroupComponent(root)); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.dstu3.model.ContactDetail convertQuestionnaireContactComponent(org.hl7.fhir.dstu2.model.ContactPoint src) throws FHIRException { 077 if (src == null || src.isEmpty()) 078 return null; 079 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 080 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 081 tgt.addTelecom(ContactPoint10_30.convertContactPoint(src)); 082 return tgt; 083 } 084 085 public static org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent convertQuestionnaireGroupComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 086 if (src == null || src.isEmpty()) 087 return null; 088 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent tgt = new org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent(); 089 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 090 if (src.hasLinkIdElement()) 091 tgt.setLinkIdElement(String10_30.convertString(src.getLinkIdElement())); 092 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addConcept(Coding10_30.convertCoding(t)); 093 if (src.hasTextElement()) 094 tgt.setTextElement(String10_30.convertString(src.getTextElement())); 095 if (src.hasRequiredElement()) 096 tgt.setRequiredElement(Boolean10_30.convertBoolean(src.getRequiredElement())); 097 if (src.hasRepeatsElement()) 098 tgt.setRepeatsElement(Boolean10_30.convertBoolean(src.getRepeatsElement())); 099 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 100 if (t.getType() == org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.GROUP) 101 tgt.addGroup(convertQuestionnaireGroupComponent(t)); 102 else 103 tgt.addQuestion(convertQuestionnaireQuestionComponent(t)); 104 return tgt; 105 } 106 107 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireGroupComponent(org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent src) throws FHIRException { 108 if (src == null || src.isEmpty()) 109 return null; 110 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent(); 111 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 112 if (src.hasLinkIdElement()) 113 tgt.setLinkIdElement(String10_30.convertString(src.getLinkIdElement())); 114 for (org.hl7.fhir.dstu2.model.Coding t : src.getConcept()) tgt.addCode(Coding10_30.convertCoding(t)); 115 if (src.hasTextElement()) 116 tgt.setTextElement(String10_30.convertString(src.getTextElement())); 117 tgt.setType(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.GROUP); 118 if (src.hasRequiredElement()) 119 tgt.setRequiredElement(Boolean10_30.convertBoolean(src.getRequiredElement())); 120 if (src.hasRepeatsElement()) 121 tgt.setRepeatsElement(Boolean10_30.convertBoolean(src.getRepeatsElement())); 122 for (org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent t : src.getGroup()) 123 tgt.addItem(convertQuestionnaireGroupComponent(t)); 124 for (org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent t : src.getQuestion()) 125 tgt.addItem(convertQuestionnaireQuestionComponent(t)); 126 return tgt; 127 } 128 129 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat> convertQuestionnaireItemType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 130 if (src == null || src.isEmpty()) 131 return null; 132 Enumeration<Questionnaire.AnswerFormat> tgt = new Enumeration<>(new Questionnaire.AnswerFormatEnumFactory()); 133 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 134 if (src.getValue() == null) { 135 tgt.setValue(null); 136 } else { 137 switch (src.getValue()) { 138 case BOOLEAN: 139 tgt.setValue(Questionnaire.AnswerFormat.BOOLEAN); 140 break; 141 case DECIMAL: 142 tgt.setValue(Questionnaire.AnswerFormat.DECIMAL); 143 break; 144 case INTEGER: 145 tgt.setValue(Questionnaire.AnswerFormat.INTEGER); 146 break; 147 case DATE: 148 tgt.setValue(Questionnaire.AnswerFormat.DATE); 149 break; 150 case DATETIME: 151 tgt.setValue(Questionnaire.AnswerFormat.DATETIME); 152 break; 153 case TIME: 154 tgt.setValue(Questionnaire.AnswerFormat.TIME); 155 break; 156 case STRING: 157 tgt.setValue(Questionnaire.AnswerFormat.STRING); 158 break; 159 case TEXT: 160 tgt.setValue(Questionnaire.AnswerFormat.TEXT); 161 break; 162 case URL: 163 tgt.setValue(Questionnaire.AnswerFormat.URL); 164 break; 165 case CHOICE: 166 tgt.setValue(Questionnaire.AnswerFormat.CHOICE); 167 break; 168 case OPENCHOICE: 169 tgt.setValue(Questionnaire.AnswerFormat.OPENCHOICE); 170 break; 171 case ATTACHMENT: 172 tgt.setValue(Questionnaire.AnswerFormat.ATTACHMENT); 173 break; 174 case REFERENCE: 175 tgt.setValue(Questionnaire.AnswerFormat.REFERENCE); 176 break; 177 case QUANTITY: 178 tgt.setValue(Questionnaire.AnswerFormat.QUANTITY); 179 break; 180 default: 181 tgt.setValue(Questionnaire.AnswerFormat.NULL); 182 break; 183 } 184 } 185 return tgt; 186 } 187 188 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireQuestionComponent(org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent src) throws FHIRException { 189 if (src == null || src.isEmpty()) 190 return null; 191 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent(); 192 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 193 if (src.hasLinkIdElement()) 194 tgt.setLinkIdElement(String10_30.convertString(src.getLinkIdElement())); 195 for (org.hl7.fhir.dstu2.model.Coding t : src.getConcept()) tgt.addCode(Coding10_30.convertCoding(t)); 196 if (src.hasTextElement()) 197 tgt.setTextElement(String10_30.convertString(src.getTextElement())); 198 if (src.hasType()) 199 tgt.setTypeElement(convertQuestionnaireQuestionType(src.getTypeElement())); 200 if (src.hasRequiredElement()) 201 tgt.setRequiredElement(Boolean10_30.convertBoolean(src.getRequiredElement())); 202 if (src.hasRepeatsElement()) 203 tgt.setRepeatsElement(Boolean10_30.convertBoolean(src.getRepeatsElement())); 204 if (src.hasOptions()) 205 tgt.setOptions(Reference10_30.convertReference(src.getOptions())); 206 for (org.hl7.fhir.dstu2.model.Coding t : src.getOption()) tgt.addOption().setValue(Coding10_30.convertCoding(t)); 207 for (org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent t : src.getGroup()) 208 tgt.addItem(convertQuestionnaireGroupComponent(t)); 209 return tgt; 210 } 211 212 public static org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent convertQuestionnaireQuestionComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 213 if (src == null || src.isEmpty()) 214 return null; 215 org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent tgt = new org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent(); 216 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 217 if (src.hasLinkIdElement()) 218 tgt.setLinkIdElement(String10_30.convertString(src.getLinkIdElement())); 219 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addConcept(Coding10_30.convertCoding(t)); 220 if (src.hasTextElement()) 221 tgt.setTextElement(String10_30.convertString(src.getTextElement())); 222 if (src.hasType()) 223 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 224 if (src.hasRequiredElement()) 225 tgt.setRequiredElement(Boolean10_30.convertBoolean(src.getRequiredElement())); 226 if (src.hasRepeatsElement()) 227 tgt.setRepeatsElement(Boolean10_30.convertBoolean(src.getRepeatsElement())); 228 if (src.hasOptions()) 229 tgt.setOptions(Reference10_30.convertReference(src.getOptions())); 230 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent t : src.getOption()) 231 if (t.hasValueCoding()) 232 try { 233 tgt.addOption(Coding10_30.convertCoding(t.getValueCoding())); 234 } catch (org.hl7.fhir.exceptions.FHIRException e) { 235 throw new FHIRException(e); 236 } 237 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 238 tgt.addGroup(convertQuestionnaireGroupComponent(t)); 239 return tgt; 240 } 241 242 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireQuestionType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat> src) throws FHIRException { 243 if (src == null || src.isEmpty()) 244 return null; 245 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 246 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 247 if (src.getValue() == null) { 248 tgt.setValue(null); 249 } else { 250 switch (src.getValue()) { 251 case BOOLEAN: 252 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.BOOLEAN); 253 break; 254 case DECIMAL: 255 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DECIMAL); 256 break; 257 case INTEGER: 258 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.INTEGER); 259 break; 260 case DATE: 261 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DATE); 262 break; 263 case DATETIME: 264 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DATETIME); 265 break; 266 case INSTANT: 267 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.DATETIME); 268 break; 269 case TIME: 270 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.TIME); 271 break; 272 case STRING: 273 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.STRING); 274 break; 275 case TEXT: 276 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.TEXT); 277 break; 278 case URL: 279 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.URL); 280 break; 281 case CHOICE: 282 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.CHOICE); 283 break; 284 case OPENCHOICE: 285 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.OPENCHOICE); 286 break; 287 case ATTACHMENT: 288 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.ATTACHMENT); 289 break; 290 case REFERENCE: 291 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.REFERENCE); 292 break; 293 case QUANTITY: 294 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.QUANTITY); 295 break; 296 default: 297 tgt.setValue(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType.NULL); 298 break; 299 } 300 } 301 return tgt; 302 } 303 304 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus> src) throws FHIRException { 305 if (src == null || src.isEmpty()) 306 return null; 307 Enumeration<Questionnaire.QuestionnaireStatus> tgt = new Enumeration<>(new Questionnaire.QuestionnaireStatusEnumFactory()); 308 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 309 if (src.getValue() == null) { 310 tgt.setValue(null); 311 } else { 312 switch (src.getValue()) { 313 case DRAFT: 314 tgt.setValue(Questionnaire.QuestionnaireStatus.DRAFT); 315 break; 316 case ACTIVE: 317 tgt.setValue(Questionnaire.QuestionnaireStatus.PUBLISHED); 318 break; 319 case RETIRED: 320 tgt.setValue(Questionnaire.QuestionnaireStatus.RETIRED); 321 break; 322 default: 323 tgt.setValue(Questionnaire.QuestionnaireStatus.NULL); 324 break; 325 } 326 } 327 return tgt; 328 } 329 330 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus> src) throws FHIRException { 331 if (src == null || src.isEmpty()) 332 return null; 333 org.hl7.fhir.dstu3.model.Enumeration<Enumerations.PublicationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new Enumerations.PublicationStatusEnumFactory()); 334 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 335 if (src.getValue() == null) { 336 tgt.setValue(null); 337 } else { 338 switch (src.getValue()) { 339 case DRAFT: 340 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 341 break; 342 case PUBLISHED: 343 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 344 break; 345 case RETIRED: 346 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 347 break; 348 default: 349 tgt.setValue(Enumerations.PublicationStatus.NULL); 350 break; 351 } 352 } 353 return tgt; 354 } 355}