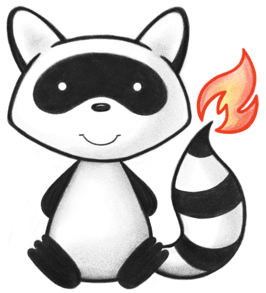
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Address10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Attachment10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.HumanName10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Period10_30; 012import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Date10_30; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class RelatedPerson10_30 { 016 017 public static org.hl7.fhir.dstu3.model.RelatedPerson convertRelatedPerson(org.hl7.fhir.dstu2.model.RelatedPerson src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.dstu3.model.RelatedPerson tgt = new org.hl7.fhir.dstu3.model.RelatedPerson(); 021 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 024 if (src.hasPatient()) 025 tgt.setPatient(Reference10_30.convertReference(src.getPatient())); 026 if (src.hasRelationship()) 027 tgt.setRelationship(CodeableConcept10_30.convertCodeableConcept(src.getRelationship())); 028 if (src.hasName()) 029 tgt.addName(HumanName10_30.convertHumanName(src.getName())); 030 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 031 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 032 if (src.hasGender()) 033 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 034 if (src.hasBirthDateElement()) 035 tgt.setBirthDateElement(Date10_30.convertDate(src.getBirthDateElement())); 036 for (org.hl7.fhir.dstu2.model.Address t : src.getAddress()) tgt.addAddress(Address10_30.convertAddress(t)); 037 for (org.hl7.fhir.dstu2.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_30.convertAttachment(t)); 038 if (src.hasPeriod()) 039 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 040 return tgt; 041 } 042 043 public static org.hl7.fhir.dstu2.model.RelatedPerson convertRelatedPerson(org.hl7.fhir.dstu3.model.RelatedPerson src) throws FHIRException { 044 if (src == null || src.isEmpty()) 045 return null; 046 org.hl7.fhir.dstu2.model.RelatedPerson tgt = new org.hl7.fhir.dstu2.model.RelatedPerson(); 047 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 048 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 049 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 050 if (src.hasPatient()) 051 tgt.setPatient(Reference10_30.convertReference(src.getPatient())); 052 if (src.hasRelationship()) 053 tgt.setRelationship(CodeableConcept10_30.convertCodeableConcept(src.getRelationship())); 054 if (!src.getName().isEmpty()) 055 tgt.setName(HumanName10_30.convertHumanName(src.getName().get(0))); 056 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 057 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 058 if (src.hasGender()) 059 tgt.setGenderElement(Enumerations10_30.convertAdministrativeGender(src.getGenderElement())); 060 if (src.hasBirthDateElement()) 061 tgt.setBirthDateElement(Date10_30.convertDate(src.getBirthDateElement())); 062 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) tgt.addAddress(Address10_30.convertAddress(t)); 063 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_30.convertAttachment(t)); 064 if (src.hasPeriod()) 065 tgt.setPeriod(Period10_30.convertPeriod(src.getPeriod())); 066 return tgt; 067 } 068}