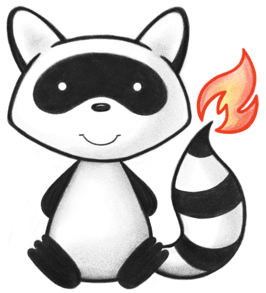
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.convertors.context.ConversionContext10_30; 007import org.hl7.fhir.convertors.conv10_30.VersionConvertor_10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.ElementDefinition10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Coding10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 012import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 013import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 014import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Code10_30; 015import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 016import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Id10_30; 017import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 018import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Uri10_30; 019import org.hl7.fhir.dstu3.model.Enumeration; 020import org.hl7.fhir.dstu3.model.StructureDefinition; 021import org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind; 022import org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule; 023import org.hl7.fhir.exceptions.FHIRException; 024import org.hl7.fhir.utilities.Utilities; 025 026public class StructureDefinition10_30 { 027 028 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext> convertExtensionContext(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext> src) throws FHIRException { 029 if (src == null || src.isEmpty()) 030 return null; 031 Enumeration<StructureDefinition.ExtensionContext> tgt = new Enumeration<>(new StructureDefinition.ExtensionContextEnumFactory()); 032 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 033 if (src.getValue() == null) { 034 tgt.setValue(null); 035 } else { 036 switch (src.getValue()) { 037 case RESOURCE: 038 tgt.setValue(StructureDefinition.ExtensionContext.RESOURCE); 039 break; 040 case DATATYPE: 041 tgt.setValue(StructureDefinition.ExtensionContext.DATATYPE); 042 break; 043 case EXTENSION: 044 tgt.setValue(StructureDefinition.ExtensionContext.EXTENSION); 045 break; 046 default: 047 tgt.setValue(StructureDefinition.ExtensionContext.NULL); 048 break; 049 } 050 } 051 return tgt; 052 } 053 054 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext> convertExtensionContext(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext> src) throws FHIRException { 055 if (src == null || src.isEmpty()) 056 return null; 057 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContextEnumFactory()); 058 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 059 if (src.getValue() == null) { 060 tgt.setValue(null); 061 } else { 062 switch (src.getValue()) { 063 case RESOURCE: 064 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.RESOURCE); 065 break; 066 case DATATYPE: 067 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.DATATYPE); 068 break; 069 case EXTENSION: 070 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.EXTENSION); 071 break; 072 default: 073 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.NULL); 074 break; 075 } 076 } 077 return tgt; 078 } 079 080 public static org.hl7.fhir.dstu2.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.dstu3.model.StructureDefinition src) throws FHIRException { 081 if (src == null || src.isEmpty()) 082 return null; 083 org.hl7.fhir.dstu2.model.StructureDefinition tgt = new org.hl7.fhir.dstu2.model.StructureDefinition(); 084 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 085 if (src.hasUrlElement()) 086 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 087 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 088 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 089 if (src.hasVersionElement()) 090 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 091 if (src.hasNameElement()) 092 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 093 if (src.hasTitleElement()) 094 tgt.setDisplayElement(String10_30.convertString(src.getTitleElement())); 095 if (src.hasStatus()) 096 tgt.setStatusElement(Enumerations10_30.convertConformanceResourceStatus(src.getStatusElement())); 097 if (src.hasExperimental()) 098 tgt.setExperimentalElement(Boolean10_30.convertBoolean(src.getExperimentalElement())); 099 if (src.hasPublisherElement()) 100 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 101 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 102 tgt.addContact(convertStructureDefinitionContactComponent(t)); 103 if (src.hasDate()) 104 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 105 if (src.hasDescription()) 106 tgt.setDescription(src.getDescription()); 107 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 108 if (t.hasValueCodeableConcept()) 109 tgt.addUseContext(CodeableConcept10_30.convertCodeableConcept(t.getValueCodeableConcept())); 110 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 111 tgt.addUseContext(CodeableConcept10_30.convertCodeableConcept(t)); 112 if (src.hasPurpose()) 113 tgt.setRequirements(src.getPurpose()); 114 if (src.hasCopyright()) 115 tgt.setCopyright(src.getCopyright()); 116 for (org.hl7.fhir.dstu3.model.Coding t : src.getKeyword()) tgt.addCode(Coding10_30.convertCoding(t)); 117 if (src.hasFhirVersionElement()) 118 tgt.setFhirVersionElement(Id10_30.convertId(src.getFhirVersionElement())); 119 for (org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 120 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 121 if (src.hasKind()) 122 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 123 if (src.hasAbstractElement()) 124 tgt.setAbstractElement(Boolean10_30.convertBoolean(src.getAbstractElement())); 125 if (src.hasContextType()) 126 tgt.setContextTypeElement(convertExtensionContext(src.getContextTypeElement())); 127 for (org.hl7.fhir.dstu3.model.StringType t : src.getContext()) tgt.addContext(t.getValue()); 128 if (src.hasTypeElement()) 129 tgt.setConstrainedTypeElement(Code10_30.convertCode(src.getTypeElement())); 130 if (src.hasBaseDefinitionElement()) 131 tgt.setBaseElement(Uri10_30.convertUri(src.getBaseDefinitionElement())); 132 if (src.hasSnapshot()) 133 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 134 if (src.hasDifferential()) 135 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 136 if (tgt.hasBase()) { 137 if (tgt.hasDifferential()) 138 tgt.getDifferential().getElement().get(0).addType().setCode(tail(tgt.getBase())); 139 if (tgt.hasSnapshot()) 140 tgt.getSnapshot().getElement().get(0).addType().setCode(tail(tgt.getBase())); 141 } 142 return tgt; 143 } 144 145 public static org.hl7.fhir.dstu3.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.dstu2.model.StructureDefinition src) throws FHIRException { 146 if (src == null || src.isEmpty()) 147 return null; 148 org.hl7.fhir.dstu3.model.StructureDefinition tgt = new org.hl7.fhir.dstu3.model.StructureDefinition(); 149 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 150 if (src.hasUrlElement()) 151 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 152 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 153 tgt.addIdentifier(Identifier10_30.convertIdentifier(t)); 154 if (src.hasVersionElement()) 155 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 156 if (src.hasNameElement()) 157 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 158 if (src.hasDisplayElement()) 159 tgt.setTitleElement(String10_30.convertString(src.getDisplayElement())); 160 if (src.hasStatus()) 161 tgt.setStatusElement(Enumerations10_30.convertConformanceResourceStatus(src.getStatusElement())); 162 if (src.hasExperimental()) 163 tgt.setExperimentalElement(Boolean10_30.convertBoolean(src.getExperimentalElement())); 164 if (src.hasPublisherElement()) 165 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 166 for (org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent t : src.getContact()) 167 tgt.addContact(convertStructureDefinitionContactComponent(t)); 168 if (src.hasDate()) 169 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 170 if (src.hasDescription()) 171 tgt.setDescription(src.getDescription()); 172 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 173 if (VersionConvertor_10_30.isJurisdiction(t)) 174 tgt.addJurisdiction(CodeableConcept10_30.convertCodeableConcept(t)); 175 else 176 tgt.addUseContext(CodeableConcept10_30.convertCodeableConceptToUsageContext(t)); 177 if (src.hasRequirements()) 178 tgt.setPurpose(src.getRequirements()); 179 if (src.hasCopyright()) 180 tgt.setCopyright(src.getCopyright()); 181 for (org.hl7.fhir.dstu2.model.Coding t : src.getCode()) tgt.addKeyword(Coding10_30.convertCoding(t)); 182 if (src.hasFhirVersionElement()) 183 tgt.setFhirVersionElement(Id10_30.convertId(src.getFhirVersionElement())); 184 for (org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 185 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 186 if (src.hasKind()) 187 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement(), tgt.getId())); 188 if (src.hasAbstractElement()) 189 tgt.setAbstractElement(Boolean10_30.convertBoolean(src.getAbstractElement())); 190 if (src.hasContextType()) 191 tgt.setContextTypeElement(convertExtensionContext(src.getContextTypeElement())); 192 for (org.hl7.fhir.dstu2.model.StringType t : src.getContext()) tgt.addContext(t.getValue()); 193 if (src.hasConstrainedType()) 194 tgt.setTypeElement(Code10_30.convertCode(src.getConstrainedTypeElement())); 195 else if (src.getSnapshot().hasElement()) 196 tgt.setType(src.getSnapshot().getElement().get(0).getPath()); 197 else if (src.getDifferential().hasElement() && !src.getDifferential().getElement().get(0).getPath().contains(".")) 198 tgt.setType(src.getDifferential().getElement().get(0).getPath()); 199 else 200 tgt.setType(src.getDifferential().getElement().get(0).getPath().substring(0, src.getDifferential().getElement().get(0).getPath().indexOf("."))); 201 if (src.hasBaseElement()) 202 tgt.setBaseDefinitionElement(Uri10_30.convertUri(src.getBaseElement())); 203 tgt.setDerivation(src.hasConstrainedType() ? org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule.CONSTRAINT : org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 204 if (src.hasSnapshot()) 205 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 206 if (src.hasDifferential()) 207 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 208 if (tgt.hasSnapshot()) 209 tgt.getSnapshot().getElementFirstRep().getType().clear(); 210 if (tgt.hasDifferential()) 211 tgt.getDifferential().getElementFirstRep().getType().clear(); 212 if (tgt.getKind() == StructureDefinitionKind.PRIMITIVETYPE && !tgt.getType().equals(tgt.getId())) { 213 tgt.setDerivation(TypeDerivationRule.SPECIALIZATION); 214 tgt.setBaseDefinition("http://hl7.org/fhir/StructureDefinition/" + tgt.getType()); 215 tgt.setType(tgt.getId()); 216 } 217 return tgt; 218 } 219 220 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent convertStructureDefinitionContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 221 if (src == null || src.isEmpty()) 222 return null; 223 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent(); 224 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 225 if (src.hasNameElement()) 226 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 227 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 228 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 229 return tgt; 230 } 231 232 public static org.hl7.fhir.dstu3.model.ContactDetail convertStructureDefinitionContactComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent src) throws FHIRException { 233 if (src == null || src.isEmpty()) 234 return null; 235 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 236 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 237 if (src.hasNameElement()) 238 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 239 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 240 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 241 return tgt; 242 } 243 244 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 245 if (src == null || src.isEmpty()) 246 return null; 247 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 248 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 249 for (org.hl7.fhir.dstu3.model.ElementDefinition t : src.getElement()) 250 tgt.addElement(ElementDefinition10_30.convertElementDefinition(t)); 251 return tgt; 252 } 253 254 public static org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 255 if (src == null || src.isEmpty()) 256 return null; 257 org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 258 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 259 List<String> slicePaths = new ArrayList<String>(); 260 for (org.hl7.fhir.dstu2.model.ElementDefinition t : src.getElement()) { 261 if (t.hasSlicing()) 262 slicePaths.add(t.getPath()); 263 tgt.addElement(ElementDefinition10_30.convertElementDefinition(t, slicePaths)); 264 } 265 return tgt; 266 } 267 268 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 269 if (src == null || src.isEmpty()) 270 return null; 271 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 272 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 273 if (src.getValue() == null) { 274 tgt.setValue(null); 275 } else { 276 switch (src.getValue()) { 277 case PRIMITIVETYPE: 278 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 279 break; 280 case COMPLEXTYPE: 281 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 282 break; 283 case RESOURCE: 284 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 285 break; 286 case LOGICAL: 287 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 288 break; 289 default: 290 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.NULL); 291 break; 292 } 293 } 294 return tgt; 295 } 296 297 public static org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind> src, String dtName) throws FHIRException { 298 if (src == null || src.isEmpty()) 299 return null; 300 Enumeration<StructureDefinitionKind> tgt = new Enumeration<>(new StructureDefinition.StructureDefinitionKindEnumFactory()); 301 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 302 if (src.getValue() == null) { 303 tgt.setValue(null); 304 } else { 305 switch (src.getValue()) { 306 case DATATYPE: 307 if (Utilities.existsInList(dtName, "boolean", "integer", "decimal", "base64Binary", "instant", 308 "string", "uri", "date", "dateTime", "time", "code", "oid", "uuid", "id", "unsignedInt", 309 "positiveInt", "markdown", "xhtml", "url", "canonical")) 310 tgt.setValue(StructureDefinitionKind.PRIMITIVETYPE); 311 else 312 tgt.setValue(StructureDefinitionKind.COMPLEXTYPE); 313 break; 314 case RESOURCE: 315 tgt.setValue(StructureDefinitionKind.RESOURCE); 316 break; 317 case LOGICAL: 318 tgt.setValue(StructureDefinitionKind.LOGICAL); 319 break; 320 default: 321 tgt.setValue(StructureDefinitionKind.NULL); 322 break; 323 } 324 } 325 return tgt; 326 } 327 328 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 329 if (src == null || src.isEmpty()) 330 return null; 331 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent(); 332 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 333 if (src.hasIdentityElement()) 334 tgt.setIdentityElement(Id10_30.convertId(src.getIdentityElement())); 335 if (src.hasUriElement()) 336 tgt.setUriElement(Uri10_30.convertUri(src.getUriElement())); 337 if (src.hasNameElement()) 338 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 339 if (src.hasCommentElement()) 340 tgt.setCommentsElement(String10_30.convertString(src.getCommentElement())); 341 return tgt; 342 } 343 344 public static org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 345 if (src == null || src.isEmpty()) 346 return null; 347 org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent(); 348 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 349 if (src.hasIdentityElement()) 350 tgt.setIdentityElement(Id10_30.convertId(src.getIdentityElement())); 351 if (src.hasUriElement()) 352 tgt.setUriElement(Uri10_30.convertUri(src.getUriElement())); 353 if (src.hasNameElement()) 354 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 355 if (src.hasCommentsElement()) 356 tgt.setCommentElement(String10_30.convertString(src.getCommentsElement())); 357 return tgt; 358 } 359 360 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 361 if (src == null || src.isEmpty()) 362 return null; 363 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 364 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 365 for (org.hl7.fhir.dstu3.model.ElementDefinition t : src.getElement()) 366 tgt.addElement(ElementDefinition10_30.convertElementDefinition(t)); 367 return tgt; 368 } 369 370 public static org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 371 if (src == null || src.isEmpty()) 372 return null; 373 org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 374 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 375 List<String> slicePaths = new ArrayList<String>(); 376 for (org.hl7.fhir.dstu2.model.ElementDefinition t : src.getElement()) { 377 if (t.hasSlicing()) 378 slicePaths.add(t.getPath()); 379 tgt.addElement(ElementDefinition10_30.convertElementDefinition(t, slicePaths)); 380 } 381 return tgt; 382 } 383 384 static public String tail(String base) { 385 return base.substring(base.lastIndexOf("/") + 1); 386 } 387}