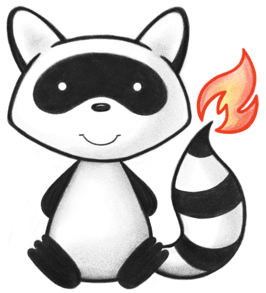
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_30; 004import org.hl7.fhir.convertors.conv10_30.VersionConvertor_10_30; 005import org.hl7.fhir.convertors.conv10_30.datatypes10_30.Reference10_30; 006import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 007import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Coding10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Code10_30; 012import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 013import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Id10_30; 014import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Integer10_30; 015import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 016import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Uri10_30; 017import org.hl7.fhir.dstu2.model.Enumeration; 018import org.hl7.fhir.dstu2.model.TestScript; 019import org.hl7.fhir.exceptions.FHIRException; 020 021public class TestScript10_30 { 022 023 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> src) throws FHIRException { 024 if (src == null || src.isEmpty()) 025 return null; 026 Enumeration<TestScript.AssertionDirectionType> tgt = new Enumeration<>(new TestScript.AssertionDirectionTypeEnumFactory()); 027 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 028 if (src.getValue() == null) { 029 tgt.setValue(null); 030 } else { 031 switch (src.getValue()) { 032 case RESPONSE: 033 tgt.setValue(TestScript.AssertionDirectionType.RESPONSE); 034 break; 035 case REQUEST: 036 tgt.setValue(TestScript.AssertionDirectionType.REQUEST); 037 break; 038 default: 039 tgt.setValue(TestScript.AssertionDirectionType.NULL); 040 break; 041 } 042 } 043 return tgt; 044 } 045 046 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionDirectionType> src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionTypeEnumFactory()); 050 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 051 if (src.getValue() == null) { 052 tgt.setValue(null); 053 } else { 054 switch (src.getValue()) { 055 case RESPONSE: 056 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.RESPONSE); 057 break; 058 case REQUEST: 059 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.REQUEST); 060 break; 061 default: 062 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.NULL); 063 break; 064 } 065 } 066 return tgt; 067 } 068 069 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionOperatorType> src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorTypeEnumFactory()); 073 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 074 if (src.getValue() == null) { 075 tgt.setValue(null); 076 } else { 077 switch (src.getValue()) { 078 case EQUALS: 079 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EQUALS); 080 break; 081 case NOTEQUALS: 082 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTEQUALS); 083 break; 084 case IN: 085 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.IN); 086 break; 087 case NOTIN: 088 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTIN); 089 break; 090 case GREATERTHAN: 091 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.GREATERTHAN); 092 break; 093 case LESSTHAN: 094 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.LESSTHAN); 095 break; 096 case EMPTY: 097 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EMPTY); 098 break; 099 case NOTEMPTY: 100 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTEMPTY); 101 break; 102 case CONTAINS: 103 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.CONTAINS); 104 break; 105 case NOTCONTAINS: 106 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTCONTAINS); 107 break; 108 default: 109 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NULL); 110 break; 111 } 112 } 113 return tgt; 114 } 115 116 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 Enumeration<TestScript.AssertionOperatorType> tgt = new Enumeration<>(new TestScript.AssertionOperatorTypeEnumFactory()); 120 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 121 if (src.getValue() == null) { 122 tgt.setValue(null); 123 } else { 124 switch (src.getValue()) { 125 case EQUALS: 126 tgt.setValue(TestScript.AssertionOperatorType.EQUALS); 127 break; 128 case NOTEQUALS: 129 tgt.setValue(TestScript.AssertionOperatorType.NOTEQUALS); 130 break; 131 case IN: 132 tgt.setValue(TestScript.AssertionOperatorType.IN); 133 break; 134 case NOTIN: 135 tgt.setValue(TestScript.AssertionOperatorType.NOTIN); 136 break; 137 case GREATERTHAN: 138 tgt.setValue(TestScript.AssertionOperatorType.GREATERTHAN); 139 break; 140 case LESSTHAN: 141 tgt.setValue(TestScript.AssertionOperatorType.LESSTHAN); 142 break; 143 case EMPTY: 144 tgt.setValue(TestScript.AssertionOperatorType.EMPTY); 145 break; 146 case NOTEMPTY: 147 tgt.setValue(TestScript.AssertionOperatorType.NOTEMPTY); 148 break; 149 case CONTAINS: 150 tgt.setValue(TestScript.AssertionOperatorType.CONTAINS); 151 break; 152 case NOTCONTAINS: 153 tgt.setValue(TestScript.AssertionOperatorType.NOTCONTAINS); 154 break; 155 default: 156 tgt.setValue(TestScript.AssertionOperatorType.NULL); 157 break; 158 } 159 } 160 return tgt; 161 } 162 163 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 164 if (src == null || src.isEmpty()) 165 return null; 166 Enumeration<TestScript.AssertionResponseTypes> tgt = new Enumeration<>(new TestScript.AssertionResponseTypesEnumFactory()); 167 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 168 if (src.getValue() == null) { 169 tgt.setValue(null); 170 } else { 171 switch (src.getValue()) { 172 case OKAY: 173 tgt.setValue(TestScript.AssertionResponseTypes.OKAY); 174 break; 175 case CREATED: 176 tgt.setValue(TestScript.AssertionResponseTypes.CREATED); 177 break; 178 case NOCONTENT: 179 tgt.setValue(TestScript.AssertionResponseTypes.NOCONTENT); 180 break; 181 case NOTMODIFIED: 182 tgt.setValue(TestScript.AssertionResponseTypes.NOTMODIFIED); 183 break; 184 case BAD: 185 tgt.setValue(TestScript.AssertionResponseTypes.BAD); 186 break; 187 case FORBIDDEN: 188 tgt.setValue(TestScript.AssertionResponseTypes.FORBIDDEN); 189 break; 190 case NOTFOUND: 191 tgt.setValue(TestScript.AssertionResponseTypes.NOTFOUND); 192 break; 193 case METHODNOTALLOWED: 194 tgt.setValue(TestScript.AssertionResponseTypes.METHODNOTALLOWED); 195 break; 196 case CONFLICT: 197 tgt.setValue(TestScript.AssertionResponseTypes.CONFLICT); 198 break; 199 case GONE: 200 tgt.setValue(TestScript.AssertionResponseTypes.GONE); 201 break; 202 case PRECONDITIONFAILED: 203 tgt.setValue(TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 204 break; 205 case UNPROCESSABLE: 206 tgt.setValue(TestScript.AssertionResponseTypes.UNPROCESSABLE); 207 break; 208 default: 209 tgt.setValue(TestScript.AssertionResponseTypes.NULL); 210 break; 211 } 212 } 213 return tgt; 214 } 215 216 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 217 if (src == null || src.isEmpty()) 218 return null; 219 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypesEnumFactory()); 220 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 221 if (src.getValue() == null) { 222 tgt.setValue(null); 223 } else { 224 switch (src.getValue()) { 225 case OKAY: 226 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.OKAY); 227 break; 228 case CREATED: 229 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.CREATED); 230 break; 231 case NOCONTENT: 232 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOCONTENT); 233 break; 234 case NOTMODIFIED: 235 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 236 break; 237 case BAD: 238 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.BAD); 239 break; 240 case FORBIDDEN: 241 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.FORBIDDEN); 242 break; 243 case NOTFOUND: 244 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOTFOUND); 245 break; 246 case METHODNOTALLOWED: 247 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 248 break; 249 case CONFLICT: 250 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.CONFLICT); 251 break; 252 case GONE: 253 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.GONE); 254 break; 255 case PRECONDITIONFAILED: 256 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 257 break; 258 case UNPROCESSABLE: 259 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.UNPROCESSABLE); 260 break; 261 default: 262 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NULL); 263 break; 264 } 265 } 266 return tgt; 267 } 268 269 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.ContentType> convertContentType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.ContentType> src) throws FHIRException { 270 if (src == null || src.isEmpty()) 271 return null; 272 Enumeration<TestScript.ContentType> tgt = new Enumeration<>(new TestScript.ContentTypeEnumFactory()); 273 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 274 if (src.getValue() == null) { 275 tgt.setValue(null); 276 } else { 277 switch (src.getValue()) { 278 case XML: 279 tgt.setValue(TestScript.ContentType.XML); 280 break; 281 case JSON: 282 tgt.setValue(TestScript.ContentType.JSON); 283 break; 284 default: 285 tgt.setValue(TestScript.ContentType.NULL); 286 break; 287 } 288 } 289 return tgt; 290 } 291 292 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.ContentType> convertContentType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.ContentType> src) throws FHIRException { 293 if (src == null || src.isEmpty()) 294 return null; 295 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.ContentType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.ContentTypeEnumFactory()); 296 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 297 if (src.getValue() == null) { 298 tgt.setValue(null); 299 } else { 300 switch (src.getValue()) { 301 case XML: 302 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.ContentType.XML); 303 break; 304 case JSON: 305 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.ContentType.JSON); 306 break; 307 default: 308 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.ContentType.NULL); 309 break; 310 } 311 } 312 return tgt; 313 } 314 315 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent src) throws FHIRException { 316 if (src == null || src.isEmpty()) 317 return null; 318 org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent(); 319 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 320 if (src.hasLabelElement()) 321 tgt.setLabelElement(String10_30.convertString(src.getLabelElement())); 322 if (src.hasDescriptionElement()) 323 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 324 if (src.hasDirection()) 325 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 326 if (src.hasCompareToSourceIdElement()) 327 tgt.setCompareToSourceIdElement(String10_30.convertString(src.getCompareToSourceIdElement())); 328 if (src.hasCompareToSourcePathElement()) 329 tgt.setCompareToSourcePathElement(String10_30.convertString(src.getCompareToSourcePathElement())); 330 if (src.hasContentType()) 331 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 332 if (src.hasHeaderFieldElement()) 333 tgt.setHeaderFieldElement(String10_30.convertString(src.getHeaderFieldElement())); 334 if (src.hasMinimumIdElement()) 335 tgt.setMinimumIdElement(String10_30.convertString(src.getMinimumIdElement())); 336 if (src.hasNavigationLinksElement()) 337 tgt.setNavigationLinksElement(Boolean10_30.convertBoolean(src.getNavigationLinksElement())); 338 if (src.hasOperator()) 339 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 340 if (src.hasPathElement()) 341 tgt.setPathElement(String10_30.convertString(src.getPathElement())); 342 if (src.hasResourceElement()) 343 tgt.setResourceElement(Code10_30.convertCode(src.getResourceElement())); 344 if (src.hasResponse()) 345 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 346 if (src.hasResponseCodeElement()) 347 tgt.setResponseCodeElement(String10_30.convertString(src.getResponseCodeElement())); 348 if (src.hasSourceIdElement()) 349 tgt.setSourceIdElement(Id10_30.convertId(src.getSourceIdElement())); 350 if (src.hasValidateProfileIdElement()) 351 tgt.setValidateProfileIdElement(Id10_30.convertId(src.getValidateProfileIdElement())); 352 if (src.hasValueElement()) 353 tgt.setValueElement(String10_30.convertString(src.getValueElement())); 354 if (src.hasWarningOnlyElement()) 355 tgt.setWarningOnlyElement(Boolean10_30.convertBoolean(src.getWarningOnlyElement())); 356 return tgt; 357 } 358 359 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 360 if (src == null || src.isEmpty()) 361 return null; 362 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent(); 363 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 364 if (src.hasLabelElement()) 365 tgt.setLabelElement(String10_30.convertString(src.getLabelElement())); 366 if (src.hasDescriptionElement()) 367 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 368 if (src.hasDirection()) 369 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 370 if (src.hasCompareToSourceIdElement()) 371 tgt.setCompareToSourceIdElement(String10_30.convertString(src.getCompareToSourceIdElement())); 372 if (src.hasCompareToSourcePathElement()) 373 tgt.setCompareToSourcePathElement(String10_30.convertString(src.getCompareToSourcePathElement())); 374 if (src.hasContentType()) 375 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 376 if (src.hasHeaderFieldElement()) 377 tgt.setHeaderFieldElement(String10_30.convertString(src.getHeaderFieldElement())); 378 if (src.hasMinimumIdElement()) 379 tgt.setMinimumIdElement(String10_30.convertString(src.getMinimumIdElement())); 380 if (src.hasNavigationLinksElement()) 381 tgt.setNavigationLinksElement(Boolean10_30.convertBoolean(src.getNavigationLinksElement())); 382 if (src.hasOperator()) 383 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 384 if (src.hasPathElement()) 385 tgt.setPathElement(String10_30.convertString(src.getPathElement())); 386 if (src.hasResourceElement()) 387 tgt.setResourceElement(Code10_30.convertCode(src.getResourceElement())); 388 if (src.hasResponse()) 389 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 390 if (src.hasResponseCodeElement()) 391 tgt.setResponseCodeElement(String10_30.convertString(src.getResponseCodeElement())); 392 if (src.hasSourceIdElement()) 393 tgt.setSourceIdElement(Id10_30.convertId(src.getSourceIdElement())); 394 if (src.hasValidateProfileIdElement()) 395 tgt.setValidateProfileIdElement(Id10_30.convertId(src.getValidateProfileIdElement())); 396 if (src.hasValueElement()) 397 tgt.setValueElement(String10_30.convertString(src.getValueElement())); 398 if (src.hasWarningOnlyElement()) 399 tgt.setWarningOnlyElement(Boolean10_30.convertBoolean(src.getWarningOnlyElement())); 400 return tgt; 401 } 402 403 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent src) throws FHIRException { 404 if (src == null || src.isEmpty()) 405 return null; 406 org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent(); 407 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 408 if (src.hasOperation()) 409 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 410 if (src.hasAssert()) 411 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 412 return tgt; 413 } 414 415 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent src) throws FHIRException { 416 if (src == null || src.isEmpty()) 417 return null; 418 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent(); 419 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 420 if (src.hasOperation()) 421 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 422 if (src.hasAssert()) 423 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 424 return tgt; 425 } 426 427 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 428 if (src == null || src.isEmpty()) 429 return null; 430 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent(); 431 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 432 if (src.hasType()) 433 tgt.setType(Coding10_30.convertCoding(src.getType())); 434 if (src.hasResourceElement()) 435 tgt.setResourceElement(Code10_30.convertCode(src.getResourceElement())); 436 if (src.hasLabelElement()) 437 tgt.setLabelElement(String10_30.convertString(src.getLabelElement())); 438 if (src.hasDescriptionElement()) 439 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 440 if (src.hasAccept()) 441 tgt.setAcceptElement(convertContentType(src.getAcceptElement())); 442 if (src.hasContentType()) 443 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 444 if (src.hasDestinationElement()) 445 tgt.setDestinationElement(Integer10_30.convertInteger(src.getDestinationElement())); 446 if (src.hasEncodeRequestUrlElement()) 447 tgt.setEncodeRequestUrlElement(Boolean10_30.convertBoolean(src.getEncodeRequestUrlElement())); 448 if (src.hasParamsElement()) 449 tgt.setParamsElement(String10_30.convertString(src.getParamsElement())); 450 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 451 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 452 if (src.hasResponseIdElement()) 453 tgt.setResponseIdElement(Id10_30.convertId(src.getResponseIdElement())); 454 if (src.hasSourceIdElement()) 455 tgt.setSourceIdElement(Id10_30.convertId(src.getSourceIdElement())); 456 if (src.hasTargetId()) 457 tgt.setTargetId(src.getTargetId()); 458 if (src.hasUrlElement()) 459 tgt.setUrlElement(String10_30.convertString(src.getUrlElement())); 460 return tgt; 461 } 462 463 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent src) throws FHIRException { 464 if (src == null || src.isEmpty()) 465 return null; 466 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent(); 467 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 468 if (src.hasType()) 469 tgt.setType(Coding10_30.convertCoding(src.getType())); 470 if (src.hasResourceElement()) 471 tgt.setResourceElement(Code10_30.convertCode(src.getResourceElement())); 472 if (src.hasLabelElement()) 473 tgt.setLabelElement(String10_30.convertString(src.getLabelElement())); 474 if (src.hasDescriptionElement()) 475 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 476 if (src.hasAccept()) 477 tgt.setAcceptElement(convertContentType(src.getAcceptElement())); 478 if (src.hasContentType()) 479 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 480 if (src.hasDestinationElement()) 481 tgt.setDestinationElement(Integer10_30.convertInteger(src.getDestinationElement())); 482 if (src.hasEncodeRequestUrlElement()) 483 tgt.setEncodeRequestUrlElement(Boolean10_30.convertBoolean(src.getEncodeRequestUrlElement())); 484 if (src.hasParamsElement()) 485 tgt.setParamsElement(String10_30.convertString(src.getParamsElement())); 486 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 487 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 488 if (src.hasResponseIdElement()) 489 tgt.setResponseIdElement(Id10_30.convertId(src.getResponseIdElement())); 490 if (src.hasSourceIdElement()) 491 tgt.setSourceIdElement(Id10_30.convertId(src.getSourceIdElement())); 492 if (src.hasTargetId()) 493 tgt.setTargetId(src.getTargetId()); 494 if (src.hasUrlElement()) 495 tgt.setUrlElement(String10_30.convertString(src.getUrlElement())); 496 return tgt; 497 } 498 499 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent src) throws FHIRException { 500 if (src == null || src.isEmpty()) 501 return null; 502 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent(); 503 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 504 if (src.hasFieldElement()) 505 tgt.setFieldElement(String10_30.convertString(src.getFieldElement())); 506 if (src.hasValueElement()) 507 tgt.setValueElement(String10_30.convertString(src.getValueElement())); 508 return tgt; 509 } 510 511 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 512 if (src == null || src.isEmpty()) 513 return null; 514 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent(); 515 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 516 if (src.hasFieldElement()) 517 tgt.setFieldElement(String10_30.convertString(src.getFieldElement())); 518 if (src.hasValueElement()) 519 tgt.setValueElement(String10_30.convertString(src.getValueElement())); 520 return tgt; 521 } 522 523 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent src) throws FHIRException { 524 if (src == null || src.isEmpty()) 525 return null; 526 org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent(); 527 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 528 if (src.hasOperation()) 529 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 530 return tgt; 531 } 532 533 public static org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent src) throws FHIRException { 534 if (src == null || src.isEmpty()) 535 return null; 536 org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent(); 537 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 538 if (src.hasOperation()) 539 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 540 return tgt; 541 } 542 543 public static org.hl7.fhir.dstu3.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent src) throws FHIRException { 544 if (src == null || src.isEmpty()) 545 return null; 546 org.hl7.fhir.dstu3.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestActionComponent(); 547 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 548 if (src.hasOperation()) 549 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 550 if (src.hasAssert()) 551 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 552 return tgt; 553 } 554 555 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent convertTestActionComponent(org.hl7.fhir.dstu3.model.TestScript.TestActionComponent src) throws FHIRException { 556 if (src == null || src.isEmpty()) 557 return null; 558 org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent(); 559 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 560 if (src.hasOperation()) 561 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 562 if (src.hasAssert()) 563 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 564 return tgt; 565 } 566 567 public static org.hl7.fhir.dstu2.model.TestScript convertTestScript(org.hl7.fhir.dstu3.model.TestScript src) throws FHIRException { 568 if (src == null || src.isEmpty()) 569 return null; 570 org.hl7.fhir.dstu2.model.TestScript tgt = new org.hl7.fhir.dstu2.model.TestScript(); 571 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 572 if (src.hasUrlElement()) 573 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 574 if (src.hasVersionElement()) 575 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 576 if (src.hasNameElement()) 577 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 578 if (src.hasStatus()) 579 tgt.setStatusElement(Enumerations10_30.convertConformanceResourceStatus(src.getStatusElement())); 580 if (src.hasIdentifier()) 581 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 582 if (src.hasExperimental()) 583 tgt.setExperimentalElement(Boolean10_30.convertBoolean(src.getExperimentalElement())); 584 if (src.hasPublisherElement()) 585 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 586 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 587 tgt.addContact(convertTestScriptContactComponent(t)); 588 if (src.hasDate()) 589 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 590 if (src.hasDescription()) 591 tgt.setDescription(src.getDescription()); 592 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 593 if (t.hasValueCodeableConcept()) 594 tgt.addUseContext(CodeableConcept10_30.convertCodeableConcept(t.getValueCodeableConcept())); 595 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 596 tgt.addUseContext(CodeableConcept10_30.convertCodeableConcept(t)); 597 if (src.hasPurpose()) 598 tgt.setRequirements(src.getPurpose()); 599 if (src.hasCopyright()) 600 tgt.setCopyright(src.getCopyright()); 601 if (src.hasMetadata()) 602 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 603 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 604 tgt.addFixture(convertTestScriptFixtureComponent(t)); 605 for (org.hl7.fhir.dstu3.model.Reference t : src.getProfile()) tgt.addProfile(Reference10_30.convertReference(t)); 606 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 607 tgt.addVariable(convertTestScriptVariableComponent(t)); 608 if (src.hasSetup()) 609 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 610 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent t : src.getTest()) 611 tgt.addTest(convertTestScriptTestComponent(t)); 612 if (src.hasTeardown()) 613 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 614 return tgt; 615 } 616 617 public static org.hl7.fhir.dstu3.model.TestScript convertTestScript(org.hl7.fhir.dstu2.model.TestScript src) throws FHIRException { 618 if (src == null || src.isEmpty()) 619 return null; 620 org.hl7.fhir.dstu3.model.TestScript tgt = new org.hl7.fhir.dstu3.model.TestScript(); 621 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 622 if (src.hasUrlElement()) 623 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 624 if (src.hasVersionElement()) 625 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 626 if (src.hasNameElement()) 627 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 628 if (src.hasStatus()) 629 tgt.setStatusElement(Enumerations10_30.convertConformanceResourceStatus(src.getStatusElement())); 630 if (src.hasIdentifier()) 631 tgt.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 632 if (src.hasExperimental()) 633 tgt.setExperimentalElement(Boolean10_30.convertBoolean(src.getExperimentalElement())); 634 if (src.hasPublisherElement()) 635 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 636 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent t : src.getContact()) 637 tgt.addContact(convertTestScriptContactComponent(t)); 638 if (src.hasDate()) 639 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 640 if (src.hasDescription()) 641 tgt.setDescription(src.getDescription()); 642 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 643 if (VersionConvertor_10_30.isJurisdiction(t)) 644 tgt.addJurisdiction(CodeableConcept10_30.convertCodeableConcept(t)); 645 else 646 tgt.addUseContext(CodeableConcept10_30.convertCodeableConceptToUsageContext(t)); 647 if (src.hasRequirements()) 648 tgt.setPurpose(src.getRequirements()); 649 if (src.hasCopyright()) 650 tgt.setCopyright(src.getCopyright()); 651 if (src.hasMetadata()) 652 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 653 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 654 tgt.addFixture(convertTestScriptFixtureComponent(t)); 655 for (org.hl7.fhir.dstu2.model.Reference t : src.getProfile()) tgt.addProfile(Reference10_30.convertReference(t)); 656 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 657 tgt.addVariable(convertTestScriptVariableComponent(t)); 658 if (src.hasSetup()) 659 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 660 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent t : src.getTest()) 661 tgt.addTest(convertTestScriptTestComponent(t)); 662 if (src.hasTeardown()) 663 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 664 return tgt; 665 } 666 667 public static org.hl7.fhir.dstu3.model.ContactDetail convertTestScriptContactComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent src) throws FHIRException { 668 if (src == null || src.isEmpty()) 669 return null; 670 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 671 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 672 if (src.hasNameElement()) 673 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 674 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 675 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 676 return tgt; 677 } 678 679 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent convertTestScriptContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 680 if (src == null || src.isEmpty()) 681 return null; 682 org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent(); 683 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 684 if (src.hasNameElement()) 685 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 686 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 687 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 688 return tgt; 689 } 690 691 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 692 if (src == null || src.isEmpty()) 693 return null; 694 org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent(); 695 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 696 if (src.hasAutocreateElement()) 697 tgt.setAutocreateElement(Boolean10_30.convertBoolean(src.getAutocreateElement())); 698 if (src.hasAutodeleteElement()) 699 tgt.setAutodeleteElement(Boolean10_30.convertBoolean(src.getAutodeleteElement())); 700 if (src.hasResource()) 701 tgt.setResource(Reference10_30.convertReference(src.getResource())); 702 return tgt; 703 } 704 705 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 706 if (src == null || src.isEmpty()) 707 return null; 708 org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent(); 709 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 710 if (src.hasAutocreateElement()) 711 tgt.setAutocreateElement(Boolean10_30.convertBoolean(src.getAutocreateElement())); 712 if (src.hasAutodeleteElement()) 713 tgt.setAutodeleteElement(Boolean10_30.convertBoolean(src.getAutodeleteElement())); 714 if (src.hasResource()) 715 tgt.setResource(Reference10_30.convertReference(src.getResource())); 716 return tgt; 717 } 718 719 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 720 if (src == null || src.isEmpty()) 721 return null; 722 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent(); 723 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 724 if (src.hasRequiredElement()) 725 tgt.setRequiredElement(Boolean10_30.convertBoolean(src.getRequiredElement())); 726 if (src.hasValidatedElement()) 727 tgt.setValidatedElement(Boolean10_30.convertBoolean(src.getValidatedElement())); 728 if (src.hasDescriptionElement()) 729 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 730 if (src.hasDestinationElement()) 731 tgt.setDestinationElement(Integer10_30.convertInteger(src.getDestinationElement())); 732 for (org.hl7.fhir.dstu3.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 733 if (src.hasCapabilities()) 734 tgt.setConformance(Reference10_30.convertReference(src.getCapabilities())); 735 return tgt; 736 } 737 738 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 739 if (src == null || src.isEmpty()) 740 return null; 741 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent(); 742 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 743 if (src.hasRequiredElement()) 744 tgt.setRequiredElement(Boolean10_30.convertBoolean(src.getRequiredElement())); 745 if (src.hasValidatedElement()) 746 tgt.setValidatedElement(Boolean10_30.convertBoolean(src.getValidatedElement())); 747 if (src.hasDescriptionElement()) 748 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 749 if (src.hasDestinationElement()) 750 tgt.setDestinationElement(Integer10_30.convertInteger(src.getDestinationElement())); 751 for (org.hl7.fhir.dstu2.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 752 if (src.hasConformance()) 753 tgt.setCapabilities(Reference10_30.convertReference(src.getConformance())); 754 return tgt; 755 } 756 757 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 758 if (src == null || src.isEmpty()) 759 return null; 760 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent(); 761 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 762 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 763 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 764 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 765 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 766 return tgt; 767 } 768 769 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 770 if (src == null || src.isEmpty()) 771 return null; 772 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent(); 773 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 774 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 775 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 776 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 777 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 778 return tgt; 779 } 780 781 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 782 if (src == null || src.isEmpty()) 783 return null; 784 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent(); 785 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 786 if (src.hasUrlElement()) 787 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 788 if (src.hasDescriptionElement()) 789 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 790 return tgt; 791 } 792 793 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 794 if (src == null || src.isEmpty()) 795 return null; 796 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent(); 797 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 798 if (src.hasUrlElement()) 799 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 800 if (src.hasDescriptionElement()) 801 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 802 return tgt; 803 } 804 805 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 806 if (src == null || src.isEmpty()) 807 return null; 808 org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent(); 809 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 810 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent t : src.getAction()) 811 tgt.addAction(convertSetupActionComponent(t)); 812 return tgt; 813 } 814 815 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 816 if (src == null || src.isEmpty()) 817 return null; 818 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent(); 819 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 820 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent t : src.getAction()) 821 tgt.addAction(convertSetupActionComponent(t)); 822 return tgt; 823 } 824 825 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 826 if (src == null || src.isEmpty()) 827 return null; 828 org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent(); 829 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 830 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent t : src.getAction()) 831 tgt.addAction(convertTeardownActionComponent(t)); 832 return tgt; 833 } 834 835 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 836 if (src == null || src.isEmpty()) 837 return null; 838 org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent(); 839 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 840 for (org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent t : src.getAction()) 841 tgt.addAction(convertTeardownActionComponent(t)); 842 return tgt; 843 } 844 845 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent src) throws FHIRException { 846 if (src == null || src.isEmpty()) 847 return null; 848 org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent(); 849 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 850 if (src.hasNameElement()) 851 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 852 if (src.hasDescriptionElement()) 853 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 854 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent t : src.getAction()) 855 tgt.addAction(convertTestActionComponent(t)); 856 return tgt; 857 } 858 859 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent src) throws FHIRException { 860 if (src == null || src.isEmpty()) 861 return null; 862 org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent(); 863 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 864 if (src.hasNameElement()) 865 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 866 if (src.hasDescriptionElement()) 867 tgt.setDescriptionElement(String10_30.convertString(src.getDescriptionElement())); 868 for (org.hl7.fhir.dstu3.model.TestScript.TestActionComponent t : src.getAction()) 869 tgt.addAction(convertTestActionComponent(t)); 870 return tgt; 871 } 872 873 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 874 if (src == null || src.isEmpty()) 875 return null; 876 org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent(); 877 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 878 if (src.hasNameElement()) 879 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 880 if (src.hasHeaderFieldElement()) 881 tgt.setHeaderFieldElement(String10_30.convertString(src.getHeaderFieldElement())); 882 if (src.hasPathElement()) 883 tgt.setPathElement(String10_30.convertString(src.getPathElement())); 884 if (src.hasSourceIdElement()) 885 tgt.setSourceIdElement(Id10_30.convertId(src.getSourceIdElement())); 886 return tgt; 887 } 888 889 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 890 if (src == null || src.isEmpty()) 891 return null; 892 org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent(); 893 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 894 if (src.hasNameElement()) 895 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 896 if (src.hasHeaderFieldElement()) 897 tgt.setHeaderFieldElement(String10_30.convertString(src.getHeaderFieldElement())); 898 if (src.hasPathElement()) 899 tgt.setPathElement(String10_30.convertString(src.getPathElement())); 900 if (src.hasSourceIdElement()) 901 tgt.setSourceIdElement(Id10_30.convertId(src.getSourceIdElement())); 902 return tgt; 903 } 904}