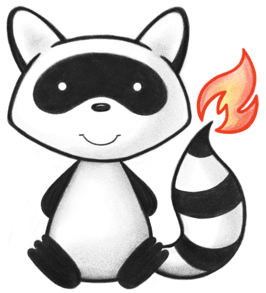
001package org.hl7.fhir.convertors.conv10_30.resources10_30; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_30; 006import org.hl7.fhir.convertors.context.ConversionContext10_30; 007import org.hl7.fhir.convertors.conv10_30.VersionConvertor_10_30; 008import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.CodeableConcept10_30; 009import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Coding10_30; 010import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.ContactPoint10_30; 011import org.hl7.fhir.convertors.conv10_30.datatypes10_30.complextypes10_30.Identifier10_30; 012import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Boolean10_30; 013import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Code10_30; 014import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.DateTime10_30; 015import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Integer10_30; 016import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.String10_30; 017import org.hl7.fhir.convertors.conv10_30.datatypes10_30.primitivetypes10_30.Uri10_30; 018import org.hl7.fhir.dstu2.model.ValueSet; 019import org.hl7.fhir.dstu3.model.CodeSystem; 020import org.hl7.fhir.dstu3.model.CodeSystem.CodeSystemContentMode; 021import org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent; 022import org.hl7.fhir.dstu3.model.Enumeration; 023import org.hl7.fhir.dstu3.terminologies.CodeSystemUtilities; 024import org.hl7.fhir.exceptions.FHIRException; 025 026public class ValueSet10_30 { 027 028 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 029 if (src == null || src.isEmpty()) 030 return null; 031 org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent(); 032 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 033 if (src.hasCodeElement()) 034 tgt.setCodeElement(Code10_30.convertCode(src.getCodeElement())); 035 if (src.hasDisplayElement()) 036 tgt.setDisplayElement(String10_30.convertString(src.getDisplayElement())); 037 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 038 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 039 return tgt; 040 } 041 042 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 043 if (src == null || src.isEmpty()) 044 return null; 045 org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent(); 046 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 047 if (src.hasCodeElement()) 048 tgt.setCodeElement(Code10_30.convertCode(src.getCodeElement())); 049 if (src.hasDisplayElement()) 050 tgt.setDisplayElement(String10_30.convertString(src.getDisplayElement())); 051 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent t : src.getDesignation()) 052 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent(); 060 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 061 if (src.hasLanguageElement()) 062 tgt.setLanguageElement(Code10_30.convertCode(src.getLanguageElement())); 063 if (src.hasUse()) 064 tgt.setUse(Coding10_30.convertCoding(src.getUse())); 065 if (src.hasValueElement()) 066 tgt.setValueElement(String10_30.convertString(src.getValueElement())); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 071 if (src == null || src.isEmpty()) 072 return null; 073 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent(); 074 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 075 if (src.hasLanguageElement()) 076 tgt.setLanguageElement(Code10_30.convertCode(src.getLanguageElement())); 077 if (src.hasUse()) 078 tgt.setUse(Coding10_30.convertCoding(src.getUse())); 079 if (src.hasValueElement()) 080 tgt.setValueElement(String10_30.convertString(src.getValueElement())); 081 return tgt; 082 } 083 084 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent src) throws FHIRException { 085 if (src == null || src.isEmpty()) 086 return null; 087 org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent(); 088 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 089 if (src.hasSystemElement()) 090 tgt.setSystemElement(Uri10_30.convertUri(src.getSystemElement())); 091 if (src.hasVersionElement()) 092 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 093 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 094 tgt.addConcept(convertConceptReferenceComponent(t)); 095 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 096 tgt.addFilter(convertConceptSetFilterComponent(t)); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent(); 104 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 105 if (src.hasSystemElement()) 106 tgt.setSystemElement(Uri10_30.convertUri(src.getSystemElement())); 107 if (src.hasVersionElement()) 108 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 109 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 110 tgt.addConcept(convertConceptReferenceComponent(t)); 111 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 112 tgt.addFilter(convertConceptSetFilterComponent(t)); 113 return tgt; 114 } 115 116 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent(); 120 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 121 if (src.hasPropertyElement()) 122 tgt.setPropertyElement(Code10_30.convertCode(src.getPropertyElement())); 123 if (src.hasOp()) 124 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 125 if (src.hasValueElement()) 126 tgt.setValueElement(Code10_30.convertCode(src.getValueElement())); 127 return tgt; 128 } 129 130 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent(); 134 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 135 if (src.hasPropertyElement()) 136 tgt.setPropertyElement(Code10_30.convertCode(src.getPropertyElement())); 137 if (src.hasOp()) 138 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 139 if (src.hasValueElement()) 140 tgt.setValueElement(Code10_30.convertCode(src.getValueElement())); 141 return tgt; 142 } 143 144 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ValueSet.FilterOperator> src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> tgt = new Enumeration<>(new org.hl7.fhir.dstu3.model.ValueSet.FilterOperatorEnumFactory()); 148 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 149 if (src.getValue() == null) { 150 tgt.setValue(null); 151 } else { 152 switch (src.getValue()) { 153 case EQUAL: 154 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.EQUAL); 155 break; 156 case ISA: 157 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.ISA); 158 break; 159 case ISNOTA: 160 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.ISNOTA); 161 break; 162 case REGEX: 163 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.REGEX); 164 break; 165 case IN: 166 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.IN); 167 break; 168 case NOTIN: 169 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.NOTIN); 170 break; 171 default: 172 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.NULL); 173 break; 174 } 175 } 176 return tgt; 177 } 178 179 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> src) throws FHIRException { 180 if (src == null || src.isEmpty()) 181 return null; 182 org.hl7.fhir.dstu2.model.Enumeration<ValueSet.FilterOperator> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new ValueSet.FilterOperatorEnumFactory()); 183 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 184 if (src.getValue() == null) { 185 tgt.setValue(null); 186 } else { 187 switch (src.getValue()) { 188 case EQUAL: 189 tgt.setValue(ValueSet.FilterOperator.EQUAL); 190 break; 191 case ISA: 192 tgt.setValue(ValueSet.FilterOperator.ISA); 193 break; 194 case ISNOTA: 195 tgt.setValue(ValueSet.FilterOperator.ISNOTA); 196 break; 197 case REGEX: 198 tgt.setValue(ValueSet.FilterOperator.REGEX); 199 break; 200 case IN: 201 tgt.setValue(ValueSet.FilterOperator.IN); 202 break; 203 case NOTIN: 204 tgt.setValue(ValueSet.FilterOperator.NOTIN); 205 break; 206 default: 207 tgt.setValue(ValueSet.FilterOperator.NULL); 208 break; 209 } 210 } 211 return tgt; 212 } 213 214 public static org.hl7.fhir.dstu3.model.ValueSet convertValueSet(org.hl7.fhir.dstu2.model.ValueSet src, BaseAdvisor_10_30 advisor) throws FHIRException { 215 if (src == null || src.isEmpty()) 216 return null; 217 org.hl7.fhir.dstu3.model.ValueSet tgt = new org.hl7.fhir.dstu3.model.ValueSet(); 218 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 219 if (src.hasUrlElement()) 220 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 221 if (src.hasIdentifier()) 222 tgt.addIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 223 if (src.hasVersionElement()) 224 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 225 if (src.hasNameElement()) 226 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 227 if (src.hasStatus()) 228 tgt.setStatusElement(Enumerations10_30.convertConformanceResourceStatus(src.getStatusElement())); 229 if (src.hasExperimental()) 230 tgt.setExperimentalElement(Boolean10_30.convertBoolean(src.getExperimentalElement())); 231 if (src.hasPublisherElement()) 232 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 233 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent t : src.getContact()) 234 tgt.addContact(convertValueSetContactComponent(t)); 235 if (src.hasDate()) 236 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 237 if (src.hasDescription()) 238 tgt.setDescription(src.getDescription()); 239 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 240 if (VersionConvertor_10_30.isJurisdiction(t)) 241 tgt.addJurisdiction(CodeableConcept10_30.convertCodeableConcept(t)); 242 else 243 tgt.addUseContext(CodeableConcept10_30.convertCodeableConceptToUsageContext(t)); 244 if (src.hasImmutableElement()) 245 tgt.setImmutableElement(Boolean10_30.convertBoolean(src.getImmutableElement())); 246 if (src.hasRequirements()) 247 tgt.setPurpose(src.getRequirements()); 248 if (src.hasCopyright()) 249 tgt.setCopyright(src.getCopyright()); 250 if (src.hasExtensibleElement()) 251 tgt.setExtensibleElement(Boolean10_30.convertBoolean(src.getExtensibleElement())); 252 if (src.hasCompose()) { 253 if (src.hasCompose()) 254 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 255 tgt.getCompose().setLockedDate(src.getLockedDate()); 256 } 257 //TODO 258 if (src.hasCodeSystem() && advisor != null) { 259 org.hl7.fhir.dstu3.model.CodeSystem tgtcs = new org.hl7.fhir.dstu3.model.CodeSystem(); 260 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgtcs); 261 tgtcs.setUrl(src.getCodeSystem().getSystem()); 262 tgtcs.setIdentifier(Identifier10_30.convertIdentifier(src.getIdentifier())); 263 tgtcs.setVersion(src.getCodeSystem().getVersion()); 264 tgtcs.setName(src.getName() + " Code System"); 265 tgtcs.setStatusElement(Enumerations10_30.convertConformanceResourceStatus(src.getStatusElement())); 266 if (src.hasExperimental()) 267 tgtcs.setExperimental(src.getExperimental()); 268 tgtcs.setPublisher(src.getPublisher()); 269 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent t : src.getContact()) 270 tgtcs.addContact(convertValueSetContactComponent(t)); 271 if (src.hasDate()) 272 tgtcs.setDate(src.getDate()); 273 tgtcs.setDescription(src.getDescription()); 274 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 275 if (VersionConvertor_10_30.isJurisdiction(t)) 276 tgtcs.addJurisdiction(CodeableConcept10_30.convertCodeableConcept(t)); 277 else 278 tgtcs.addUseContext(CodeableConcept10_30.convertCodeableConceptToUsageContext(t)); 279 tgtcs.setPurpose(src.getRequirements()); 280 tgtcs.setCopyright(src.getCopyright()); 281 tgtcs.setContent(CodeSystemContentMode.COMPLETE); 282 tgtcs.setCaseSensitive(src.getCodeSystem().getCaseSensitive()); 283 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent cs : src.getCodeSystem().getConcept()) 284 processConcept(tgtcs.getConcept(), cs, tgtcs); 285 advisor.handleCodeSystem(tgtcs, tgt); 286 tgt.setUserData("r2-cs", tgtcs); 287 tgt.getCompose().addInclude().setSystem(tgtcs.getUrl()); 288 } 289 if (src.hasExpansion()) 290 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 291 return tgt; 292 } 293 294 public static org.hl7.fhir.dstu2.model.ValueSet convertValueSet(org.hl7.fhir.dstu3.model.ValueSet src, BaseAdvisor_10_30 advisor) throws FHIRException { 295 if (src == null || src.isEmpty()) 296 return null; 297 org.hl7.fhir.dstu2.model.ValueSet tgt = new org.hl7.fhir.dstu2.model.ValueSet(); 298 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyDomainResource(src, tgt); 299 if (src.hasUrlElement()) 300 tgt.setUrlElement(Uri10_30.convertUri(src.getUrlElement())); 301 for (org.hl7.fhir.dstu3.model.Identifier i : src.getIdentifier()) 302 tgt.setIdentifier(Identifier10_30.convertIdentifier(i)); 303 if (src.hasVersionElement()) 304 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 305 if (src.hasNameElement()) 306 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 307 if (src.hasStatus()) 308 tgt.setStatusElement(Enumerations10_30.convertConformanceResourceStatus(src.getStatusElement())); 309 if (src.hasExperimental()) 310 tgt.setExperimentalElement(Boolean10_30.convertBoolean(src.getExperimentalElement())); 311 if (src.hasPublisherElement()) 312 tgt.setPublisherElement(String10_30.convertString(src.getPublisherElement())); 313 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 314 tgt.addContact(convertValueSetContactComponent(t)); 315 if (src.hasDate()) 316 tgt.setDateElement(DateTime10_30.convertDateTime(src.getDateElement())); 317 tgt.setLockedDate(src.getCompose().getLockedDate()); 318 if (src.hasDescription()) 319 tgt.setDescription(src.getDescription()); 320 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 321 if (t.hasValueCodeableConcept()) 322 tgt.addUseContext(CodeableConcept10_30.convertCodeableConcept(t.getValueCodeableConcept())); 323 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 324 tgt.addUseContext(CodeableConcept10_30.convertCodeableConcept(t)); 325 if (src.hasImmutableElement()) 326 tgt.setImmutableElement(Boolean10_30.convertBoolean(src.getImmutableElement())); 327 if (src.hasPurpose()) 328 tgt.setRequirements(src.getPurpose()); 329 if (src.hasCopyright()) 330 tgt.setCopyright(src.getCopyright()); 331 if (src.hasExtensibleElement()) 332 tgt.setExtensibleElement(Boolean10_30.convertBoolean(src.getExtensibleElement())); 333 org.hl7.fhir.dstu3.model.CodeSystem srcCS = (CodeSystem) src.getUserData("r2-cs"); 334 if (srcCS == null) 335 srcCS = advisor.getCodeSystem(src); 336 if (srcCS != null) { 337 tgt.getCodeSystem().setSystem(srcCS.getUrl()); 338 tgt.getCodeSystem().setVersion(srcCS.getVersion()); 339 tgt.getCodeSystem().setCaseSensitive(srcCS.getCaseSensitive()); 340 for (org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent cs : srcCS.getConcept()) 341 processConcept(tgt.getCodeSystem().getConcept(), cs, srcCS); 342 } 343 if (src.hasCompose()) 344 tgt.setCompose(convertValueSetComposeComponent(src.getCompose(), srcCS == null ? null : srcCS.getUrl())); 345 if (src.hasExpansion()) 346 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 347 return tgt; 348 } 349 350 public static org.hl7.fhir.dstu3.model.ValueSet convertValueSet(org.hl7.fhir.dstu2.model.ValueSet src) throws FHIRException { 351 return convertValueSet(src, null); 352 } 353 354 public static org.hl7.fhir.dstu2.model.ValueSet convertValueSet(org.hl7.fhir.dstu3.model.ValueSet src) throws FHIRException { 355 return convertValueSet(src, null); 356 } 357 358 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 359 if (src == null || src.isEmpty()) 360 return null; 361 org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent(); 362 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 363 for (org.hl7.fhir.dstu2.model.UriType t : src.getImport()) tgt.addInclude().addValueSet(t.getValue()); 364 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent t : src.getInclude()) 365 tgt.addInclude(convertConceptSetComponent(t)); 366 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent t : src.getExclude()) 367 tgt.addExclude(convertConceptSetComponent(t)); 368 return tgt; 369 } 370 371 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent src, String noSystem) throws FHIRException { 372 if (src == null || src.isEmpty()) 373 return null; 374 org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent(); 375 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 376 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent t : src.getInclude()) { 377 for (org.hl7.fhir.dstu3.model.UriType ti : t.getValueSet()) tgt.addImport(ti.getValue()); 378 if (noSystem == null || !t.getSystem().equals(noSystem)) 379 tgt.addInclude(convertConceptSetComponent(t)); 380 } 381 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent t : src.getExclude()) 382 tgt.addExclude(convertConceptSetComponent(t)); 383 return tgt; 384 } 385 386 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent convertValueSetContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 387 if (src == null || src.isEmpty()) 388 return null; 389 org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent(); 390 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 391 if (src.hasNameElement()) 392 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 393 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 394 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 395 return tgt; 396 } 397 398 public static org.hl7.fhir.dstu3.model.ContactDetail convertValueSetContactComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent src) throws FHIRException { 399 if (src == null || src.isEmpty()) 400 return null; 401 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 402 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 403 if (src.hasNameElement()) 404 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 405 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 406 tgt.addTelecom(ContactPoint10_30.convertContactPoint(t)); 407 return tgt; 408 } 409 410 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 411 if (src == null || src.isEmpty()) 412 return null; 413 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent(); 414 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 415 if (src.hasIdentifierElement()) 416 tgt.setIdentifierElement(Uri10_30.convertUri(src.getIdentifierElement())); 417 if (src.hasTimestampElement()) 418 tgt.setTimestampElement(DateTime10_30.convertDateTime(src.getTimestampElement())); 419 if (src.hasTotalElement()) 420 tgt.setTotalElement(Integer10_30.convertInteger(src.getTotalElement())); 421 if (src.hasOffsetElement()) 422 tgt.setOffsetElement(Integer10_30.convertInteger(src.getOffsetElement())); 423 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 424 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 425 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 426 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 427 return tgt; 428 } 429 430 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 431 if (src == null || src.isEmpty()) 432 return null; 433 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent(); 434 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 435 if (src.hasIdentifierElement()) 436 tgt.setIdentifierElement(Uri10_30.convertUri(src.getIdentifierElement())); 437 if (src.hasTimestampElement()) 438 tgt.setTimestampElement(DateTime10_30.convertDateTime(src.getTimestampElement())); 439 if (src.hasTotalElement()) 440 tgt.setTotalElement(Integer10_30.convertInteger(src.getTotalElement())); 441 if (src.hasOffsetElement()) 442 tgt.setOffsetElement(Integer10_30.convertInteger(src.getOffsetElement())); 443 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 444 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 445 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 446 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 447 return tgt; 448 } 449 450 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 451 if (src == null || src.isEmpty()) 452 return null; 453 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent(); 454 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 455 if (src.hasSystemElement()) 456 tgt.setSystemElement(Uri10_30.convertUri(src.getSystemElement())); 457 if (src.hasAbstractElement()) 458 tgt.setAbstractElement(Boolean10_30.convertBoolean(src.getAbstractElement())); 459 if (src.hasVersionElement()) 460 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 461 if (src.hasCodeElement()) 462 tgt.setCodeElement(Code10_30.convertCode(src.getCodeElement())); 463 if (src.hasDisplayElement()) 464 tgt.setDisplayElement(String10_30.convertString(src.getDisplayElement())); 465 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 466 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 467 return tgt; 468 } 469 470 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 471 if (src == null || src.isEmpty()) 472 return null; 473 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent(); 474 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 475 if (src.hasSystemElement()) 476 tgt.setSystemElement(Uri10_30.convertUri(src.getSystemElement())); 477 if (src.hasAbstractElement()) 478 tgt.setAbstractElement(Boolean10_30.convertBoolean(src.getAbstractElement())); 479 if (src.hasVersionElement()) 480 tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 481 if (src.hasCodeElement()) 482 tgt.setCodeElement(Code10_30.convertCode(src.getCodeElement())); 483 if (src.hasDisplayElement()) 484 tgt.setDisplayElement(String10_30.convertString(src.getDisplayElement())); 485 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 486 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 487 return tgt; 488 } 489 490 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 491 if (src == null || src.isEmpty()) 492 return null; 493 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent(); 494 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 495 if (src.hasNameElement()) 496 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 497 if (src.hasValue()) 498 tgt.setValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getValue())); 499 return tgt; 500 } 501 502 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 503 if (src == null || src.isEmpty()) 504 return null; 505 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent(); 506 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 507 if (src.hasNameElement()) 508 tgt.setNameElement(String10_30.convertString(src.getNameElement())); 509 if (src.hasValue()) 510 tgt.setValue(ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().convertType(src.getValue())); 511 return tgt; 512 } 513 514 static public void processConcept(List<ConceptDefinitionComponent> concepts, org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent cs, CodeSystem tgtcs) throws FHIRException { 515 org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent ct = new org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent(); 516 concepts.add(ct); 517 ct.setCode(cs.getCode()); 518 ct.setDisplay(cs.getDisplay()); 519 ct.setDefinition(cs.getDefinition()); 520 if (cs.getAbstract()) 521 CodeSystemUtilities.setNotSelectable(tgtcs, ct); 522 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent csd : cs.getDesignation()) { 523 org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionDesignationComponent cst = new org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionDesignationComponent(); 524 cst.setLanguage(csd.getLanguage()); 525 cst.setUse(Coding10_30.convertCoding(csd.getUse())); 526 cst.setValue(csd.getValue()); 527 } 528 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent csc : cs.getConcept()) 529 processConcept(ct.getConcept(), csc, tgtcs); 530 } 531 532 static public void processConcept(List<ValueSet.ConceptDefinitionComponent> concepts, ConceptDefinitionComponent cs, CodeSystem srcCS) throws FHIRException { 533 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent ct = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent(); 534 concepts.add(ct); 535 ct.setCode(cs.getCode()); 536 ct.setDisplay(cs.getDisplay()); 537 ct.setDefinition(cs.getDefinition()); 538 if (CodeSystemUtilities.isNotSelectable(srcCS, cs)) 539 ct.setAbstract(true); 540 for (org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionDesignationComponent csd : cs.getDesignation()) { 541 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent cst = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent(); 542 cst.setLanguage(csd.getLanguage()); 543 cst.setUse(Coding10_30.convertCoding(csd.getUse())); 544 cst.setValue(csd.getValue()); 545 } 546 for (ConceptDefinitionComponent csc : cs.getConcept()) processConcept(ct.getConcept(), csc, srcCS); 547 } 548 549 public static ValueSet.ValueSetCodeSystemComponent convertCodeSystem(CodeSystem src) throws FHIRException { 550 if (src == null || src.isEmpty()) return null; 551 ValueSet.ValueSetCodeSystemComponent tgt = new ValueSet.ValueSetCodeSystemComponent(); 552 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyElement(src, tgt); 553 if (src.hasUrlElement()) tgt.setSystemElement(Uri10_30.convertUri(src.getUrlElement())); 554 if (src.hasVersionElement()) tgt.setVersionElement(String10_30.convertString(src.getVersionElement())); 555 if (src.hasCaseSensitiveElement()) 556 tgt.setCaseSensitiveElement(Boolean10_30.convertBoolean(src.getCaseSensitiveElement())); 557 for (ConceptDefinitionComponent cc : src.getConcept()) tgt.addConcept(convertCodeSystemConcept(src, cc)); 558 return tgt; 559 } 560 561 public static ValueSet.ConceptDefinitionComponent convertCodeSystemConcept(CodeSystem cs, ConceptDefinitionComponent src) throws FHIRException { 562 if (src == null || src.isEmpty()) return null; 563 ValueSet.ConceptDefinitionComponent tgt = new ValueSet.ConceptDefinitionComponent(); 564 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 565 tgt.setAbstract(CodeSystemUtilities.isNotSelectable(cs, src)); 566 tgt.setCode(src.getCode()); 567 tgt.setDefinition(src.getDefinition()); 568 tgt.setDisplay(src.getDisplay()); 569 for (ConceptDefinitionComponent cc : src.getConcept()) tgt.addConcept(convertCodeSystemConcept(cs, cc)); 570 for (CodeSystem.ConceptDefinitionDesignationComponent cc : src.getDesignation()) 571 tgt.addDesignation(convertCodeSystemDesignation(cc)); 572 return tgt; 573 } 574 575 public static ValueSet.ConceptDefinitionDesignationComponent convertCodeSystemDesignation(CodeSystem.ConceptDefinitionDesignationComponent src) throws FHIRException { 576 if (src == null || src.isEmpty()) return null; 577 ValueSet.ConceptDefinitionDesignationComponent tgt = new ValueSet.ConceptDefinitionDesignationComponent(); 578 ConversionContext10_30.INSTANCE.getVersionConvertor_10_30().copyBackboneElement(src,tgt); 579 tgt.setUse(Coding10_30.convertCoding(src.getUse())); 580 tgt.setLanguage(src.getLanguage()); 581 tgt.setValue(src.getValue()); 582 return tgt; 583 } 584}