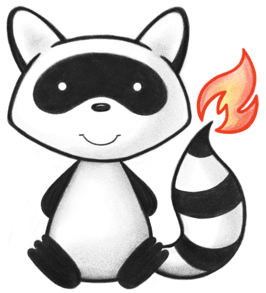
001package org.hl7.fhir.convertors.conv10_40.datatypes10_40; 002 003import java.util.List; 004import java.util.stream.Collectors; 005 006import org.hl7.fhir.convertors.VersionConvertorConstants; 007import org.hl7.fhir.convertors.context.ConversionContext10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Code10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Id10_40; 012import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Integer10_40; 013import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.MarkDown10_40; 014import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 015import org.hl7.fhir.dstu2.utils.ToolingExtensions; 016import org.hl7.fhir.exceptions.FHIRException; 017import org.hl7.fhir.r4.conformance.ProfileUtilities; 018import org.hl7.fhir.r4.model.ElementDefinition; 019import org.hl7.fhir.utilities.Utilities; 020 021public class ElementDefinition10_40 { 022 public static org.hl7.fhir.r4.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu2.model.ElementDefinition src, List<String> slicePaths, List<org.hl7.fhir.dstu2.model.ElementDefinition> context, int pos) throws FHIRException { 023 if (src == null || src.isEmpty()) return null; 024 org.hl7.fhir.r4.model.ElementDefinition tgt = new org.hl7.fhir.r4.model.ElementDefinition(); 025 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 026 if (src.hasPathElement()) tgt.setPathElement(String10_40.convertString(src.getPathElement())); 027 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition10_40::convertPropertyRepresentation).collect(Collectors.toList())); 028 if (src.hasName()) { 029 if (slicePaths.contains(src.getPath())) tgt.setSliceNameElement(String10_40.convertString(src.getNameElement())); 030 if (src.hasNameElement()) tgt.setIdElement(String10_40.convertString(src.getNameElement())); 031 } 032 if (src.hasLabel()) tgt.setLabelElement(String10_40.convertString(src.getLabelElement())); 033 for (org.hl7.fhir.dstu2.model.Coding t : src.getCode()) tgt.addCode(Coding10_40.convertCoding(t)); 034 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing(), context, pos)); 035 if (src.hasShort()) tgt.setShortElement(String10_40.convertString(src.getShortElement())); 036 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown10_40.convertMarkdown(src.getDefinitionElement())); 037 if (src.hasComments()) tgt.setCommentElement(MarkDown10_40.convertMarkdown(src.getCommentsElement())); 038 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown10_40.convertMarkdown(src.getRequirementsElement())); 039 for (org.hl7.fhir.dstu2.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 040 if (src.hasMin()) tgt.setMin(src.getMin()); 041 if (src.hasMax()) tgt.setMaxElement(String10_40.convertString(src.getMaxElement())); 042 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 043 if (src.hasNameReference()) tgt.setContentReference("#" + src.getNameReference()); 044 for (org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent t : src.getType()) 045 convertElementDefinitionTypeComponent(t, tgt.getType()); 046 if (src.hasDefaultValue()) 047 tgt.setDefaultValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getDefaultValue())); 048 if (src.hasMeaningWhenMissing()) 049 tgt.setMeaningWhenMissingElement(MarkDown10_40.convertMarkdown(src.getMeaningWhenMissingElement())); 050 if (src.hasFixed()) 051 tgt.setFixed(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getFixed())); 052 if (src.hasPattern()) 053 tgt.setPattern(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getPattern())); 054 if (src.hasExample()) 055 tgt.addExample().setLabel("General").setValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getExample())); 056 if (src.hasMinValue()) 057 tgt.setMinValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getMinValue())); 058 if (src.hasMaxValue()) 059 tgt.setMaxValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getMaxValue())); 060 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer10_40.convertInteger(src.getMaxLengthElement())); 061 for (org.hl7.fhir.dstu2.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 062 for (org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 063 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 064 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean10_40.convertBoolean(src.getMustSupportElement())); 065 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean10_40.convertBoolean(src.getIsModifierElement())); 066 if (tgt.getIsModifier()) { 067 String reason = org.hl7.fhir.dstu2.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.MODIFIER_REASON_EXTENSION); 068 if (Utilities.noString(reason)) reason = VersionConvertorConstants.MODIFIER_REASON_LEGACY; 069 tgt.setIsModifierReason(reason); 070 } 071 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean10_40.convertBoolean(src.getIsSummaryElement())); 072 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 073 for (org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 074 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 075 if (!tgt.hasId()) tgt.setId(tgt.getPath()); 076 return tgt; 077 } 078 079 public static org.hl7.fhir.dstu2.model.ElementDefinition convertElementDefinition(org.hl7.fhir.r4.model.ElementDefinition src) throws FHIRException { 080 if (src == null || src.isEmpty()) return null; 081 org.hl7.fhir.dstu2.model.ElementDefinition tgt = new org.hl7.fhir.dstu2.model.ElementDefinition(); 082 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 083 if (src.hasPathElement()) tgt.setPathElement(String10_40.convertString(src.getPathElement())); 084 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition10_40::convertPropertyRepresentation).collect(Collectors.toList())); 085 if (src.hasSliceName()) tgt.setNameElement(String10_40.convertString(src.getSliceNameElement())); 086 else tgt.setNameElement(String10_40.convertString(src.getIdElement())); 087 if (src.hasLabelElement()) tgt.setLabelElement(String10_40.convertString(src.getLabelElement())); 088 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addCode(Coding10_40.convertCoding(t)); 089 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 090 if (src.hasShortElement()) tgt.setShortElement(String10_40.convertString(src.getShortElement())); 091 if (src.hasDefinitionElement()) tgt.setDefinitionElement(MarkDown10_40.convertMarkdown(src.getDefinitionElement())); 092 if (src.hasCommentElement()) tgt.setCommentsElement(MarkDown10_40.convertMarkdown(src.getCommentElement())); 093 if (src.hasRequirementsElement()) 094 tgt.setRequirementsElement(MarkDown10_40.convertMarkdown(src.getRequirementsElement())); 095 for (org.hl7.fhir.r4.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 096 tgt.setMin(src.getMin()); 097 if (src.hasMaxElement()) tgt.setMaxElement(String10_40.convertString(src.getMaxElement())); 098 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 099 if (src.hasContentReference()) tgt.setNameReference(src.getContentReference().substring(1)); 100 for (org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent t : src.getType()) 101 convertElementDefinitionTypeComponent(t, tgt.getType()); 102 if (src.hasDefaultValue()) 103 tgt.setDefaultValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getDefaultValue())); 104 if (src.hasMeaningWhenMissingElement()) 105 tgt.setMeaningWhenMissingElement(MarkDown10_40.convertMarkdown(src.getMeaningWhenMissingElement())); 106 if (src.hasFixed()) 107 tgt.setFixed(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getFixed())); 108 if (src.hasPattern()) 109 tgt.setPattern(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getPattern())); 110 if (src.hasExample()) 111 tgt.setExample(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getExampleFirstRep().getValue())); 112 if (src.hasMinValue()) 113 tgt.setMinValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getMinValue())); 114 if (src.hasMaxValue()) 115 tgt.setMaxValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getMaxValue())); 116 if (src.hasMaxLengthElement()) tgt.setMaxLengthElement(Integer10_40.convertInteger(src.getMaxLengthElement())); 117 for (org.hl7.fhir.r4.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 118 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 119 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 120 if (src.hasMustSupportElement()) 121 tgt.setMustSupportElement(Boolean10_40.convertBoolean(src.getMustSupportElement())); 122 if (src.hasIsModifierElement()) tgt.setIsModifierElement(Boolean10_40.convertBoolean(src.getIsModifierElement())); 123 if (src.hasIsModifierReason() && !VersionConvertorConstants.MODIFIER_REASON_LEGACY.equals(src.getIsModifierReason())) 124 org.hl7.fhir.dstu2.utils.ToolingExtensions.setStringExtension(tgt, VersionConvertorConstants.MODIFIER_REASON_EXTENSION, src.getIsModifierReason()); 125 if (src.hasIsSummaryElement()) tgt.setIsSummaryElement(Boolean10_40.convertBoolean(src.getIsSummaryElement())); 126 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 127 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 128 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 129 return tgt; 130 } 131 132 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 133 if (src == null || src.isEmpty()) return null; 134 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentationEnumFactory()); 135 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 136 if (src.getValue() == null) { 137 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.NULL); 138 } else { 139 switch (src.getValue()) { 140 case XMLATTR: 141 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.XMLATTR); 142 break; 143 default: 144 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.NULL); 145 break; 146 } 147 } 148 return tgt; 149 } 150 151 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 152 if (src == null || src.isEmpty()) return null; 153 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentationEnumFactory()); 154 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 155 if (src.getValue() == null) { 156 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation.NULL); 157 } else { 158 switch (src.getValue()) { 159 case XMLATTR: 160 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation.XMLATTR); 161 break; 162 default: 163 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.PropertyRepresentation.NULL); 164 break; 165 } 166 } 167 return tgt; 168 } 169 170 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent src, List<org.hl7.fhir.dstu2.model.ElementDefinition> context, int pos) throws FHIRException { 171 if (src == null || src.isEmpty()) return null; 172 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent(); 173 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 174 org.hl7.fhir.dstu2.model.ElementDefinition slicingElement = context.get(pos); 175 for (org.hl7.fhir.dstu2.model.StringType t : src.getDiscriminator()) { 176 boolean isExists = false; 177 if (!t.asStringValue().contains("@")) { 178 int slices = 0; 179 boolean existsSlicePresent = false; 180 boolean notExistsSlicePresent = false; 181 String existsPath = slicingElement.getPath() + "." + t.asStringValue(); 182 for (int i = pos + 1; i < context.size(); i++) { 183 org.hl7.fhir.dstu2.model.ElementDefinition e = context.get(i); 184 if (e.getPath().equals(slicingElement.getPath())) slices++; 185 else if (!e.getPath().startsWith(slicingElement.getPath() + ".")) break; 186 else if (e.getPath().equals(existsPath)) { 187 if (e.hasMin() && e.getMin() > 0) existsSlicePresent = true; 188 else if (e.hasMax() && e.getMax().equals("0")) notExistsSlicePresent = true; 189 } 190 } 191 isExists = (slices == 2 && existsSlicePresent && notExistsSlicePresent) || (slices == 1 && existsSlicePresent != notExistsSlicePresent); 192 } 193 tgt.addDiscriminator(ProfileUtilities.interpretR2Discriminator(t.getValue(), isExists)); 194 } 195 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 196 if (src.hasOrderedElement()) tgt.setOrderedElement(Boolean10_40.convertBoolean(src.getOrderedElement())); 197 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 198 return tgt; 199 } 200 201 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 202 if (src == null || src.isEmpty()) return null; 203 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionSlicingComponent(); 204 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 205 for (ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 206 tgt.addDiscriminator(ProfileUtilities.buildR2Discriminator(t)); 207 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 208 if (src.hasOrderedElement()) tgt.setOrderedElement(Boolean10_40.convertBoolean(src.getOrderedElement())); 209 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 210 return tgt; 211 } 212 213 static public org.hl7.fhir.r4.model.Enumeration<ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules> src) throws FHIRException { 214 if (src == null || src.isEmpty()) return null; 215 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ElementDefinition.SlicingRulesEnumFactory()); 216 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 217 if (src.getValue() == null) { 218 tgt.setValue(ElementDefinition.SlicingRules.NULL); 219 } else { 220 switch (src.getValue()) { 221 case CLOSED: 222 tgt.setValue(ElementDefinition.SlicingRules.CLOSED); 223 break; 224 case OPEN: 225 tgt.setValue(ElementDefinition.SlicingRules.OPEN); 226 break; 227 case OPENATEND: 228 tgt.setValue(ElementDefinition.SlicingRules.OPENATEND); 229 break; 230 default: 231 tgt.setValue(ElementDefinition.SlicingRules.NULL); 232 break; 233 } 234 } 235 return tgt; 236 } 237 238 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.r4.model.Enumeration<ElementDefinition.SlicingRules> src) throws FHIRException { 239 if (src == null || src.isEmpty()) return null; 240 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRulesEnumFactory()); 241 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 242 if (src.getValue() == null) { 243 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.NULL); 244 } else { 245 switch (src.getValue()) { 246 case CLOSED: 247 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.CLOSED); 248 break; 249 case OPEN: 250 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.OPEN); 251 break; 252 case OPENATEND: 253 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.OPENATEND); 254 break; 255 default: 256 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.SlicingRules.NULL); 257 break; 258 } 259 } 260 return tgt; 261 } 262 263 public static ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 264 if (src == null || src.isEmpty()) return null; 265 ElementDefinition.ElementDefinitionBaseComponent tgt = new ElementDefinition.ElementDefinitionBaseComponent(); 266 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 267 if (src.hasPathElement()) tgt.setPathElement(String10_40.convertString(src.getPathElement())); 268 tgt.setMin(src.getMin()); 269 if (src.hasMaxElement()) tgt.setMaxElement(String10_40.convertString(src.getMaxElement())); 270 return tgt; 271 } 272 273 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 274 if (src == null || src.isEmpty()) return null; 275 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBaseComponent(); 276 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 277 if (src.hasPathElement()) tgt.setPathElement(String10_40.convertString(src.getPathElement())); 278 tgt.setMin(src.getMin()); 279 if (src.hasMaxElement()) tgt.setMaxElement(String10_40.convertString(src.getMaxElement())); 280 return tgt; 281 } 282 283 public static void convertElementDefinitionTypeComponent(org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent src, List<ElementDefinition.TypeRefComponent> list) throws FHIRException { 284 if (src == null) return; 285 ElementDefinition.TypeRefComponent tgt = null; 286 for (ElementDefinition.TypeRefComponent t : list) 287 if (t.getCode().equals(src.getCode())) tgt = t; 288 if (tgt == null) { 289 tgt = new ElementDefinition.TypeRefComponent(); 290 list.add(tgt); 291 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 292 tgt.setCode(src.getCode()); 293 } 294 if (tgt.hasTarget()) { 295 for (org.hl7.fhir.dstu2.model.UriType u : src.getProfile()) tgt.addTargetProfile(u.getValue()); 296 } else { 297 for (org.hl7.fhir.dstu2.model.UriType u : src.getProfile()) tgt.addProfile(u.getValue()); 298 } 299 for (org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode> t : src.getAggregation()) { 300 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> a = convertAggregationMode(t); 301 if (!tgt.hasAggregation(a.getValue())) 302 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(t, tgt.addAggregationElement().setValue(a.getValue())); 303 } 304 } 305 306 public static void convertElementDefinitionTypeComponent(ElementDefinition.TypeRefComponent src, List<org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent> list) throws FHIRException { 307 if (src == null) return; 308 org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.TypeRefComponent(); 309 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 310 tgt.setCode(src.getCode()); 311 list.add(tgt); 312 if (src.hasTarget()) { 313 for (org.hl7.fhir.r4.model.UriType u : src.getTargetProfile()) { 314 tgt.addProfile(u.getValue()); 315 } 316 } else { 317 for (org.hl7.fhir.r4.model.UriType u : src.getProfile()) { 318 tgt.addProfile(u.getValue()); 319 } 320 } 321 } 322 323 static public org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode> src) throws FHIRException { 324 if (src == null || src.isEmpty()) return null; 325 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ElementDefinition.AggregationModeEnumFactory()); 326 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 327 if (src.getValue() == null) { 328 tgt.setValue(ElementDefinition.AggregationMode.NULL); 329 } else { 330 switch (src.getValue()) { 331 case CONTAINED: 332 tgt.setValue(ElementDefinition.AggregationMode.CONTAINED); 333 break; 334 case REFERENCED: 335 tgt.setValue(ElementDefinition.AggregationMode.REFERENCED); 336 break; 337 case BUNDLED: 338 tgt.setValue(ElementDefinition.AggregationMode.BUNDLED); 339 break; 340 default: 341 tgt.setValue(ElementDefinition.AggregationMode.NULL); 342 break; 343 } 344 } 345 return tgt; 346 } 347 348 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.r4.model.Enumeration<ElementDefinition.AggregationMode> src) throws FHIRException { 349 if (src == null || src.isEmpty()) return null; 350 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.AggregationModeEnumFactory()); 351 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 352 if (src.getValue() == null) { 353 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.NULL); 354 } else { 355 switch (src.getValue()) { 356 case CONTAINED: 357 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.CONTAINED); 358 break; 359 case REFERENCED: 360 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.REFERENCED); 361 break; 362 case BUNDLED: 363 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.BUNDLED); 364 break; 365 default: 366 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.AggregationMode.NULL); 367 break; 368 } 369 } 370 return tgt; 371 } 372 373 public static ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 374 if (src == null || src.isEmpty()) return null; 375 ElementDefinition.ElementDefinitionConstraintComponent tgt = new ElementDefinition.ElementDefinitionConstraintComponent(); 376 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 377 if (src.hasKeyElement()) tgt.setKeyElement(Id10_40.convertId(src.getKeyElement())); 378 if (src.hasRequirementsElement()) 379 tgt.setRequirementsElement(String10_40.convertString(src.getRequirementsElement())); 380 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 381 if (src.hasHumanElement()) tgt.setHumanElement(String10_40.convertString(src.getHumanElement())); 382 tgt.setExpression(ToolingExtensions.readStringExtension(src, ToolingExtensions.EXT_EXPRESSION)); 383 if (src.hasXpathElement()) tgt.setXpathElement(String10_40.convertString(src.getXpathElement())); 384 return tgt; 385 } 386 387 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 388 if (src == null || src.isEmpty()) return null; 389 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionConstraintComponent(); 390 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 391 if (src.hasKeyElement()) tgt.setKeyElement(Id10_40.convertId(src.getKeyElement())); 392 if (src.hasRequirementsElement()) 393 tgt.setRequirementsElement(String10_40.convertString(src.getRequirementsElement())); 394 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 395 if (src.hasHumanElement()) tgt.setHumanElement(String10_40.convertString(src.getHumanElement())); 396 if (src.hasExpression()) 397 ToolingExtensions.addStringExtension(tgt, ToolingExtensions.EXT_EXPRESSION, src.getExpression()); 398 if (src.hasXpathElement()) tgt.setXpathElement(String10_40.convertString(src.getXpathElement())); 399 return tgt; 400 } 401 402 static public org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 403 if (src == null || src.isEmpty()) return null; 404 org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ElementDefinition.ConstraintSeverityEnumFactory()); 405 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 406 if (src.getValue() == null) { 407 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 408 } else { 409 switch (src.getValue()) { 410 case ERROR: 411 tgt.setValue(ElementDefinition.ConstraintSeverity.ERROR); 412 break; 413 case WARNING: 414 tgt.setValue(ElementDefinition.ConstraintSeverity.WARNING); 415 break; 416 default: 417 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 418 break; 419 } 420 } 421 return tgt; 422 } 423 424 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.r4.model.Enumeration<ElementDefinition.ConstraintSeverity> src) throws FHIRException { 425 if (src == null || src.isEmpty()) return null; 426 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverityEnumFactory()); 427 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 428 if (src.getValue() == null) { 429 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity.NULL); 430 } else { 431 switch (src.getValue()) { 432 case ERROR: 433 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity.ERROR); 434 break; 435 case WARNING: 436 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity.WARNING); 437 break; 438 default: 439 tgt.setValue(org.hl7.fhir.dstu2.model.ElementDefinition.ConstraintSeverity.NULL); 440 break; 441 } 442 } 443 return tgt; 444 } 445 446 public static ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 447 if (src == null || src.isEmpty()) return null; 448 ElementDefinition.ElementDefinitionBindingComponent tgt = new ElementDefinition.ElementDefinitionBindingComponent(); 449 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 450 if (src.hasStrength()) tgt.setStrengthElement(convertBindingStrength(src.getStrengthElement())); 451 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 452 if (src.hasValueSet()) { 453 org.hl7.fhir.r4.model.Type vs = ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getValueSet()); 454 if (vs != null) { 455 tgt.setValueSet(vs instanceof org.hl7.fhir.r4.model.Reference ? ((org.hl7.fhir.r4.model.Reference) vs).getReference() : vs.primitiveValue()); 456 tgt.setValueSet(VersionConvertorConstants.refToVS(tgt.getValueSet())); 457 } 458 } 459 return tgt; 460 } 461 462 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 463 if (src == null || src.isEmpty()) return null; 464 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionBindingComponent(); 465 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 466 if (src.hasStrength()) tgt.setStrengthElement(convertBindingStrength(src.getStrengthElement())); 467 if (src.hasDescriptionElement()) tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 468 if (src.hasValueSet()) { 469 String vsr = VersionConvertorConstants.vsToRef(src.getValueSet()); 470 if (vsr != null) tgt.setValueSet(new org.hl7.fhir.dstu2.model.UriType(vsr)); 471 else tgt.setValueSet(new org.hl7.fhir.dstu2.model.Reference(src.getValueSet())); 472 } 473 return tgt; 474 } 475 476 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> src) throws FHIRException { 477 if (src == null || src.isEmpty()) return null; 478 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.BindingStrengthEnumFactory()); 479 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 480 if (src.getValue() == null) { 481 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.NULL); 482 } else { 483 switch (src.getValue()) { 484 case REQUIRED: 485 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.REQUIRED); 486 break; 487 case EXTENSIBLE: 488 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.EXTENSIBLE); 489 break; 490 case PREFERRED: 491 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.PREFERRED); 492 break; 493 case EXAMPLE: 494 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.EXAMPLE); 495 break; 496 default: 497 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.NULL); 498 break; 499 } 500 } 501 return tgt; 502 } 503 504 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> src) throws FHIRException { 505 if (src == null || src.isEmpty()) return null; 506 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.BindingStrengthEnumFactory()); 507 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 508 if (src.getValue() == null) { 509 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.NULL); 510 } else { 511 switch (src.getValue()) { 512 case REQUIRED: 513 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.REQUIRED); 514 break; 515 case EXTENSIBLE: 516 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.EXTENSIBLE); 517 break; 518 case PREFERRED: 519 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.PREFERRED); 520 break; 521 case EXAMPLE: 522 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.EXAMPLE); 523 break; 524 default: 525 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.NULL); 526 break; 527 } 528 } 529 return tgt; 530 } 531 532 public static ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 533 if (src == null || src.isEmpty()) return null; 534 ElementDefinition.ElementDefinitionMappingComponent tgt = new ElementDefinition.ElementDefinitionMappingComponent(); 535 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 536 if (src.hasIdentityElement()) tgt.setIdentityElement(Id10_40.convertId(src.getIdentityElement())); 537 if (src.hasLanguageElement()) tgt.setLanguageElement(Code10_40.convertCode(src.getLanguageElement())); 538 if (src.hasMapElement()) tgt.setMapElement(String10_40.convertString(src.getMapElement())); 539 return tgt; 540 } 541 542 public static org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 543 if (src == null || src.isEmpty()) return null; 544 org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.dstu2.model.ElementDefinition.ElementDefinitionMappingComponent(); 545 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 546 if (src.hasIdentityElement()) tgt.setIdentityElement(Id10_40.convertId(src.getIdentityElement())); 547 if (src.hasLanguageElement()) tgt.setLanguageElement(Code10_40.convertCode(src.getLanguageElement())); 548 if (src.hasMapElement()) tgt.setMapElement(String10_40.convertString(src.getMapElement())); 549 return tgt; 550 } 551}