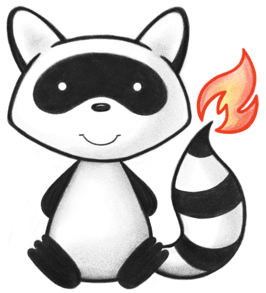
001package org.hl7.fhir.convertors.conv10_40.datatypes10_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 008import org.hl7.fhir.exceptions.FHIRException; 009import org.hl7.fhir.r4.model.Immunization; 010 011public class Reference10_40 { 012 public static org.hl7.fhir.r4.model.Reference convertReference(org.hl7.fhir.dstu2.model.Reference src) throws FHIRException { 013 if (src == null || src.isEmpty()) return null; 014 org.hl7.fhir.r4.model.Reference tgt = new org.hl7.fhir.r4.model.Reference(); 015 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 016 tgt.setReference(src.getReference()); 017 if (src.hasDisplayElement()) tgt.setDisplayElement(String10_40.convertString(src.getDisplayElement())); 018 return tgt; 019 } 020 021 public static org.hl7.fhir.dstu2.model.Reference convertReference(org.hl7.fhir.r4.model.Reference src) throws FHIRException { 022 if (src == null || src.isEmpty()) return null; 023 org.hl7.fhir.dstu2.model.Reference tgt = new org.hl7.fhir.dstu2.model.Reference(); 024 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 025 tgt.setReference(src.getReference()); 026 if (src.hasDisplayElement()) tgt.setDisplayElement(String10_40.convertString(src.getDisplayElement())); 027 return tgt; 028 } 029 030 static public org.hl7.fhir.r4.model.Reference getPerformer(List<Immunization.ImmunizationPerformerComponent> practitioner) { 031 for (Immunization.ImmunizationPerformerComponent p : practitioner) { 032 if (CodeableConcept10_40.hasConcept(p.getFunction(), "http://hl7.org/fhir/v2/0443", "AP")) return p.getActor(); 033 } 034 return null; 035 } 036 037 static public org.hl7.fhir.r4.model.Reference getRequester(List<Immunization.ImmunizationPerformerComponent> practitioner) { 038 for (Immunization.ImmunizationPerformerComponent p : practitioner) { 039 if (CodeableConcept10_40.hasConcept(p.getFunction(), "http://hl7.org/fhir/v2/0443", "OP")) return p.getActor(); 040 } 041 return null; 042 } 043}