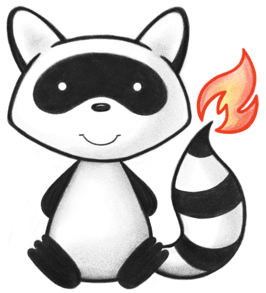
001package org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.r4.model.Identifier; 009 010public class Identifier10_40 { 011 public static org.hl7.fhir.r4.model.Identifier convertIdentifier(org.hl7.fhir.dstu2.model.Identifier src) throws FHIRException { 012 if (src == null || src.isEmpty()) return null; 013 org.hl7.fhir.r4.model.Identifier tgt = new org.hl7.fhir.r4.model.Identifier(); 014 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 015 if (src.hasUse()) tgt.setUseElement(convertIdentifierUse(src.getUseElement())); 016 if (src.hasType()) tgt.setType(CodeableConcept10_40.convertCodeableConcept(src.getType())); 017 if (src.hasSystemElement()) tgt.setSystemElement(Uri10_40.convertUri(src.getSystemElement())); 018 if (src.hasValueElement()) tgt.setValueElement(String10_40.convertString(src.getValueElement())); 019 if (src.hasPeriod()) tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 020 if (src.hasAssigner()) tgt.setAssigner(Reference10_40.convertReference(src.getAssigner())); 021 return tgt; 022 } 023 024 public static org.hl7.fhir.dstu2.model.Identifier convertIdentifier(org.hl7.fhir.r4.model.Identifier src) throws FHIRException { 025 if (src == null || src.isEmpty()) return null; 026 org.hl7.fhir.dstu2.model.Identifier tgt = new org.hl7.fhir.dstu2.model.Identifier(); 027 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 028 if (src.hasUse()) tgt.setUseElement(convertIdentifierUse(src.getUseElement())); 029 if (src.hasType()) tgt.setType(CodeableConcept10_40.convertCodeableConcept(src.getType())); 030 if (src.hasSystem()) tgt.setSystemElement(Uri10_40.convertUri(src.getSystemElement())); 031 if (src.hasValue()) tgt.setValueElement(String10_40.convertString(src.getValueElement())); 032 if (src.hasPeriod()) tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 033 if (src.hasAssigner()) tgt.setAssigner(Reference10_40.convertReference(src.getAssigner())); 034 return tgt; 035 } 036 037 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Identifier.IdentifierUse> convertIdentifierUse(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Identifier.IdentifierUse> src) throws FHIRException { 038 if (src == null || src.isEmpty()) return null; 039 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Identifier.IdentifierUse> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Identifier.IdentifierUseEnumFactory()); 040 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 041 if (src.getValue() == null) { 042 tgt.setValue(null); 043} else { 044 switch(src.getValue()) { 045 case USUAL: 046 tgt.setValue(Identifier.IdentifierUse.USUAL); 047 break; 048 case OFFICIAL: 049 tgt.setValue(Identifier.IdentifierUse.OFFICIAL); 050 break; 051 case TEMP: 052 tgt.setValue(Identifier.IdentifierUse.TEMP); 053 break; 054 case SECONDARY: 055 tgt.setValue(Identifier.IdentifierUse.SECONDARY); 056 break; 057 default: 058 tgt.setValue(Identifier.IdentifierUse.NULL); 059 break; 060 } 061} 062 return tgt; 063 } 064 065 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Identifier.IdentifierUse> convertIdentifierUse(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Identifier.IdentifierUse> src) throws FHIRException { 066 if (src == null || src.isEmpty()) return null; 067 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Identifier.IdentifierUse> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Identifier.IdentifierUseEnumFactory()); 068 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 069 if (src.getValue() == null) { 070 tgt.setValue(null); 071} else { 072 switch(src.getValue()) { 073 case USUAL: 074 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.USUAL); 075 break; 076 case OFFICIAL: 077 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.OFFICIAL); 078 break; 079 case TEMP: 080 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.TEMP); 081 break; 082 case SECONDARY: 083 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.SECONDARY); 084 break; 085 default: 086 tgt.setValue(org.hl7.fhir.dstu2.model.Identifier.IdentifierUse.NULL); 087 break; 088 } 089} 090 return tgt; 091 } 092}