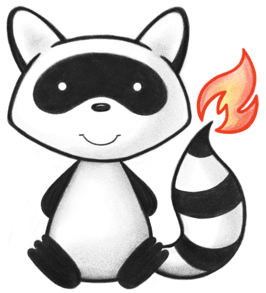
001package org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40; 002 003import java.util.Collections; 004 005import org.hl7.fhir.convertors.context.ConversionContext10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Decimal10_40; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.r4.model.Timing; 009 010public class Timing10_40 { 011 public static org.hl7.fhir.r4.model.Timing convertTiming(org.hl7.fhir.dstu2.model.Timing src) throws FHIRException { 012 if (src == null || src.isEmpty()) return null; 013 org.hl7.fhir.r4.model.Timing tgt = new org.hl7.fhir.r4.model.Timing(); 014 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 015 for (org.hl7.fhir.dstu2.model.DateTimeType t : src.getEvent()) tgt.addEvent(t.getValue()); 016 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 017 if (src.hasCode()) tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 018 return tgt; 019 } 020 021 public static org.hl7.fhir.dstu2.model.Timing convertTiming(org.hl7.fhir.r4.model.Timing src) throws FHIRException { 022 if (src == null || src.isEmpty()) return null; 023 org.hl7.fhir.dstu2.model.Timing tgt = new org.hl7.fhir.dstu2.model.Timing(); 024 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 025 for (org.hl7.fhir.r4.model.DateTimeType t : src.getEvent()) tgt.addEvent(t.getValue()); 026 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 027 if (src.hasCode()) tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 028 return tgt; 029 } 030 031 public static org.hl7.fhir.r4.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.dstu2.model.Timing.TimingRepeatComponent src) throws FHIRException { 032 if (src == null || src.isEmpty()) return null; 033 org.hl7.fhir.r4.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.r4.model.Timing.TimingRepeatComponent(); 034 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 035 if (src.hasBounds()) 036 tgt.setBounds(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getBounds())); 037 if (src.hasCount()) tgt.setCount(src.getCount()); 038 if (src.hasDurationElement()) tgt.setDurationElement(Decimal10_40.convertDecimal(src.getDurationElement())); 039 if (src.hasDurationMaxElement()) 040 tgt.setDurationMaxElement(Decimal10_40.convertDecimal(src.getDurationMaxElement())); 041 if (src.hasDurationUnits()) tgt.setDurationUnitElement(convertUnitsOfTime(src.getDurationUnitsElement())); 042 if (src.hasFrequency()) tgt.setFrequency(src.getFrequency()); 043 if (src.hasFrequencyMax()) tgt.setFrequencyMax(src.getFrequencyMax()); 044 if (src.hasPeriodElement()) tgt.setPeriodElement(Decimal10_40.convertDecimal(src.getPeriodElement())); 045 if (src.hasPeriodMaxElement()) tgt.setPeriodMaxElement(Decimal10_40.convertDecimal(src.getPeriodMaxElement())); 046 if (src.hasPeriodUnits()) tgt.setPeriodUnitElement(convertUnitsOfTime(src.getPeriodUnitsElement())); 047 if (src.hasWhen()) tgt.setWhen(Collections.singletonList(convertEventTiming(src.getWhenElement()))); 048 return tgt; 049 } 050 051 public static org.hl7.fhir.dstu2.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.r4.model.Timing.TimingRepeatComponent src) throws FHIRException { 052 if (src == null || src.isEmpty()) return null; 053 org.hl7.fhir.dstu2.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.dstu2.model.Timing.TimingRepeatComponent(); 054 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 055 if (src.hasBounds()) 056 tgt.setBounds(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getBounds())); 057 if (src.hasCount()) tgt.setCount(src.getCount()); 058 if (src.hasDurationElement()) tgt.setDurationElement(Decimal10_40.convertDecimal(src.getDurationElement())); 059 if (src.hasDurationMaxElement()) 060 tgt.setDurationMaxElement(Decimal10_40.convertDecimal(src.getDurationMaxElement())); 061 if (src.hasDurationUnit()) tgt.setDurationUnitsElement(convertUnitsOfTime(src.getDurationUnitElement())); 062 if (src.hasFrequency()) tgt.setFrequency(src.getFrequency()); 063 if (src.hasFrequencyMax()) tgt.setFrequencyMax(src.getFrequencyMax()); 064 if (src.hasPeriodElement()) tgt.setPeriodElement(Decimal10_40.convertDecimal(src.getPeriodElement())); 065 if (src.hasPeriodMaxElement()) tgt.setPeriodMaxElement(Decimal10_40.convertDecimal(src.getPeriodMaxElement())); 066 if (src.hasPeriodUnit()) tgt.setPeriodUnitsElement(convertUnitsOfTime(src.getPeriodUnitElement())); 067 if (src.hasWhen()) tgt.setWhenElement(convertEventTiming(src.getWhen().get(0))); 068 return tgt; 069 } 070 071 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Timing.UnitsOfTime> src) throws FHIRException { 072 if (src == null || src.isEmpty()) return null; 073 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Timing.UnitsOfTimeEnumFactory()); 074 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 075 if (src.getValue() == null) { 076 tgt.setValue(null); 077} else { 078 switch(src.getValue()) { 079 case S: 080 tgt.setValue(Timing.UnitsOfTime.S); 081 break; 082 case MIN: 083 tgt.setValue(Timing.UnitsOfTime.MIN); 084 break; 085 case H: 086 tgt.setValue(Timing.UnitsOfTime.H); 087 break; 088 case D: 089 tgt.setValue(Timing.UnitsOfTime.D); 090 break; 091 case WK: 092 tgt.setValue(Timing.UnitsOfTime.WK); 093 break; 094 case MO: 095 tgt.setValue(Timing.UnitsOfTime.MO); 096 break; 097 case A: 098 tgt.setValue(Timing.UnitsOfTime.A); 099 break; 100 default: 101 tgt.setValue(Timing.UnitsOfTime.NULL); 102 break; 103 } 104} 105 return tgt; 106 } 107 108 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> src) throws FHIRException { 109 if (src == null || src.isEmpty()) return null; 110 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Timing.UnitsOfTimeEnumFactory()); 111 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 112 if (src.getValue() == null) { 113 tgt.setValue(null); 114} else { 115 switch(src.getValue()) { 116 case S: 117 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.S); 118 break; 119 case MIN: 120 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.MIN); 121 break; 122 case H: 123 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.H); 124 break; 125 case D: 126 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.D); 127 break; 128 case WK: 129 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.WK); 130 break; 131 case MO: 132 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.MO); 133 break; 134 case A: 135 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.A); 136 break; 137 default: 138 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.UnitsOfTime.NULL); 139 break; 140 } 141} 142 return tgt; 143 } 144 145 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Timing.EventTiming> src) throws FHIRException { 146 if (src == null || src.isEmpty()) return null; 147 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Timing.EventTimingEnumFactory()); 148 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 149 if (src.getValue() == null) { 150 tgt.setValue(null); 151} else { 152 switch(src.getValue()) { 153 case HS: 154 tgt.setValue(Timing.EventTiming.HS); 155 break; 156 case WAKE: 157 tgt.setValue(Timing.EventTiming.WAKE); 158 break; 159 case C: 160 tgt.setValue(Timing.EventTiming.C); 161 break; 162 case CM: 163 tgt.setValue(Timing.EventTiming.CM); 164 break; 165 case CD: 166 tgt.setValue(Timing.EventTiming.CD); 167 break; 168 case CV: 169 tgt.setValue(Timing.EventTiming.CV); 170 break; 171 case AC: 172 tgt.setValue(Timing.EventTiming.AC); 173 break; 174 case ACM: 175 tgt.setValue(Timing.EventTiming.ACM); 176 break; 177 case ACD: 178 tgt.setValue(Timing.EventTiming.ACD); 179 break; 180 case ACV: 181 tgt.setValue(Timing.EventTiming.ACV); 182 break; 183 case PC: 184 tgt.setValue(Timing.EventTiming.PC); 185 break; 186 case PCM: 187 tgt.setValue(Timing.EventTiming.PCM); 188 break; 189 case PCD: 190 tgt.setValue(Timing.EventTiming.PCD); 191 break; 192 case PCV: 193 tgt.setValue(Timing.EventTiming.PCV); 194 break; 195 default: 196 tgt.setValue(Timing.EventTiming.NULL); 197 break; 198 } 199} 200 return tgt; 201 } 202 203 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> src) throws FHIRException { 204 if (src == null || src.isEmpty()) return null; 205 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Timing.EventTiming> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Timing.EventTimingEnumFactory()); 206 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 207 if (src.getValue() == null) { 208 tgt.setValue(null); 209} else { 210 switch(src.getValue()) { 211 case HS: 212 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.HS); 213 break; 214 case WAKE: 215 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.WAKE); 216 break; 217 case C: 218 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.C); 219 break; 220 case CM: 221 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.CM); 222 break; 223 case CD: 224 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.CD); 225 break; 226 case CV: 227 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.CV); 228 break; 229 case AC: 230 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.AC); 231 break; 232 case ACM: 233 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.ACM); 234 break; 235 case ACD: 236 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.ACD); 237 break; 238 case ACV: 239 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.ACV); 240 break; 241 case PC: 242 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.PC); 243 break; 244 case PCM: 245 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.PCM); 246 break; 247 case PCD: 248 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.PCD); 249 break; 250 case PCV: 251 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.PCV); 252 break; 253 default: 254 tgt.setValue(org.hl7.fhir.dstu2.model.Timing.EventTiming.NULL); 255 break; 256 } 257} 258 return tgt; 259 } 260}