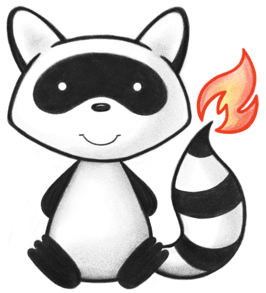
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Instant10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.PositiveInt10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.UnsignedInt10_40; 011import org.hl7.fhir.dstu2.model.Appointment; 012import org.hl7.fhir.dstu2.model.Enumeration; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class Appointment10_40 { 016 017 public static org.hl7.fhir.r4.model.Appointment convertAppointment(org.hl7.fhir.dstu2.model.Appointment src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.r4.model.Appointment tgt = new org.hl7.fhir.r4.model.Appointment(); 021 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 024 if (src.hasStatus()) 025 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 026 if (src.hasType()) 027 tgt.addServiceType(CodeableConcept10_40.convertCodeableConcept(src.getType())); 028 if (src.hasPriorityElement()) 029 tgt.setPriorityElement(UnsignedInt10_40.convertUnsignedInt(src.getPriorityElement())); 030 if (src.hasDescriptionElement()) 031 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 032 if (src.hasStartElement()) 033 tgt.setStartElement(Instant10_40.convertInstant(src.getStartElement())); 034 if (src.hasEndElement()) 035 tgt.setEndElement(Instant10_40.convertInstant(src.getEndElement())); 036 if (src.hasMinutesDurationElement()) 037 tgt.setMinutesDurationElement(PositiveInt10_40.convertPositiveInt(src.getMinutesDurationElement())); 038 for (org.hl7.fhir.dstu2.model.Reference t : src.getSlot()) tgt.addSlot(Reference10_40.convertReference(t)); 039 if (src.hasCommentElement()) 040 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 041 for (org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 042 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.dstu2.model.Appointment convertAppointment(org.hl7.fhir.r4.model.Appointment src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.dstu2.model.Appointment tgt = new org.hl7.fhir.dstu2.model.Appointment(); 050 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 055 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceType()) 056 tgt.setType(CodeableConcept10_40.convertCodeableConcept(t)); 057 if (src.hasPriorityElement()) 058 tgt.setPriorityElement(UnsignedInt10_40.convertUnsignedInt(src.getPriorityElement())); 059 if (src.hasDescriptionElement()) 060 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 061 if (src.hasStartElement()) 062 tgt.setStartElement(Instant10_40.convertInstant(src.getStartElement())); 063 if (src.hasEndElement()) 064 tgt.setEndElement(Instant10_40.convertInstant(src.getEndElement())); 065 if (src.hasMinutesDurationElement()) 066 tgt.setMinutesDurationElement(PositiveInt10_40.convertPositiveInt(src.getMinutesDurationElement())); 067 for (org.hl7.fhir.r4.model.Reference t : src.getSlot()) tgt.addSlot(Reference10_40.convertReference(t)); 068 if (src.hasCommentElement()) 069 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 070 for (org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 071 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 072 return tgt; 073 } 074 075 public static org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 076 if (src == null || src.isEmpty()) 077 return null; 078 org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent(); 079 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 080 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getType()) 081 tgt.addType(CodeableConcept10_40.convertCodeableConcept(t)); 082 if (src.hasActor()) 083 tgt.setActor(Reference10_40.convertReference(src.getActor())); 084 if (src.hasRequired()) 085 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 086 if (src.hasStatus()) 087 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent(); 095 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 096 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 097 tgt.addType(CodeableConcept10_40.convertCodeableConcept(t)); 098 if (src.hasActor()) 099 tgt.setActor(Reference10_40.convertReference(src.getActor())); 100 if (src.hasRequired()) 101 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 102 if (src.hasStatus()) 103 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 104 return tgt; 105 } 106 107 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> src) throws FHIRException { 108 if (src == null || src.isEmpty()) 109 return null; 110 Enumeration<Appointment.AppointmentStatus> tgt = new Enumeration<>(new Appointment.AppointmentStatusEnumFactory()); 111 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 112 if (src.getValue() == null) { 113 tgt.setValue(null); 114 } else { 115 switch (src.getValue()) { 116 case PROPOSED: 117 tgt.setValue(Appointment.AppointmentStatus.PROPOSED); 118 break; 119 case PENDING: 120 tgt.setValue(Appointment.AppointmentStatus.PENDING); 121 break; 122 case BOOKED: 123 tgt.setValue(Appointment.AppointmentStatus.BOOKED); 124 break; 125 case ARRIVED: 126 tgt.setValue(Appointment.AppointmentStatus.ARRIVED); 127 break; 128 case FULFILLED: 129 tgt.setValue(Appointment.AppointmentStatus.FULFILLED); 130 break; 131 case CANCELLED: 132 tgt.setValue(Appointment.AppointmentStatus.CANCELLED); 133 break; 134 case NOSHOW: 135 tgt.setValue(Appointment.AppointmentStatus.NOSHOW); 136 break; 137 default: 138 tgt.setValue(Appointment.AppointmentStatus.NULL); 139 break; 140 } 141 } 142 return tgt; 143 } 144 145 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus> src) throws FHIRException { 146 if (src == null || src.isEmpty()) 147 return null; 148 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.AppointmentStatusEnumFactory()); 149 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 150 if (src.getValue() == null) { 151 tgt.setValue(null); 152 } else { 153 switch (src.getValue()) { 154 case PROPOSED: 155 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.PROPOSED); 156 break; 157 case PENDING: 158 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.PENDING); 159 break; 160 case BOOKED: 161 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.BOOKED); 162 break; 163 case ARRIVED: 164 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.ARRIVED); 165 break; 166 case FULFILLED: 167 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.FULFILLED); 168 break; 169 case CANCELLED: 170 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.CANCELLED); 171 break; 172 case NOSHOW: 173 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.NOSHOW); 174 break; 175 default: 176 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.NULL); 177 break; 178 } 179 } 180 return tgt; 181 } 182 183 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> convertParticipantRequired(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipantRequired> src) throws FHIRException { 184 if (src == null || src.isEmpty()) 185 return null; 186 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.ParticipantRequiredEnumFactory()); 187 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 188 if (src.getValue() == null) { 189 tgt.setValue(null); 190 } else { 191 switch (src.getValue()) { 192 case REQUIRED: 193 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.REQUIRED); 194 break; 195 case OPTIONAL: 196 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.OPTIONAL); 197 break; 198 case INFORMATIONONLY: 199 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.INFORMATIONONLY); 200 break; 201 default: 202 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.NULL); 203 break; 204 } 205 } 206 return tgt; 207 } 208 209 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipantRequired> convertParticipantRequired(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> src) throws FHIRException { 210 if (src == null || src.isEmpty()) 211 return null; 212 Enumeration<Appointment.ParticipantRequired> tgt = new Enumeration<>(new Appointment.ParticipantRequiredEnumFactory()); 213 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 214 if (src.getValue() == null) { 215 tgt.setValue(null); 216 } else { 217 switch (src.getValue()) { 218 case REQUIRED: 219 tgt.setValue(Appointment.ParticipantRequired.REQUIRED); 220 break; 221 case OPTIONAL: 222 tgt.setValue(Appointment.ParticipantRequired.OPTIONAL); 223 break; 224 case INFORMATIONONLY: 225 tgt.setValue(Appointment.ParticipantRequired.INFORMATIONONLY); 226 break; 227 default: 228 tgt.setValue(Appointment.ParticipantRequired.NULL); 229 break; 230 } 231 } 232 return tgt; 233 } 234 235 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> src) throws FHIRException { 236 if (src == null || src.isEmpty()) 237 return null; 238 Enumeration<Appointment.ParticipationStatus> tgt = new Enumeration<>(new Appointment.ParticipationStatusEnumFactory()); 239 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 240 if (src.getValue() == null) { 241 tgt.setValue(null); 242 } else { 243 switch (src.getValue()) { 244 case ACCEPTED: 245 tgt.setValue(Appointment.ParticipationStatus.ACCEPTED); 246 break; 247 case DECLINED: 248 tgt.setValue(Appointment.ParticipationStatus.DECLINED); 249 break; 250 case TENTATIVE: 251 tgt.setValue(Appointment.ParticipationStatus.TENTATIVE); 252 break; 253 case NEEDSACTION: 254 tgt.setValue(Appointment.ParticipationStatus.NEEDSACTION); 255 break; 256 default: 257 tgt.setValue(Appointment.ParticipationStatus.NULL); 258 break; 259 } 260 } 261 return tgt; 262 } 263 264 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus> src) throws FHIRException { 265 if (src == null || src.isEmpty()) 266 return null; 267 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.ParticipationStatusEnumFactory()); 268 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 269 if (src.getValue() == null) { 270 tgt.setValue(null); 271 } else { 272 switch (src.getValue()) { 273 case ACCEPTED: 274 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.ACCEPTED); 275 break; 276 case DECLINED: 277 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.DECLINED); 278 break; 279 case TENTATIVE: 280 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.TENTATIVE); 281 break; 282 case NEEDSACTION: 283 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.NEEDSACTION); 284 break; 285 default: 286 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.NULL); 287 break; 288 } 289 } 290 return tgt; 291 } 292}