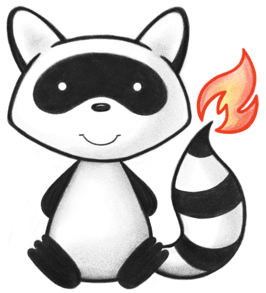
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Instant10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 009import org.hl7.fhir.dstu2.model.AppointmentResponse; 010import org.hl7.fhir.dstu2.model.Enumeration; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class AppointmentResponse10_40 { 014 015 public static org.hl7.fhir.r4.model.AppointmentResponse convertAppointmentResponse(org.hl7.fhir.dstu2.model.AppointmentResponse src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 org.hl7.fhir.r4.model.AppointmentResponse tgt = new org.hl7.fhir.r4.model.AppointmentResponse(); 019 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 020 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 021 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 022 if (src.hasAppointment()) 023 tgt.setAppointment(Reference10_40.convertReference(src.getAppointment())); 024 if (src.hasStartElement()) 025 tgt.setStartElement(Instant10_40.convertInstant(src.getStartElement())); 026 if (src.hasEndElement()) 027 tgt.setEndElement(Instant10_40.convertInstant(src.getEndElement())); 028 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getParticipantType()) 029 tgt.addParticipantType(CodeableConcept10_40.convertCodeableConcept(t)); 030 if (src.hasActor()) 031 tgt.setActor(Reference10_40.convertReference(src.getActor())); 032 if (src.hasParticipantStatus()) 033 tgt.setParticipantStatusElement(convertParticipantStatus(src.getParticipantStatusElement())); 034 if (src.hasCommentElement()) 035 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 036 return tgt; 037 } 038 039 public static org.hl7.fhir.dstu2.model.AppointmentResponse convertAppointmentResponse(org.hl7.fhir.r4.model.AppointmentResponse src) throws FHIRException { 040 if (src == null || src.isEmpty()) 041 return null; 042 org.hl7.fhir.dstu2.model.AppointmentResponse tgt = new org.hl7.fhir.dstu2.model.AppointmentResponse(); 043 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 044 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 045 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 046 if (src.hasAppointment()) 047 tgt.setAppointment(Reference10_40.convertReference(src.getAppointment())); 048 if (src.hasStartElement()) 049 tgt.setStartElement(Instant10_40.convertInstant(src.getStartElement())); 050 if (src.hasEndElement()) 051 tgt.setEndElement(Instant10_40.convertInstant(src.getEndElement())); 052 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getParticipantType()) 053 tgt.addParticipantType(CodeableConcept10_40.convertCodeableConcept(t)); 054 if (src.hasActor()) 055 tgt.setActor(Reference10_40.convertReference(src.getActor())); 056 if (src.hasParticipantStatus()) 057 tgt.setParticipantStatusElement(convertParticipantStatus(src.getParticipantStatusElement())); 058 if (src.hasCommentElement()) 059 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 060 return tgt; 061 } 062 063 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AppointmentResponse.ParticipantStatus> convertParticipantStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus> src) throws FHIRException { 064 if (src == null || src.isEmpty()) 065 return null; 066 Enumeration<AppointmentResponse.ParticipantStatus> tgt = new Enumeration<>(new AppointmentResponse.ParticipantStatusEnumFactory()); 067 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 068 if (src.getValue() == null) { 069 tgt.setValue(null); 070 } else { 071 switch (src.getValue()) { 072 case ACCEPTED: 073 tgt.setValue(AppointmentResponse.ParticipantStatus.ACCEPTED); 074 break; 075 case DECLINED: 076 tgt.setValue(AppointmentResponse.ParticipantStatus.DECLINED); 077 break; 078 case TENTATIVE: 079 tgt.setValue(AppointmentResponse.ParticipantStatus.TENTATIVE); 080 break; 081 case NEEDSACTION: 082 tgt.setValue(AppointmentResponse.ParticipantStatus.NEEDSACTION); 083 break; 084 default: 085 tgt.setValue(AppointmentResponse.ParticipantStatus.NULL); 086 break; 087 } 088 } 089 return tgt; 090 } 091 092 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus> convertParticipantStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AppointmentResponse.ParticipantStatus> src) throws FHIRException { 093 if (src == null || src.isEmpty()) 094 return null; 095 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatusEnumFactory()); 096 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 097 if (src.getValue() == null) { 098 tgt.setValue(null); 099 } else { 100 switch (src.getValue()) { 101 case ACCEPTED: 102 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.ACCEPTED); 103 break; 104 case DECLINED: 105 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.DECLINED); 106 break; 107 case TENTATIVE: 108 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.TENTATIVE); 109 break; 110 case INPROCESS: 111 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.ACCEPTED); 112 break; 113 case COMPLETED: 114 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.ACCEPTED); 115 break; 116 case NEEDSACTION: 117 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.NEEDSACTION); 118 break; 119 default: 120 tgt.setValue(org.hl7.fhir.r4.model.AppointmentResponse.ParticipantStatus.NULL); 121 break; 122 } 123 } 124 return tgt; 125 } 126}