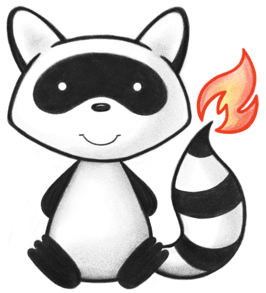
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Base64Binary10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class AuditEvent10_40 { 014 015 public static org.hl7.fhir.r4.model.AuditEvent convertAuditEvent(org.hl7.fhir.dstu2.model.AuditEvent src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 org.hl7.fhir.r4.model.AuditEvent tgt = new org.hl7.fhir.r4.model.AuditEvent(); 019 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 020 if (src.hasEvent()) { 021 if (src.hasType()) 022 tgt.setType(Coding10_40.convertCoding(src.getEvent().getType())); 023 for (org.hl7.fhir.dstu2.model.Coding t : src.getEvent().getSubtype()) 024 tgt.addSubtype(Coding10_40.convertCoding(t)); 025 tgt.setActionElement(convertAuditEventAction(src.getEvent().getActionElement())); 026 tgt.setRecorded(src.getEvent().getDateTime()); 027 tgt.setOutcomeElement(convertAuditEventOutcome(src.getEvent().getOutcomeElement())); 028 tgt.setOutcomeDesc(src.getEvent().getOutcomeDesc()); 029 for (org.hl7.fhir.dstu2.model.Coding t : src.getEvent().getPurposeOfEvent()) 030 tgt.addPurposeOfEvent().addCoding(Coding10_40.convertCoding(t)); 031 } 032 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent t : src.getParticipant()) 033 tgt.addAgent(convertAuditEventAgentComponent(t)); 034 if (src.hasSource()) 035 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 036 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent t : src.getObject()) 037 tgt.addEntity(convertAuditEventEntityComponent(t)); 038 return tgt; 039 } 040 041 public static org.hl7.fhir.dstu2.model.AuditEvent convertAuditEvent(org.hl7.fhir.r4.model.AuditEvent src) throws FHIRException { 042 if (src == null || src.isEmpty()) 043 return null; 044 org.hl7.fhir.dstu2.model.AuditEvent tgt = new org.hl7.fhir.dstu2.model.AuditEvent(); 045 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 046 tgt.getEvent().setType(Coding10_40.convertCoding(src.getType())); 047 for (org.hl7.fhir.r4.model.Coding t : src.getSubtype()) tgt.getEvent().addSubtype(Coding10_40.convertCoding(t)); 048 tgt.getEvent().setActionElement(convertAuditEventAction(src.getActionElement())); 049 tgt.getEvent().setDateTime(src.getRecorded()); 050 tgt.getEvent().setOutcomeElement(convertAuditEventOutcome(src.getOutcomeElement())); 051 tgt.getEvent().setOutcomeDesc(src.getOutcomeDesc()); 052 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getPurposeOfEvent()) 053 for (org.hl7.fhir.r4.model.Coding cc : t.getCoding()) 054 tgt.getEvent().addPurposeOfEvent(Coding10_40.convertCoding(cc)); 055 for (org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentComponent t : src.getAgent()) 056 tgt.addParticipant(convertAuditEventAgentComponent(t)); 057 if (src.hasSource()) 058 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 059 for (org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityComponent t : src.getEntity()) 060 tgt.addObject(convertAuditEventEntityComponent(t)); 061 return tgt; 062 } 063 064 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventAction> src) throws FHIRException { 065 if (src == null || src.isEmpty()) 066 return null; 067 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventActionEnumFactory()); 068 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 069 switch (src.getValue()) { 070 case C: 071 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.C); 072 break; 073 case R: 074 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.R); 075 break; 076 case U: 077 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.U); 078 break; 079 case D: 080 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.D); 081 break; 082 case E: 083 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.E); 084 break; 085 default: 086 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.NULL); 087 break; 088 } 089 return tgt; 090 } 091 092 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> src) throws FHIRException { 093 if (src == null || src.isEmpty()) 094 return null; 095 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AuditEvent.AuditEventActionEnumFactory()); 096 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 097 switch (src.getValue()) { 098 case C: 099 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAction.C); 100 break; 101 case R: 102 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAction.R); 103 break; 104 case U: 105 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAction.U); 106 break; 107 case D: 108 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAction.D); 109 break; 110 case E: 111 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAction.E); 112 break; 113 default: 114 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAction.NULL); 115 break; 116 } 117 return tgt; 118 } 119 120 public static org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentComponent convertAuditEventAgentComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent src) throws FHIRException { 121 if (src == null || src.isEmpty()) 122 return null; 123 org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentComponent tgt = new org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentComponent(); 124 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 125 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getRole()) 126 tgt.addRole(CodeableConcept10_40.convertCodeableConcept(t)); 127 if (src.hasReference()) 128 tgt.setWho(Reference10_40.convertReference(src.getReference())); 129 if (src.hasUserId()) 130 tgt.getWho().setIdentifier(Identifier10_40.convertIdentifier(src.getUserId())); 131 if (src.hasAltIdElement()) 132 tgt.setAltIdElement(String10_40.convertString(src.getAltIdElement())); 133 if (src.hasNameElement()) 134 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 135 if (src.hasRequestorElement()) 136 tgt.setRequestorElement(Boolean10_40.convertBoolean(src.getRequestorElement())); 137 if (src.hasLocation()) 138 tgt.setLocation(Reference10_40.convertReference(src.getLocation())); 139 for (org.hl7.fhir.dstu2.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 140 if (src.hasMedia()) 141 tgt.setMedia(Coding10_40.convertCoding(src.getMedia())); 142 if (src.hasNetwork()) 143 tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 144 for (org.hl7.fhir.dstu2.model.Coding t : src.getPurposeOfUse()) 145 tgt.addPurposeOfUse().addCoding(Coding10_40.convertCoding(t)); 146 return tgt; 147 } 148 149 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent convertAuditEventAgentComponent(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentComponent src) throws FHIRException { 150 if (src == null || src.isEmpty()) 151 return null; 152 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent(); 153 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 154 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getRole()) 155 tgt.addRole(CodeableConcept10_40.convertCodeableConcept(t)); 156 if (src.hasWho()) { 157 if (src.getWho().hasIdentifier()) 158 tgt.setUserId(Identifier10_40.convertIdentifier(src.getWho().getIdentifier())); 159 if (src.getWho().hasReference() || src.getWho().hasDisplay() || src.getWho().hasExtension() || src.getWho().hasId()) 160 tgt.setReference(Reference10_40.convertReference(src.getWho())); 161 } 162 if (src.hasAltIdElement()) 163 tgt.setAltIdElement(String10_40.convertString(src.getAltIdElement())); 164 if (src.hasNameElement()) 165 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 166 if (src.hasRequestorElement()) 167 tgt.setRequestorElement(Boolean10_40.convertBoolean(src.getRequestorElement())); 168 if (src.hasLocation()) 169 tgt.setLocation(Reference10_40.convertReference(src.getLocation())); 170 for (org.hl7.fhir.r4.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 171 if (src.hasMedia()) 172 tgt.setMedia(Coding10_40.convertCoding(src.getMedia())); 173 if (src.hasNetwork()) 174 tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 175 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getPurposeOfUse()) 176 for (org.hl7.fhir.r4.model.Coding cc : t.getCoding()) tgt.addPurposeOfUse(Coding10_40.convertCoding(cc)); 177 return tgt; 178 } 179 180 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkComponent src) throws FHIRException { 181 if (src == null || src.isEmpty()) 182 return null; 183 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent(); 184 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 185 if (src.hasAddressElement()) 186 tgt.setAddressElement(String10_40.convertString(src.getAddressElement())); 187 if (src.hasType()) 188 tgt.setTypeElement(convertAuditEventParticipantNetworkType(src.getTypeElement())); 189 return tgt; 190 } 191 192 public static org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent src) throws FHIRException { 193 if (src == null || src.isEmpty()) 194 return null; 195 org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkComponent tgt = new org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkComponent(); 196 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 197 if (src.hasAddressElement()) 198 tgt.setAddressElement(String10_40.convertString(src.getAddressElement())); 199 if (src.hasType()) 200 tgt.setTypeElement(convertAuditEventParticipantNetworkType(src.getTypeElement())); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityComponent convertAuditEventEntityComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent src) throws FHIRException { 205 if (src == null || src.isEmpty()) 206 return null; 207 org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityComponent tgt = new org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityComponent(); 208 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 209 if (src.hasIdentifier()) 210 tgt.getWhat().setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 211 if (src.hasReference()) 212 tgt.setWhat(Reference10_40.convertReference(src.getReference())); 213 if (src.hasType()) 214 tgt.setType(Coding10_40.convertCoding(src.getType())); 215 if (src.hasRole()) 216 tgt.setRole(Coding10_40.convertCoding(src.getRole())); 217 if (src.hasLifecycle()) 218 tgt.setLifecycle(Coding10_40.convertCoding(src.getLifecycle())); 219 for (org.hl7.fhir.dstu2.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding10_40.convertCoding(t)); 220 if (src.hasNameElement()) 221 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 222 if (src.hasDescriptionElement()) 223 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 224 if (src.hasQueryElement()) 225 tgt.setQueryElement(Base64Binary10_40.convertBase64Binary(src.getQueryElement())); 226 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent t : src.getDetail()) 227 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 228 return tgt; 229 } 230 231 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent convertAuditEventEntityComponent(org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityComponent src) throws FHIRException { 232 if (src == null || src.isEmpty()) 233 return null; 234 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent(); 235 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 236 if (src.hasWhat()) { 237 if (src.getWhat().hasIdentifier()) 238 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getWhat().getIdentifier())); 239 if (src.getWhat().hasReference() || src.getWhat().hasDisplay() || src.getWhat().hasExtension() || src.getWhat().hasId()) 240 tgt.setReference(Reference10_40.convertReference(src.getWhat())); 241 } 242 if (src.hasType()) 243 tgt.setType(Coding10_40.convertCoding(src.getType())); 244 if (src.hasRole()) 245 tgt.setRole(Coding10_40.convertCoding(src.getRole())); 246 if (src.hasLifecycle()) 247 tgt.setLifecycle(Coding10_40.convertCoding(src.getLifecycle())); 248 for (org.hl7.fhir.r4.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding10_40.convertCoding(t)); 249 if (src.hasNameElement()) 250 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 251 if (src.hasDescriptionElement()) 252 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 253 if (src.hasQueryElement()) 254 tgt.setQueryElement(Base64Binary10_40.convertBase64Binary(src.getQueryElement())); 255 for (org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityDetailComponent t : src.getDetail()) 256 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 257 return tgt; 258 } 259 260 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityDetailComponent src) throws FHIRException { 261 if (src == null || src.isEmpty()) 262 return null; 263 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent(); 264 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 265 if (src.hasTypeElement()) 266 tgt.setTypeElement(String10_40.convertString(src.getTypeElement())); 267 if (src.hasValueStringType()) 268 tgt.setValue(src.getValueStringType().getValue().getBytes()); 269 else if (src.hasValueBase64BinaryType()) 270 tgt.setValue(src.getValueBase64BinaryType().getValue()); 271 return tgt; 272 } 273 274 public static org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent src) throws FHIRException { 275 if (src == null || src.isEmpty()) 276 return null; 277 org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityDetailComponent tgt = new org.hl7.fhir.r4.model.AuditEvent.AuditEventEntityDetailComponent(); 278 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 279 if (src.hasTypeElement()) 280 tgt.setTypeElement(String10_40.convertString(src.getTypeElement())); 281 if (src.hasValue()) 282 tgt.setValue(new org.hl7.fhir.r4.model.Base64BinaryType(src.getValue())); 283 return tgt; 284 } 285 286 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome> convertAuditEventOutcome(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome> src) throws FHIRException { 287 if (src == null || src.isEmpty()) 288 return null; 289 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcomeEnumFactory()); 290 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 291 switch (src.getValue()) { 292 case _0: 293 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome._0); 294 break; 295 case _4: 296 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome._4); 297 break; 298 case _8: 299 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome._8); 300 break; 301 case _12: 302 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome._12); 303 break; 304 default: 305 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome.NULL); 306 break; 307 } 308 return tgt; 309 } 310 311 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome> convertAuditEventOutcome(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventOutcome> src) throws FHIRException { 312 if (src == null || src.isEmpty()) 313 return null; 314 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcomeEnumFactory()); 315 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 316 switch (src.getValue()) { 317 case _0: 318 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._0); 319 break; 320 case _4: 321 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._4); 322 break; 323 case _8: 324 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._8); 325 break; 326 case _12: 327 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome._12); 328 break; 329 default: 330 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventOutcome.NULL); 331 break; 332 } 333 return tgt; 334 } 335 336 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> convertAuditEventParticipantNetworkType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType> src) throws FHIRException { 337 if (src == null || src.isEmpty()) 338 return null; 339 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkTypeEnumFactory()); 340 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 341 switch (src.getValue()) { 342 case _1: 343 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._1); 344 break; 345 case _2: 346 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._2); 347 break; 348 case _3: 349 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._3); 350 break; 351 case _4: 352 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._4); 353 break; 354 case _5: 355 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._5); 356 break; 357 default: 358 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType.NULL); 359 break; 360 } 361 return tgt; 362 } 363 364 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType> convertAuditEventParticipantNetworkType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> src) throws FHIRException { 365 if (src == null || src.isEmpty()) 366 return null; 367 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkTypeEnumFactory()); 368 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 369 switch (src.getValue()) { 370 case _1: 371 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType._1); 372 break; 373 case _2: 374 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType._2); 375 break; 376 case _3: 377 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType._3); 378 break; 379 case _4: 380 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType._4); 381 break; 382 case _5: 383 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType._5); 384 break; 385 default: 386 tgt.setValue(org.hl7.fhir.r4.model.AuditEvent.AuditEventAgentNetworkType.NULL); 387 break; 388 } 389 return tgt; 390 } 391 392 public static org.hl7.fhir.r4.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 393 if (src == null || src.isEmpty()) 394 return null; 395 org.hl7.fhir.r4.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.r4.model.AuditEvent.AuditEventSourceComponent(); 396 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 397 if (src.hasSiteElement()) 398 tgt.setSiteElement(String10_40.convertString(src.getSiteElement())); 399 if (src.hasIdentifier()) 400 tgt.getObserver().setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 401 for (org.hl7.fhir.dstu2.model.Coding t : src.getType()) tgt.addType(Coding10_40.convertCoding(t)); 402 return tgt; 403 } 404 405 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.r4.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 406 if (src == null || src.isEmpty()) 407 return null; 408 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent(); 409 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 410 if (src.hasSiteElement()) 411 tgt.setSiteElement(String10_40.convertString(src.getSiteElement())); 412 if (src.hasObserver()) 413 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getObserver().getIdentifier())); 414 for (org.hl7.fhir.r4.model.Coding t : src.getType()) tgt.addType(Coding10_40.convertCoding(t)); 415 return tgt; 416 } 417}