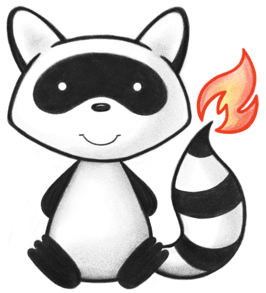
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_40; 004import org.hl7.fhir.convertors.context.ConversionContext10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Signature10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Decimal10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Instant10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.UnsignedInt10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 011import org.hl7.fhir.dstu2.model.Bundle; 012import org.hl7.fhir.dstu2.model.Enumeration; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.utilities.FhirPublication; 015 016public class Bundle10_40 { 017 018 public static org.hl7.fhir.r4.model.Bundle convertBundle(org.hl7.fhir.dstu2.model.Bundle src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 org.hl7.fhir.r4.model.Bundle tgt = new org.hl7.fhir.r4.model.Bundle(); 022 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyResource(src, tgt); 023 if (src.hasType()) 024 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 025 if (src.hasTotal()) 026 tgt.setTotalElement(UnsignedInt10_40.convertUnsignedInt(src.getTotalElement())); 027 for (org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent t : src.getLink()) 028 tgt.addLink(convertBundleLinkComponent(t)); 029 for (org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent t : src.getEntry()) 030 tgt.addEntry(convertBundleEntryComponent(t)); 031 if (src.hasSignature()) 032 tgt.setSignature(Signature10_40.convertSignature(src.getSignature())); 033 return tgt; 034 } 035 036 public static org.hl7.fhir.dstu2.model.Bundle convertBundle(org.hl7.fhir.r4.model.Bundle src, BaseAdvisor_10_40 advisor) throws FHIRException { 037 if (src == null || src.isEmpty()) 038 return null; 039 org.hl7.fhir.dstu2.model.Bundle tgt = new org.hl7.fhir.dstu2.model.Bundle(); 040 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyResource(src, tgt); 041 if (src.hasType()) 042 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 043 if (src.hasTotal()) 044 tgt.setTotalElement(UnsignedInt10_40.convertUnsignedInt(src.getTotalElement())); 045 for (org.hl7.fhir.r4.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 046 for (org.hl7.fhir.r4.model.Bundle.BundleEntryComponent t : src.getEntry()) 047 tgt.addEntry(convertBundleEntryComponent(t, advisor)); 048 if (src.hasSignature()) 049 tgt.setSignature(Signature10_40.convertSignature(src.getSignature())); 050 return tgt; 051 } 052 053 public static org.hl7.fhir.dstu2.model.Bundle convertBundle(org.hl7.fhir.r4.model.Bundle src) throws FHIRException { 054 return convertBundle(src, null); 055 } 056 057 public static org.hl7.fhir.r4.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent src) throws FHIRException { 058 if (src == null || src.isEmpty()) 059 return null; 060 org.hl7.fhir.r4.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryComponent(); 061 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 062 for (org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent t : src.getLink()) 063 tgt.addLink(convertBundleLinkComponent(t)); 064 if (src.hasFullUrlElement()) 065 tgt.setFullUrlElement(Uri10_40.convertUri(src.getFullUrlElement())); 066 if (src.hasResource()) 067 tgt.setResource(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertResource(src.getResource())); 068 if (src.hasSearch()) 069 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 070 if (src.hasRequest()) 071 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 072 if (src.hasResponse()) 073 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 074 return tgt; 075 } 076 077 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryComponent src, BaseAdvisor_10_40 advisor) throws FHIRException { 078 if (src == null || src.isEmpty()) 079 return null; 080 if (advisor.ignoreEntry(src, FhirPublication.DSTU2)) 081 return null; 082 org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent(); 083 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 084 for (org.hl7.fhir.r4.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 085 if (src.hasFullUrlElement()) 086 tgt.setFullUrlElement(Uri10_40.convertUri(src.getFullUrlElement())); 087 if (src.hasResource()) 088 tgt.setResource(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertResource(src.getResource())); 089 if (src.hasSearch()) 090 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 091 if (src.hasRequest()) 092 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 093 if (src.hasResponse()) 094 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryComponent src) throws FHIRException { 099 return convertBundleEntryComponent(src, null); 100 } 101 102 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 103 if (src == null || src.isEmpty()) 104 return null; 105 org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent(); 106 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 107 if (src.hasMethod()) 108 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 109 if (src.hasUrlElement()) 110 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 111 if (src.hasIfNoneMatchElement()) 112 tgt.setIfNoneMatchElement(String10_40.convertString(src.getIfNoneMatchElement())); 113 if (src.hasIfModifiedSinceElement()) 114 tgt.setIfModifiedSinceElement(Instant10_40.convertInstant(src.getIfModifiedSinceElement())); 115 if (src.hasIfMatchElement()) 116 tgt.setIfMatchElement(String10_40.convertString(src.getIfMatchElement())); 117 if (src.hasIfNoneExistElement()) 118 tgt.setIfNoneExistElement(String10_40.convertString(src.getIfNoneExistElement())); 119 return tgt; 120 } 121 122 public static org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 123 if (src == null || src.isEmpty()) 124 return null; 125 org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryRequestComponent(); 126 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 127 if (src.hasMethod()) 128 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 129 if (src.hasUrlElement()) 130 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 131 if (src.hasIfNoneMatchElement()) 132 tgt.setIfNoneMatchElement(String10_40.convertString(src.getIfNoneMatchElement())); 133 if (src.hasIfModifiedSinceElement()) 134 tgt.setIfModifiedSinceElement(Instant10_40.convertInstant(src.getIfModifiedSinceElement())); 135 if (src.hasIfMatchElement()) 136 tgt.setIfMatchElement(String10_40.convertString(src.getIfMatchElement())); 137 if (src.hasIfNoneExistElement()) 138 tgt.setIfNoneExistElement(String10_40.convertString(src.getIfNoneExistElement())); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 143 if (src == null || src.isEmpty()) 144 return null; 145 org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent(); 146 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 147 if (src.hasStatusElement()) 148 tgt.setStatusElement(String10_40.convertString(src.getStatusElement())); 149 if (src.hasLocationElement()) 150 tgt.setLocationElement(Uri10_40.convertUri(src.getLocationElement())); 151 if (src.hasEtagElement()) 152 tgt.setEtagElement(String10_40.convertString(src.getEtagElement())); 153 if (src.hasLastModifiedElement()) 154 tgt.setLastModifiedElement(Instant10_40.convertInstant(src.getLastModifiedElement())); 155 return tgt; 156 } 157 158 public static org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 159 if (src == null || src.isEmpty()) 160 return null; 161 org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntryResponseComponent(); 162 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 163 if (src.hasStatusElement()) 164 tgt.setStatusElement(String10_40.convertString(src.getStatusElement())); 165 if (src.hasLocationElement()) 166 tgt.setLocationElement(Uri10_40.convertUri(src.getLocationElement())); 167 if (src.hasEtagElement()) 168 tgt.setEtagElement(String10_40.convertString(src.getEtagElement())); 169 if (src.hasLastModifiedElement()) 170 tgt.setLastModifiedElement(Instant10_40.convertInstant(src.getLastModifiedElement())); 171 return tgt; 172 } 173 174 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 175 if (src == null || src.isEmpty()) 176 return null; 177 org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent(); 178 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 179 if (src.hasMode()) 180 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 181 if (src.hasScoreElement()) 182 tgt.setScoreElement(Decimal10_40.convertDecimal(src.getScoreElement())); 183 return tgt; 184 } 185 186 public static org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 187 if (src == null || src.isEmpty()) 188 return null; 189 org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleEntrySearchComponent(); 190 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 191 if (src.hasMode()) 192 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 193 if (src.hasScoreElement()) 194 tgt.setScoreElement(Decimal10_40.convertDecimal(src.getScoreElement())); 195 return tgt; 196 } 197 198 public static org.hl7.fhir.r4.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent src) throws FHIRException { 199 if (src == null || src.isEmpty()) 200 return null; 201 org.hl7.fhir.r4.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r4.model.Bundle.BundleLinkComponent(); 202 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 203 if (src.hasRelationElement()) 204 tgt.setRelationElement(String10_40.convertString(src.getRelationElement())); 205 if (src.hasUrlElement()) 206 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 207 return tgt; 208 } 209 210 public static org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r4.model.Bundle.BundleLinkComponent src) throws FHIRException { 211 if (src == null || src.isEmpty()) 212 return null; 213 org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent(); 214 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 215 if (src.hasRelationElement()) 216 tgt.setRelationElement(String10_40.convertString(src.getRelationElement())); 217 if (src.hasUrlElement()) 218 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 219 return tgt; 220 } 221 222 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> src) throws FHIRException { 223 if (src == null || src.isEmpty()) 224 return null; 225 Enumeration<Bundle.BundleType> tgt = new Enumeration<>(new Bundle.BundleTypeEnumFactory()); 226 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 227 if (src.getValue() == null) { 228 tgt.setValue(null); 229 } else { 230 switch (src.getValue()) { 231 case DOCUMENT: 232 tgt.setValue(Bundle.BundleType.DOCUMENT); 233 break; 234 case MESSAGE: 235 tgt.setValue(Bundle.BundleType.MESSAGE); 236 break; 237 case TRANSACTION: 238 tgt.setValue(Bundle.BundleType.TRANSACTION); 239 break; 240 case TRANSACTIONRESPONSE: 241 tgt.setValue(Bundle.BundleType.TRANSACTIONRESPONSE); 242 break; 243 case BATCH: 244 tgt.setValue(Bundle.BundleType.BATCH); 245 break; 246 case BATCHRESPONSE: 247 tgt.setValue(Bundle.BundleType.BATCHRESPONSE); 248 break; 249 case HISTORY: 250 tgt.setValue(Bundle.BundleType.HISTORY); 251 break; 252 case SEARCHSET: 253 tgt.setValue(Bundle.BundleType.SEARCHSET); 254 break; 255 case COLLECTION: 256 tgt.setValue(Bundle.BundleType.COLLECTION); 257 break; 258 default: 259 tgt.setValue(Bundle.BundleType.NULL); 260 break; 261 } 262 } 263 return tgt; 264 } 265 266 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> src) throws FHIRException { 267 if (src == null || src.isEmpty()) 268 return null; 269 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.BundleType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.BundleTypeEnumFactory()); 270 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 271 if (src.getValue() == null) { 272 tgt.setValue(null); 273 } else { 274 switch (src.getValue()) { 275 case DOCUMENT: 276 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.DOCUMENT); 277 break; 278 case MESSAGE: 279 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.MESSAGE); 280 break; 281 case TRANSACTION: 282 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.TRANSACTION); 283 break; 284 case TRANSACTIONRESPONSE: 285 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.TRANSACTIONRESPONSE); 286 break; 287 case BATCH: 288 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.BATCH); 289 break; 290 case BATCHRESPONSE: 291 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.BATCHRESPONSE); 292 break; 293 case HISTORY: 294 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.HISTORY); 295 break; 296 case SEARCHSET: 297 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.SEARCHSET); 298 break; 299 case COLLECTION: 300 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.COLLECTION); 301 break; 302 default: 303 tgt.setValue(org.hl7.fhir.r4.model.Bundle.BundleType.NULL); 304 break; 305 } 306 } 307 return tgt; 308 } 309 310 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> src) throws FHIRException { 311 if (src == null || src.isEmpty()) 312 return null; 313 Enumeration<Bundle.HTTPVerb> tgt = new Enumeration<>(new Bundle.HTTPVerbEnumFactory()); 314 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 315 if (src.getValue() == null) { 316 tgt.setValue(null); 317 } else { 318 switch (src.getValue()) { 319 case GET: 320 tgt.setValue(Bundle.HTTPVerb.GET); 321 break; 322 case POST: 323 tgt.setValue(Bundle.HTTPVerb.POST); 324 break; 325 case PUT: 326 tgt.setValue(Bundle.HTTPVerb.PUT); 327 break; 328 case DELETE: 329 tgt.setValue(Bundle.HTTPVerb.DELETE); 330 break; 331 default: 332 tgt.setValue(Bundle.HTTPVerb.NULL); 333 break; 334 } 335 } 336 return tgt; 337 } 338 339 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> src) throws FHIRException { 340 if (src == null || src.isEmpty()) 341 return null; 342 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.HTTPVerbEnumFactory()); 343 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 344 if (src.getValue() == null) { 345 tgt.setValue(null); 346 } else { 347 switch (src.getValue()) { 348 case GET: 349 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.GET); 350 break; 351 case POST: 352 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.POST); 353 break; 354 case PUT: 355 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.PUT); 356 break; 357 case DELETE: 358 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.DELETE); 359 break; 360 default: 361 tgt.setValue(org.hl7.fhir.r4.model.Bundle.HTTPVerb.NULL); 362 break; 363 } 364 } 365 return tgt; 366 } 367 368 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> src) throws FHIRException { 369 if (src == null || src.isEmpty()) 370 return null; 371 Enumeration<Bundle.SearchEntryMode> tgt = new Enumeration<>(new Bundle.SearchEntryModeEnumFactory()); 372 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 373 if (src.getValue() == null) { 374 tgt.setValue(null); 375 } else { 376 switch (src.getValue()) { 377 case MATCH: 378 tgt.setValue(Bundle.SearchEntryMode.MATCH); 379 break; 380 case INCLUDE: 381 tgt.setValue(Bundle.SearchEntryMode.INCLUDE); 382 break; 383 case OUTCOME: 384 tgt.setValue(Bundle.SearchEntryMode.OUTCOME); 385 break; 386 default: 387 tgt.setValue(Bundle.SearchEntryMode.NULL); 388 break; 389 } 390 } 391 return tgt; 392 } 393 394 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> src) throws FHIRException { 395 if (src == null || src.isEmpty()) 396 return null; 397 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Bundle.SearchEntryModeEnumFactory()); 398 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 399 if (src.getValue() == null) { 400 tgt.setValue(null); 401 } else { 402 switch (src.getValue()) { 403 case MATCH: 404 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.MATCH); 405 break; 406 case INCLUDE: 407 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.INCLUDE); 408 break; 409 case OUTCOME: 410 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.OUTCOME); 411 break; 412 default: 413 tgt.setValue(org.hl7.fhir.r4.model.Bundle.SearchEntryMode.NULL); 414 break; 415 } 416 } 417 return tgt; 418 } 419}