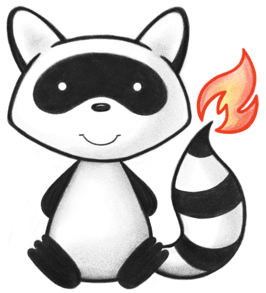
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Annotation10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Period10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.SimpleQuantity10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.CarePlan; 014import org.hl7.fhir.r4.model.Enumeration; 015 016public class CarePlan10_40 { 017 018 public static org.hl7.fhir.r4.model.CarePlan convertCarePlan(org.hl7.fhir.dstu2.model.CarePlan src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 org.hl7.fhir.r4.model.CarePlan tgt = new org.hl7.fhir.r4.model.CarePlan(); 022 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 023 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 024 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 025 if (src.hasSubject()) 026 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 027 if (src.hasStatus()) 028 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 029 if (src.hasContext()) 030 tgt.setEncounter(Reference10_40.convertReference(src.getContext())); 031 if (src.hasPeriod()) 032 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 033 for (org.hl7.fhir.dstu2.model.Reference t : src.getAuthor()) 034 if (!tgt.hasAuthor()) 035 tgt.setAuthor(Reference10_40.convertReference(t)); 036 else 037 tgt.addContributor(Reference10_40.convertReference(t)); 038 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCategory()) 039 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(t)); 040 if (src.hasDescriptionElement()) 041 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 042 for (org.hl7.fhir.dstu2.model.Reference t : src.getAddresses()) 043 tgt.addAddresses(Reference10_40.convertReference(t)); 044 for (org.hl7.fhir.dstu2.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_40.convertReference(t)); 045 for (org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 046 tgt.addActivity(convertCarePlanActivityComponent(t)); 047 return tgt; 048 } 049 050 public static org.hl7.fhir.dstu2.model.CarePlan convertCarePlan(org.hl7.fhir.r4.model.CarePlan src) throws FHIRException { 051 if (src == null || src.isEmpty()) 052 return null; 053 org.hl7.fhir.dstu2.model.CarePlan tgt = new org.hl7.fhir.dstu2.model.CarePlan(); 054 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 055 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 056 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 057 if (src.hasSubject()) 058 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 059 if (src.hasStatus()) 060 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 061 if (src.hasEncounter()) 062 tgt.setContext(Reference10_40.convertReference(src.getEncounter())); 063 if (src.hasPeriod()) 064 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 065 if (src.hasAuthor()) 066 tgt.addAuthor(Reference10_40.convertReference(src.getAuthor())); 067 for (org.hl7.fhir.r4.model.Reference t : src.getContributor()) tgt.addAuthor(Reference10_40.convertReference(t)); 068 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 069 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(t)); 070 if (src.hasDescriptionElement()) 071 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 072 for (org.hl7.fhir.r4.model.Reference t : src.getAddresses()) tgt.addAddresses(Reference10_40.convertReference(t)); 073 for (org.hl7.fhir.r4.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_40.convertReference(t)); 074 for (org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 075 tgt.addActivity(convertCarePlanActivityComponent(t)); 076 return tgt; 077 } 078 079 public static org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 080 if (src == null || src.isEmpty()) 081 return null; 082 org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent(); 083 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 084 for (org.hl7.fhir.dstu2.model.Annotation t : src.getProgress()) 085 tgt.addProgress(Annotation10_40.convertAnnotation(t)); 086 if (src.hasReference()) 087 tgt.setReference(Reference10_40.convertReference(src.getReference())); 088 if (src.hasDetail()) 089 tgt.setDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 090 return tgt; 091 } 092 093 public static org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 094 if (src == null || src.isEmpty()) 095 return null; 096 org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent(); 097 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 098 for (org.hl7.fhir.r4.model.Annotation t : src.getProgress()) tgt.addProgress(Annotation10_40.convertAnnotation(t)); 099 if (src.hasReference()) 100 tgt.setReference(Reference10_40.convertReference(src.getReference())); 101 if (src.hasDetail()) 102 tgt.setDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 103 return tgt; 104 } 105 106 public static org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 107 if (src == null || src.isEmpty()) 108 return null; 109 org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent(); 110 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 111 if (src.hasCode()) 112 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 113 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 114 tgt.addReasonCode(CodeableConcept10_40.convertCodeableConcept(t)); 115 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 116 tgt.addReasonReference(Reference10_40.convertReference(t)); 117 for (org.hl7.fhir.r4.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_40.convertReference(t)); 118 if (src.hasStatus()) 119 tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 120 if (src.hasDoNotPerformElement()) 121 tgt.setProhibitedElement(Boolean10_40.convertBoolean(src.getDoNotPerformElement())); 122 if (src.hasScheduled()) 123 tgt.setScheduled(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getScheduled())); 124 if (src.hasLocation()) 125 tgt.setLocation(Reference10_40.convertReference(src.getLocation())); 126 for (org.hl7.fhir.r4.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference10_40.convertReference(t)); 127 if (src.hasProduct()) 128 tgt.setProduct(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getProduct())); 129 if (src.hasDailyAmount()) 130 tgt.setDailyAmount(SimpleQuantity10_40.convertSimpleQuantity(src.getDailyAmount())); 131 if (src.hasQuantity()) 132 tgt.setQuantity(SimpleQuantity10_40.convertSimpleQuantity(src.getQuantity())); 133 if (src.hasDescriptionElement()) 134 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 135 return tgt; 136 } 137 138 public static org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.r4.model.CarePlan.CarePlanActivityDetailComponent(); 142 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 143 if (src.hasCode()) 144 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 145 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReasonCode()) 146 tgt.addReasonCode(CodeableConcept10_40.convertCodeableConcept(t)); 147 for (org.hl7.fhir.dstu2.model.Reference t : src.getReasonReference()) 148 tgt.addReasonReference(Reference10_40.convertReference(t)); 149 for (org.hl7.fhir.dstu2.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_40.convertReference(t)); 150 if (src.hasStatus()) 151 tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 152 if (src.hasProhibitedElement()) 153 tgt.setDoNotPerformElement(Boolean10_40.convertBoolean(src.getProhibitedElement())); 154 if (src.hasScheduled()) 155 tgt.setScheduled(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getScheduled())); 156 if (src.hasLocation()) 157 tgt.setLocation(Reference10_40.convertReference(src.getLocation())); 158 for (org.hl7.fhir.dstu2.model.Reference t : src.getPerformer()) 159 tgt.addPerformer(Reference10_40.convertReference(t)); 160 if (src.hasProduct()) 161 tgt.setProduct(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getProduct())); 162 if (src.hasDailyAmount()) 163 tgt.setDailyAmount(SimpleQuantity10_40.convertSimpleQuantity(src.getDailyAmount())); 164 if (src.hasQuantity()) 165 tgt.setQuantity(SimpleQuantity10_40.convertSimpleQuantity(src.getQuantity())); 166 if (src.hasDescriptionElement()) 167 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 168 return tgt; 169 } 170 171 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 172 if (src == null || src.isEmpty()) 173 return null; 174 Enumeration<CarePlan.CarePlanActivityStatus> tgt = new Enumeration<>(new CarePlan.CarePlanActivityStatusEnumFactory()); 175 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 176 if (src.getValue() == null) { 177 tgt.setValue(null); 178 } else { 179 switch (src.getValue()) { 180 case NOTSTARTED: 181 tgt.setValue(CarePlan.CarePlanActivityStatus.NOTSTARTED); 182 break; 183 case SCHEDULED: 184 tgt.setValue(CarePlan.CarePlanActivityStatus.SCHEDULED); 185 break; 186 case INPROGRESS: 187 tgt.setValue(CarePlan.CarePlanActivityStatus.INPROGRESS); 188 break; 189 case ONHOLD: 190 tgt.setValue(CarePlan.CarePlanActivityStatus.ONHOLD); 191 break; 192 case COMPLETED: 193 tgt.setValue(CarePlan.CarePlanActivityStatus.COMPLETED); 194 break; 195 case CANCELLED: 196 tgt.setValue(CarePlan.CarePlanActivityStatus.CANCELLED); 197 break; 198 default: 199 tgt.setValue(CarePlan.CarePlanActivityStatus.NULL); 200 break; 201 } 202 } 203 return tgt; 204 } 205 206 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 207 if (src == null || src.isEmpty()) 208 return null; 209 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatusEnumFactory()); 210 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 211 if (src.getValue() == null) { 212 tgt.setValue(null); 213 } else { 214 switch (src.getValue()) { 215 case NOTSTARTED: 216 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 217 break; 218 case SCHEDULED: 219 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 220 break; 221 case INPROGRESS: 222 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 223 break; 224 case ONHOLD: 225 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.ONHOLD); 226 break; 227 case COMPLETED: 228 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.COMPLETED); 229 break; 230 case CANCELLED: 231 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.CANCELLED); 232 break; 233 default: 234 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.NULL); 235 break; 236 } 237 } 238 return tgt; 239 } 240 241 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> src) throws FHIRException { 242 if (src == null || src.isEmpty()) 243 return null; 244 Enumeration<CarePlan.CarePlanStatus> tgt = new Enumeration<>(new CarePlan.CarePlanStatusEnumFactory()); 245 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 246 if (src.getValue() == null) { 247 tgt.setValue(null); 248 } else { 249 switch (src.getValue()) { 250 case PROPOSED: 251 tgt.setValue(CarePlan.CarePlanStatus.DRAFT); 252 break; 253 case DRAFT: 254 tgt.setValue(CarePlan.CarePlanStatus.DRAFT); 255 break; 256 case ACTIVE: 257 tgt.setValue(CarePlan.CarePlanStatus.ACTIVE); 258 break; 259 case COMPLETED: 260 tgt.setValue(CarePlan.CarePlanStatus.COMPLETED); 261 break; 262 case CANCELLED: 263 tgt.setValue(CarePlan.CarePlanStatus.REVOKED); 264 break; 265 default: 266 tgt.setValue(CarePlan.CarePlanStatus.NULL); 267 break; 268 } 269 } 270 return tgt; 271 } 272 273 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CarePlan.CarePlanStatus> src) throws FHIRException { 274 if (src == null || src.isEmpty()) 275 return null; 276 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatusEnumFactory()); 277 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 278 if (src.getValue() == null) { 279 tgt.setValue(null); 280 } else { 281 switch (src.getValue()) { 282 case DRAFT: 283 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.DRAFT); 284 break; 285 case ACTIVE: 286 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.ACTIVE); 287 break; 288 case COMPLETED: 289 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.COMPLETED); 290 break; 291 case REVOKED: 292 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.CANCELLED); 293 break; 294 default: 295 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.NULL); 296 break; 297 } 298 } 299 return tgt; 300 } 301}