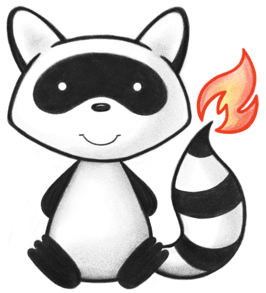
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 008import org.hl7.fhir.dstu2.model.Communication; 009import org.hl7.fhir.dstu2.model.Enumeration; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Communication10_40 { 013 014 public static org.hl7.fhir.r4.model.Communication convertCommunication(org.hl7.fhir.dstu2.model.Communication src) throws FHIRException { 015 if (src == null || src.isEmpty()) 016 return null; 017 org.hl7.fhir.r4.model.Communication tgt = new org.hl7.fhir.r4.model.Communication(); 018 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 021 if (src.hasCategory()) 022 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(src.getCategory())); 023 if (src.hasSender()) 024 tgt.setSender(Reference10_40.convertReference(src.getSender())); 025 for (org.hl7.fhir.dstu2.model.Reference t : src.getRecipient()) 026 tgt.addRecipient(Reference10_40.convertReference(t)); 027 for (org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 028 tgt.addPayload(convertCommunicationPayloadComponent(t)); 029 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getMedium()) 030 tgt.addMedium(CodeableConcept10_40.convertCodeableConcept(t)); 031 if (src.hasStatus()) 032 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 033 if (src.hasEncounter()) 034 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 035 if (src.hasSentElement()) 036 tgt.setSentElement(DateTime10_40.convertDateTime(src.getSentElement())); 037 if (src.hasReceivedElement()) 038 tgt.setReceivedElement(DateTime10_40.convertDateTime(src.getReceivedElement())); 039 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReason()) 040 tgt.addReasonCode(CodeableConcept10_40.convertCodeableConcept(t)); 041 if (src.hasSubject()) 042 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.dstu2.model.Communication convertCommunication(org.hl7.fhir.r4.model.Communication src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.dstu2.model.Communication tgt = new org.hl7.fhir.dstu2.model.Communication(); 050 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 053 if (src.hasCategory()) 054 tgt.setCategory(CodeableConcept10_40.convertCodeableConcept(src.getCategoryFirstRep())); 055 if (src.hasSender()) 056 tgt.setSender(Reference10_40.convertReference(src.getSender())); 057 for (org.hl7.fhir.r4.model.Reference t : src.getRecipient()) tgt.addRecipient(Reference10_40.convertReference(t)); 058 for (org.hl7.fhir.r4.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 059 tgt.addPayload(convertCommunicationPayloadComponent(t)); 060 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getMedium()) 061 tgt.addMedium(CodeableConcept10_40.convertCodeableConcept(t)); 062 if (src.hasStatus()) 063 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 064 if (src.hasEncounter()) 065 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 066 if (src.hasSentElement()) 067 tgt.setSentElement(DateTime10_40.convertDateTime(src.getSentElement())); 068 if (src.hasReceivedElement()) 069 tgt.setReceivedElement(DateTime10_40.convertDateTime(src.getReceivedElement())); 070 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 071 tgt.addReason(CodeableConcept10_40.convertCodeableConcept(t)); 072 if (src.hasSubject()) 073 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 074 return tgt; 075 } 076 077 public static org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.r4.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 078 if (src == null || src.isEmpty()) 079 return null; 080 org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent(); 081 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 082 if (src.hasContent()) 083 tgt.setContent(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getContent())); 084 return tgt; 085 } 086 087 public static org.hl7.fhir.r4.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 088 if (src == null || src.isEmpty()) 089 return null; 090 org.hl7.fhir.r4.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.r4.model.Communication.CommunicationPayloadComponent(); 091 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 092 if (src.hasContent()) 093 tgt.setContent(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getContent())); 094 return tgt; 095 } 096 097 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Communication.CommunicationStatus> convertCommunicationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Communication.CommunicationStatus> src) throws FHIRException { 098 if (src == null || src.isEmpty()) 099 return null; 100 Enumeration<Communication.CommunicationStatus> tgt = new Enumeration<>(new Communication.CommunicationStatusEnumFactory()); 101 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 102 if (src.getValue() == null) { 103 tgt.setValue(null); 104 } else { 105 switch (src.getValue()) { 106 case INPROGRESS: 107 tgt.setValue(Communication.CommunicationStatus.INPROGRESS); 108 break; 109 case COMPLETED: 110 tgt.setValue(Communication.CommunicationStatus.COMPLETED); 111 break; 112 case ONHOLD: 113 tgt.setValue(Communication.CommunicationStatus.SUSPENDED); 114 break; 115 case ENTEREDINERROR: 116 tgt.setValue(Communication.CommunicationStatus.REJECTED); 117 break; 118 case NOTDONE: 119 tgt.setValue(Communication.CommunicationStatus.FAILED); 120 break; 121 default: 122 tgt.setValue(Communication.CommunicationStatus.NULL); 123 break; 124 } 125 } 126 return tgt; 127 } 128 129 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Communication.CommunicationStatus> convertCommunicationStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Communication.CommunicationStatus> src) throws FHIRException { 130 if (src == null || src.isEmpty()) 131 return null; 132 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Communication.CommunicationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Communication.CommunicationStatusEnumFactory()); 133 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 134 if (src.getValue() == null) { 135 tgt.setValue(null); 136 } else { 137 switch (src.getValue()) { 138 case INPROGRESS: 139 tgt.setValue(org.hl7.fhir.r4.model.Communication.CommunicationStatus.INPROGRESS); 140 break; 141 case COMPLETED: 142 tgt.setValue(org.hl7.fhir.r4.model.Communication.CommunicationStatus.COMPLETED); 143 break; 144 case SUSPENDED: 145 tgt.setValue(org.hl7.fhir.r4.model.Communication.CommunicationStatus.ONHOLD); 146 break; 147 case REJECTED: 148 tgt.setValue(org.hl7.fhir.r4.model.Communication.CommunicationStatus.ENTEREDINERROR); 149 break; 150 case FAILED: 151 tgt.setValue(org.hl7.fhir.r4.model.Communication.CommunicationStatus.NOTDONE); 152 break; 153 default: 154 tgt.setValue(org.hl7.fhir.r4.model.Communication.CommunicationStatus.NULL); 155 break; 156 } 157 } 158 return tgt; 159 } 160}