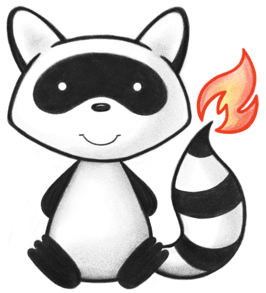
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 007import org.hl7.fhir.exceptions.FHIRException; 008 009public class Condition10_40 { 010 011 public static org.hl7.fhir.dstu2.model.Condition convertCondition(org.hl7.fhir.r4.model.Condition src) throws FHIRException { 012 if (src == null || src.isEmpty()) 013 return null; 014 org.hl7.fhir.dstu2.model.Condition tgt = new org.hl7.fhir.dstu2.model.Condition(); 015 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 016 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 017 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 018 if (src.hasSubject()) 019 tgt.setPatient(Reference10_40.convertReference(src.getSubject())); 020 if (src.hasEncounter()) 021 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 022 if (src.hasAsserter()) 023 tgt.setAsserter(Reference10_40.convertReference(src.getAsserter())); 024 if (src.hasRecordedDate()) 025 tgt.setDateRecorded(src.getRecordedDate()); 026 if (src.hasCode()) 027 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 028 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 029 tgt.setCategory(CodeableConcept10_40.convertCodeableConcept(t)); 030 if (src.hasClinicalStatus()) 031 tgt.setClinicalStatus(convertConditionClinicalStatus(src.getClinicalStatus())); 032 if (src.hasVerificationStatus()) 033 tgt.setVerificationStatus(convertConditionVerificationStatus(src.getVerificationStatus())); 034 if (src.hasSeverity()) 035 tgt.setSeverity(CodeableConcept10_40.convertCodeableConcept(src.getSeverity())); 036 if (src.hasOnset()) 037 tgt.setOnset(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getOnset())); 038 if (src.hasAbatement() && !(src.getAbatement() instanceof org.hl7.fhir.r4.model.BooleanType)) 039 tgt.setAbatement(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getAbatement())); 040 if (src.hasStage()) 041 tgt.setStage(convertConditionStageComponent(src.getStageFirstRep())); 042 for (org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent t : src.getEvidence()) 043 tgt.addEvidence(convertConditionEvidenceComponent(t)); 044 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getBodySite()) 045 tgt.addBodySite(CodeableConcept10_40.convertCodeableConcept(t)); 046 return tgt; 047 } 048 049 public static org.hl7.fhir.r4.model.Condition convertCondition(org.hl7.fhir.dstu2.model.Condition src) throws FHIRException { 050 if (src == null || src.isEmpty()) 051 return null; 052 org.hl7.fhir.r4.model.Condition tgt = new org.hl7.fhir.r4.model.Condition(); 053 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 056 if (src.hasPatient()) 057 tgt.setSubject(Reference10_40.convertReference(src.getPatient())); 058 if (src.hasEncounter()) 059 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 060 if (src.hasAsserter()) 061 tgt.setAsserter(Reference10_40.convertReference(src.getAsserter())); 062 if (src.hasDateRecorded()) 063 tgt.setRecordedDate(src.getDateRecorded()); 064 if (src.hasCode()) 065 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 066 if (src.hasCategory()) 067 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(src.getCategory())); 068 if (src.hasClinicalStatus()) 069 tgt.setClinicalStatus(convertConditionClinicalStatus(src.getClinicalStatus())); 070 if (src.hasVerificationStatus()) 071 tgt.setVerificationStatus(convertConditionVerificationStatus(src.getVerificationStatus())); 072 if (src.hasSeverity()) 073 tgt.setSeverity(CodeableConcept10_40.convertCodeableConcept(src.getSeverity())); 074 if (src.hasOnset()) 075 tgt.setOnset(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getOnset())); 076 if (src.hasAbatement() && !(src.getAbatement() instanceof org.hl7.fhir.dstu2.model.BooleanType)) 077 tgt.setAbatement(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getAbatement())); 078 if (src.hasStage()) 079 tgt.addStage(convertConditionStageComponent(src.getStage())); 080 for (org.hl7.fhir.dstu2.model.Condition.ConditionEvidenceComponent t : src.getEvidence()) 081 tgt.addEvidence(convertConditionEvidenceComponent(t)); 082 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getBodySite()) 083 tgt.addBodySite(CodeableConcept10_40.convertCodeableConcept(t)); 084 return tgt; 085 } 086 087 static public org.hl7.fhir.r4.model.CodeableConcept convertConditionClinicalStatus(String src) throws FHIRException { 088 if (src == null) 089 return null; 090 org.hl7.fhir.r4.model.CodeableConcept cc = new org.hl7.fhir.r4.model.CodeableConcept(); 091 cc.addCoding().setSystem("http://hl7.org/fhir/condition-clinical").setCode(src); 092 return cc; 093 } 094 095 static public String convertConditionClinicalStatus(org.hl7.fhir.r4.model.CodeableConcept src) throws FHIRException { 096 if (src == null) 097 return null; 098 for (org.hl7.fhir.r4.model.Coding c : src.getCoding()) { 099 if ("http://hl7.org/fhir/condition-clinical".equals(c.getSystem())) 100 return c.getCode(); 101 } 102 return null; 103 } 104 105 public static org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent convertConditionEvidenceComponent(org.hl7.fhir.dstu2.model.Condition.ConditionEvidenceComponent src) throws FHIRException { 106 if (src == null || src.isEmpty()) 107 return null; 108 org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent tgt = new org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent(); 109 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 110 if (src.hasCode()) 111 tgt.addCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 112 for (org.hl7.fhir.dstu2.model.Reference t : src.getDetail()) tgt.addDetail(Reference10_40.convertReference(t)); 113 return tgt; 114 } 115 116 public static org.hl7.fhir.dstu2.model.Condition.ConditionEvidenceComponent convertConditionEvidenceComponent(org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 org.hl7.fhir.dstu2.model.Condition.ConditionEvidenceComponent tgt = new org.hl7.fhir.dstu2.model.Condition.ConditionEvidenceComponent(); 120 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 121 for (org.hl7.fhir.r4.model.CodeableConcept cc : src.getCode()) 122 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(cc)); 123 for (org.hl7.fhir.r4.model.Reference t : src.getDetail()) tgt.addDetail(Reference10_40.convertReference(t)); 124 return tgt; 125 } 126 127 public static org.hl7.fhir.dstu2.model.Condition.ConditionStageComponent convertConditionStageComponent(org.hl7.fhir.r4.model.Condition.ConditionStageComponent src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.dstu2.model.Condition.ConditionStageComponent tgt = new org.hl7.fhir.dstu2.model.Condition.ConditionStageComponent(); 131 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 132 if (src.hasSummary()) 133 tgt.setSummary(CodeableConcept10_40.convertCodeableConcept(src.getSummary())); 134 for (org.hl7.fhir.r4.model.Reference t : src.getAssessment()) tgt.addAssessment(Reference10_40.convertReference(t)); 135 return tgt; 136 } 137 138 public static org.hl7.fhir.r4.model.Condition.ConditionStageComponent convertConditionStageComponent(org.hl7.fhir.dstu2.model.Condition.ConditionStageComponent src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.r4.model.Condition.ConditionStageComponent tgt = new org.hl7.fhir.r4.model.Condition.ConditionStageComponent(); 142 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 143 if (src.hasSummary()) 144 tgt.setSummary(CodeableConcept10_40.convertCodeableConcept(src.getSummary())); 145 for (org.hl7.fhir.dstu2.model.Reference t : src.getAssessment()) 146 tgt.addAssessment(Reference10_40.convertReference(t)); 147 return tgt; 148 } 149 150 static public org.hl7.fhir.r4.model.CodeableConcept convertConditionVerificationStatus(org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus src) throws FHIRException { 151 if (src == null) 152 return null; 153 org.hl7.fhir.r4.model.CodeableConcept cc = new org.hl7.fhir.r4.model.CodeableConcept(); 154 switch (src) { 155 case PROVISIONAL: 156 cc.addCoding().setSystem("http://hl7.org/fhir/condition-ver-status").setCode("provisional"); 157 return cc; 158 case DIFFERENTIAL: 159 cc.addCoding().setSystem("http://hl7.org/fhir/condition-ver-status").setCode("differential"); 160 return cc; 161 case CONFIRMED: 162 cc.addCoding().setSystem("http://hl7.org/fhir/condition-ver-status").setCode("confirmed"); 163 return cc; 164 case REFUTED: 165 cc.addCoding().setSystem("http://hl7.org/fhir/condition-ver-status").setCode("refuted"); 166 return cc; 167 case ENTEREDINERROR: 168 cc.addCoding().setSystem("http://hl7.org/fhir/condition-ver-status").setCode("entered-in-error"); 169 return cc; 170 default: 171 return null; 172 } 173 } 174 175 static public org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus convertConditionVerificationStatus(org.hl7.fhir.r4.model.CodeableConcept src) throws FHIRException { 176 if (src == null) 177 return null; 178 if (src.hasCoding("http://hl7.org/fhir/condition-clinical", "provisional")) 179 return org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus.PROVISIONAL; 180 if (src.hasCoding("http://hl7.org/fhir/condition-clinical", "differential")) 181 return org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus.DIFFERENTIAL; 182 if (src.hasCoding("http://hl7.org/fhir/condition-clinical", "confirmed")) 183 return org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus.CONFIRMED; 184 if (src.hasCoding("http://hl7.org/fhir/condition-clinical", "refuted")) 185 return org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus.REFUTED; 186 if (src.hasCoding("http://hl7.org/fhir/condition-clinical", "entered-in-error")) 187 return org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus.ENTEREDINERROR; 188 return org.hl7.fhir.dstu2.model.Condition.ConditionVerificationStatus.NULL; 189 } 190}