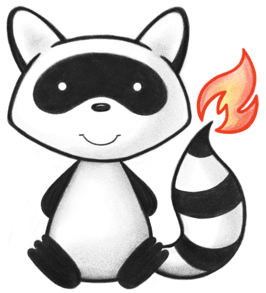
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_40; 005import org.hl7.fhir.convertors.context.ConversionContext10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Canonical10_40; 012import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Code10_40; 013import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 014import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 015import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.UnsignedInt10_40; 016import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 017import org.hl7.fhir.dstu2.model.Conformance; 018import org.hl7.fhir.dstu2.model.Enumeration; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r4.model.CapabilityStatement; 021import org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent; 022import org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent; 023import org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction; 024 025public class Conformance10_40 { 026 027 private static final String[] IGNORED_EXTENSION_URLS = new String[]{ 028 VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL 029 }; 030 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus> src) throws FHIRException { 031 if (src == null || src.isEmpty()) 032 return null; 033 Enumeration<Conformance.ConditionalDeleteStatus> tgt = new Enumeration<>(new Conformance.ConditionalDeleteStatusEnumFactory()); 034 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 035 if (src.getValue() == null) { 036 tgt.setValue(null); 037 } else { 038 switch (src.getValue()) { 039 case NOTSUPPORTED: 040 tgt.setValue(Conformance.ConditionalDeleteStatus.NOTSUPPORTED); 041 break; 042 case SINGLE: 043 tgt.setValue(Conformance.ConditionalDeleteStatus.SINGLE); 044 break; 045 case MULTIPLE: 046 tgt.setValue(Conformance.ConditionalDeleteStatus.MULTIPLE); 047 break; 048 default: 049 tgt.setValue(Conformance.ConditionalDeleteStatus.NULL); 050 break; 051 } 052 } 053 return tgt; 054 } 055 056 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConditionalDeleteStatus> src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 org.hl7.fhir.r4.model.Enumeration<CapabilityStatement.ConditionalDeleteStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new CapabilityStatement.ConditionalDeleteStatusEnumFactory()); 060 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 061 if (src.getValue() == null) { 062 tgt.setValue(null); 063 } else { 064 switch (src.getValue()) { 065 case NOTSUPPORTED: 066 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.NOTSUPPORTED); 067 break; 068 case SINGLE: 069 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.SINGLE); 070 break; 071 case MULTIPLE: 072 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.MULTIPLE); 073 break; 074 default: 075 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.NULL); 076 break; 077 } 078 } 079 return tgt; 080 } 081 082 public static org.hl7.fhir.dstu2.model.Conformance convertConformance(org.hl7.fhir.r4.model.CapabilityStatement src, BaseAdvisor_10_40 advisor) throws FHIRException { 083 if (src == null || src.isEmpty()) 084 return null; 085 org.hl7.fhir.dstu2.model.Conformance tgt = new org.hl7.fhir.dstu2.model.Conformance(); 086 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt, IGNORED_EXTENSION_URLS); 087 if (src.hasUrlElement()) 088 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 089 if (src.hasVersionElement()) 090 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 091 if (src.hasNameElement()) 092 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 093 if (src.hasStatus()) 094 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 095 if (src.hasExperimental()) 096 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 097 if (src.hasDate()) 098 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 099 if (src.hasPublisherElement()) 100 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 101 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 102 tgt.addContact(convertConformanceContactComponent(t)); 103 if (src.hasDescription()) 104 tgt.setDescription(src.getDescription()); 105 if (src.hasPurpose()) 106 tgt.setRequirements(src.getPurpose()); 107 if (src.hasCopyright()) 108 tgt.setCopyright(src.getCopyright()); 109 if (src.hasKind()) 110 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 111 if (src.hasSoftware()) 112 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 113 if (src.hasImplementation()) 114 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 115 if (src.hasFhirVersion()) 116 tgt.setFhirVersion(src.getFhirVersion().toCode()); 117 if (src.hasExtension(VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL)) 118 tgt.setAcceptUnknown(org.hl7.fhir.dstu2.model.Conformance.UnknownContentCode.fromCode(src.getExtensionByUrl(VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL).getValue().primitiveValue())); 119 for (org.hl7.fhir.r4.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 120 for (CapabilityStatementRestComponent r : src.getRest()) 121 for (CapabilityStatementRestResourceComponent rr : r.getResource()) 122 for (org.hl7.fhir.r4.model.CanonicalType t : rr.getSupportedProfile()) 123 tgt.addProfile(Canonical10_40.convertCanonicalToReference(t)); 124 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent t : src.getRest()) 125 tgt.addRest(convertConformanceRestComponent(t)); 126 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent t : src.getMessaging()) 127 tgt.addMessaging(convertConformanceMessagingComponent(t)); 128 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent t : src.getDocument()) 129 tgt.addDocument(convertConformanceDocumentComponent(t)); 130 return tgt; 131 } 132 133 public static org.hl7.fhir.r4.model.CapabilityStatement convertConformance(org.hl7.fhir.dstu2.model.Conformance src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 org.hl7.fhir.r4.model.CapabilityStatement tgt = new org.hl7.fhir.r4.model.CapabilityStatement(); 137 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 138 if (src.hasUrlElement()) 139 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 140 if (src.hasVersionElement()) 141 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 142 if (src.hasNameElement()) 143 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 144 if (src.hasStatus()) 145 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 146 if (src.hasExperimental()) 147 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 148 if (src.hasDate()) 149 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 150 if (src.hasPublisherElement()) 151 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 152 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent t : src.getContact()) 153 tgt.addContact(convertConformanceContactComponent(t)); 154 if (src.hasDescription()) 155 tgt.setDescription(src.getDescription()); 156 if (src.hasRequirements()) 157 tgt.setPurpose(src.getRequirements()); 158 if (src.hasCopyright()) 159 tgt.setCopyright(src.getCopyright()); 160 if (src.hasKind()) 161 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 162 if (src.hasSoftware()) 163 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 164 if (src.hasImplementation()) 165 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 166 if (src.hasFhirVersion()) 167 tgt.setFhirVersion(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 168 if (src.hasAcceptUnknown()) 169 tgt.addExtension().setUrl(VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL).setValue(new org.hl7.fhir.r4.model.CodeType(src.getAcceptUnknownElement().asStringValue())); 170 for (org.hl7.fhir.dstu2.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 171 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent t : src.getRest()) 172 tgt.addRest(convertConformanceRestComponent(t)); 173 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent t : src.getMessaging()) 174 tgt.addMessaging(convertConformanceMessagingComponent(t)); 175 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent t : src.getDocument()) 176 tgt.addDocument(convertConformanceDocumentComponent(t)); 177 return tgt; 178 } 179 180 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent convertConformanceContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 181 if (src == null || src.isEmpty()) 182 return null; 183 org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent(); 184 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 185 if (src.hasNameElement()) 186 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 187 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 188 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 189 return tgt; 190 } 191 192 public static org.hl7.fhir.r4.model.ContactDetail convertConformanceContactComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent src) throws FHIRException { 193 if (src == null || src.isEmpty()) 194 return null; 195 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 196 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 197 if (src.hasNameElement()) 198 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 199 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 200 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent src) throws FHIRException { 205 if (src == null || src.isEmpty()) 206 return null; 207 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent(); 208 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 209 if (src.hasMode()) 210 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 211 if (src.hasDocumentation()) 212 tgt.setDocumentation(src.getDocumentation()); 213 if (src.hasProfile()) 214 tgt.setProfileElement(Canonical10_40.convertReferenceToCanonical(src.getProfile())); 215 return tgt; 216 } 217 218 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementDocumentComponent src) throws FHIRException { 219 if (src == null || src.isEmpty()) 220 return null; 221 org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent(); 222 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 223 if (src.hasMode()) 224 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 225 if (src.hasDocumentation()) 226 tgt.setDocumentation(src.getDocumentation()); 227 if (src.hasProfileElement()) 228 tgt.setProfile(Canonical10_40.convertCanonicalToReference(src.getProfileElement())); 229 return tgt; 230 } 231 232 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent src) throws FHIRException { 233 if (src == null || src.isEmpty()) 234 return null; 235 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent(); 236 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 237 if (src.hasDescriptionElement()) 238 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 239 if (src.hasUrl()) 240 tgt.setUrl(src.getUrl()); 241 return tgt; 242 } 243 244 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementImplementationComponent src) throws FHIRException { 245 if (src == null || src.isEmpty()) 246 return null; 247 org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent(); 248 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 249 if (src.hasDescriptionElement()) 250 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 251 if (src.hasUrl()) 252 tgt.setUrl(src.getUrl()); 253 return tgt; 254 } 255 256 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent src) throws FHIRException { 257 if (src == null || src.isEmpty()) 258 return null; 259 org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent(); 260 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 261 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent t : src.getEndpoint()) 262 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 263 if (src.hasReliableCacheElement()) 264 tgt.setReliableCacheElement(UnsignedInt10_40.convertUnsignedInt(src.getReliableCacheElement())); 265 if (src.hasDocumentation()) 266 tgt.setDocumentation(src.getDocumentation()); 267 for (org.hl7.fhir.r4.model.Extension e : src.getExtensionsByUrl(VersionConvertorConstants.EXT_IG_CONFORMANCE_MESSAGE_EVENT)) { 268 org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEventComponent event = new org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEventComponent(); 269 tgt.addEvent(event); 270 event.setCode(Coding10_40.convertCoding((org.hl7.fhir.r4.model.Coding) e.getExtensionByUrl("code").getValue())); 271 if (e.hasExtension("category")) 272 event.setCategory(org.hl7.fhir.dstu2.model.Conformance.MessageSignificanceCategory.fromCode(e.getExtensionByUrl("category").getValue().toString())); 273 event.setMode(org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode.fromCode(e.getExtensionByUrl("mode").getValue().toString())); 274 org.hl7.fhir.r4.model.Extension focusE = e.getExtensionByUrl("focus"); 275 if (focusE.getValue().hasPrimitiveValue()) 276 event.setFocus(focusE.getValue().toString()); 277 else { 278 event.setFocusElement(new org.hl7.fhir.dstu2.model.CodeType()); 279 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(focusE.getValue(), event.getFocusElement()); 280 } 281 event.setRequest(Reference10_40.convertReference((org.hl7.fhir.r4.model.Reference) e.getExtensionByUrl("request").getValue())); 282 event.setResponse(Reference10_40.convertReference((org.hl7.fhir.r4.model.Reference) e.getExtensionByUrl("response").getValue())); 283 if (e.hasExtension("documentation")) 284 event.setDocumentation(e.getExtensionByUrl("documentation").getValue().toString()); 285 } 286 return tgt; 287 } 288 289 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent src) throws FHIRException { 290 if (src == null || src.isEmpty()) 291 return null; 292 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingComponent(); 293 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 294 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent t : src.getEndpoint()) 295 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 296 if (src.hasReliableCacheElement()) 297 tgt.setReliableCacheElement(UnsignedInt10_40.convertUnsignedInt(src.getReliableCacheElement())); 298 if (src.hasDocumentation()) 299 tgt.setDocumentation(src.getDocumentation()); 300 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEventComponent t : src.getEvent()) { 301 org.hl7.fhir.r4.model.Extension e = new org.hl7.fhir.r4.model.Extension(VersionConvertorConstants.EXT_IG_CONFORMANCE_MESSAGE_EVENT); 302 e.addExtension(new org.hl7.fhir.r4.model.Extension("code", Coding10_40.convertCoding(t.getCode()))); 303 if (t.hasCategory()) 304 e.addExtension(new org.hl7.fhir.r4.model.Extension("category", new org.hl7.fhir.r4.model.CodeType(t.getCategory().toCode()))); 305 e.addExtension(new org.hl7.fhir.r4.model.Extension("mode", new org.hl7.fhir.r4.model.CodeType(t.getMode().toCode()))); 306 if (t.getFocusElement().hasValue()) 307 e.addExtension(new org.hl7.fhir.r4.model.Extension("focus", new org.hl7.fhir.r4.model.StringType(t.getFocus()))); 308 else { 309 org.hl7.fhir.r4.model.CodeType focus = new org.hl7.fhir.r4.model.CodeType(); 310 org.hl7.fhir.r4.model.Extension focusE = new org.hl7.fhir.r4.model.Extension("focus", focus); 311 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(t.getFocusElement(), focus); 312 e.addExtension(focusE); 313 } 314 e.addExtension(new org.hl7.fhir.r4.model.Extension("request", Reference10_40.convertReference(t.getRequest()))); 315 e.addExtension(new org.hl7.fhir.r4.model.Extension("response", Reference10_40.convertReference(t.getResponse()))); 316 if (t.hasDocumentation()) 317 e.addExtension(new org.hl7.fhir.r4.model.Extension("documentation", new org.hl7.fhir.r4.model.StringType(t.getDocumentation()))); 318 tgt.addExtension(e); 319 } 320 return tgt; 321 } 322 323 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent src) throws FHIRException { 324 if (src == null || src.isEmpty()) 325 return null; 326 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent(); 327 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 328 if (src.hasProtocol()) 329 tgt.setProtocol(Coding10_40.convertCoding(src.getProtocol())); 330 if (src.hasAddress()) 331 tgt.setAddress(src.getAddress()); 332 return tgt; 333 } 334 335 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent src) throws FHIRException { 336 if (src == null || src.isEmpty()) 337 return null; 338 org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent(); 339 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 340 if (src.hasProtocol()) 341 tgt.setProtocol(Coding10_40.convertCoding(src.getProtocol())); 342 if (src.hasAddress()) 343 tgt.setAddress(src.getAddress()); 344 return tgt; 345 } 346 347 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent convertConformanceRestComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent src) throws FHIRException { 348 if (src == null || src.isEmpty()) 349 return null; 350 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent(); 351 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 352 if (src.hasMode()) 353 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 354 if (src.hasDocumentation()) 355 tgt.setDocumentation(src.getDocumentation()); 356 if (src.hasSecurity()) 357 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 358 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent t : src.getResource()) 359 tgt.addResource(convertConformanceRestResourceComponent(t)); 360 for (org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent t : src.getInteraction()) 361 tgt.addInteraction(convertSystemInteractionComponent(t)); 362 if (src.getTransactionMode() == org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BATCH || src.getTransactionMode() == org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BOTH) 363 tgt.addInteraction().setCode(SystemRestfulInteraction.BATCH); 364 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 365 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 366 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent t : src.getOperation()) 367 tgt.addOperation(convertConformanceRestOperationComponent(t)); 368 for (org.hl7.fhir.dstu2.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 369 return tgt; 370 } 371 372 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent convertConformanceRestComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestComponent src) throws FHIRException { 373 if (src == null || src.isEmpty()) 374 return null; 375 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent(); 376 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 377 if (src.hasMode()) 378 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 379 if (src.hasDocumentation()) 380 tgt.setDocumentation(src.getDocumentation()); 381 if (src.hasSecurity()) 382 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 383 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent t : src.getResource()) 384 tgt.addResource(convertConformanceRestResourceComponent(t)); 385 boolean batch = false; 386 boolean transaction = false; 387 for (org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent t : src.getInteraction()) { 388 if (t.getCode().equals(SystemRestfulInteraction.BATCH)) 389 batch = true; 390 else 391 tgt.addInteraction(convertSystemInteractionComponent(t)); 392 if (t.getCode().equals(SystemRestfulInteraction.TRANSACTION)) 393 transaction = true; 394 } 395 if (batch) 396 tgt.setTransactionMode(transaction ? org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BOTH : org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BATCH); 397 else 398 tgt.setTransactionMode(transaction ? org.hl7.fhir.dstu2.model.Conformance.TransactionMode.TRANSACTION : org.hl7.fhir.dstu2.model.Conformance.TransactionMode.NOTSUPPORTED); 399 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 400 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 401 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent t : src.getOperation()) 402 tgt.addOperation(convertConformanceRestOperationComponent(t)); 403 for (org.hl7.fhir.r4.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 404 return tgt; 405 } 406 407 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent src) throws FHIRException { 408 if (src == null || src.isEmpty()) 409 return null; 410 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent(); 411 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 412 if (src.hasNameElement()) 413 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 414 if (src.hasDefinition()) 415 tgt.setDefinitionElement(Canonical10_40.convertReferenceToCanonical(src.getDefinition())); 416 return tgt; 417 } 418 419 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent src) throws FHIRException { 420 if (src == null || src.isEmpty()) 421 return null; 422 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent(); 423 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 424 if (src.hasNameElement()) 425 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 426 if (src.hasDefinitionElement()) 427 tgt.setDefinition(Canonical10_40.convertCanonicalToReference(src.getDefinitionElement())); 428 return tgt; 429 } 430 431 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent src) throws FHIRException { 432 if (src == null || src.isEmpty()) 433 return null; 434 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent(); 435 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 436 if (src.hasTypeElement()) 437 tgt.setTypeElement(Code10_40.convertCode(src.getTypeElement())); 438 if (src.hasProfile()) 439 tgt.setProfileElement(Canonical10_40.convertReferenceToCanonical(src.getProfile())); 440 for (org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent t : src.getInteraction()) 441 tgt.addInteraction(convertResourceInteractionComponent(t)); 442 if (src.hasVersioning()) 443 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 444 if (src.hasReadHistoryElement()) 445 tgt.setReadHistoryElement(Boolean10_40.convertBoolean(src.getReadHistoryElement())); 446 if (src.hasUpdateCreateElement()) 447 tgt.setUpdateCreateElement(Boolean10_40.convertBoolean(src.getUpdateCreateElement())); 448 if (src.hasConditionalCreateElement()) 449 tgt.setConditionalCreateElement(Boolean10_40.convertBoolean(src.getConditionalCreateElement())); 450 if (src.hasConditionalUpdateElement()) 451 tgt.setConditionalUpdateElement(Boolean10_40.convertBoolean(src.getConditionalUpdateElement())); 452 if (src.hasConditionalDelete()) 453 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 454 for (org.hl7.fhir.dstu2.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 455 for (org.hl7.fhir.dstu2.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 456 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 457 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 458 return tgt; 459 } 460 461 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceComponent src) throws FHIRException { 462 if (src == null || src.isEmpty()) 463 return null; 464 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent(); 465 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 466 if (src.hasTypeElement()) 467 tgt.setTypeElement(Code10_40.convertCode(src.getTypeElement())); 468 if (src.hasProfile()) 469 tgt.setProfile(Canonical10_40.convertCanonicalToReference(src.getProfileElement())); 470 for (org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent t : src.getInteraction()) 471 tgt.addInteraction(convertResourceInteractionComponent(t)); 472 if (src.hasVersioning()) 473 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 474 if (src.hasReadHistoryElement()) 475 tgt.setReadHistoryElement(Boolean10_40.convertBoolean(src.getReadHistoryElement())); 476 if (src.hasUpdateCreateElement()) 477 tgt.setUpdateCreateElement(Boolean10_40.convertBoolean(src.getUpdateCreateElement())); 478 if (src.hasConditionalCreateElement()) 479 tgt.setConditionalCreateElement(Boolean10_40.convertBoolean(src.getConditionalCreateElement())); 480 if (src.hasConditionalUpdateElement()) 481 tgt.setConditionalUpdateElement(Boolean10_40.convertBoolean(src.getConditionalUpdateElement())); 482 if (src.hasConditionalDelete()) 483 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 484 for (org.hl7.fhir.r4.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 485 for (org.hl7.fhir.r4.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 486 for (org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 487 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 488 return tgt; 489 } 490 491 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent src) throws FHIRException { 492 if (src == null || src.isEmpty()) 493 return null; 494 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent(); 495 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 496 if (src.hasNameElement()) 497 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 498 if (src.hasDefinition()) 499 tgt.setDefinition(src.getDefinition()); 500 if (src.hasType()) 501 tgt.setTypeElement(Enumerations10_40.convertSearchParamType(src.getTypeElement())); 502 if (src.hasDocumentation()) 503 tgt.setDocumentation(src.getDocumentation()); 504 return tgt; 505 } 506 507 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent src) throws FHIRException { 508 if (src == null || src.isEmpty()) 509 return null; 510 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent(); 511 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 512 if (src.hasNameElement()) 513 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 514 if (src.hasDefinition()) 515 tgt.setDefinition(src.getDefinition()); 516 if (src.hasType()) 517 tgt.setTypeElement(Enumerations10_40.convertSearchParamType(src.getTypeElement())); 518 if (src.hasDocumentation()) 519 tgt.setDocumentation(src.getDocumentation()); 520 return tgt; 521 } 522 523 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent src) throws FHIRException { 524 if (src == null || src.isEmpty()) 525 return null; 526 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent(); 527 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 528 if (src.hasCorsElement()) 529 tgt.setCorsElement(Boolean10_40.convertBoolean(src.getCorsElement())); 530 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getService()) 531 tgt.addService(CodeableConcept10_40.convertCodeableConcept(t)); 532 if (src.hasDescription()) 533 tgt.setDescription(src.getDescription()); 534 return tgt; 535 } 536 537 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent src) throws FHIRException { 538 if (src == null || src.isEmpty()) 539 return null; 540 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementRestSecurityComponent(); 541 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 542 if (src.hasCorsElement()) 543 tgt.setCorsElement(Boolean10_40.convertBoolean(src.getCorsElement())); 544 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getService()) 545 tgt.addService(CodeableConcept10_40.convertCodeableConcept(t)); 546 if (src.hasDescription()) 547 tgt.setDescription(src.getDescription()); 548 return tgt; 549 } 550 551 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent src) throws FHIRException { 552 if (src == null || src.isEmpty()) 553 return null; 554 org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent(); 555 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 556 if (src.hasNameElement()) 557 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 558 if (src.hasVersionElement()) 559 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 560 if (src.hasReleaseDateElement()) 561 tgt.setReleaseDateElement(DateTime10_40.convertDateTime(src.getReleaseDateElement())); 562 return tgt; 563 } 564 565 public static org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent src) throws FHIRException { 566 if (src == null || src.isEmpty()) 567 return null; 568 org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementSoftwareComponent(); 569 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 570 if (src.hasNameElement()) 571 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 572 if (src.hasVersionElement()) 573 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 574 if (src.hasReleaseDateElement()) 575 tgt.setReleaseDateElement(DateTime10_40.convertDateTime(src.getReleaseDateElement())); 576 return tgt; 577 } 578 579 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceStatementKind> convertConformanceStatementKind(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind> src) throws FHIRException { 580 if (src == null || src.isEmpty()) 581 return null; 582 Enumeration<Conformance.ConformanceStatementKind> tgt = new Enumeration<>(new Conformance.ConformanceStatementKindEnumFactory()); 583 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 584 if (src.getValue() == null) { 585 tgt.setValue(null); 586 } else { 587 switch (src.getValue()) { 588 case INSTANCE: 589 tgt.setValue(Conformance.ConformanceStatementKind.INSTANCE); 590 break; 591 case CAPABILITY: 592 tgt.setValue(Conformance.ConformanceStatementKind.CAPABILITY); 593 break; 594 case REQUIREMENTS: 595 tgt.setValue(Conformance.ConformanceStatementKind.REQUIREMENTS); 596 break; 597 default: 598 tgt.setValue(Conformance.ConformanceStatementKind.NULL); 599 break; 600 } 601 } 602 return tgt; 603 } 604 605 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.CapabilityStatementKind> convertConformanceStatementKind(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceStatementKind> src) throws FHIRException { 606 if (src == null || src.isEmpty()) 607 return null; 608 org.hl7.fhir.r4.model.Enumeration<CapabilityStatement.CapabilityStatementKind> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new CapabilityStatement.CapabilityStatementKindEnumFactory()); 609 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 610 if (src.getValue() == null) { 611 tgt.setValue(null); 612 } else { 613 switch (src.getValue()) { 614 case INSTANCE: 615 tgt.setValue(CapabilityStatement.CapabilityStatementKind.INSTANCE); 616 break; 617 case CAPABILITY: 618 tgt.setValue(CapabilityStatement.CapabilityStatementKind.CAPABILITY); 619 break; 620 case REQUIREMENTS: 621 tgt.setValue(CapabilityStatement.CapabilityStatementKind.REQUIREMENTS); 622 break; 623 default: 624 tgt.setValue(CapabilityStatement.CapabilityStatementKind.NULL); 625 break; 626 } 627 } 628 return tgt; 629 } 630 631 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.DocumentMode> convertDocumentMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode> src) throws FHIRException { 632 if (src == null || src.isEmpty()) 633 return null; 634 Enumeration<Conformance.DocumentMode> tgt = new Enumeration<>(new Conformance.DocumentModeEnumFactory()); 635 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 636 if (src.getValue() == null) { 637 tgt.setValue(null); 638 } else { 639 switch (src.getValue()) { 640 case PRODUCER: 641 tgt.setValue(Conformance.DocumentMode.PRODUCER); 642 break; 643 case CONSUMER: 644 tgt.setValue(Conformance.DocumentMode.CONSUMER); 645 break; 646 default: 647 tgt.setValue(Conformance.DocumentMode.NULL); 648 break; 649 } 650 } 651 return tgt; 652 } 653 654 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.DocumentMode> convertDocumentMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.DocumentMode> src) throws FHIRException { 655 if (src == null || src.isEmpty()) 656 return null; 657 org.hl7.fhir.r4.model.Enumeration<CapabilityStatement.DocumentMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new CapabilityStatement.DocumentModeEnumFactory()); 658 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 659 if (src.getValue() == null) { 660 tgt.setValue(null); 661 } else { 662 switch (src.getValue()) { 663 case PRODUCER: 664 tgt.setValue(CapabilityStatement.DocumentMode.PRODUCER); 665 break; 666 case CONSUMER: 667 tgt.setValue(CapabilityStatement.DocumentMode.CONSUMER); 668 break; 669 default: 670 tgt.setValue(CapabilityStatement.DocumentMode.NULL); 671 break; 672 } 673 } 674 return tgt; 675 } 676 677 public static org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent src) throws FHIRException { 678 if (src == null || src.isEmpty()) 679 return null; 680 org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent(); 681 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 682 if (src.hasCode()) 683 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 684 if (src.hasDocumentation()) 685 tgt.setDocumentation(src.getDocumentation()); 686 return tgt; 687 } 688 689 public static org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.r4.model.CapabilityStatement.ResourceInteractionComponent src) throws FHIRException { 690 if (src == null || src.isEmpty()) 691 return null; 692 org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent(); 693 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 694 if (src.hasCode()) 695 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 696 if (src.hasDocumentation()) 697 tgt.setDocumentation(src.getDocumentation()); 698 return tgt; 699 } 700 701 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ResourceVersionPolicy> src) throws FHIRException { 702 if (src == null || src.isEmpty()) 703 return null; 704 org.hl7.fhir.r4.model.Enumeration<CapabilityStatement.ResourceVersionPolicy> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new CapabilityStatement.ResourceVersionPolicyEnumFactory()); 705 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 706 if (src.getValue() == null) { 707 tgt.setValue(null); 708 } else { 709 switch (src.getValue()) { 710 case NOVERSION: 711 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.NOVERSION); 712 break; 713 case VERSIONED: 714 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.VERSIONED); 715 break; 716 case VERSIONEDUPDATE: 717 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.VERSIONEDUPDATE); 718 break; 719 default: 720 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.NULL); 721 break; 722 } 723 } 724 return tgt; 725 } 726 727 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.ResourceVersionPolicy> src) throws FHIRException { 728 if (src == null || src.isEmpty()) 729 return null; 730 Enumeration<Conformance.ResourceVersionPolicy> tgt = new Enumeration<>(new Conformance.ResourceVersionPolicyEnumFactory()); 731 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 732 if (src.getValue() == null) { 733 tgt.setValue(null); 734 } else { 735 switch (src.getValue()) { 736 case NOVERSION: 737 tgt.setValue(Conformance.ResourceVersionPolicy.NOVERSION); 738 break; 739 case VERSIONED: 740 tgt.setValue(Conformance.ResourceVersionPolicy.VERSIONED); 741 break; 742 case VERSIONEDUPDATE: 743 tgt.setValue(Conformance.ResourceVersionPolicy.VERSIONEDUPDATE); 744 break; 745 default: 746 tgt.setValue(Conformance.ResourceVersionPolicy.NULL); 747 break; 748 } 749 } 750 return tgt; 751 } 752 753 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode> convertRestfulConformanceMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.RestfulConformanceMode> src) throws FHIRException { 754 if (src == null || src.isEmpty()) 755 return null; 756 org.hl7.fhir.r4.model.Enumeration<CapabilityStatement.RestfulCapabilityMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new CapabilityStatement.RestfulCapabilityModeEnumFactory()); 757 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 758 if (src.getValue() == null) { 759 tgt.setValue(null); 760 } else { 761 switch (src.getValue()) { 762 case CLIENT: 763 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.CLIENT); 764 break; 765 case SERVER: 766 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.SERVER); 767 break; 768 default: 769 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.NULL); 770 break; 771 } 772 } 773 return tgt; 774 } 775 776 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.RestfulConformanceMode> convertRestfulConformanceMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.RestfulCapabilityMode> src) throws FHIRException { 777 if (src == null || src.isEmpty()) 778 return null; 779 Enumeration<Conformance.RestfulConformanceMode> tgt = new Enumeration<>(new Conformance.RestfulConformanceModeEnumFactory()); 780 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 781 if (src.getValue() == null) { 782 tgt.setValue(null); 783 } else { 784 switch (src.getValue()) { 785 case CLIENT: 786 tgt.setValue(Conformance.RestfulConformanceMode.CLIENT); 787 break; 788 case SERVER: 789 tgt.setValue(Conformance.RestfulConformanceMode.SERVER); 790 break; 791 default: 792 tgt.setValue(Conformance.RestfulConformanceMode.NULL); 793 break; 794 } 795 } 796 return tgt; 797 } 798 799 public static org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent src) throws FHIRException { 800 if (src == null || src.isEmpty()) 801 return null; 802 org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent(); 803 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 804 if (src.hasCode()) 805 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 806 if (src.hasDocumentation()) 807 tgt.setDocumentation(src.getDocumentation()); 808 return tgt; 809 } 810 811 public static org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent src) throws FHIRException { 812 if (src == null || src.isEmpty()) 813 return null; 814 org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent tgt = new org.hl7.fhir.r4.model.CapabilityStatement.SystemInteractionComponent(); 815 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 816 if (src.hasCode()) 817 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 818 if (src.hasDocumentation()) 819 tgt.setDocumentation(src.getDocumentation()); 820 return tgt; 821 } 822 823 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction> src) throws FHIRException { 824 if (src == null || src.isEmpty()) 825 return null; 826 Enumeration<Conformance.SystemRestfulInteraction> tgt = new Enumeration<>(new Conformance.SystemRestfulInteractionEnumFactory()); 827 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 828 if (src.getValue() == null) { 829 tgt.setValue(null); 830 } else { 831 switch (src.getValue()) { 832 case TRANSACTION: 833 tgt.setValue(Conformance.SystemRestfulInteraction.TRANSACTION); 834 break; 835 case SEARCHSYSTEM: 836 tgt.setValue(Conformance.SystemRestfulInteraction.SEARCHSYSTEM); 837 break; 838 case HISTORYSYSTEM: 839 tgt.setValue(Conformance.SystemRestfulInteraction.HISTORYSYSTEM); 840 break; 841 default: 842 tgt.setValue(Conformance.SystemRestfulInteraction.NULL); 843 break; 844 } 845 } 846 return tgt; 847 } 848 849 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.SystemRestfulInteraction> src) throws FHIRException { 850 if (src == null || src.isEmpty()) 851 return null; 852 org.hl7.fhir.r4.model.Enumeration<SystemRestfulInteraction> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new CapabilityStatement.SystemRestfulInteractionEnumFactory()); 853 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 854 if (src.getValue() == null) { 855 tgt.setValue(null); 856 } else { 857 switch (src.getValue()) { 858 case TRANSACTION: 859 tgt.setValue(SystemRestfulInteraction.TRANSACTION); 860 break; 861 case SEARCHSYSTEM: 862 tgt.setValue(SystemRestfulInteraction.SEARCHSYSTEM); 863 break; 864 case HISTORYSYSTEM: 865 tgt.setValue(SystemRestfulInteraction.HISTORYSYSTEM); 866 break; 867 default: 868 tgt.setValue(SystemRestfulInteraction.NULL); 869 break; 870 } 871 } 872 return tgt; 873 } 874 875 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction> src) throws FHIRException { 876 if (src == null || src.isEmpty()) 877 return null; 878 Enumeration<Conformance.TypeRestfulInteraction> tgt = new Enumeration<>(new Conformance.TypeRestfulInteractionEnumFactory()); 879 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 880 if (src.getValue() == null) { 881 tgt.setValue(null); 882 } else { 883 switch (src.getValue()) { 884 case READ: 885 tgt.setValue(Conformance.TypeRestfulInteraction.READ); 886 break; 887 case VREAD: 888 tgt.setValue(Conformance.TypeRestfulInteraction.VREAD); 889 break; 890 case UPDATE: 891 tgt.setValue(Conformance.TypeRestfulInteraction.UPDATE); 892 break; 893 case DELETE: 894 tgt.setValue(Conformance.TypeRestfulInteraction.DELETE); 895 break; 896 case HISTORYINSTANCE: 897 tgt.setValue(Conformance.TypeRestfulInteraction.HISTORYINSTANCE); 898 break; 899 case HISTORYTYPE: 900 tgt.setValue(Conformance.TypeRestfulInteraction.HISTORYTYPE); 901 break; 902 case CREATE: 903 tgt.setValue(Conformance.TypeRestfulInteraction.CREATE); 904 break; 905 case SEARCHTYPE: 906 tgt.setValue(Conformance.TypeRestfulInteraction.SEARCHTYPE); 907 break; 908 default: 909 tgt.setValue(Conformance.TypeRestfulInteraction.NULL); 910 break; 911 } 912 } 913 return tgt; 914 } 915 916 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.TypeRestfulInteraction> src) throws FHIRException { 917 if (src == null || src.isEmpty()) 918 return null; 919 org.hl7.fhir.r4.model.Enumeration<CapabilityStatement.TypeRestfulInteraction> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new CapabilityStatement.TypeRestfulInteractionEnumFactory()); 920 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 921 if (src.getValue() == null) { 922 tgt.setValue(null); 923 } else { 924 switch (src.getValue()) { 925 case READ: 926 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.READ); 927 break; 928 case VREAD: 929 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.VREAD); 930 break; 931 case UPDATE: 932 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.UPDATE); 933 break; 934 case DELETE: 935 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.DELETE); 936 break; 937 case HISTORYINSTANCE: 938 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.HISTORYINSTANCE); 939 break; 940 case HISTORYTYPE: 941 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.HISTORYTYPE); 942 break; 943 case CREATE: 944 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.CREATE); 945 break; 946 case SEARCHTYPE: 947 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.SEARCHTYPE); 948 break; 949 default: 950 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.NULL); 951 break; 952 } 953 } 954 return tgt; 955 } 956 957 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode> convertConformanceEventMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode> src) throws FHIRException { 958 if (src == null || src.isEmpty()) return null; 959 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityModeEnumFactory()); 960 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 961 if (src.getValue() == null) { 962 tgt.setValue(null); 963} else { 964 switch(src.getValue()) { 965 case SENDER: 966 tgt.setValue(CapabilityStatement.EventCapabilityMode.SENDER); 967 break; 968 case RECEIVER: 969 tgt.setValue(CapabilityStatement.EventCapabilityMode.RECEIVER); 970 break; 971 default: 972 tgt.setValue(CapabilityStatement.EventCapabilityMode.NULL); 973 break; 974 } 975} 976 return tgt; 977 } 978 979 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode> convertConformanceEventMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.CapabilityStatement.EventCapabilityMode> src) throws FHIRException { 980 if (src == null || src.isEmpty()) return null; 981 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Conformance.ConformanceEventModeEnumFactory()); 982 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 983 if (src.getValue() == null) { 984 tgt.setValue(null); 985} else { 986 switch(src.getValue()) { 987 case SENDER: 988 tgt.setValue(Conformance.ConformanceEventMode.SENDER); 989 break; 990 case RECEIVER: 991 tgt.setValue(Conformance.ConformanceEventMode.RECEIVER); 992 break; 993 default: 994 tgt.setValue(Conformance.ConformanceEventMode.NULL); 995 break; 996 } 997} 998 return tgt; 999 } 1000}