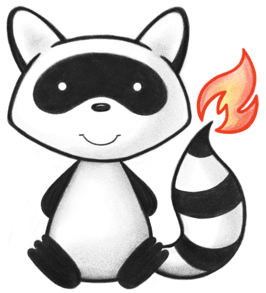
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Attachment10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Instant10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r4.model.DiagnosticReport; 012import org.hl7.fhir.r4.model.Enumeration; 013 014public class DiagnosticReport10_40 { 015 016 public static org.hl7.fhir.dstu2.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.r4.model.DiagnosticReport src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.dstu2.model.DiagnosticReport tgt = new org.hl7.fhir.dstu2.model.DiagnosticReport(); 020 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 025 if (src.hasCategory()) 026 tgt.setCategory(CodeableConcept10_40.convertCodeableConcept(src.getCategoryFirstRep())); 027 if (src.hasCode()) 028 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 029 if (src.hasSubject()) 030 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 031 if (src.hasEncounter()) 032 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 033 if (src.hasEffective()) 034 tgt.setEffective(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getEffective())); 035 if (src.hasIssuedElement()) 036 tgt.setIssuedElement(Instant10_40.convertInstant(src.getIssuedElement())); 037 for (org.hl7.fhir.r4.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference10_40.convertReference(t)); 038 for (org.hl7.fhir.r4.model.Reference t : src.getResult()) tgt.addResult(Reference10_40.convertReference(t)); 039 for (org.hl7.fhir.r4.model.Reference t : src.getImagingStudy()) 040 tgt.addImagingStudy(Reference10_40.convertReference(t)); 041 for (org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent t : src.getMedia()) 042 tgt.addImage(convertDiagnosticReportImageComponent(t)); 043 if (src.hasConclusionElement()) 044 tgt.setConclusionElement(String10_40.convertString(src.getConclusionElement())); 045 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getConclusionCode()) 046 tgt.addCodedDiagnosis(CodeableConcept10_40.convertCodeableConcept(t)); 047 for (org.hl7.fhir.r4.model.Attachment t : src.getPresentedForm()) 048 tgt.addPresentedForm(Attachment10_40.convertAttachment(t)); 049 return tgt; 050 } 051 052 public static org.hl7.fhir.r4.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.dstu2.model.DiagnosticReport src) throws FHIRException { 053 if (src == null || src.isEmpty()) 054 return null; 055 org.hl7.fhir.r4.model.DiagnosticReport tgt = new org.hl7.fhir.r4.model.DiagnosticReport(); 056 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 057 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 058 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 059 if (src.hasStatus()) 060 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 061 if (src.hasCategory()) 062 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(src.getCategory())); 063 if (src.hasCode()) 064 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 065 if (src.hasSubject()) 066 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 067 if (src.hasEncounter()) 068 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 069 if (src.hasEffective()) 070 tgt.setEffective(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getEffective())); 071 if (src.hasIssuedElement()) 072 tgt.setIssuedElement(Instant10_40.convertInstant(src.getIssuedElement())); 073 for (org.hl7.fhir.dstu2.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference10_40.convertReference(t)); 074 for (org.hl7.fhir.dstu2.model.Reference t : src.getResult()) tgt.addResult(Reference10_40.convertReference(t)); 075 for (org.hl7.fhir.dstu2.model.Reference t : src.getImagingStudy()) 076 tgt.addImagingStudy(Reference10_40.convertReference(t)); 077 for (org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent t : src.getImage()) 078 tgt.addMedia(convertDiagnosticReportImageComponent(t)); 079 if (src.hasConclusionElement()) 080 tgt.setConclusionElement(String10_40.convertString(src.getConclusionElement())); 081 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCodedDiagnosis()) 082 tgt.addConclusionCode(CodeableConcept10_40.convertCodeableConcept(t)); 083 for (org.hl7.fhir.dstu2.model.Attachment t : src.getPresentedForm()) 084 tgt.addPresentedForm(Attachment10_40.convertAttachment(t)); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent convertDiagnosticReportImageComponent(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent src) throws FHIRException { 089 if (src == null || src.isEmpty()) 090 return null; 091 org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent tgt = new org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent(); 092 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 093 if (src.hasCommentElement()) 094 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 095 if (src.hasLink()) 096 tgt.setLink(Reference10_40.convertReference(src.getLink())); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent convertDiagnosticReportImageComponent(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent tgt = new org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent(); 104 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 105 if (src.hasCommentElement()) 106 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 107 if (src.hasLink()) 108 tgt.setLink(Reference10_40.convertReference(src.getLink())); 109 return tgt; 110 } 111 112 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 113 if (src == null || src.isEmpty()) 114 return null; 115 Enumeration<DiagnosticReport.DiagnosticReportStatus> tgt = new Enumeration<>(new DiagnosticReport.DiagnosticReportStatusEnumFactory()); 116 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 117 if (src.getValue() == null) { 118 tgt.setValue(null); 119 } else { 120 switch (src.getValue()) { 121 case REGISTERED: 122 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.REGISTERED); 123 break; 124 case PARTIAL: 125 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PARTIAL); 126 break; 127 case FINAL: 128 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.FINAL); 129 break; 130 case CORRECTED: 131 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CORRECTED); 132 break; 133 case APPENDED: 134 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.APPENDED); 135 break; 136 case CANCELLED: 137 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CANCELLED); 138 break; 139 case ENTEREDINERROR: 140 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 141 break; 142 default: 143 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.NULL); 144 break; 145 } 146 } 147 return tgt; 148 } 149 150 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 151 if (src == null || src.isEmpty()) 152 return null; 153 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatusEnumFactory()); 154 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 155 if (src.getValue() == null) { 156 tgt.setValue(null); 157 } else { 158 switch (src.getValue()) { 159 case REGISTERED: 160 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.REGISTERED); 161 break; 162 case PARTIAL: 163 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.PARTIAL); 164 break; 165 case FINAL: 166 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.FINAL); 167 break; 168 case CORRECTED: 169 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.CORRECTED); 170 break; 171 case APPENDED: 172 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.APPENDED); 173 break; 174 case CANCELLED: 175 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.CANCELLED); 176 break; 177 case ENTEREDINERROR: 178 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 179 break; 180 default: 181 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.NULL); 182 break; 183 } 184 } 185 return tgt; 186 } 187}