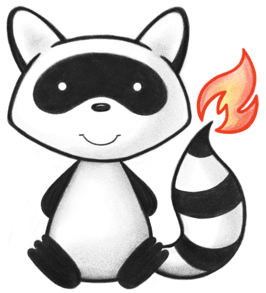
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Attachment10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Period10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 011import org.hl7.fhir.dstu2.model.CodeableConcept; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.DocumentReference.ReferredDocumentStatus; 014 015public class DocumentReference10_40 { 016 017 static public CodeableConcept convertDocStatus(ReferredDocumentStatus docStatus) { 018 CodeableConcept cc = new CodeableConcept(); 019 switch (docStatus) { 020 case AMENDED: 021 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("amended"); 022 break; 023 case ENTEREDINERROR: 024 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("entered-in-error"); 025 break; 026 case FINAL: 027 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("final"); 028 break; 029 case PRELIMINARY: 030 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("preliminary"); 031 break; 032 default: 033 return null; 034 } 035 return cc; 036 } 037 038 static public ReferredDocumentStatus convertDocStatus(CodeableConcept cc) { 039 if (CodeableConcept10_40.hasConcept(cc, "http://hl7.org/fhir/composition-status", "preliminary")) 040 return ReferredDocumentStatus.PRELIMINARY; 041 if (CodeableConcept10_40.hasConcept(cc, "http://hl7.org/fhir/composition-status", "final")) 042 return ReferredDocumentStatus.FINAL; 043 if (CodeableConcept10_40.hasConcept(cc, "http://hl7.org/fhir/composition-status", "amended")) 044 return ReferredDocumentStatus.AMENDED; 045 if (CodeableConcept10_40.hasConcept(cc, "http://hl7.org/fhir/composition-status", "entered-in-error")) 046 return ReferredDocumentStatus.ENTEREDINERROR; 047 return null; 048 } 049 050 public static org.hl7.fhir.dstu2.model.DocumentReference convertDocumentReference(org.hl7.fhir.r4.model.DocumentReference src) throws FHIRException { 051 if (src == null || src.isEmpty()) 052 return null; 053 org.hl7.fhir.dstu2.model.DocumentReference tgt = new org.hl7.fhir.dstu2.model.DocumentReference(); 054 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 055 if (src.hasMasterIdentifier()) 056 tgt.setMasterIdentifier(Identifier10_40.convertIdentifier(src.getMasterIdentifier())); 057 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 058 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 059 if (src.hasSubject()) 060 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 061 if (src.hasType()) 062 tgt.setType(CodeableConcept10_40.convertCodeableConcept(src.getType())); 063 if (src.hasCategory()) 064 tgt.setClass_(CodeableConcept10_40.convertCodeableConcept(src.getCategoryFirstRep())); 065 if (src.hasCustodian()) 066 tgt.setCustodian(Reference10_40.convertReference(src.getCustodian())); 067 if (src.hasAuthenticator()) 068 tgt.setAuthenticator(Reference10_40.convertReference(src.getAuthenticator())); 069 if (src.hasDate()) 070 tgt.setCreated(src.getDate()); 071 if (src.hasStatus()) 072 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 073 if (src.hasDocStatus()) 074 tgt.setDocStatus(convertDocStatus(src.getDocStatus())); 075 for (org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 076 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 077 if (src.hasDescriptionElement()) 078 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 079 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSecurityLabel()) 080 tgt.addSecurityLabel(CodeableConcept10_40.convertCodeableConcept(t)); 081 for (org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 082 tgt.addContent(convertDocumentReferenceContentComponent(t)); 083 if (src.hasContext()) 084 tgt.setContext(convertDocumentReferenceContextComponent(src.getContext())); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.r4.model.DocumentReference convertDocumentReference(org.hl7.fhir.dstu2.model.DocumentReference src) throws FHIRException { 089 if (src == null || src.isEmpty()) 090 return null; 091 org.hl7.fhir.r4.model.DocumentReference tgt = new org.hl7.fhir.r4.model.DocumentReference(); 092 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 093 if (src.hasMasterIdentifier()) 094 tgt.setMasterIdentifier(Identifier10_40.convertIdentifier(src.getMasterIdentifier())); 095 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 096 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 097 if (src.hasSubject()) 098 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 099 if (src.hasType()) 100 tgt.setType(CodeableConcept10_40.convertCodeableConcept(src.getType())); 101 if (src.hasClass_()) 102 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(src.getClass_())); 103 if (src.hasCustodian()) 104 tgt.setCustodian(Reference10_40.convertReference(src.getCustodian())); 105 if (src.hasAuthenticator()) 106 tgt.setAuthenticator(Reference10_40.convertReference(src.getAuthenticator())); 107 if (src.hasCreated()) 108 tgt.setDate(src.getCreated()); 109 if (src.hasStatus()) 110 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 111 if (src.hasDocStatus()) 112 tgt.setDocStatus(convertDocStatus(src.getDocStatus())); 113 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 114 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 115 if (src.hasDescriptionElement()) 116 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 117 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getSecurityLabel()) 118 tgt.addSecurityLabel(CodeableConcept10_40.convertCodeableConcept(t)); 119 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 120 tgt.addContent(convertDocumentReferenceContentComponent(t)); 121 if (src.hasContext()) 122 tgt.setContext(convertDocumentReferenceContextComponent(src.getContext())); 123 return tgt; 124 } 125 126 public static org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 127 if (src == null || src.isEmpty()) 128 return null; 129 org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent(); 130 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 131 if (src.hasAttachment()) 132 tgt.setAttachment(Attachment10_40.convertAttachment(src.getAttachment())); 133 for (org.hl7.fhir.dstu2.model.Coding t : src.getFormat()) tgt.setFormat(Coding10_40.convertCoding(t)); 134 return tgt; 135 } 136 137 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 138 if (src == null || src.isEmpty()) 139 return null; 140 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent(); 141 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 142 if (src.hasAttachment()) 143 tgt.setAttachment(Attachment10_40.convertAttachment(src.getAttachment())); 144 if (src.hasFormat()) 145 tgt.addFormat(Coding10_40.convertCoding(src.getFormat())); 146 return tgt; 147 } 148 149 public static org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContextComponent convertDocumentReferenceContextComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent src) throws FHIRException { 150 if (src == null || src.isEmpty()) 151 return null; 152 org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContextComponent tgt = new org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContextComponent(); 153 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 154 if (src.hasEncounter()) 155 tgt.addEncounter(Reference10_40.convertReference(src.getEncounter())); 156 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getEvent()) 157 tgt.addEvent(CodeableConcept10_40.convertCodeableConcept(t)); 158 if (src.hasPeriod()) 159 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 160 if (src.hasFacilityType()) 161 tgt.setFacilityType(CodeableConcept10_40.convertCodeableConcept(src.getFacilityType())); 162 if (src.hasPracticeSetting()) 163 tgt.setPracticeSetting(CodeableConcept10_40.convertCodeableConcept(src.getPracticeSetting())); 164 if (src.hasSourcePatientInfo()) 165 tgt.setSourcePatientInfo(Reference10_40.convertReference(src.getSourcePatientInfo())); 166 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent t : src.getRelated()) 167 tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 168 return tgt; 169 } 170 171 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent convertDocumentReferenceContextComponent(org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContextComponent src) throws FHIRException { 172 if (src == null || src.isEmpty()) 173 return null; 174 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent(); 175 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 176 if (src.hasEncounter()) 177 tgt.setEncounter(Reference10_40.convertReference(src.getEncounterFirstRep())); 178 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getEvent()) 179 tgt.addEvent(CodeableConcept10_40.convertCodeableConcept(t)); 180 if (src.hasPeriod()) 181 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 182 if (src.hasFacilityType()) 183 tgt.setFacilityType(CodeableConcept10_40.convertCodeableConcept(src.getFacilityType())); 184 if (src.hasPracticeSetting()) 185 tgt.setPracticeSetting(CodeableConcept10_40.convertCodeableConcept(src.getPracticeSetting())); 186 if (src.hasSourcePatientInfo()) 187 tgt.setSourcePatientInfo(Reference10_40.convertReference(src.getSourcePatientInfo())); 188 for (org.hl7.fhir.r4.model.Reference t : src.getRelated()) 189 tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 190 return tgt; 191 } 192 193 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.r4.model.Reference src) throws FHIRException { 194 if (src == null || src.isEmpty()) 195 return null; 196 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent(); 197 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 198 if (src.hasIdentifier()) 199 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 200 tgt.setRef(Reference10_40.convertReference(src)); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.r4.model.Reference convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent src) throws FHIRException { 205 if (src == null || src.isEmpty()) 206 return null; 207 org.hl7.fhir.r4.model.Reference tgt = Reference10_40.convertReference(src.getRef()); 208 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 209 if (src.hasIdentifier()) 210 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 211 return tgt; 212 } 213 214 public static org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 215 if (src == null || src.isEmpty()) 216 return null; 217 org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent(); 218 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 219 if (src.hasCode()) 220 tgt.setCodeElement(convertDocumentRelationshipType(src.getCodeElement())); 221 if (src.hasTarget()) 222 tgt.setTarget(Reference10_40.convertReference(src.getTarget())); 223 return tgt; 224 } 225 226 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 227 if (src == null || src.isEmpty()) 228 return null; 229 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent(); 230 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 231 if (src.hasCode()) 232 tgt.setCodeElement(convertDocumentRelationshipType(src.getCodeElement())); 233 if (src.hasTarget()) 234 tgt.setTarget(Reference10_40.convertReference(src.getTarget())); 235 return tgt; 236 } 237 238 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 239 if (src == null || src.isEmpty()) 240 return null; 241 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatusEnumFactory()); 242 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 243 switch (src.getValue()) { 244 case CURRENT: 245 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.CURRENT); 246 break; 247 case SUPERSEDED: 248 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.SUPERSEDED); 249 break; 250 case ENTEREDINERROR: 251 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 252 break; 253 default: 254 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.NULL); 255 break; 256 } 257 return tgt; 258 } 259 260 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 261 if (src == null || src.isEmpty()) 262 return null; 263 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatusEnumFactory()); 264 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 265 switch (src.getValue()) { 266 case CURRENT: 267 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.CURRENT); 268 break; 269 case SUPERSEDED: 270 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.SUPERSEDED); 271 break; 272 case ENTEREDINERROR: 273 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 274 break; 275 default: 276 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus.NULL); 277 break; 278 } 279 return tgt; 280 } 281 282 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType> src) throws FHIRException { 283 if (src == null || src.isEmpty()) 284 return null; 285 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipTypeEnumFactory()); 286 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 287 switch (src.getValue()) { 288 case REPLACES: 289 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.REPLACES); 290 break; 291 case TRANSFORMS: 292 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.TRANSFORMS); 293 break; 294 case SIGNS: 295 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.SIGNS); 296 break; 297 case APPENDS: 298 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.APPENDS); 299 break; 300 default: 301 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.NULL); 302 break; 303 } 304 return tgt; 305 } 306 307 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> src) throws FHIRException { 308 if (src == null || src.isEmpty()) 309 return null; 310 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipTypeEnumFactory()); 311 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 312 switch (src.getValue()) { 313 case REPLACES: 314 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.REPLACES); 315 break; 316 case TRANSFORMS: 317 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.TRANSFORMS); 318 break; 319 case SIGNS: 320 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.SIGNS); 321 break; 322 case APPENDS: 323 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.APPENDS); 324 break; 325 default: 326 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.NULL); 327 break; 328 } 329 return tgt; 330 } 331}