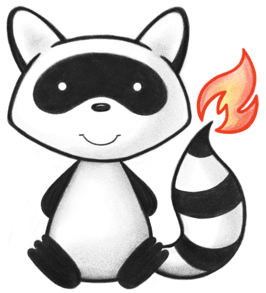
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.exceptions.FHIRException; 005 006public class Enumerations10_40 { 007 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> src) throws FHIRException { 008 if (src == null || src.isEmpty()) return null; 009 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory()); 010 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 011 if (src.getValue() == null) { 012 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.NULL); 013 } else { 014 switch (src.getValue()) { 015 case MALE: 016 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.MALE); 017 break; 018 case FEMALE: 019 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.FEMALE); 020 break; 021 case OTHER: 022 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.OTHER); 023 break; 024 case UNKNOWN: 025 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.UNKNOWN); 026 break; 027 default: 028 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.NULL); 029 break; 030 } 031 } 032 return tgt; 033 } 034 035 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.AdministrativeGender> src) throws FHIRException { 036 if (src == null || src.isEmpty()) return null; 037 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory()); 038 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 039 if (src.getValue() == null) { 040 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.NULL); 041 } else { 042 switch (src.getValue()) { 043 case MALE: 044 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.MALE); 045 break; 046 case FEMALE: 047 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.FEMALE); 048 break; 049 case OTHER: 050 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.OTHER); 051 break; 052 case UNKNOWN: 053 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.UNKNOWN); 054 break; 055 default: 056 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.NULL); 057 break; 058 } 059 } 060 return tgt; 061 } 062 063 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.SearchParamType> src) throws FHIRException { 064 if (src == null || src.isEmpty()) return null; 065 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory()); 066 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 067 if (src.getValue() == null) { 068 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.NULL); 069 } else { 070 switch (src.getValue()) { 071 case NUMBER: 072 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.NUMBER); 073 break; 074 case DATE: 075 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.DATE); 076 break; 077 case STRING: 078 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.STRING); 079 break; 080 case TOKEN: 081 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.TOKEN); 082 break; 083 case REFERENCE: 084 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.REFERENCE); 085 break; 086 case COMPOSITE: 087 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.COMPOSITE); 088 break; 089 case QUANTITY: 090 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.QUANTITY); 091 break; 092 case URI: 093 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.URI); 094 break; 095 default: 096 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.NULL); 097 break; 098 } 099 } 100 return tgt; 101 } 102 103 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> src) throws FHIRException { 104 if (src == null || src.isEmpty()) return null; 105 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.SearchParamTypeEnumFactory()); 106 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 107 if (src.getValue() == null) { 108 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.NULL); 109 } else { 110 switch (src.getValue()) { 111 case NUMBER: 112 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.NUMBER); 113 break; 114 case DATE: 115 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.DATE); 116 break; 117 case STRING: 118 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.STRING); 119 break; 120 case TOKEN: 121 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.TOKEN); 122 break; 123 case REFERENCE: 124 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.REFERENCE); 125 break; 126 case COMPOSITE: 127 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.COMPOSITE); 128 break; 129 case QUANTITY: 130 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.QUANTITY); 131 break; 132 case URI: 133 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.URI); 134 break; 135 default: 136 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.NULL); 137 break; 138 } 139 } 140 return tgt; 141 } 142 143 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> convertConformanceResourceStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> src) throws FHIRException { 144 if (src == null || src.isEmpty()) return null; 145 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory()); 146 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 147 if (src.getValue() == null) { 148 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.NULL); 149 } else { 150 switch (src.getValue()) { 151 case DRAFT: 152 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.DRAFT); 153 break; 154 case ACTIVE: 155 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.ACTIVE); 156 break; 157 case RETIRED: 158 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.RETIRED); 159 break; 160 default: 161 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.NULL); 162 break; 163 } 164 } 165 return tgt; 166 } 167 168 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> convertConformanceResourceStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> src) throws FHIRException { 169 if (src == null || src.isEmpty()) return null; 170 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory()); 171 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 172 if (src.getValue() == null) { 173 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.NULL); 174 } else { 175 switch (src.getValue()) { 176 case DRAFT: 177 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.DRAFT); 178 break; 179 case ACTIVE: 180 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.ACTIVE); 181 break; 182 case RETIRED: 183 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.RETIRED); 184 break; 185 default: 186 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.NULL); 187 break; 188 } 189 } 190 return tgt; 191 } 192}