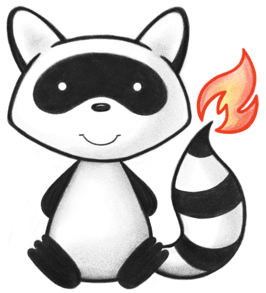
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Period10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.UnsignedInt10_40; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class Group10_40 { 014 015 public static org.hl7.fhir.r4.model.Group convertGroup(org.hl7.fhir.dstu2.model.Group src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 org.hl7.fhir.r4.model.Group tgt = new org.hl7.fhir.r4.model.Group(); 019 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 020 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 021 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 022 if (src.hasType()) 023 tgt.setTypeElement(convertGroupType(src.getTypeElement())); 024 if (src.hasActualElement()) 025 tgt.setActualElement(Boolean10_40.convertBoolean(src.getActualElement())); 026 if (src.hasCode()) 027 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 028 if (src.hasNameElement()) 029 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 030 if (src.hasQuantityElement()) 031 tgt.setQuantityElement(UnsignedInt10_40.convertUnsignedInt(src.getQuantityElement())); 032 for (org.hl7.fhir.dstu2.model.Group.GroupCharacteristicComponent t : src.getCharacteristic()) 033 tgt.addCharacteristic(convertGroupCharacteristicComponent(t)); 034 for (org.hl7.fhir.dstu2.model.Group.GroupMemberComponent t : src.getMember()) 035 tgt.addMember(convertGroupMemberComponent(t)); 036 return tgt; 037 } 038 039 public static org.hl7.fhir.dstu2.model.Group convertGroup(org.hl7.fhir.r4.model.Group src) throws FHIRException { 040 if (src == null || src.isEmpty()) 041 return null; 042 org.hl7.fhir.dstu2.model.Group tgt = new org.hl7.fhir.dstu2.model.Group(); 043 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 044 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 045 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 046 if (src.hasType()) 047 tgt.setTypeElement(convertGroupType(src.getTypeElement())); 048 if (src.hasActualElement()) 049 tgt.setActualElement(Boolean10_40.convertBoolean(src.getActualElement())); 050 if (src.hasCode()) 051 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 052 if (src.hasNameElement()) 053 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 054 if (src.hasQuantityElement()) 055 tgt.setQuantityElement(UnsignedInt10_40.convertUnsignedInt(src.getQuantityElement())); 056 for (org.hl7.fhir.r4.model.Group.GroupCharacteristicComponent t : src.getCharacteristic()) 057 tgt.addCharacteristic(convertGroupCharacteristicComponent(t)); 058 for (org.hl7.fhir.r4.model.Group.GroupMemberComponent t : src.getMember()) 059 tgt.addMember(convertGroupMemberComponent(t)); 060 return tgt; 061 } 062 063 public static org.hl7.fhir.r4.model.Group.GroupCharacteristicComponent convertGroupCharacteristicComponent(org.hl7.fhir.dstu2.model.Group.GroupCharacteristicComponent src) throws FHIRException { 064 if (src == null || src.isEmpty()) 065 return null; 066 org.hl7.fhir.r4.model.Group.GroupCharacteristicComponent tgt = new org.hl7.fhir.r4.model.Group.GroupCharacteristicComponent(); 067 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 068 if (src.hasCode()) 069 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 070 if (src.hasValue()) 071 tgt.setValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getValue())); 072 if (src.hasExcludeElement()) 073 tgt.setExcludeElement(Boolean10_40.convertBoolean(src.getExcludeElement())); 074 if (src.hasPeriod()) 075 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 076 return tgt; 077 } 078 079 public static org.hl7.fhir.dstu2.model.Group.GroupCharacteristicComponent convertGroupCharacteristicComponent(org.hl7.fhir.r4.model.Group.GroupCharacteristicComponent src) throws FHIRException { 080 if (src == null || src.isEmpty()) 081 return null; 082 org.hl7.fhir.dstu2.model.Group.GroupCharacteristicComponent tgt = new org.hl7.fhir.dstu2.model.Group.GroupCharacteristicComponent(); 083 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 084 if (src.hasCode()) 085 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 086 if (src.hasValue()) 087 tgt.setValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getValue())); 088 if (src.hasExcludeElement()) 089 tgt.setExcludeElement(Boolean10_40.convertBoolean(src.getExcludeElement())); 090 if (src.hasPeriod()) 091 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 092 return tgt; 093 } 094 095 public static org.hl7.fhir.r4.model.Group.GroupMemberComponent convertGroupMemberComponent(org.hl7.fhir.dstu2.model.Group.GroupMemberComponent src) throws FHIRException { 096 if (src == null || src.isEmpty()) 097 return null; 098 org.hl7.fhir.r4.model.Group.GroupMemberComponent tgt = new org.hl7.fhir.r4.model.Group.GroupMemberComponent(); 099 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 100 if (src.hasEntity()) 101 tgt.setEntity(Reference10_40.convertReference(src.getEntity())); 102 if (src.hasPeriod()) 103 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 104 if (src.hasInactiveElement()) 105 tgt.setInactiveElement(Boolean10_40.convertBoolean(src.getInactiveElement())); 106 return tgt; 107 } 108 109 public static org.hl7.fhir.dstu2.model.Group.GroupMemberComponent convertGroupMemberComponent(org.hl7.fhir.r4.model.Group.GroupMemberComponent src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 org.hl7.fhir.dstu2.model.Group.GroupMemberComponent tgt = new org.hl7.fhir.dstu2.model.Group.GroupMemberComponent(); 113 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 114 if (src.hasEntity()) 115 tgt.setEntity(Reference10_40.convertReference(src.getEntity())); 116 if (src.hasPeriod()) 117 tgt.setPeriod(Period10_40.convertPeriod(src.getPeriod())); 118 if (src.hasInactiveElement()) 119 tgt.setInactiveElement(Boolean10_40.convertBoolean(src.getInactiveElement())); 120 return tgt; 121 } 122 123 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Group.GroupType> convertGroupType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Group.GroupType> src) throws FHIRException { 124 if (src == null || src.isEmpty()) 125 return null; 126 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Group.GroupType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Group.GroupTypeEnumFactory()); 127 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 128 switch (src.getValue()) { 129 case PERSON: 130 tgt.setValue(org.hl7.fhir.dstu2.model.Group.GroupType.PERSON); 131 break; 132 case ANIMAL: 133 tgt.setValue(org.hl7.fhir.dstu2.model.Group.GroupType.ANIMAL); 134 break; 135 case PRACTITIONER: 136 tgt.setValue(org.hl7.fhir.dstu2.model.Group.GroupType.PRACTITIONER); 137 break; 138 case DEVICE: 139 tgt.setValue(org.hl7.fhir.dstu2.model.Group.GroupType.DEVICE); 140 break; 141 case MEDICATION: 142 tgt.setValue(org.hl7.fhir.dstu2.model.Group.GroupType.MEDICATION); 143 break; 144 case SUBSTANCE: 145 tgt.setValue(org.hl7.fhir.dstu2.model.Group.GroupType.SUBSTANCE); 146 break; 147 default: 148 tgt.setValue(org.hl7.fhir.dstu2.model.Group.GroupType.NULL); 149 break; 150 } 151 return tgt; 152 } 153 154 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Group.GroupType> convertGroupType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Group.GroupType> src) throws FHIRException { 155 if (src == null || src.isEmpty()) 156 return null; 157 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Group.GroupType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Group.GroupTypeEnumFactory()); 158 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 159 switch (src.getValue()) { 160 case PERSON: 161 tgt.setValue(org.hl7.fhir.r4.model.Group.GroupType.PERSON); 162 break; 163 case ANIMAL: 164 tgt.setValue(org.hl7.fhir.r4.model.Group.GroupType.ANIMAL); 165 break; 166 case PRACTITIONER: 167 tgt.setValue(org.hl7.fhir.r4.model.Group.GroupType.PRACTITIONER); 168 break; 169 case DEVICE: 170 tgt.setValue(org.hl7.fhir.r4.model.Group.GroupType.DEVICE); 171 break; 172 case MEDICATION: 173 tgt.setValue(org.hl7.fhir.r4.model.Group.GroupType.MEDICATION); 174 break; 175 case SUBSTANCE: 176 tgt.setValue(org.hl7.fhir.r4.model.Group.GroupType.SUBSTANCE); 177 break; 178 default: 179 tgt.setValue(org.hl7.fhir.r4.model.Group.GroupType.NULL); 180 break; 181 } 182 return tgt; 183 } 184}