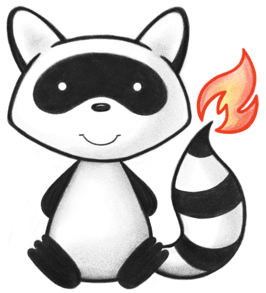
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Attachment10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Period10_40; 012import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 013import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 014import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Time10_40; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r4.model.Enumeration; 017import org.hl7.fhir.r4.model.HealthcareService; 018 019public class HealthcareService10_40 { 020 021 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.HealthcareService.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek> src) throws FHIRException { 022 if (src == null || src.isEmpty()) 023 return null; 024 Enumeration<HealthcareService.DaysOfWeek> tgt = new Enumeration<>(new HealthcareService.DaysOfWeekEnumFactory()); 025 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 026 if (src.getValue() == null) { 027 tgt.setValue(null); 028 } else { 029 switch (src.getValue()) { 030 case MON: 031 tgt.setValue(HealthcareService.DaysOfWeek.MON); 032 break; 033 case TUE: 034 tgt.setValue(HealthcareService.DaysOfWeek.TUE); 035 break; 036 case WED: 037 tgt.setValue(HealthcareService.DaysOfWeek.WED); 038 break; 039 case THU: 040 tgt.setValue(HealthcareService.DaysOfWeek.THU); 041 break; 042 case FRI: 043 tgt.setValue(HealthcareService.DaysOfWeek.FRI); 044 break; 045 case SAT: 046 tgt.setValue(HealthcareService.DaysOfWeek.SAT); 047 break; 048 case SUN: 049 tgt.setValue(HealthcareService.DaysOfWeek.SUN); 050 break; 051 default: 052 tgt.setValue(HealthcareService.DaysOfWeek.NULL); 053 break; 054 } 055 } 056 return tgt; 057 } 058 059 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.HealthcareService.DaysOfWeek> src) throws FHIRException { 060 if (src == null || src.isEmpty()) 061 return null; 062 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeekEnumFactory()); 063 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 064 if (src.getValue() == null) { 065 tgt.setValue(null); 066 } else { 067 switch (src.getValue()) { 068 case MON: 069 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.MON); 070 break; 071 case TUE: 072 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.TUE); 073 break; 074 case WED: 075 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.WED); 076 break; 077 case THU: 078 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.THU); 079 break; 080 case FRI: 081 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.FRI); 082 break; 083 case SAT: 084 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.SAT); 085 break; 086 case SUN: 087 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.SUN); 088 break; 089 default: 090 tgt.setValue(org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek.NULL); 091 break; 092 } 093 } 094 return tgt; 095 } 096 097 public static org.hl7.fhir.r4.model.HealthcareService convertHealthcareService(org.hl7.fhir.dstu2.model.HealthcareService src) throws FHIRException { 098 if (src == null || src.isEmpty()) 099 return null; 100 org.hl7.fhir.r4.model.HealthcareService tgt = new org.hl7.fhir.r4.model.HealthcareService(); 101 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 102 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 103 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 104 if (src.hasProvidedBy()) 105 tgt.setProvidedBy(Reference10_40.convertReference(src.getProvidedBy())); 106 for (org.hl7.fhir.dstu2.model.HealthcareService.ServiceTypeComponent t : src.getServiceType()) { 107 for (org.hl7.fhir.dstu2.model.CodeableConcept tj : t.getSpecialty()) 108 tgt.addSpecialty(CodeableConcept10_40.convertCodeableConcept(tj)); 109 } 110 if (src.hasLocation()) 111 tgt.addLocation(Reference10_40.convertReference(src.getLocation())); 112 if (src.hasCommentElement()) 113 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 114 if (src.hasExtraDetails()) 115 tgt.setExtraDetails(src.getExtraDetails()); 116 if (src.hasPhoto()) 117 tgt.setPhoto(Attachment10_40.convertAttachment(src.getPhoto())); 118 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 119 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 120 for (org.hl7.fhir.dstu2.model.Reference t : src.getCoverageArea()) 121 tgt.addCoverageArea(Reference10_40.convertReference(t)); 122 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getServiceProvisionCode()) 123 tgt.addServiceProvisionCode(CodeableConcept10_40.convertCodeableConcept(t)); 124 if (src.hasEligibility()) 125 tgt.getEligibilityFirstRep().setCode(CodeableConcept10_40.convertCodeableConcept(src.getEligibility())); 126 if (src.hasEligibilityNote()) 127 tgt.getEligibilityFirstRep().setComment(src.getEligibilityNote()); 128 for (org.hl7.fhir.dstu2.model.StringType t : src.getProgramName()) tgt.addProgram().setText(t.getValue()); 129 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCharacteristic()) 130 tgt.addCharacteristic(CodeableConcept10_40.convertCodeableConcept(t)); 131 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReferralMethod()) 132 tgt.addReferralMethod(CodeableConcept10_40.convertCodeableConcept(t)); 133 if (src.hasAppointmentRequiredElement()) 134 tgt.setAppointmentRequiredElement(Boolean10_40.convertBoolean(src.getAppointmentRequiredElement())); 135 for (org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 136 tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 137 for (org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 138 tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 139 if (src.hasAvailabilityExceptionsElement()) 140 tgt.setAvailabilityExceptionsElement(String10_40.convertString(src.getAvailabilityExceptionsElement())); 141 return tgt; 142 } 143 144 public static org.hl7.fhir.dstu2.model.HealthcareService convertHealthcareService(org.hl7.fhir.r4.model.HealthcareService src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 org.hl7.fhir.dstu2.model.HealthcareService tgt = new org.hl7.fhir.dstu2.model.HealthcareService(); 148 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 149 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 150 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 151 if (src.hasProvidedBy()) 152 tgt.setProvidedBy(Reference10_40.convertReference(src.getProvidedBy())); 153 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialty()) { 154 if (!tgt.hasServiceType()) 155 tgt.addServiceType(); 156 tgt.getServiceType().get(0).addSpecialty(CodeableConcept10_40.convertCodeableConcept(t)); 157 } 158 for (org.hl7.fhir.r4.model.Reference t : src.getLocation()) tgt.setLocation(Reference10_40.convertReference(t)); 159 if (src.hasCommentElement()) 160 tgt.setCommentElement(String10_40.convertString(src.getCommentElement())); 161 if (src.hasExtraDetails()) 162 tgt.setExtraDetails(src.getExtraDetails()); 163 if (src.hasPhoto()) 164 tgt.setPhoto(Attachment10_40.convertAttachment(src.getPhoto())); 165 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 166 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 167 for (org.hl7.fhir.r4.model.Reference t : src.getCoverageArea()) 168 tgt.addCoverageArea(Reference10_40.convertReference(t)); 169 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceProvisionCode()) 170 tgt.addServiceProvisionCode(CodeableConcept10_40.convertCodeableConcept(t)); 171 tgt.setEligibility(CodeableConcept10_40.convertCodeableConcept(src.getEligibilityFirstRep().getCode())); 172 if (src.hasCommentElement()) 173 tgt.setEligibilityNoteElement(String10_40.convertString(src.getCommentElement())); 174 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getProgram()) 175 if (t.hasText()) 176 tgt.addProgramName(t.getText()); 177 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCharacteristic()) 178 tgt.addCharacteristic(CodeableConcept10_40.convertCodeableConcept(t)); 179 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReferralMethod()) 180 tgt.addReferralMethod(CodeableConcept10_40.convertCodeableConcept(t)); 181 if (src.hasAppointmentRequiredElement()) 182 tgt.setAppointmentRequiredElement(Boolean10_40.convertBoolean(src.getAppointmentRequiredElement())); 183 for (org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 184 tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 185 for (org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 186 tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 187 if (src.hasAvailabilityExceptionsElement()) 188 tgt.setAvailabilityExceptionsElement(String10_40.convertString(src.getAvailabilityExceptionsElement())); 189 return tgt; 190 } 191 192 public static org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 193 if (src == null || src.isEmpty()) 194 return null; 195 org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 196 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 197 tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 198 .map(HealthcareService10_40::convertDaysOfWeek) 199 .collect(Collectors.toList())); 200 if (src.hasAllDayElement()) 201 tgt.setAllDayElement(Boolean10_40.convertBoolean(src.getAllDayElement())); 202 if (src.hasAvailableStartTimeElement()) 203 tgt.setAvailableStartTimeElement(Time10_40.convertTime(src.getAvailableStartTimeElement())); 204 if (src.hasAvailableEndTimeElement()) 205 tgt.setAvailableEndTimeElement(Time10_40.convertTime(src.getAvailableEndTimeElement())); 206 return tgt; 207 } 208 209 public static org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 210 if (src == null || src.isEmpty()) 211 return null; 212 org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 213 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 214 tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 215 .map(HealthcareService10_40::convertDaysOfWeek) 216 .collect(Collectors.toList())); 217 if (src.hasAllDayElement()) 218 tgt.setAllDayElement(Boolean10_40.convertBoolean(src.getAllDayElement())); 219 if (src.hasAvailableStartTimeElement()) 220 tgt.setAvailableStartTimeElement(Time10_40.convertTime(src.getAvailableStartTimeElement())); 221 if (src.hasAvailableEndTimeElement()) 222 tgt.setAvailableEndTimeElement(Time10_40.convertTime(src.getAvailableEndTimeElement())); 223 return tgt; 224 } 225 226 public static org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 227 if (src == null || src.isEmpty()) 228 return null; 229 org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 230 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 231 if (src.hasDescriptionElement()) 232 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 233 if (src.hasDuring()) 234 tgt.setDuring(Period10_40.convertPeriod(src.getDuring())); 235 return tgt; 236 } 237 238 public static org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 239 if (src == null || src.isEmpty()) 240 return null; 241 org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 242 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 243 if (src.hasDescriptionElement()) 244 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 245 if (src.hasDuring()) 246 tgt.setDuring(Period10_40.convertPeriod(src.getDuring())); 247 return tgt; 248 } 249}