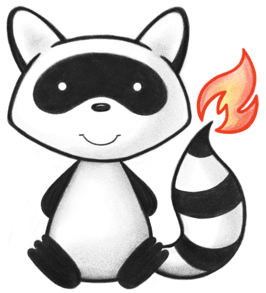
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext10_40; 006import org.hl7.fhir.convertors.conv10_40.VersionConvertor_10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Canonical10_40; 012import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Code10_40; 013import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 014import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 015import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 016import org.hl7.fhir.dstu2.model.ImplementationGuide; 017import org.hl7.fhir.dstu2.model.ImplementationGuide.GuidePageKind; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r4.model.Enumeration; 020import org.hl7.fhir.r4.model.ImplementationGuide.GuidePageGeneration; 021 022public class ImplementationGuide10_40 { 023 024 public static org.hl7.fhir.r4.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.dstu2.model.ImplementationGuide src) throws FHIRException { 025 if (src == null || src.isEmpty()) 026 return null; 027 org.hl7.fhir.r4.model.ImplementationGuide tgt = new org.hl7.fhir.r4.model.ImplementationGuide(); 028 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 029 if (src.hasUrlElement()) 030 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 031 if (src.hasVersionElement()) 032 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 033 if (src.hasNameElement()) 034 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 035 if (src.hasStatus()) 036 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 037 if (src.hasExperimental()) 038 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 039 if (src.hasPublisherElement()) 040 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 041 for (org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideContactComponent t : src.getContact()) 042 tgt.addContact(convertImplementationGuideContactComponent(t)); 043 if (src.hasDate()) 044 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 045 if (src.hasDescription()) 046 tgt.setDescription(src.getDescription()); 047 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 048 if (VersionConvertor_10_40.isJurisdiction(t)) 049 tgt.addJurisdiction(CodeableConcept10_40.convertCodeableConcept(t)); 050 else 051 tgt.addUseContext(CodeableConcept10_40.convertCodeableConceptToUsageContext(t)); 052 if (src.hasCopyright()) 053 tgt.setCopyright(src.getCopyright()); 054 if (src.hasFhirVersion()) 055 tgt.addFhirVersion(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 056 for (org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideDependencyComponent t : src.getDependency()) 057 tgt.addDependsOn(convertImplementationGuideDependencyComponent(t)); 058 for (org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent t : src.getPackage()) 059 tgt.getDefinition().addGrouping(convertImplementationGuidePackageComponent(tgt.getDefinition(), t)); 060 for (org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 061 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 062 tgt.getDefinition().setPage(convertImplementationGuidePageComponent(src.getPage())); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.dstu2.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.r4.model.ImplementationGuide src) throws FHIRException { 067 if (src == null || src.isEmpty()) 068 return null; 069 org.hl7.fhir.dstu2.model.ImplementationGuide tgt = new org.hl7.fhir.dstu2.model.ImplementationGuide(); 070 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 071 if (src.hasUrlElement()) 072 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 073 if (src.hasVersionElement()) 074 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 075 if (src.hasNameElement()) 076 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 077 if (src.hasStatus()) 078 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 079 if (src.hasExperimental()) 080 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 081 if (src.hasPublisherElement()) 082 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 083 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 084 tgt.addContact(convertImplementationGuideContactComponent(t)); 085 if (src.hasDate()) 086 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 087 if (src.hasDescription()) 088 tgt.setDescription(src.getDescription()); 089 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 090 if (t.hasValueCodeableConcept()) 091 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t.getValueCodeableConcept())); 092 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 093 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t)); 094 if (src.hasCopyright()) 095 tgt.setCopyright(src.getCopyright()); 096 for (Enumeration<org.hl7.fhir.r4.model.Enumerations.FHIRVersion> v : src.getFhirVersion()) { 097 tgt.setFhirVersion(v.asStringValue()); 098 } 099 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent t : src.getDependsOn()) 100 tgt.addDependency(convertImplementationGuideDependencyComponent(t)); 101 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent t : src.getDefinition().getGrouping()) 102 tgt.addPackage(convertImplementationGuidePackageComponent(t)); 103 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent t : src.getDefinition().getResource()) 104 findPackage(tgt.getPackage(), t.getGroupingId()).addResource(convertImplementationGuidePackageResourceComponent(t)); 105 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 106 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 107 tgt.setPage(convertImplementationGuidePageComponent(src.getDefinition().getPage())); 108 return tgt; 109 } 110 111 public static org.hl7.fhir.r4.model.ContactDetail convertImplementationGuideContactComponent(org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideContactComponent src) throws FHIRException { 112 if (src == null || src.isEmpty()) 113 return null; 114 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 115 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 116 if (src.hasNameElement()) 117 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 118 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 119 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 120 return tgt; 121 } 122 123 public static org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideContactComponent convertImplementationGuideContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 124 if (src == null || src.isEmpty()) 125 return null; 126 org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideContactComponent tgt = new org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideContactComponent(); 127 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 128 if (src.hasNameElement()) 129 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 130 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 131 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 132 return tgt; 133 } 134 135 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideDependencyComponent src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent(); 139 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 140 if (src.hasUri()) 141 tgt.setUri(src.getUri()); 142 return tgt; 143 } 144 145 public static org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideDependencyComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent src) throws FHIRException { 146 if (src == null || src.isEmpty()) 147 return null; 148 org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideDependencyComponent tgt = new org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideDependencyComponent(); 149 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 150 tgt.setType(org.hl7.fhir.dstu2.model.ImplementationGuide.GuideDependencyType.REFERENCE); 151 if (src.hasUri()) 152 tgt.setUri(src.getUri()); 153 return tgt; 154 } 155 156 public static org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 157 if (src == null || src.isEmpty()) 158 return null; 159 org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 160 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 161 if (src.hasTypeElement()) 162 tgt.setTypeElement(Code10_40.convertCode(src.getTypeElement())); 163 if (src.hasProfileElement()) 164 tgt.setProfile(Canonical10_40.convertCanonicalToReference(src.getProfileElement())); 165 return tgt; 166 } 167 168 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 169 if (src == null || src.isEmpty()) 170 return null; 171 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 172 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 173 if (src.hasTypeElement()) 174 tgt.setTypeElement(Code10_40.convertCode(src.getTypeElement())); 175 if (src.hasProfile()) 176 tgt.setProfileElement(Canonical10_40.convertReferenceToCanonical(src.getProfile())); 177 return tgt; 178 } 179 180 public static org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent src) throws FHIRException { 181 if (src == null || src.isEmpty()) 182 return null; 183 org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent tgt = new org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent(); 184 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 185 tgt.setId(src.getId()); 186 if (src.hasNameElement()) 187 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 188 if (src.hasDescriptionElement()) 189 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 190 return tgt; 191 } 192 193 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionComponent context, org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent src) throws FHIRException { 194 if (src == null || src.isEmpty()) 195 return null; 196 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent(); 197 tgt.setId("p" + (context.getGrouping().size() + 1)); 198 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 199 if (src.hasName()) 200 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 201 if (src.hasDescription()) 202 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 203 for (org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageResourceComponent t : src.getResource()) { 204 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tn = convertImplementationGuidePackageResourceComponent(t); 205 tn.setGroupingId(tgt.getId()); 206 context.addResource(tn); 207 } 208 return tgt; 209 } 210 211 public static org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent src) throws FHIRException { 212 if (src == null || src.isEmpty()) 213 return null; 214 org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageResourceComponent tgt = new org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageResourceComponent(); 215 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 216 if (src.hasExampleCanonicalType()) 217 tgt.setExampleFor(Canonical10_40.convertCanonicalToReference(src.getExampleCanonicalType())); 218 if (src.hasName()) 219 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 220 if (src.hasDescription()) 221 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 222 if (src.hasReference()) 223 tgt.setSource(Reference10_40.convertReference(src.getReference())); 224 return tgt; 225 } 226 227 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageResourceComponent src) throws FHIRException { 228 if (src == null || src.isEmpty()) 229 return null; 230 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent(); 231 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 232 if (src.hasExampleFor()) 233 tgt.setExample(Canonical10_40.convertReferenceToCanonical(src.getExampleFor())); 234 if (src.hasName()) 235 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 236 if (src.hasDescription()) 237 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 238 if (src.hasSourceReference()) 239 tgt.setReference(Reference10_40.convertReference(src.getSourceReference())); 240 else if (src.hasSourceUriType()) 241 tgt.setReference(new org.hl7.fhir.r4.model.Reference(src.getSourceUriType().getValue())); 242 return tgt; 243 } 244 245 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent convertImplementationGuidePageComponent(org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePageComponent src) throws FHIRException { 246 if (src == null || src.isEmpty()) 247 return null; 248 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent(); 249 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 250 if (src.hasSource()) { 251 if (src.hasSourceElement()) 252 tgt.setName(convertUriToUrl(src.getSourceElement())); 253 } 254 if (src.hasNameElement()) 255 tgt.setTitleElement(String10_40.convertString(src.getNameElement())); 256 if (src.hasKind()) 257 tgt.setGeneration(convertPageGeneration(src.getKind())); 258 for (org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePageComponent t : src.getPage()) 259 tgt.addPage(convertImplementationGuidePageComponent(t)); 260 return tgt; 261 } 262 263 public static org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePageComponent convertImplementationGuidePageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent src) throws FHIRException { 264 if (src == null || src.isEmpty()) 265 return null; 266 org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePageComponent tgt = new org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePageComponent(); 267 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 268 if (src.hasNameUrlType()) 269 tgt.setSource(src.getNameUrlType().getValue()); 270 if (src.hasTitleElement()) 271 tgt.setNameElement(String10_40.convertString(src.getTitleElement())); 272 if (src.hasGeneration()) 273 tgt.setKind(convertPageGeneration(src.getGeneration())); 274 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent t : src.getPage()) 275 tgt.addPage(convertImplementationGuidePageComponent(t)); 276 return tgt; 277 } 278 279 static public GuidePageKind convertPageGeneration(GuidePageGeneration generation) { 280 switch (generation) { 281 case HTML: 282 return GuidePageKind.PAGE; 283 default: 284 return GuidePageKind.RESOURCE; 285 } 286 } 287 288 static public GuidePageGeneration convertPageGeneration(GuidePageKind kind) { 289 switch (kind) { 290 case PAGE: 291 return GuidePageGeneration.HTML; 292 default: 293 return GuidePageGeneration.GENERATED; 294 } 295 } 296 297 public static org.hl7.fhir.r4.model.UrlType convertUriToUrl(org.hl7.fhir.dstu2.model.UriType src) throws FHIRException { 298 org.hl7.fhir.r4.model.UrlType tgt = new org.hl7.fhir.r4.model.UrlType(src.getValue()); 299 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 300 return tgt; 301 } 302 303 static public org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent findPackage(List<ImplementationGuide.ImplementationGuidePackageComponent> definition, String id) { 304 if (id != null) 305 for (org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent t : definition) 306 if (id.equals(t.getId())) 307 return t; 308 org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent t = new org.hl7.fhir.dstu2.model.ImplementationGuide.ImplementationGuidePackageComponent(); 309 t.setName("Default Package"); 310 t.setId(id); 311 return t; 312 } 313}