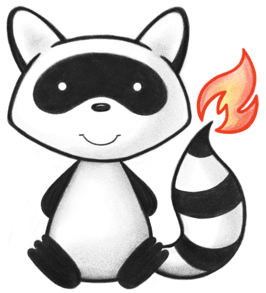
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Extension10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 011import org.hl7.fhir.dstu2.model.Enumeration; 012import org.hl7.fhir.dstu2.model.List_; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r4.model.ListResource; 015 016public class List10_40 { 017 018 public static org.hl7.fhir.r4.model.ListResource convertList(org.hl7.fhir.dstu2.model.List_ src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 org.hl7.fhir.r4.model.ListResource tgt = new org.hl7.fhir.r4.model.ListResource(); 022 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 023 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 024 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 025 if (src.hasTitleElement()) 026 tgt.setTitleElement(String10_40.convertString(src.getTitleElement())); 027 if (src.hasCode()) 028 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 029 if (src.hasSubject()) 030 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 031 if (src.hasSource()) 032 tgt.setSource(Reference10_40.convertReference(src.getSource())); 033 if (src.hasEncounter()) 034 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 035 if (src.hasStatus()) 036 tgt.setStatusElement(convertListStatus(src.getStatusElement())); 037 if (src.hasDate()) 038 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 039 if (src.hasOrderedBy()) 040 tgt.setOrderedBy(CodeableConcept10_40.convertCodeableConcept(src.getOrderedBy())); 041 if (src.hasMode()) 042 tgt.setModeElement(convertListMode(src.getModeElement())); 043 if (src.hasNote()) 044 tgt.addNote(new org.hl7.fhir.r4.model.Annotation().setText(src.getNote())); 045 for (org.hl7.fhir.dstu2.model.List_.ListEntryComponent t : src.getEntry()) tgt.addEntry(convertListEntry(t)); 046 return tgt; 047 } 048 049 public static org.hl7.fhir.dstu2.model.List_ convertList(org.hl7.fhir.r4.model.ListResource src) throws FHIRException { 050 if (src == null || src.isEmpty()) 051 return null; 052 org.hl7.fhir.dstu2.model.List_ tgt = new org.hl7.fhir.dstu2.model.List_(); 053 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 056 if (src.hasTitleElement()) 057 tgt.setTitleElement(String10_40.convertString(src.getTitleElement())); 058 if (src.hasCode()) 059 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 060 if (src.hasSubject()) 061 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 062 if (src.hasSource()) 063 tgt.setSource(Reference10_40.convertReference(src.getSource())); 064 if (src.hasEncounter()) 065 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 066 if (src.hasStatus()) 067 tgt.setStatusElement(convertListStatus(src.getStatusElement())); 068 if (src.hasDate()) 069 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 070 if (src.hasOrderedBy()) 071 tgt.setOrderedBy(CodeableConcept10_40.convertCodeableConcept(src.getOrderedBy())); 072 if (src.hasMode()) 073 tgt.setModeElement(convertListMode(src.getModeElement())); 074 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.setNote(t.getText()); 075 for (org.hl7.fhir.r4.model.ListResource.ListEntryComponent t : src.getEntry()) tgt.addEntry(convertListEntry(t)); 076 return tgt; 077 } 078 079 public static org.hl7.fhir.r4.model.ListResource.ListEntryComponent convertListEntry(org.hl7.fhir.dstu2.model.List_.ListEntryComponent src) throws FHIRException { 080 if (src == null || src.isEmpty()) 081 return null; 082 org.hl7.fhir.r4.model.ListResource.ListEntryComponent tgt = new org.hl7.fhir.r4.model.ListResource.ListEntryComponent(); 083 copyBackboneElement(src, tgt); 084 if (src.hasFlag()) 085 tgt.setFlag(CodeableConcept10_40.convertCodeableConcept(src.getFlag())); 086 if (src.hasDeletedElement()) 087 tgt.setDeletedElement(Boolean10_40.convertBoolean(src.getDeletedElement())); 088 if (src.hasDate()) 089 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 090 if (src.hasItem()) 091 tgt.setItem(Reference10_40.convertReference(src.getItem())); 092 return tgt; 093 } 094 095 public static org.hl7.fhir.dstu2.model.List_.ListEntryComponent convertListEntry(org.hl7.fhir.r4.model.ListResource.ListEntryComponent src) throws FHIRException { 096 if (src == null || src.isEmpty()) 097 return null; 098 org.hl7.fhir.dstu2.model.List_.ListEntryComponent tgt = new org.hl7.fhir.dstu2.model.List_.ListEntryComponent(); 099 copyBackboneElement(src, tgt); 100 if (src.hasFlag()) 101 tgt.setFlag(CodeableConcept10_40.convertCodeableConcept(src.getFlag())); 102 if (src.hasDeletedElement()) 103 tgt.setDeletedElement(Boolean10_40.convertBoolean(src.getDeletedElement())); 104 if (src.hasDate()) 105 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 106 if (src.hasItem()) 107 tgt.setItem(Reference10_40.convertReference(src.getItem())); 108 return tgt; 109 } 110 111 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListMode> convertListMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ListResource.ListMode> src) throws FHIRException { 112 if (src == null || src.isEmpty()) 113 return null; 114 Enumeration<List_.ListMode> tgt = new Enumeration<>(new List_.ListModeEnumFactory()); 115 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 116 if (src.getValue() == null) { 117 tgt.setValue(null); 118 } else { 119 switch (src.getValue()) { 120 case WORKING: 121 tgt.setValue(List_.ListMode.WORKING); 122 break; 123 case SNAPSHOT: 124 tgt.setValue(List_.ListMode.SNAPSHOT); 125 break; 126 case CHANGES: 127 tgt.setValue(List_.ListMode.CHANGES); 128 break; 129 default: 130 tgt.setValue(List_.ListMode.NULL); 131 break; 132 } 133 } 134 return tgt; 135 } 136 137 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ListResource.ListMode> convertListMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListMode> src) throws FHIRException { 138 if (src == null || src.isEmpty()) 139 return null; 140 org.hl7.fhir.r4.model.Enumeration<ListResource.ListMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ListResource.ListModeEnumFactory()); 141 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 142 if (src.getValue() == null) { 143 tgt.setValue(null); 144 } else { 145 switch (src.getValue()) { 146 case WORKING: 147 tgt.setValue(ListResource.ListMode.WORKING); 148 break; 149 case SNAPSHOT: 150 tgt.setValue(ListResource.ListMode.SNAPSHOT); 151 break; 152 case CHANGES: 153 tgt.setValue(ListResource.ListMode.CHANGES); 154 break; 155 default: 156 tgt.setValue(ListResource.ListMode.NULL); 157 break; 158 } 159 } 160 return tgt; 161 } 162 163 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ListResource.ListStatus> convertListStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListStatus> src) throws FHIRException { 164 if (src == null || src.isEmpty()) 165 return null; 166 org.hl7.fhir.r4.model.Enumeration<ListResource.ListStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ListResource.ListStatusEnumFactory()); 167 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 168 if (src.getValue() == null) { 169 tgt.setValue(null); 170 } else { 171 switch (src.getValue()) { 172 case CURRENT: 173 tgt.setValue(ListResource.ListStatus.CURRENT); 174 break; 175 case RETIRED: 176 tgt.setValue(ListResource.ListStatus.RETIRED); 177 break; 178 case ENTEREDINERROR: 179 tgt.setValue(ListResource.ListStatus.ENTEREDINERROR); 180 break; 181 default: 182 tgt.setValue(ListResource.ListStatus.NULL); 183 break; 184 } 185 } 186 return tgt; 187 } 188 189 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListStatus> convertListStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ListResource.ListStatus> src) throws FHIRException { 190 if (src == null || src.isEmpty()) 191 return null; 192 Enumeration<List_.ListStatus> tgt = new Enumeration<>(new List_.ListStatusEnumFactory()); 193 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 194 if (src.getValue() == null) { 195 tgt.setValue(null); 196 } else { 197 switch (src.getValue()) { 198 case CURRENT: 199 tgt.setValue(List_.ListStatus.CURRENT); 200 break; 201 case RETIRED: 202 tgt.setValue(List_.ListStatus.RETIRED); 203 break; 204 case ENTEREDINERROR: 205 tgt.setValue(List_.ListStatus.ENTEREDINERROR); 206 break; 207 default: 208 tgt.setValue(List_.ListStatus.NULL); 209 break; 210 } 211 } 212 return tgt; 213 } 214 215 public static void copyBackboneElement(org.hl7.fhir.r4.model.BackboneElement src, org.hl7.fhir.dstu2.model.BackboneElement tgt) throws FHIRException { 216 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 217 for (org.hl7.fhir.r4.model.Extension e : src.getModifierExtension()) { 218 tgt.addModifierExtension(Extension10_40.convertExtension(e)); 219 } 220 } 221 222 public static void copyBackboneElement(org.hl7.fhir.dstu2.model.BackboneElement src, org.hl7.fhir.r4.model.BackboneElement tgt) throws FHIRException { 223 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 224 for (org.hl7.fhir.dstu2.model.Extension e : src.getModifierExtension()) { 225 tgt.addModifierExtension(Extension10_40.convertExtension(e)); 226 } 227 } 228}