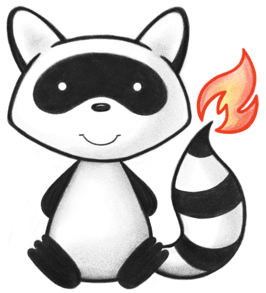
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Ratio10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.SimpleQuantity10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 010import org.hl7.fhir.dstu2.model.Medication; 011import org.hl7.fhir.dstu2.model.Medication.MedicationPackageContentComponent; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.Extension; 014 015public class Medication10_40 { 016 017 public static org.hl7.fhir.r4.model.Medication convertMedication(org.hl7.fhir.dstu2.model.Medication src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.r4.model.Medication tgt = new org.hl7.fhir.r4.model.Medication(); 021 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 022 if (src.hasCode()) 023 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 024 if (src.hasIsBrandElement()) 025 tgt.addExtension( 026 VersionConvertorConstants.EXT_MED_ISBRAND, 027 Boolean10_40.convertBoolean(src.getIsBrandElement()) 028 ); 029 if (src.hasManufacturer()) 030 tgt.setManufacturer(Reference10_40.convertReference(src.getManufacturer())); 031 if (src.hasProduct()) { 032 if (src.getProduct().hasForm()) 033 tgt.setForm(CodeableConcept10_40.convertCodeableConcept(src.getProduct().getForm())); 034 for (org.hl7.fhir.dstu2.model.Medication.MedicationProductIngredientComponent ingridient : src.getProduct().getIngredient()) 035 tgt.addIngredient(convertMedicationIngridient(ingridient)); 036 if (src.getProduct().hasBatch()) 037 tgt.setBatch(batch(src.getProduct().getBatch().get(0))); 038 } 039 if (src.hasPackage()) { 040 org.hl7.fhir.dstu2.model.Medication.MedicationPackageComponent package_ = src.getPackage(); 041 if (package_.hasContainer()) 042 tgt.addExtension( 043 VersionConvertorConstants.EXT_MED_CONT, 044 CodeableConcept10_40.convertCodeableConcept(package_.getContainer()) 045 ); 046 for (org.hl7.fhir.dstu2.model.Medication.MedicationPackageContentComponent c : package_.getContent()) 047 tgt.addExtension( 048 VersionConvertorConstants.EXT_MED_PACK_CONT, 049 Medication10_40.content(c) 050 ); 051 } 052 return tgt; 053 } 054 055 private static org.hl7.fhir.r4.model.Medication.MedicationBatchComponent batch(org.hl7.fhir.dstu2.model.Medication.MedicationProductBatchComponent src) { 056 if (src == null || src.isEmpty()) 057 return null; 058 org.hl7.fhir.r4.model.Medication.MedicationBatchComponent tgt = new org.hl7.fhir.r4.model.Medication.MedicationBatchComponent(); 059 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 060 if (src.hasLotNumber()) 061 tgt.setLotNumber(src.getLotNumber()); 062 if (src.hasExpirationDate()) 063 tgt.setExpirationDate(src.getExpirationDate()); 064 return tgt; 065 } 066 067 private static org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent convertMedicationIngridient(org.hl7.fhir.dstu2.model.Medication.MedicationProductIngredientComponent src) { 068 if (src == null || src.isEmpty()) 069 return null; 070 org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent tgt = new org.hl7.fhir.r4.model.Medication.MedicationIngredientComponent(); 071 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 072 if (src.hasItem()) 073 tgt.setItem(Reference10_40.convertReference(src.getItem())); 074 if (src.hasAmount()) 075 tgt.setStrength(Ratio10_40.convertRatio(src.getAmount())); 076 return tgt; 077 } 078 079 public static org.hl7.fhir.r4.model.Extension content(org.hl7.fhir.dstu2.model.Medication.MedicationPackageContentComponent src) { 080 if (src == null || src.isEmpty()) 081 return null; 082 org.hl7.fhir.r4.model.Extension tgt = new org.hl7.fhir.r4.model.Extension(); 083 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 084 if (src.hasItem()) 085 tgt.addExtension( 086 VersionConvertorConstants.EXT_MED_PACK_CONT, 087 Reference10_40.convertReference(src.getItem()) 088 ); 089 if (src.hasAmount()) 090 tgt.addExtension( 091 VersionConvertorConstants.EXT_MED_PACK_AMOUNT, 092 SimpleQuantity10_40.convertSimpleQuantity(src.getAmount()) 093 ); 094 return tgt; 095 } 096}