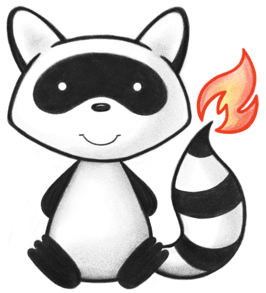
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Ratio10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Timing10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r4.model.Dosage.DosageDoseAndRateComponent; 013import org.hl7.fhir.r4.model.Enumeration; 014import org.hl7.fhir.r4.model.MedicationStatement; 015 016public class MedicationStatement10_40 { 017 018 public static org.hl7.fhir.dstu2.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.r4.model.MedicationStatement src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 org.hl7.fhir.dstu2.model.MedicationStatement tgt = new org.hl7.fhir.dstu2.model.MedicationStatement(); 022 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 023 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 024 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 025 if (src.hasStatus()) 026 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 027 if (src.hasMedication()) 028 tgt.setMedication(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getMedication())); 029 if (src.hasSubject()) 030 tgt.setPatient(Reference10_40.convertReference(src.getSubject())); 031 if (src.hasEffective()) 032 tgt.setEffective(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getEffective())); 033 if (src.hasInformationSource()) 034 tgt.setInformationSource(Reference10_40.convertReference(src.getInformationSource())); 035 for (org.hl7.fhir.r4.model.Reference t : src.getDerivedFrom()) 036 tgt.addSupportingInformation(Reference10_40.convertReference(t)); 037 if (src.hasDateAsserted()) 038 tgt.setDateAssertedElement(DateTime10_40.convertDateTime(src.getDateAssertedElement())); 039 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.setNote(t.getText()); 040 for (org.hl7.fhir.r4.model.Dosage t : src.getDosage()) tgt.addDosage(convertMedicationStatementDosageComponent(t)); 041 return tgt; 042 } 043 044 public static org.hl7.fhir.r4.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.dstu2.model.MedicationStatement src) throws FHIRException { 045 if (src == null || src.isEmpty()) 046 return null; 047 org.hl7.fhir.r4.model.MedicationStatement tgt = new org.hl7.fhir.r4.model.MedicationStatement(); 048 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 049 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 050 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 051 if (src.hasStatus()) 052 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 053 if (src.hasMedication()) 054 tgt.setMedication(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getMedication())); 055 if (src.hasPatient()) 056 tgt.setSubject(Reference10_40.convertReference(src.getPatient())); 057 if (src.hasEffective()) 058 tgt.setEffective(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getEffective())); 059 if (src.hasInformationSource()) 060 tgt.setInformationSource(Reference10_40.convertReference(src.getInformationSource())); 061 for (org.hl7.fhir.dstu2.model.Reference t : src.getSupportingInformation()) 062 tgt.addDerivedFrom(Reference10_40.convertReference(t)); 063 if (src.hasDateAsserted()) 064 tgt.setDateAssertedElement(DateTime10_40.convertDateTime(src.getDateAssertedElement())); 065 if (src.hasNote()) 066 tgt.addNote().setText(src.getNote()); 067 for (org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent t : src.getDosage()) 068 tgt.addDosage(convertMedicationStatementDosageComponent(t)); 069 return tgt; 070 } 071 072 public static org.hl7.fhir.r4.model.Dosage convertMedicationStatementDosageComponent(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent src) throws FHIRException { 073 if (src == null || src.isEmpty()) 074 return null; 075 org.hl7.fhir.r4.model.Dosage tgt = new org.hl7.fhir.r4.model.Dosage(); 076 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 077 if (src.hasTextElement()) 078 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 079 if (src.hasTiming()) 080 tgt.setTiming(Timing10_40.convertTiming(src.getTiming())); 081 if (src.hasAsNeeded()) 082 tgt.setAsNeeded(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getAsNeeded())); 083 if (src.hasSiteCodeableConcept()) 084 tgt.setSite(CodeableConcept10_40.convertCodeableConcept(src.getSiteCodeableConcept())); 085 if (src.hasRoute()) 086 tgt.setRoute(CodeableConcept10_40.convertCodeableConcept(src.getRoute())); 087 if (src.hasMethod()) 088 tgt.setMethod(CodeableConcept10_40.convertCodeableConcept(src.getMethod())); 089 if (src.hasRate()) { 090 DosageDoseAndRateComponent dr = tgt.addDoseAndRate(); 091 if (src.hasRate()) 092 dr.setRate(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getRate())); 093 } 094 if (src.hasMaxDosePerPeriod()) 095 tgt.setMaxDosePerPeriod(Ratio10_40.convertRatio(src.getMaxDosePerPeriod())); 096 return tgt; 097 } 098 099 public static org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent convertMedicationStatementDosageComponent(org.hl7.fhir.r4.model.Dosage src) throws FHIRException { 100 if (src == null || src.isEmpty()) 101 return null; 102 org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent tgt = new org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent(); 103 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 104 if (src.hasTextElement()) 105 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 106 if (src.hasTiming()) 107 tgt.setTiming(Timing10_40.convertTiming(src.getTiming())); 108 if (src.hasAsNeeded()) 109 tgt.setAsNeeded(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getAsNeeded())); 110 if (src.hasSite()) 111 tgt.setSite(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getSite())); 112 if (src.hasRoute()) 113 tgt.setRoute(CodeableConcept10_40.convertCodeableConcept(src.getRoute())); 114 if (src.hasMethod()) 115 tgt.setMethod(CodeableConcept10_40.convertCodeableConcept(src.getMethod())); 116 if (src.hasDoseAndRate() && src.getDoseAndRate().get(0).hasRate()) 117 tgt.setRate(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getDoseAndRate().get(0).getRate())); 118 if (src.hasMaxDosePerPeriod()) 119 tgt.setMaxDosePerPeriod(Ratio10_40.convertRatio(src.getMaxDosePerPeriod())); 120 return tgt; 121 } 122 123 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus> convertMedicationStatementStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> src) throws FHIRException { 124 if (src == null || src.isEmpty()) 125 return null; 126 Enumeration<MedicationStatement.MedicationStatementStatus> tgt = new Enumeration<>(new MedicationStatement.MedicationStatementStatusEnumFactory()); 127 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 128 if (src.getValue() == null) { 129 tgt.setValue(null); 130 } else { 131 switch (src.getValue()) { 132 case ACTIVE: 133 tgt.setValue(MedicationStatement.MedicationStatementStatus.ACTIVE); 134 break; 135 case COMPLETED: 136 tgt.setValue(MedicationStatement.MedicationStatementStatus.COMPLETED); 137 break; 138 case ENTEREDINERROR: 139 tgt.setValue(MedicationStatement.MedicationStatementStatus.ENTEREDINERROR); 140 break; 141 case INTENDED: 142 tgt.setValue(MedicationStatement.MedicationStatementStatus.INTENDED); 143 break; 144 default: 145 tgt.setValue(MedicationStatement.MedicationStatementStatus.NULL); 146 break; 147 } 148 } 149 return tgt; 150 } 151 152 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> convertMedicationStatementStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus> src) throws FHIRException { 153 if (src == null || src.isEmpty()) 154 return null; 155 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatusEnumFactory()); 156 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 157 if (src.getValue() == null) { 158 tgt.setValue(null); 159 } else { 160 switch (src.getValue()) { 161 case ACTIVE: 162 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.ACTIVE); 163 break; 164 case COMPLETED: 165 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.COMPLETED); 166 break; 167 case ENTEREDINERROR: 168 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.ENTEREDINERROR); 169 break; 170 case INTENDED: 171 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.INTENDED); 172 break; 173 default: 174 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.NULL); 175 break; 176 } 177 } 178 return tgt; 179 } 180}