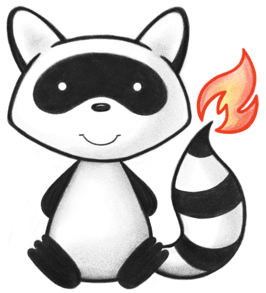
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_40; 004import org.hl7.fhir.convertors.context.ConversionContext10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Canonical10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 012import org.hl7.fhir.dstu2.model.Enumeration; 013import org.hl7.fhir.dstu2.model.Questionnaire; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r4.model.ContactDetail; 016import org.hl7.fhir.r4.model.Enumerations; 017import org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent; 018 019public class Questionnaire10_40 { 020 021 public static org.hl7.fhir.dstu2.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r4.model.Questionnaire src, BaseAdvisor_10_40 advisor) { 022 if (src == null || src.isEmpty()) 023 return null; 024 org.hl7.fhir.dstu2.model.Questionnaire tgt = new org.hl7.fhir.dstu2.model.Questionnaire(); 025 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 026 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 027 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 028 if (src.hasVersionElement()) 029 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 030 if (src.hasStatus()) 031 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 032 if (src.hasDate()) 033 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 034 if (src.hasPublisherElement()) 035 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 036 for (ContactDetail t : src.getContact()) 037 for (org.hl7.fhir.r4.model.ContactPoint t1 : t.getTelecom()) 038 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t1)); 039 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent root = tgt.getGroup(); 040 root.setTitle(src.getTitle()); 041 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) { 042 root.addConcept(Coding10_40.convertCoding(t)); 043 } 044 for (org.hl7.fhir.r4.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 045 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 046 if (t.getType() == org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.GROUP) 047 root.addGroup(convertQuestionnaireGroupComponent(t)); 048 else 049 root.addQuestion(convertQuestionnaireQuestionComponent(t)); 050 return tgt; 051 } 052 053 public static org.hl7.fhir.r4.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu2.model.Questionnaire src) throws FHIRException { 054 return convertQuestionnaire(src, null); 055 } 056 057 public static org.hl7.fhir.r4.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu2.model.Questionnaire src, BaseAdvisor_10_40 advisor) throws FHIRException { 058 if (src == null || src.isEmpty()) 059 return null; 060 org.hl7.fhir.r4.model.Questionnaire tgt = new org.hl7.fhir.r4.model.Questionnaire(); 061 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 062 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 063 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 064 if (src.hasVersionElement()) 065 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 066 if (src.hasStatus()) 067 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 068 if (src.hasDate()) 069 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 070 if (src.hasPublisherElement()) 071 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 072 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 073 tgt.addContact(convertQuestionnaireContactComponent(t)); 074 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent root = src.getGroup(); 075 tgt.setTitle(root.getTitle()); 076 for (org.hl7.fhir.dstu2.model.Coding t : root.getConcept()) tgt.addCode(Coding10_40.convertCoding(t)); 077 for (org.hl7.fhir.dstu2.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 078 tgt.addItem(convertQuestionnaireGroupComponent(root)); 079 return tgt; 080 } 081 082 public static org.hl7.fhir.r4.model.ContactDetail convertQuestionnaireContactComponent(org.hl7.fhir.dstu2.model.ContactPoint src) throws FHIRException { 083 if (src == null || src.isEmpty()) 084 return null; 085 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 086 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 087 tgt.addTelecom(ContactPoint10_40.convertContactPoint(src)); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent convertQuestionnaireGroupComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent tgt = new org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent(); 095 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 096 if (src.hasLinkIdElement()) 097 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 098 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addConcept(Coding10_40.convertCoding(t)); 099 if (src.hasTextElement()) 100 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 101 if (src.hasRequiredElement()) 102 tgt.setRequiredElement(Boolean10_40.convertBoolean(src.getRequiredElement())); 103 if (src.hasRepeatsElement()) 104 tgt.setRepeatsElement(Boolean10_40.convertBoolean(src.getRepeatsElement())); 105 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 106 if (t.getType() == org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.GROUP) 107 tgt.addGroup(convertQuestionnaireGroupComponent(t)); 108 else 109 tgt.addQuestion(convertQuestionnaireQuestionComponent(t)); 110 return tgt; 111 } 112 113 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireGroupComponent(org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent src) throws FHIRException { 114 if (src == null || src.isEmpty()) 115 return null; 116 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent(); 117 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 118 if (src.hasLinkIdElement()) 119 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 120 for (org.hl7.fhir.dstu2.model.Coding t : src.getConcept()) tgt.addCode(Coding10_40.convertCoding(t)); 121 if (src.hasTextElement()) 122 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 123 tgt.setType(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.GROUP); 124 if (src.hasRequiredElement()) 125 tgt.setRequiredElement(Boolean10_40.convertBoolean(src.getRequiredElement())); 126 if (src.hasRepeatsElement()) 127 tgt.setRepeatsElement(Boolean10_40.convertBoolean(src.getRepeatsElement())); 128 for (org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent t : src.getGroup()) 129 tgt.addItem(convertQuestionnaireGroupComponent(t)); 130 for (org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent t : src.getQuestion()) 131 tgt.addItem(convertQuestionnaireQuestionComponent(t)); 132 return tgt; 133 } 134 135 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat> convertQuestionnaireItemType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 Enumeration<Questionnaire.AnswerFormat> tgt = new Enumeration<>(new Questionnaire.AnswerFormatEnumFactory()); 139 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 140 if (src.getValue() == null) { 141 tgt.setValue(null); 142 } else { 143 switch (src.getValue()) { 144 case BOOLEAN: 145 tgt.setValue(Questionnaire.AnswerFormat.BOOLEAN); 146 break; 147 case DECIMAL: 148 tgt.setValue(Questionnaire.AnswerFormat.DECIMAL); 149 break; 150 case INTEGER: 151 tgt.setValue(Questionnaire.AnswerFormat.INTEGER); 152 break; 153 case DATE: 154 tgt.setValue(Questionnaire.AnswerFormat.DATE); 155 break; 156 case DATETIME: 157 tgt.setValue(Questionnaire.AnswerFormat.DATETIME); 158 break; 159 case TIME: 160 tgt.setValue(Questionnaire.AnswerFormat.TIME); 161 break; 162 case STRING: 163 tgt.setValue(Questionnaire.AnswerFormat.STRING); 164 break; 165 case TEXT: 166 tgt.setValue(Questionnaire.AnswerFormat.TEXT); 167 break; 168 case URL: 169 tgt.setValue(Questionnaire.AnswerFormat.URL); 170 break; 171 case CHOICE: 172 tgt.setValue(Questionnaire.AnswerFormat.CHOICE); 173 break; 174 case OPENCHOICE: 175 tgt.setValue(Questionnaire.AnswerFormat.OPENCHOICE); 176 break; 177 case ATTACHMENT: 178 tgt.setValue(Questionnaire.AnswerFormat.ATTACHMENT); 179 break; 180 case REFERENCE: 181 tgt.setValue(Questionnaire.AnswerFormat.REFERENCE); 182 break; 183 case QUANTITY: 184 tgt.setValue(Questionnaire.AnswerFormat.QUANTITY); 185 break; 186 default: 187 tgt.setValue(Questionnaire.AnswerFormat.NULL); 188 break; 189 } 190 } 191 return tgt; 192 } 193 194 public static org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent convertQuestionnaireQuestionComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 195 if (src == null || src.isEmpty()) 196 return null; 197 org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent tgt = new org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent(); 198 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 199 if (src.hasLinkIdElement()) 200 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 201 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addConcept(Coding10_40.convertCoding(t)); 202 if (src.hasTextElement()) 203 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 204 if (src.hasType()) 205 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 206 if (src.hasRequiredElement()) 207 tgt.setRequiredElement(Boolean10_40.convertBoolean(src.getRequiredElement())); 208 if (src.hasRepeatsElement()) 209 tgt.setRepeatsElement(Boolean10_40.convertBoolean(src.getRepeatsElement())); 210 if (src.hasAnswerValueSetElement()) 211 tgt.setOptions(Canonical10_40.convertCanonicalToReference(src.getAnswerValueSetElement())); 212 for (QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 213 if (t.hasValueCoding()) 214 try { 215 tgt.addOption(Coding10_40.convertCoding(t.getValueCoding())); 216 } catch (org.hl7.fhir.exceptions.FHIRException e) { 217 throw new FHIRException(e); 218 } 219 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 220 tgt.addGroup(convertQuestionnaireGroupComponent(t)); 221 return tgt; 222 } 223 224 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireQuestionComponent(org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent src) throws FHIRException { 225 if (src == null || src.isEmpty()) 226 return null; 227 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent(); 228 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 229 if (src.hasLinkIdElement()) 230 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 231 for (org.hl7.fhir.dstu2.model.Coding t : src.getConcept()) tgt.addCode(Coding10_40.convertCoding(t)); 232 if (src.hasTextElement()) 233 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 234 if (src.hasType()) 235 tgt.setTypeElement(convertQuestionnaireQuestionType(src.getTypeElement())); 236 if (src.hasRequiredElement()) 237 tgt.setRequiredElement(Boolean10_40.convertBoolean(src.getRequiredElement())); 238 if (src.hasRepeatsElement()) 239 tgt.setRepeatsElement(Boolean10_40.convertBoolean(src.getRepeatsElement())); 240 if (src.hasOptions()) 241 tgt.setAnswerValueSetElement(Canonical10_40.convertReferenceToCanonical(src.getOptions())); 242 for (org.hl7.fhir.dstu2.model.Coding t : src.getOption()) 243 tgt.addAnswerOption().setValue(Coding10_40.convertCoding(t)); 244 for (org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent t : src.getGroup()) 245 tgt.addItem(convertQuestionnaireGroupComponent(t)); 246 return tgt; 247 } 248 249 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireQuestionType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat> src) throws FHIRException { 250 if (src == null || src.isEmpty()) 251 return null; 252 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 253 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 254 if (src.getValue() == null) { 255 tgt.setValue(null); 256 } else { 257 switch (src.getValue()) { 258 case BOOLEAN: 259 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.BOOLEAN); 260 break; 261 case DECIMAL: 262 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.DECIMAL); 263 break; 264 case INTEGER: 265 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.INTEGER); 266 break; 267 case DATE: 268 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.DATE); 269 break; 270 case DATETIME: 271 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.DATETIME); 272 break; 273 case INSTANT: 274 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.DATETIME); 275 break; 276 case TIME: 277 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.TIME); 278 break; 279 case STRING: 280 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.STRING); 281 break; 282 case TEXT: 283 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.TEXT); 284 break; 285 case URL: 286 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.URL); 287 break; 288 case CHOICE: 289 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.CHOICE); 290 break; 291 case OPENCHOICE: 292 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.OPENCHOICE); 293 break; 294 case ATTACHMENT: 295 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.ATTACHMENT); 296 break; 297 case REFERENCE: 298 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.REFERENCE); 299 break; 300 case QUANTITY: 301 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.QUANTITY); 302 break; 303 default: 304 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.NULL); 305 break; 306 } 307 } 308 return tgt; 309 } 310 311 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus> convertQuestionnaireStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> src) throws FHIRException { 312 if (src == null || src.isEmpty()) 313 return null; 314 Enumeration<Questionnaire.QuestionnaireStatus> tgt = new Enumeration<>(new Questionnaire.QuestionnaireStatusEnumFactory()); 315 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 316 if (src.getValue() == null) { 317 tgt.setValue(null); 318 } else { 319 switch (src.getValue()) { 320 case DRAFT: 321 tgt.setValue(Questionnaire.QuestionnaireStatus.DRAFT); 322 break; 323 case ACTIVE: 324 tgt.setValue(Questionnaire.QuestionnaireStatus.PUBLISHED); 325 break; 326 case RETIRED: 327 tgt.setValue(Questionnaire.QuestionnaireStatus.RETIRED); 328 break; 329 default: 330 tgt.setValue(Questionnaire.QuestionnaireStatus.NULL); 331 break; 332 } 333 } 334 return tgt; 335 } 336 337 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus> src) throws FHIRException { 338 if (src == null || src.isEmpty()) 339 return null; 340 org.hl7.fhir.r4.model.Enumeration<Enumerations.PublicationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new Enumerations.PublicationStatusEnumFactory()); 341 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 342 if (src.getValue() == null) { 343 tgt.setValue(null); 344 } else { 345 switch (src.getValue()) { 346 case DRAFT: 347 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 348 break; 349 case PUBLISHED: 350 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 351 break; 352 case RETIRED: 353 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 354 break; 355 default: 356 tgt.setValue(Enumerations.PublicationStatus.NULL); 357 break; 358 } 359 } 360 return tgt; 361 } 362}