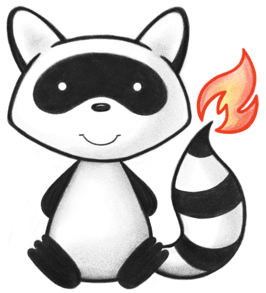
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Canonical10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r4.model.Enumeration; 011import org.hl7.fhir.r4.model.QuestionnaireResponse; 012 013public class QuestionnaireResponse10_40 { 014 015 public static org.hl7.fhir.dstu2.model.QuestionnaireResponse.GroupComponent convertQuestionnaireItemToGroup(org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 org.hl7.fhir.dstu2.model.QuestionnaireResponse.GroupComponent tgt = new org.hl7.fhir.dstu2.model.QuestionnaireResponse.GroupComponent(); 019 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 020 if (src.hasLinkIdElement()) 021 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 022 if (src.hasTextElement()) 023 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 024 for (org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent t : src.getItem()) 025 if (t.hasAnswer()) 026 tgt.addQuestion(convertQuestionnaireItemToQuestion(t)); 027 else 028 tgt.addGroup(convertQuestionnaireItemToGroup(t)); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionComponent convertQuestionnaireItemToQuestion(org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent src) throws FHIRException { 033 if (src == null || src.isEmpty()) 034 return null; 035 org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionComponent tgt = new org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionComponent(); 036 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 037 if (src.hasLinkIdElement()) 038 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 039 if (src.hasTextElement()) 040 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 041 for (org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent t : src.getAnswer()) 042 tgt.addAnswer(convertQuestionnaireResponseItemAnswerComponent(t)); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.dstu2.model.QuestionnaireResponse convertQuestionnaireResponse(org.hl7.fhir.r4.model.QuestionnaireResponse src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.dstu2.model.QuestionnaireResponse tgt = new org.hl7.fhir.dstu2.model.QuestionnaireResponse(); 050 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 051 if (src.hasIdentifier()) 052 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 053 if (src.hasQuestionnaireElement()) 054 tgt.setQuestionnaire(Canonical10_40.convertCanonicalToReference(src.getQuestionnaireElement())); 055 if (src.hasStatus()) 056 tgt.setStatusElement(convertQuestionnaireResponseStatus(src.getStatusElement())); 057 if (src.hasSubject()) 058 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 059 if (src.hasAuthor()) 060 tgt.setAuthor(Reference10_40.convertReference(src.getAuthor())); 061 if (src.hasAuthoredElement()) 062 tgt.setAuthoredElement(DateTime10_40.convertDateTime(src.getAuthoredElement())); 063 if (src.hasSource()) 064 tgt.setSource(Reference10_40.convertReference(src.getSource())); 065 if (src.hasEncounter()) 066 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 067 if (src.getItem().size() != 1) 068 throw new FHIRException("multiple root items not supported"); 069 tgt.setGroup(convertQuestionnaireItemToGroup(src.getItem().get(0))); 070 return tgt; 071 } 072 073 public static org.hl7.fhir.r4.model.QuestionnaireResponse convertQuestionnaireResponse(org.hl7.fhir.dstu2.model.QuestionnaireResponse src) throws FHIRException { 074 if (src == null || src.isEmpty()) 075 return null; 076 org.hl7.fhir.r4.model.QuestionnaireResponse tgt = new org.hl7.fhir.r4.model.QuestionnaireResponse(); 077 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 078 if (src.hasIdentifier()) 079 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 080 if (src.hasQuestionnaire()) 081 tgt.setQuestionnaireElement(Canonical10_40.convertReferenceToCanonical(src.getQuestionnaire())); 082 if (src.hasStatus()) 083 tgt.setStatusElement(convertQuestionnaireResponseStatus(src.getStatusElement())); 084 if (src.hasSubject()) 085 tgt.setSubject(Reference10_40.convertReference(src.getSubject())); 086 if (src.hasAuthor()) 087 tgt.setAuthor(Reference10_40.convertReference(src.getAuthor())); 088 if (src.hasAuthoredElement()) 089 tgt.setAuthoredElement(DateTime10_40.convertDateTime(src.getAuthoredElement())); 090 if (src.hasSource()) 091 tgt.setSource(Reference10_40.convertReference(src.getSource())); 092 if (src.hasEncounter()) 093 tgt.setEncounter(Reference10_40.convertReference(src.getEncounter())); 094 if (src.hasGroup()) 095 tgt.addItem(convertQuestionnaireResponseGroupComponent(src.getGroup())); 096 return tgt; 097 } 098 099 public static org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent convertQuestionnaireResponseGroupComponent(org.hl7.fhir.dstu2.model.QuestionnaireResponse.GroupComponent src) throws FHIRException { 100 if (src == null || src.isEmpty()) 101 return null; 102 org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent tgt = new org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent(); 103 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 104 if (src.hasLinkIdElement()) 105 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 106 if (src.hasTextElement()) 107 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 108 for (org.hl7.fhir.dstu2.model.QuestionnaireResponse.GroupComponent t : src.getGroup()) 109 tgt.addItem(convertQuestionnaireResponseGroupComponent(t)); 110 for (org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionComponent t : src.getQuestion()) 111 tgt.addItem(convertQuestionnaireResponseQuestionComponent(t)); 112 return tgt; 113 } 114 115 public static org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent convertQuestionnaireResponseItemAnswerComponent(org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionAnswerComponent src) throws FHIRException { 116 if (src == null || src.isEmpty()) 117 return null; 118 org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent tgt = new org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent(); 119 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 120 if (src.hasValue()) 121 tgt.setValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getValue())); 122 for (org.hl7.fhir.dstu2.model.QuestionnaireResponse.GroupComponent t : src.getGroup()) 123 tgt.addItem(convertQuestionnaireResponseGroupComponent(t)); 124 return tgt; 125 } 126 127 public static org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionAnswerComponent convertQuestionnaireResponseItemAnswerComponent(org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemAnswerComponent src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionAnswerComponent tgt = new org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionAnswerComponent(); 131 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 132 if (src.hasValue()) 133 tgt.setValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getValue())); 134 for (org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent t : src.getItem()) 135 tgt.addGroup(convertQuestionnaireItemToGroup(t)); 136 return tgt; 137 } 138 139 public static org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent convertQuestionnaireResponseQuestionComponent(org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionComponent src) throws FHIRException { 140 if (src == null || src.isEmpty()) 141 return null; 142 org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent tgt = new org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseItemComponent(); 143 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 144 if (src.hasLinkIdElement()) 145 tgt.setLinkIdElement(String10_40.convertString(src.getLinkIdElement())); 146 if (src.hasTextElement()) 147 tgt.setTextElement(String10_40.convertString(src.getTextElement())); 148 for (org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionAnswerComponent t : src.getAnswer()) 149 tgt.addAnswer(convertQuestionnaireResponseItemAnswerComponent(t)); 150 return tgt; 151 } 152 153 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseStatus> convertQuestionnaireResponseStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatus> src) throws FHIRException { 154 if (src == null || src.isEmpty()) 155 return null; 156 Enumeration<QuestionnaireResponse.QuestionnaireResponseStatus> tgt = new Enumeration<>(new QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory()); 157 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 158 if (src.getValue() == null) { 159 tgt.setValue(null); 160 } else { 161 switch (src.getValue()) { 162 case INPROGRESS: 163 tgt.setValue(QuestionnaireResponse.QuestionnaireResponseStatus.INPROGRESS); 164 break; 165 case COMPLETED: 166 tgt.setValue(QuestionnaireResponse.QuestionnaireResponseStatus.COMPLETED); 167 break; 168 case AMENDED: 169 tgt.setValue(QuestionnaireResponse.QuestionnaireResponseStatus.AMENDED); 170 break; 171 default: 172 tgt.setValue(QuestionnaireResponse.QuestionnaireResponseStatus.NULL); 173 break; 174 } 175 } 176 return tgt; 177 } 178 179 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatus> convertQuestionnaireResponseStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.QuestionnaireResponse.QuestionnaireResponseStatus> src) throws FHIRException { 180 if (src == null || src.isEmpty()) 181 return null; 182 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory()); 183 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 184 if (src.getValue() == null) { 185 tgt.setValue(null); 186 } else { 187 switch (src.getValue()) { 188 case INPROGRESS: 189 tgt.setValue(org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatus.INPROGRESS); 190 break; 191 case COMPLETED: 192 tgt.setValue(org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatus.COMPLETED); 193 break; 194 case AMENDED: 195 tgt.setValue(org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatus.AMENDED); 196 break; 197 default: 198 tgt.setValue(org.hl7.fhir.dstu2.model.QuestionnaireResponse.QuestionnaireResponseStatus.NULL); 199 break; 200 } 201 } 202 return tgt; 203 } 204}