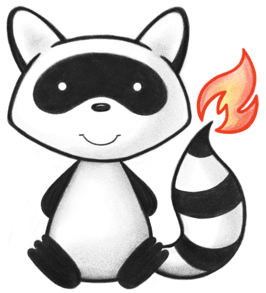
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Code10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 010import org.hl7.fhir.dstu2.model.Enumeration; 011import org.hl7.fhir.dstu2.model.SearchParameter; 012import org.hl7.fhir.dstu2.utils.ToolingExtensions; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class SearchParameter10_40 { 016 017 public static org.hl7.fhir.dstu2.model.SearchParameter convertSearchParameter(org.hl7.fhir.r4.model.SearchParameter src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.dstu2.model.SearchParameter tgt = new org.hl7.fhir.dstu2.model.SearchParameter(); 021 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 022 if (src.hasUrlElement()) 023 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 024 if (src.hasNameElement()) 025 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 026 if (src.hasStatus()) 027 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 028 if (src.hasExperimental()) 029 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 030 if (src.hasDate()) 031 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 032 if (src.hasPublisherElement()) 033 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 034 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 035 tgt.addContact(convertSearchParameterContactComponent(t)); 036 if (src.hasPurpose()) 037 tgt.setRequirements(src.getPurpose()); 038 if (src.hasCodeElement()) 039 tgt.setCodeElement(Code10_40.convertCode(src.getCodeElement())); 040 for (org.hl7.fhir.r4.model.CodeType t : src.getBase()) tgt.setBase(t.asStringValue()); 041 if (src.hasType()) 042 tgt.setTypeElement(Enumerations10_40.convertSearchParamType(src.getTypeElement())); 043 if (src.hasDescription()) 044 tgt.setDescription(src.getDescription()); 045 org.hl7.fhir.dstu2.utils.ToolingExtensions.setStringExtension(tgt, ToolingExtensions.EXT_EXPRESSION, src.getExpression()); 046 if (src.hasXpathElement()) 047 tgt.setXpathElement(String10_40.convertString(src.getXpathElement())); 048 if (src.hasXpathUsage()) 049 tgt.setXpathUsageElement(convertXPathUsageType(src.getXpathUsageElement())); 050 for (org.hl7.fhir.r4.model.CodeType t : src.getTarget()) tgt.addTarget(t.getValue()); 051 return tgt; 052 } 053 054 public static org.hl7.fhir.r4.model.SearchParameter convertSearchParameter(org.hl7.fhir.dstu2.model.SearchParameter src) throws FHIRException { 055 if (src == null || src.isEmpty()) 056 return null; 057 org.hl7.fhir.r4.model.SearchParameter tgt = new org.hl7.fhir.r4.model.SearchParameter(); 058 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 059 if (src.hasUrlElement()) 060 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 061 if (src.hasNameElement()) 062 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 063 if (src.hasStatus()) 064 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 065 if (src.hasExperimental()) 066 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 067 if (src.hasDate()) 068 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 069 if (src.hasPublisherElement()) 070 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 071 for (org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent t : src.getContact()) 072 tgt.addContact(convertSearchParameterContactComponent(t)); 073 if (src.hasRequirements()) 074 tgt.setPurpose(src.getRequirements()); 075 if (src.hasCodeElement()) 076 tgt.setCodeElement(Code10_40.convertCode(src.getCodeElement())); 077 tgt.addBase(src.getBase()); 078 if (src.hasType()) 079 tgt.setTypeElement(Enumerations10_40.convertSearchParamType(src.getTypeElement())); 080 if (src.hasDescription()) 081 tgt.setDescription(src.getDescription()); 082 tgt.setExpression(ToolingExtensions.readStringExtension(src, ToolingExtensions.EXT_EXPRESSION)); 083 if (src.hasXpathElement()) 084 tgt.setXpathElement(String10_40.convertString(src.getXpathElement())); 085 if (src.hasXpathUsage()) 086 tgt.setXpathUsageElement(convertXPathUsageType(src.getXpathUsageElement())); 087 for (org.hl7.fhir.dstu2.model.CodeType t : src.getTarget()) tgt.addTarget(t.getValue()); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.r4.model.ContactDetail convertSearchParameterContactComponent(org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 095 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 096 if (src.hasNameElement()) 097 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 098 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 099 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 100 return tgt; 101 } 102 103 public static org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent convertSearchParameterContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 104 if (src == null || src.isEmpty()) 105 return null; 106 org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent tgt = new org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent(); 107 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 108 if (src.hasNameElement()) 109 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 110 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 111 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 112 return tgt; 113 } 114 115 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType> src) throws FHIRException { 116 if (src == null || src.isEmpty()) 117 return null; 118 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SearchParameter.XPathUsageTypeEnumFactory()); 119 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 120 tgt.setValue(convertXPathUsageType(src.getValue())); 121 return tgt; 122 } 123 124 static public org.hl7.fhir.r4.model.SearchParameter.XPathUsageType convertXPathUsageType(org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType src) { 125 switch (src) { 126 case NORMAL: 127 return org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NORMAL; 128 case PHONETIC: 129 return org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.PHONETIC; 130 case NEARBY: 131 return org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NEARBY; 132 case DISTANCE: 133 return org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.DISTANCE; 134 case OTHER: 135 return org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.OTHER; 136 default: 137 return org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NULL; 138 } 139 } 140 141 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> src) throws FHIRException { 142 if (src == null || src.isEmpty()) 143 return null; 144 Enumeration<SearchParameter.XPathUsageType> tgt = new Enumeration<>(new SearchParameter.XPathUsageTypeEnumFactory()); 145 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 146 if (src.getValue() == null) { 147 tgt.setValue(null); 148 } else { 149 switch (src.getValue()) { 150 case NORMAL: 151 tgt.setValue(SearchParameter.XPathUsageType.NORMAL); 152 break; 153 case PHONETIC: 154 tgt.setValue(SearchParameter.XPathUsageType.PHONETIC); 155 break; 156 case NEARBY: 157 tgt.setValue(SearchParameter.XPathUsageType.NEARBY); 158 break; 159 case DISTANCE: 160 tgt.setValue(SearchParameter.XPathUsageType.DISTANCE); 161 break; 162 case OTHER: 163 tgt.setValue(SearchParameter.XPathUsageType.OTHER); 164 break; 165 default: 166 tgt.setValue(SearchParameter.XPathUsageType.NULL); 167 break; 168 } 169 } 170 return tgt; 171 } 172}