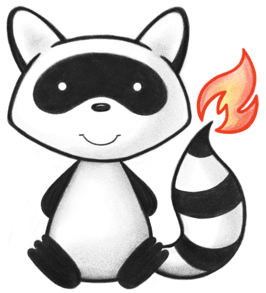
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.convertors.context.ConversionContext10_40; 007import org.hl7.fhir.convertors.conv10_40.VersionConvertor_10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.ElementDefinition10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 012import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 013import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 014import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 015import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Id10_40; 016import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 017import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r4.model.ElementDefinition; 020import org.hl7.fhir.r4.model.Enumeration; 021import org.hl7.fhir.r4.model.StructureDefinition; 022import org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind; 023import org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule; 024import org.hl7.fhir.utilities.Utilities; 025 026public class StructureDefinition10_40 { 027 028 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> convertExtensionContext(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext> src) throws FHIRException { 029 if (src == null || src.isEmpty()) 030 return null; 031 Enumeration<StructureDefinition.ExtensionContextType> tgt = new Enumeration<>(new StructureDefinition.ExtensionContextTypeEnumFactory()); 032 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 033 if (src.getValue() == null) { 034 tgt.setValue(null); 035 } else { 036 switch (src.getValue()) { 037 case RESOURCE: 038 tgt.setValue(StructureDefinition.ExtensionContextType.ELEMENT); 039 break; 040 case DATATYPE: 041 tgt.setValue(StructureDefinition.ExtensionContextType.ELEMENT); 042 break; 043 case EXTENSION: 044 tgt.setValue(StructureDefinition.ExtensionContextType.EXTENSION); 045 break; 046 default: 047 tgt.setValue(StructureDefinition.ExtensionContextType.NULL); 048 break; 049 } 050 } 051 return tgt; 052 } 053 054 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext> convertExtensionContext(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> src, String expression) throws FHIRException { 055 if (src == null || src.isEmpty()) 056 return null; 057 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContextEnumFactory()); 058 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 059 if (src.getValue() == null) { 060 tgt.setValue(null); 061 } else { 062 switch (src.getValue()) { 063 case FHIRPATH: 064 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.RESOURCE); 065 break; 066 case ELEMENT: 067 String tn = expression.contains(".") ? expression.substring(0, expression.indexOf(".")) : expression; 068 if (isResource102(tn)) { 069 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.RESOURCE); 070 } else { 071 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.DATATYPE); 072 } 073 break; 074 case EXTENSION: 075 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.EXTENSION); 076 break; 077 default: 078 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.ExtensionContext.NULL); 079 break; 080 } 081 } 082 return tgt; 083 } 084 085 public static org.hl7.fhir.r4.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.dstu2.model.StructureDefinition src) throws FHIRException { 086 if (src == null || src.isEmpty()) 087 return null; 088 org.hl7.fhir.r4.model.StructureDefinition tgt = new org.hl7.fhir.r4.model.StructureDefinition(); 089 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 090 if (src.hasUrlElement()) 091 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 092 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 093 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 094 if (src.hasVersionElement()) 095 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 096 if (src.hasNameElement()) 097 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 098 if (src.hasDisplayElement()) 099 tgt.setTitleElement(String10_40.convertString(src.getDisplayElement())); 100 if (src.hasStatus()) 101 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 102 if (src.hasExperimental()) 103 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 104 if (src.hasPublisherElement()) 105 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 106 for (org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent t : src.getContact()) 107 tgt.addContact(convertStructureDefinitionContactComponent(t)); 108 if (src.hasDate()) 109 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 110 if (src.hasDescription()) 111 tgt.setDescription(src.getDescription()); 112 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 113 if (VersionConvertor_10_40.isJurisdiction(t)) 114 tgt.addJurisdiction(CodeableConcept10_40.convertCodeableConcept(t)); 115 else 116 tgt.addUseContext(CodeableConcept10_40.convertCodeableConceptToUsageContext(t)); 117 if (src.hasRequirements()) 118 tgt.setPurpose(src.getRequirements()); 119 if (src.hasCopyright()) 120 tgt.setCopyright(src.getCopyright()); 121 for (org.hl7.fhir.dstu2.model.Coding t : src.getCode()) tgt.addKeyword(Coding10_40.convertCoding(t)); 122 if (src.hasFhirVersion()) 123 tgt.setFhirVersion(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 124 for (org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 125 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 126 if (src.hasKind()) 127 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement(), tgt.getId())); 128 if (src.hasAbstractElement()) 129 tgt.setAbstractElement(Boolean10_40.convertBoolean(src.getAbstractElement())); 130 for (org.hl7.fhir.dstu2.model.StringType t : src.getContext()) { 131 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent ec = tgt.addContext(); 132 ec.setTypeElement(convertExtensionContext(src.getContextTypeElement())); 133 ec.setExpression("*".equals(t.getValue()) ? "Element" : t.getValue()); 134 } 135 if (src.hasConstrainedType()) 136 tgt.setType(src.getConstrainedType()); 137 else if (src.getSnapshot().hasElement()) 138 tgt.setType(src.getSnapshot().getElement().get(0).getPath()); 139 else if (src.getDifferential().hasElement() && !src.getDifferential().getElement().get(0).getPath().contains(".")) 140 tgt.setType(src.getDifferential().getElement().get(0).getPath()); 141 else 142 tgt.setType(src.getDifferential().getElement().get(0).getPath().substring(0, src.getDifferential().getElement().get(0).getPath().indexOf("."))); 143 if (src.hasBase()) 144 tgt.setBaseDefinition(src.getBase()); 145 tgt.setDerivation(src.hasConstrainedType() ? org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.CONSTRAINT : org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 146 if (src.hasSnapshot()) 147 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 148 if (src.hasDifferential()) 149 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 150 if (tgt.hasSnapshot()) 151 tgt.getSnapshot().getElementFirstRep().getType().clear(); 152 if (tgt.hasDifferential()) 153 tgt.getDifferential().getElementFirstRep().getType().clear(); 154 if (tgt.getKind() == StructureDefinitionKind.PRIMITIVETYPE && !tgt.getType().equals(tgt.getId())) { 155 tgt.setDerivation(TypeDerivationRule.SPECIALIZATION); 156 tgt.setBaseDefinition("http://hl7.org/fhir/StructureDefinition/" + tgt.getType()); 157 tgt.setType(tgt.getId()); 158 } 159 if (tgt.getDerivation() == TypeDerivationRule.SPECIALIZATION) { 160 for (ElementDefinition ed : tgt.getSnapshot().getElement()) { 161 if (!ed.hasBase()) { 162 ed.getBase().setPath(ed.getPath()).setMin(ed.getMin()).setMax(ed.getMax()); 163 } 164 } 165 } 166 return tgt; 167 } 168 169 public static org.hl7.fhir.dstu2.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.r4.model.StructureDefinition src) throws FHIRException { 170 if (src == null || src.isEmpty()) 171 return null; 172 org.hl7.fhir.dstu2.model.StructureDefinition tgt = new org.hl7.fhir.dstu2.model.StructureDefinition(); 173 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 174 if (src.hasUrlElement()) 175 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 176 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 177 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 178 if (src.hasVersionElement()) 179 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 180 if (src.hasNameElement()) 181 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 182 if (src.hasTitleElement()) 183 tgt.setDisplayElement(String10_40.convertString(src.getTitleElement())); 184 if (src.hasStatus()) 185 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 186 if (src.hasExperimental()) 187 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 188 if (src.hasPublisherElement()) 189 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 190 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 191 tgt.addContact(convertStructureDefinitionContactComponent(t)); 192 if (src.hasDate()) 193 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 194 if (src.hasDescription()) 195 tgt.setDescription(src.getDescription()); 196 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 197 if (t.hasValueCodeableConcept()) 198 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t.getValueCodeableConcept())); 199 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 200 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t)); 201 if (src.hasPurpose()) 202 tgt.setRequirements(src.getPurpose()); 203 if (src.hasCopyright()) 204 tgt.setCopyright(src.getCopyright()); 205 for (org.hl7.fhir.r4.model.Coding t : src.getKeyword()) tgt.addCode(Coding10_40.convertCoding(t)); 206 if (src.hasFhirVersion()) 207 tgt.setFhirVersion(src.getFhirVersion().toCode()); 208 for (org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 209 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 210 if (src.hasKind()) 211 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 212 if (src.hasAbstractElement()) 213 tgt.setAbstractElement(Boolean10_40.convertBoolean(src.getAbstractElement())); 214 for (org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent t : src.getContext()) { 215 if (!tgt.hasContextType()) 216 tgt.setContextTypeElement(convertExtensionContext(t.getTypeElement(), t.getExpression())); 217 tgt.addContext("Element".equals(t.getExpression()) ? "*" : t.getExpression()); 218 } 219 if (src.hasType()) 220 tgt.setConstrainedType(src.getType()); 221 if (src.hasBaseDefinition()) 222 tgt.setBase(src.getBaseDefinition()); 223 if (src.hasSnapshot()) 224 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 225 if (src.hasDifferential()) 226 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 227 if (tgt.hasBase()) { 228 if (tgt.hasDifferential()) 229 tgt.getDifferential().getElement().get(0).addType().setCode(tail(tgt.getBase())); 230 if (tgt.hasSnapshot()) 231 tgt.getSnapshot().getElement().get(0).addType().setCode(tail(tgt.getBase())); 232 } 233 return tgt; 234 } 235 236 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent convertStructureDefinitionContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 237 if (src == null || src.isEmpty()) 238 return null; 239 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent(); 240 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 241 if (src.hasNameElement()) 242 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 243 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 244 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 245 return tgt; 246 } 247 248 public static org.hl7.fhir.r4.model.ContactDetail convertStructureDefinitionContactComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionContactComponent src) throws FHIRException { 249 if (src == null || src.isEmpty()) 250 return null; 251 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 252 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 253 if (src.hasNameElement()) 254 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 255 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 256 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 257 return tgt; 258 } 259 260 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 261 if (src == null || src.isEmpty()) 262 return null; 263 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 264 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 265 List<String> slicePaths = new ArrayList<String>(); 266 for (org.hl7.fhir.dstu2.model.ElementDefinition t : src.getElement()) { 267 if (t.hasSlicing()) 268 slicePaths.add(t.getPath()); 269 tgt.addElement(ElementDefinition10_40.convertElementDefinition(t, slicePaths, src.getElement(), src.getElement().indexOf(t))); 270 } 271 return tgt; 272 } 273 274 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 275 if (src == null || src.isEmpty()) 276 return null; 277 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 278 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 279 for (org.hl7.fhir.r4.model.ElementDefinition t : src.getElement()) 280 tgt.addElement(ElementDefinition10_40.convertElementDefinition(t)); 281 return tgt; 282 } 283 284 public static org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind> src, String dtName) throws FHIRException { 285 if (src == null || src.isEmpty()) 286 return null; 287 Enumeration<StructureDefinitionKind> tgt = new Enumeration<>(new StructureDefinition.StructureDefinitionKindEnumFactory()); 288 if (src.getValue() == null) { 289 tgt.setValue(null); 290 } else { 291 switch (src.getValue()) { 292 case DATATYPE: 293 if (Utilities.existsInList(dtName, "boolean", "integer", "decimal", "base64Binary", "instant", "string", "uri", "date", "dateTime", "time", "code", "oid", "uuid", "id", "unsignedInt", "positiveInt", "markdown", "xhtml", "url", "canonical")) 294 tgt.setValue(StructureDefinitionKind.PRIMITIVETYPE); 295 else 296 tgt.setValue(StructureDefinitionKind.COMPLEXTYPE); 297 break; 298 case RESOURCE: 299 tgt.setValue(StructureDefinitionKind.RESOURCE); 300 break; 301 case LOGICAL: 302 tgt.setValue(StructureDefinitionKind.LOGICAL); 303 break; 304 default: 305 tgt.setValue(StructureDefinitionKind.NULL); 306 break; 307 } 308 } 309 return tgt; 310 } 311 312 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 313 if (src == null || src.isEmpty()) 314 return null; 315 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 316 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 317 if (src.getValue() == null) { 318 tgt.setValue(null); 319 } else { 320 switch (src.getValue()) { 321 case PRIMITIVETYPE: 322 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 323 break; 324 case COMPLEXTYPE: 325 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 326 break; 327 case RESOURCE: 328 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 329 break; 330 case LOGICAL: 331 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 332 break; 333 default: 334 tgt.setValue(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionKind.NULL); 335 break; 336 } 337 } 338 return tgt; 339 } 340 341 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 342 if (src == null || src.isEmpty()) 343 return null; 344 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent(); 345 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 346 if (src.hasIdentityElement()) 347 tgt.setIdentityElement(Id10_40.convertId(src.getIdentityElement())); 348 if (src.hasUriElement()) 349 tgt.setUriElement(Uri10_40.convertUri(src.getUriElement())); 350 if (src.hasNameElement()) 351 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 352 if (src.hasCommentElement()) 353 tgt.setCommentsElement(String10_40.convertString(src.getCommentElement())); 354 return tgt; 355 } 356 357 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 358 if (src == null || src.isEmpty()) 359 return null; 360 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent(); 361 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 362 if (src.hasIdentityElement()) 363 tgt.setIdentityElement(Id10_40.convertId(src.getIdentityElement())); 364 if (src.hasUriElement()) 365 tgt.setUriElement(Uri10_40.convertUri(src.getUriElement())); 366 if (src.hasNameElement()) 367 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 368 if (src.hasCommentsElement()) 369 tgt.setCommentElement(String10_40.convertString(src.getCommentsElement())); 370 return tgt; 371 } 372 373 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 374 if (src == null || src.isEmpty()) 375 return null; 376 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 377 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 378 List<String> slicePaths = new ArrayList<String>(); 379 for (org.hl7.fhir.dstu2.model.ElementDefinition t : src.getElement()) { 380 if (t.hasSlicing()) 381 slicePaths.add(t.getPath()); 382 tgt.addElement(ElementDefinition10_40.convertElementDefinition(t, slicePaths, src.getElement(), src.getElement().indexOf(t))); 383 } 384 return tgt; 385 } 386 387 public static org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 388 if (src == null || src.isEmpty()) 389 return null; 390 org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.dstu2.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 391 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 392 for (org.hl7.fhir.r4.model.ElementDefinition t : src.getElement()) 393 tgt.addElement(ElementDefinition10_40.convertElementDefinition(t)); 394 return tgt; 395 } 396 397 static public boolean isResource102(String tn) { 398 return Utilities.existsInList(tn, "AllergyIntolerance", "Appointment", "AppointmentResponse", "AuditEvent", "Basic", "Binary", "BodySite", "Bundle", "CarePlan", "Claim", "ClaimResponse", "ClinicalImpression", "Communication", "CommunicationRequest", "Composition", "ConceptMap", "Condition", "Conformance", "Contract", "DetectedIssue", "Coverage", "DataElement", "Device", "DeviceComponent", "DeviceMetric", "DeviceUseRequest", "DeviceUseStatement", "DiagnosticOrder", "DiagnosticReport", "DocumentManifest", "DocumentReference", "EligibilityRequest", "EligibilityResponse", "Encounter", "EnrollmentRequest", "EnrollmentResponse", "EpisodeOfCare", "ExplanationOfBenefit", "FamilyMemberHistory", "Flag", "Goal", "Group", "HealthcareService", "ImagingObjectSelection", "ImagingStudy", "Immunization", "ImmunizationRecommendation", "ImplementationGuide", "List", "Location", "Media", "Medication", "MedicationAdministration", "MedicationDispense", "MedicationOrder", "MedicationStatement", "MessageHeader", "NamingSystem", "NutritionOrder", "Observation", "OperationDefinition", "OperationOutcome", "Order", "OrderResponse", "Organization", "Parameters", "Patient", "PaymentNotice", "PaymentReconciliation", "Person", "Practitioner", "Procedure", "ProcessRequest", "ProcessResponse", "ProcedureRequest", "Provenance", "Questionnaire", "QuestionnaireResponse", "ReferralRequest", "RelatedPerson", "RiskAssessment", "Schedule", "SearchParameter", "Slot", "Specimen", "StructureDefinition", "Subscription", "Substance", "SupplyRequest", "SupplyDelivery", "TestScript", "ValueSet", "VisionPrescription"); 399 } 400 401 static public String tail(String base) { 402 return base.substring(base.lastIndexOf("/") + 1); 403 } 404}