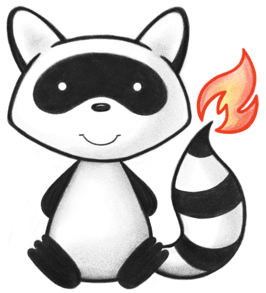
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Ratio10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.SimpleQuantity10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class Substance10_40 { 014 015 public static org.hl7.fhir.r4.model.Substance convertSubstance(org.hl7.fhir.dstu2.model.Substance src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 org.hl7.fhir.r4.model.Substance tgt = new org.hl7.fhir.r4.model.Substance(); 019 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 020 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 021 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 022 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCategory()) 023 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(t)); 024 if (src.hasCode()) 025 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 026 if (src.hasDescriptionElement()) 027 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 028 for (org.hl7.fhir.dstu2.model.Substance.SubstanceInstanceComponent t : src.getInstance()) 029 tgt.addInstance(convertSubstanceInstanceComponent(t)); 030 for (org.hl7.fhir.dstu2.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 031 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 032 return tgt; 033 } 034 035 public static org.hl7.fhir.dstu2.model.Substance convertSubstance(org.hl7.fhir.r4.model.Substance src) throws FHIRException { 036 if (src == null || src.isEmpty()) 037 return null; 038 org.hl7.fhir.dstu2.model.Substance tgt = new org.hl7.fhir.dstu2.model.Substance(); 039 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 040 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 041 tgt.addIdentifier(Identifier10_40.convertIdentifier(t)); 042 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 043 tgt.addCategory(CodeableConcept10_40.convertCodeableConcept(t)); 044 if (src.hasCode()) 045 tgt.setCode(CodeableConcept10_40.convertCodeableConcept(src.getCode())); 046 if (src.hasDescriptionElement()) 047 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 048 for (org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent t : src.getInstance()) 049 tgt.addInstance(convertSubstanceInstanceComponent(t)); 050 for (org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 051 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 052 return tgt; 053 } 054 055 public static org.hl7.fhir.dstu2.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 056 if (src == null || src.isEmpty()) 057 return null; 058 org.hl7.fhir.dstu2.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.dstu2.model.Substance.SubstanceIngredientComponent(); 059 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 060 if (src.hasQuantity()) 061 tgt.setQuantity(Ratio10_40.convertRatio(src.getQuantity())); 062 return tgt; 063 } 064 065 public static org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.dstu2.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 066 if (src == null || src.isEmpty()) 067 return null; 068 org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent(); 069 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 070 if (src.hasQuantity()) 071 tgt.setQuantity(Ratio10_40.convertRatio(src.getQuantity())); 072 if (src.hasSubstance()) 073 tgt.setSubstance(Reference10_40.convertReference(src.getSubstance())); 074 return tgt; 075 } 076 077 public static org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent convertSubstanceInstanceComponent(org.hl7.fhir.dstu2.model.Substance.SubstanceInstanceComponent src) throws FHIRException { 078 if (src == null || src.isEmpty()) 079 return null; 080 org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent tgt = new org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent(); 081 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 082 if (src.hasIdentifier()) 083 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 084 if (src.hasExpiryElement()) 085 tgt.setExpiryElement(DateTime10_40.convertDateTime(src.getExpiryElement())); 086 if (src.hasQuantity()) 087 tgt.setQuantity(SimpleQuantity10_40.convertSimpleQuantity(src.getQuantity())); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.dstu2.model.Substance.SubstanceInstanceComponent convertSubstanceInstanceComponent(org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 org.hl7.fhir.dstu2.model.Substance.SubstanceInstanceComponent tgt = new org.hl7.fhir.dstu2.model.Substance.SubstanceInstanceComponent(); 095 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 096 if (src.hasIdentifier()) 097 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 098 if (src.hasExpiryElement()) 099 tgt.setExpiryElement(DateTime10_40.convertDateTime(src.getExpiryElement())); 100 if (src.hasQuantity()) 101 tgt.setQuantity(SimpleQuantity10_40.convertSimpleQuantity(src.getQuantity())); 102 return tgt; 103 } 104}