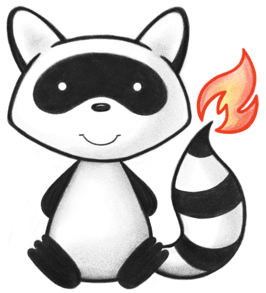
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.exceptions.FHIRException; 005import org.hl7.fhir.r4.model.SupplyRequest; 006 007public class SupplyRequest10_40 { 008 009 public static org.hl7.fhir.r4.model.SupplyRequest convertSupplyRequest(org.hl7.fhir.dstu2.model.SupplyRequest src) throws FHIRException { 010 if (src == null || src.isEmpty()) 011 return null; 012 org.hl7.fhir.r4.model.SupplyRequest tgt = new org.hl7.fhir.r4.model.SupplyRequest(); 013 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 014 return tgt; 015 } 016 017 public static org.hl7.fhir.dstu2.model.SupplyRequest convertSupplyRequest(org.hl7.fhir.r4.model.SupplyRequest src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.dstu2.model.SupplyRequest tgt = new org.hl7.fhir.dstu2.model.SupplyRequest(); 021 return tgt; 022 } 023 024 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus> convertSupplyRequestStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus> src) throws FHIRException { 025 if (src == null || src.isEmpty()) return null; 026 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatusEnumFactory()); 027 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 028 if (src.getValue() == null) { 029 tgt.setValue(null); 030} else { 031 switch(src.getValue()) { 032 case REQUESTED: 033 tgt.setValue(SupplyRequest.SupplyRequestStatus.ACTIVE); 034 break; 035 case COMPLETED: 036 tgt.setValue(SupplyRequest.SupplyRequestStatus.COMPLETED); 037 break; 038 case FAILED: 039 tgt.setValue(SupplyRequest.SupplyRequestStatus.CANCELLED); 040 break; 041 case CANCELLED: 042 tgt.setValue(SupplyRequest.SupplyRequestStatus.CANCELLED); 043 break; 044 default: 045 tgt.setValue(SupplyRequest.SupplyRequestStatus.NULL); 046 break; 047 } 048} 049 return tgt; 050 } 051 052 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus> convertSupplyRequestStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus> src) throws FHIRException { 053 if (src == null || src.isEmpty()) return null; 054 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatusEnumFactory()); 055 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 056 if (src.getValue() == null) { 057 tgt.setValue(null); 058} else { 059 switch(src.getValue()) { 060 case ACTIVE: 061 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.REQUESTED); 062 break; 063 case COMPLETED: 064 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.COMPLETED); 065 break; 066 case CANCELLED: 067 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.CANCELLED); 068 break; 069 default: 070 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.NULL); 071 break; 072 } 073} 074 return tgt; 075 } 076}