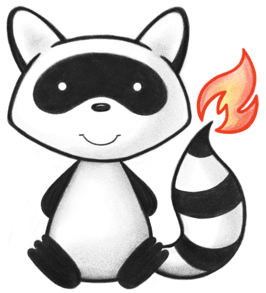
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_40; 004import org.hl7.fhir.convertors.conv10_40.VersionConvertor_10_40; 005import org.hl7.fhir.convertors.conv10_40.datatypes10_40.Reference10_40; 006import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 007import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Canonical10_40; 012import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Code10_40; 013import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 014import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Id10_40; 015import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Integer10_40; 016import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 017import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 018import org.hl7.fhir.dstu2.model.Enumeration; 019import org.hl7.fhir.dstu2.model.TestScript; 020import org.hl7.fhir.exceptions.FHIRException; 021 022public class TestScript10_40 { 023 024 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionDirectionType> src) throws FHIRException { 025 if (src == null || src.isEmpty()) 026 return null; 027 Enumeration<TestScript.AssertionDirectionType> tgt = new Enumeration<>(new TestScript.AssertionDirectionTypeEnumFactory()); 028 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 029 if (src.getValue() == null) { 030 tgt.setValue(null); 031 } else { 032 switch (src.getValue()) { 033 case RESPONSE: 034 tgt.setValue(TestScript.AssertionDirectionType.RESPONSE); 035 break; 036 case REQUEST: 037 tgt.setValue(TestScript.AssertionDirectionType.REQUEST); 038 break; 039 default: 040 tgt.setValue(TestScript.AssertionDirectionType.NULL); 041 break; 042 } 043 } 044 return tgt; 045 } 046 047 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionDirectionType> src) throws FHIRException { 048 if (src == null || src.isEmpty()) 049 return null; 050 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestScript.AssertionDirectionTypeEnumFactory()); 051 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 052 if (src.getValue() == null) { 053 tgt.setValue(null); 054 } else { 055 switch (src.getValue()) { 056 case RESPONSE: 057 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionDirectionType.RESPONSE); 058 break; 059 case REQUEST: 060 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionDirectionType.REQUEST); 061 break; 062 default: 063 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionDirectionType.NULL); 064 break; 065 } 066 } 067 return tgt; 068 } 069 070 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionOperatorType> src) throws FHIRException { 071 if (src == null || src.isEmpty()) 072 return null; 073 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestScript.AssertionOperatorTypeEnumFactory()); 074 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 075 if (src.getValue() == null) { 076 tgt.setValue(null); 077 } else { 078 switch (src.getValue()) { 079 case EQUALS: 080 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.EQUALS); 081 break; 082 case NOTEQUALS: 083 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTEQUALS); 084 break; 085 case IN: 086 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.IN); 087 break; 088 case NOTIN: 089 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTIN); 090 break; 091 case GREATERTHAN: 092 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.GREATERTHAN); 093 break; 094 case LESSTHAN: 095 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.LESSTHAN); 096 break; 097 case EMPTY: 098 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.EMPTY); 099 break; 100 case NOTEMPTY: 101 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTEMPTY); 102 break; 103 case CONTAINS: 104 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.CONTAINS); 105 break; 106 case NOTCONTAINS: 107 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NOTCONTAINS); 108 break; 109 default: 110 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionOperatorType.NULL); 111 break; 112 } 113 } 114 return tgt; 115 } 116 117 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionOperatorType> src) throws FHIRException { 118 if (src == null || src.isEmpty()) 119 return null; 120 Enumeration<TestScript.AssertionOperatorType> tgt = new Enumeration<>(new TestScript.AssertionOperatorTypeEnumFactory()); 121 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 122 if (src.getValue() == null) { 123 tgt.setValue(null); 124 } else { 125 switch (src.getValue()) { 126 case EQUALS: 127 tgt.setValue(TestScript.AssertionOperatorType.EQUALS); 128 break; 129 case NOTEQUALS: 130 tgt.setValue(TestScript.AssertionOperatorType.NOTEQUALS); 131 break; 132 case IN: 133 tgt.setValue(TestScript.AssertionOperatorType.IN); 134 break; 135 case NOTIN: 136 tgt.setValue(TestScript.AssertionOperatorType.NOTIN); 137 break; 138 case GREATERTHAN: 139 tgt.setValue(TestScript.AssertionOperatorType.GREATERTHAN); 140 break; 141 case LESSTHAN: 142 tgt.setValue(TestScript.AssertionOperatorType.LESSTHAN); 143 break; 144 case EMPTY: 145 tgt.setValue(TestScript.AssertionOperatorType.EMPTY); 146 break; 147 case NOTEMPTY: 148 tgt.setValue(TestScript.AssertionOperatorType.NOTEMPTY); 149 break; 150 case CONTAINS: 151 tgt.setValue(TestScript.AssertionOperatorType.CONTAINS); 152 break; 153 case NOTCONTAINS: 154 tgt.setValue(TestScript.AssertionOperatorType.NOTCONTAINS); 155 break; 156 default: 157 tgt.setValue(TestScript.AssertionOperatorType.NULL); 158 break; 159 } 160 } 161 return tgt; 162 } 163 164 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 165 if (src == null || src.isEmpty()) 166 return null; 167 Enumeration<TestScript.AssertionResponseTypes> tgt = new Enumeration<>(new TestScript.AssertionResponseTypesEnumFactory()); 168 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 169 if (src.getValue() == null) { 170 tgt.setValue(null); 171 } else { 172 switch (src.getValue()) { 173 case OKAY: 174 tgt.setValue(TestScript.AssertionResponseTypes.OKAY); 175 break; 176 case CREATED: 177 tgt.setValue(TestScript.AssertionResponseTypes.CREATED); 178 break; 179 case NOCONTENT: 180 tgt.setValue(TestScript.AssertionResponseTypes.NOCONTENT); 181 break; 182 case NOTMODIFIED: 183 tgt.setValue(TestScript.AssertionResponseTypes.NOTMODIFIED); 184 break; 185 case BAD: 186 tgt.setValue(TestScript.AssertionResponseTypes.BAD); 187 break; 188 case FORBIDDEN: 189 tgt.setValue(TestScript.AssertionResponseTypes.FORBIDDEN); 190 break; 191 case NOTFOUND: 192 tgt.setValue(TestScript.AssertionResponseTypes.NOTFOUND); 193 break; 194 case METHODNOTALLOWED: 195 tgt.setValue(TestScript.AssertionResponseTypes.METHODNOTALLOWED); 196 break; 197 case CONFLICT: 198 tgt.setValue(TestScript.AssertionResponseTypes.CONFLICT); 199 break; 200 case GONE: 201 tgt.setValue(TestScript.AssertionResponseTypes.GONE); 202 break; 203 case PRECONDITIONFAILED: 204 tgt.setValue(TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 205 break; 206 case UNPROCESSABLE: 207 tgt.setValue(TestScript.AssertionResponseTypes.UNPROCESSABLE); 208 break; 209 default: 210 tgt.setValue(TestScript.AssertionResponseTypes.NULL); 211 break; 212 } 213 } 214 return tgt; 215 } 216 217 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 218 if (src == null || src.isEmpty()) 219 return null; 220 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.TestScript.AssertionResponseTypesEnumFactory()); 221 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 222 if (src.getValue() == null) { 223 tgt.setValue(null); 224 } else { 225 switch (src.getValue()) { 226 case OKAY: 227 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.OKAY); 228 break; 229 case CREATED: 230 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.CREATED); 231 break; 232 case NOCONTENT: 233 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NOCONTENT); 234 break; 235 case NOTMODIFIED: 236 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 237 break; 238 case BAD: 239 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.BAD); 240 break; 241 case FORBIDDEN: 242 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.FORBIDDEN); 243 break; 244 case NOTFOUND: 245 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NOTFOUND); 246 break; 247 case METHODNOTALLOWED: 248 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 249 break; 250 case CONFLICT: 251 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.CONFLICT); 252 break; 253 case GONE: 254 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.GONE); 255 break; 256 case PRECONDITIONFAILED: 257 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 258 break; 259 case UNPROCESSABLE: 260 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.UNPROCESSABLE); 261 break; 262 default: 263 tgt.setValue(org.hl7.fhir.r4.model.TestScript.AssertionResponseTypes.NULL); 264 break; 265 } 266 } 267 return tgt; 268 } 269 270 static public String convertContentType(org.hl7.fhir.dstu2.model.TestScript.ContentType src) throws FHIRException { 271 if (src == null) 272 return null; 273 switch (src) { 274 case XML: 275 return "application/fhir+xml"; 276 case JSON: 277 return "application/fhir+json"; 278 default: 279 return null; 280 } 281 } 282 283 static public org.hl7.fhir.dstu2.model.TestScript.ContentType convertContentType(String src) throws FHIRException { 284 if (src == null) 285 return null; 286 if (src.contains("xml")) 287 return org.hl7.fhir.dstu2.model.TestScript.ContentType.XML; 288 if (src.contains("json")) 289 return org.hl7.fhir.dstu2.model.TestScript.ContentType.JSON; 290 return org.hl7.fhir.dstu2.model.TestScript.ContentType.NULL; 291 } 292 293 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 294 if (src == null || src.isEmpty()) 295 return null; 296 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent(); 297 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 298 if (src.hasLabelElement()) 299 tgt.setLabelElement(String10_40.convertString(src.getLabelElement())); 300 if (src.hasDescriptionElement()) 301 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 302 if (src.hasDirection()) 303 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 304 if (src.hasCompareToSourceIdElement()) 305 tgt.setCompareToSourceIdElement(String10_40.convertString(src.getCompareToSourceIdElement())); 306 if (src.hasCompareToSourcePathElement()) 307 tgt.setCompareToSourcePathElement(String10_40.convertString(src.getCompareToSourcePathElement())); 308 if (src.hasContentType()) 309 tgt.setContentType(convertContentType(src.getContentType())); 310 if (src.hasHeaderFieldElement()) 311 tgt.setHeaderFieldElement(String10_40.convertString(src.getHeaderFieldElement())); 312 if (src.hasMinimumIdElement()) 313 tgt.setMinimumIdElement(String10_40.convertString(src.getMinimumIdElement())); 314 if (src.hasNavigationLinksElement()) 315 tgt.setNavigationLinksElement(Boolean10_40.convertBoolean(src.getNavigationLinksElement())); 316 if (src.hasOperator()) 317 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 318 if (src.hasPathElement()) 319 tgt.setPathElement(String10_40.convertString(src.getPathElement())); 320 if (src.hasResourceElement()) 321 tgt.setResourceElement(Code10_40.convertCode(src.getResourceElement())); 322 if (src.hasResponse()) 323 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 324 if (src.hasResponseCodeElement()) 325 tgt.setResponseCodeElement(String10_40.convertString(src.getResponseCodeElement())); 326 if (src.hasSourceIdElement()) 327 tgt.setSourceIdElement(Id10_40.convertId(src.getSourceIdElement())); 328 if (src.hasValidateProfileIdElement()) 329 tgt.setValidateProfileIdElement(Id10_40.convertId(src.getValidateProfileIdElement())); 330 if (src.hasValueElement()) 331 tgt.setValueElement(String10_40.convertString(src.getValueElement())); 332 if (src.hasWarningOnlyElement()) 333 tgt.setWarningOnlyElement(Boolean10_40.convertBoolean(src.getWarningOnlyElement())); 334 return tgt; 335 } 336 337 public static org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent src) throws FHIRException { 338 if (src == null || src.isEmpty()) 339 return null; 340 org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionAssertComponent(); 341 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 342 if (src.hasLabelElement()) 343 tgt.setLabelElement(String10_40.convertString(src.getLabelElement())); 344 if (src.hasDescriptionElement()) 345 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 346 if (src.hasDirection()) 347 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 348 if (src.hasCompareToSourceIdElement()) 349 tgt.setCompareToSourceIdElement(String10_40.convertString(src.getCompareToSourceIdElement())); 350 if (src.hasCompareToSourcePathElement()) 351 tgt.setCompareToSourcePathElement(String10_40.convertString(src.getCompareToSourcePathElement())); 352 if (src.hasContentType()) 353 tgt.setContentType(convertContentType(src.getContentType())); 354 if (src.hasHeaderFieldElement()) 355 tgt.setHeaderFieldElement(String10_40.convertString(src.getHeaderFieldElement())); 356 if (src.hasMinimumIdElement()) 357 tgt.setMinimumIdElement(String10_40.convertString(src.getMinimumIdElement())); 358 if (src.hasNavigationLinksElement()) 359 tgt.setNavigationLinksElement(Boolean10_40.convertBoolean(src.getNavigationLinksElement())); 360 if (src.hasOperator()) 361 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 362 if (src.hasPathElement()) 363 tgt.setPathElement(String10_40.convertString(src.getPathElement())); 364 if (src.hasResourceElement()) 365 tgt.setResourceElement(Code10_40.convertCode(src.getResourceElement())); 366 if (src.hasResponse()) 367 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 368 if (src.hasResponseCodeElement()) 369 tgt.setResponseCodeElement(String10_40.convertString(src.getResponseCodeElement())); 370 if (src.hasSourceIdElement()) 371 tgt.setSourceIdElement(Id10_40.convertId(src.getSourceIdElement())); 372 if (src.hasValidateProfileIdElement()) 373 tgt.setValidateProfileIdElement(Id10_40.convertId(src.getValidateProfileIdElement())); 374 if (src.hasValueElement()) 375 tgt.setValueElement(String10_40.convertString(src.getValueElement())); 376 if (src.hasWarningOnlyElement()) 377 tgt.setWarningOnlyElement(Boolean10_40.convertBoolean(src.getWarningOnlyElement())); 378 return tgt; 379 } 380 381 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent convertSetupActionComponent(org.hl7.fhir.r4.model.TestScript.SetupActionComponent src) throws FHIRException { 382 if (src == null || src.isEmpty()) 383 return null; 384 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent(); 385 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 386 if (src.hasOperation()) 387 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 388 if (src.hasAssert()) 389 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 390 return tgt; 391 } 392 393 public static org.hl7.fhir.r4.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent src) throws FHIRException { 394 if (src == null || src.isEmpty()) 395 return null; 396 org.hl7.fhir.r4.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionComponent(); 397 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 398 if (src.hasOperation()) 399 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 400 if (src.hasAssert()) 401 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 402 return tgt; 403 } 404 405 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 406 if (src == null || src.isEmpty()) 407 return null; 408 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent(); 409 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 410 if (src.hasType()) 411 tgt.setType(Coding10_40.convertCoding(src.getType())); 412 if (src.hasResourceElement()) 413 tgt.setResourceElement(Code10_40.convertCode(src.getResourceElement())); 414 if (src.hasLabelElement()) 415 tgt.setLabelElement(String10_40.convertString(src.getLabelElement())); 416 if (src.hasDescriptionElement()) 417 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 418 if (src.hasAccept()) 419 tgt.setAccept(convertContentType(src.getAccept())); 420 if (src.hasContentType()) 421 tgt.setContentType(convertContentType(src.getContentType())); 422 if (src.hasDestinationElement()) 423 tgt.setDestinationElement(Integer10_40.convertInteger(src.getDestinationElement())); 424 if (src.hasEncodeRequestUrlElement()) 425 tgt.setEncodeRequestUrlElement(Boolean10_40.convertBoolean(src.getEncodeRequestUrlElement())); 426 if (src.hasParamsElement()) 427 tgt.setParamsElement(String10_40.convertString(src.getParamsElement())); 428 for (org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 429 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 430 if (src.hasResponseIdElement()) 431 tgt.setResponseIdElement(Id10_40.convertId(src.getResponseIdElement())); 432 if (src.hasSourceIdElement()) 433 tgt.setSourceIdElement(Id10_40.convertId(src.getSourceIdElement())); 434 if (src.hasTargetId()) 435 tgt.setTargetId(src.getTargetId()); 436 if (src.hasUrlElement()) 437 tgt.setUrlElement(String10_40.convertString(src.getUrlElement())); 438 return tgt; 439 } 440 441 public static org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent src) throws FHIRException { 442 if (src == null || src.isEmpty()) 443 return null; 444 org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionOperationComponent(); 445 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 446 if (src.hasType()) 447 tgt.setType(Coding10_40.convertCoding(src.getType())); 448 if (src.hasResourceElement()) 449 tgt.setResourceElement(Code10_40.convertCode(src.getResourceElement())); 450 if (src.hasLabelElement()) 451 tgt.setLabelElement(String10_40.convertString(src.getLabelElement())); 452 if (src.hasDescriptionElement()) 453 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 454 if (src.hasAccept()) 455 tgt.setAccept(convertContentType(src.getAccept())); 456 if (src.hasContentType()) 457 tgt.setContentType(convertContentType(src.getContentType())); 458 if (src.hasDestinationElement()) 459 tgt.setDestinationElement(Integer10_40.convertInteger(src.getDestinationElement())); 460 if (src.hasEncodeRequestUrlElement()) 461 tgt.setEncodeRequestUrlElement(Boolean10_40.convertBoolean(src.getEncodeRequestUrlElement())); 462 if (src.hasParamsElement()) 463 tgt.setParamsElement(String10_40.convertString(src.getParamsElement())); 464 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 465 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 466 if (src.hasResponseIdElement()) 467 tgt.setResponseIdElement(Id10_40.convertId(src.getResponseIdElement())); 468 if (src.hasSourceIdElement()) 469 tgt.setSourceIdElement(Id10_40.convertId(src.getSourceIdElement())); 470 if (src.hasTargetId()) 471 tgt.setTargetId(src.getTargetId()); 472 if (src.hasUrlElement()) 473 tgt.setUrlElement(String10_40.convertString(src.getUrlElement())); 474 return tgt; 475 } 476 477 public static org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent src) throws FHIRException { 478 if (src == null || src.isEmpty()) 479 return null; 480 org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent(); 481 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 482 if (src.hasFieldElement()) 483 tgt.setFieldElement(String10_40.convertString(src.getFieldElement())); 484 if (src.hasValueElement()) 485 tgt.setValueElement(String10_40.convertString(src.getValueElement())); 486 return tgt; 487 } 488 489 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.r4.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 490 if (src == null || src.isEmpty()) 491 return null; 492 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent(); 493 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 494 if (src.hasFieldElement()) 495 tgt.setFieldElement(String10_40.convertString(src.getFieldElement())); 496 if (src.hasValueElement()) 497 tgt.setValueElement(String10_40.convertString(src.getValueElement())); 498 return tgt; 499 } 500 501 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r4.model.TestScript.TeardownActionComponent src) throws FHIRException { 502 if (src == null || src.isEmpty()) 503 return null; 504 org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent(); 505 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 506 if (src.hasOperation()) 507 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 508 return tgt; 509 } 510 511 public static org.hl7.fhir.r4.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent src) throws FHIRException { 512 if (src == null || src.isEmpty()) 513 return null; 514 org.hl7.fhir.r4.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.r4.model.TestScript.TeardownActionComponent(); 515 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 516 if (src.hasOperation()) 517 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 518 return tgt; 519 } 520 521 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent convertTestActionComponent(org.hl7.fhir.r4.model.TestScript.TestActionComponent src) throws FHIRException { 522 if (src == null || src.isEmpty()) 523 return null; 524 org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent(); 525 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 526 if (src.hasOperation()) 527 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 528 if (src.hasAssert()) 529 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 530 return tgt; 531 } 532 533 public static org.hl7.fhir.r4.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent src) throws FHIRException { 534 if (src == null || src.isEmpty()) 535 return null; 536 org.hl7.fhir.r4.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestActionComponent(); 537 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 538 if (src.hasOperation()) 539 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 540 if (src.hasAssert()) 541 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 542 return tgt; 543 } 544 545 public static org.hl7.fhir.dstu2.model.TestScript convertTestScript(org.hl7.fhir.r4.model.TestScript src) throws FHIRException { 546 if (src == null || src.isEmpty()) 547 return null; 548 org.hl7.fhir.dstu2.model.TestScript tgt = new org.hl7.fhir.dstu2.model.TestScript(); 549 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 550 if (src.hasUrlElement()) 551 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 552 if (src.hasVersionElement()) 553 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 554 if (src.hasNameElement()) 555 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 556 if (src.hasStatus()) 557 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 558 if (src.hasIdentifier()) 559 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 560 if (src.hasExperimental()) 561 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 562 if (src.hasPublisherElement()) 563 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 564 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) tgt.addContact(convertTestScriptContactComponent(t)); 565 if (src.hasDate()) 566 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 567 if (src.hasDescription()) 568 tgt.setDescription(src.getDescription()); 569 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 570 if (t.hasValueCodeableConcept()) 571 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t.getValueCodeableConcept())); 572 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 573 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t)); 574 if (src.hasPurpose()) 575 tgt.setRequirements(src.getPurpose()); 576 if (src.hasCopyright()) 577 tgt.setCopyright(src.getCopyright()); 578 if (src.hasMetadata()) 579 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 580 for (org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 581 tgt.addFixture(convertTestScriptFixtureComponent(t)); 582 for (org.hl7.fhir.r4.model.Reference t : src.getProfile()) tgt.addProfile(Reference10_40.convertReference(t)); 583 for (org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 584 tgt.addVariable(convertTestScriptVariableComponent(t)); 585 if (src.hasSetup()) 586 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 587 for (org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent t : src.getTest()) 588 tgt.addTest(convertTestScriptTestComponent(t)); 589 if (src.hasTeardown()) 590 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 591 return tgt; 592 } 593 594 public static org.hl7.fhir.r4.model.TestScript convertTestScript(org.hl7.fhir.dstu2.model.TestScript src) throws FHIRException { 595 if (src == null || src.isEmpty()) 596 return null; 597 org.hl7.fhir.r4.model.TestScript tgt = new org.hl7.fhir.r4.model.TestScript(); 598 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 599 if (src.hasUrlElement()) 600 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 601 if (src.hasVersionElement()) 602 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 603 if (src.hasNameElement()) 604 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 605 if (src.hasStatus()) 606 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 607 if (src.hasIdentifier()) 608 tgt.setIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 609 if (src.hasExperimental()) 610 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 611 if (src.hasPublisherElement()) 612 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 613 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent t : src.getContact()) 614 tgt.addContact(convertTestScriptContactComponent(t)); 615 if (src.hasDate()) 616 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 617 if (src.hasDescription()) 618 tgt.setDescription(src.getDescription()); 619 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 620 if (VersionConvertor_10_40.isJurisdiction(t)) 621 tgt.addJurisdiction(CodeableConcept10_40.convertCodeableConcept(t)); 622 else 623 tgt.addUseContext(CodeableConcept10_40.convertCodeableConceptToUsageContext(t)); 624 if (src.hasRequirements()) 625 tgt.setPurpose(src.getRequirements()); 626 if (src.hasCopyright()) 627 tgt.setCopyright(src.getCopyright()); 628 if (src.hasMetadata()) 629 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 630 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 631 tgt.addFixture(convertTestScriptFixtureComponent(t)); 632 for (org.hl7.fhir.dstu2.model.Reference t : src.getProfile()) tgt.addProfile(Reference10_40.convertReference(t)); 633 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 634 tgt.addVariable(convertTestScriptVariableComponent(t)); 635 if (src.hasSetup()) 636 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 637 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent t : src.getTest()) 638 tgt.addTest(convertTestScriptTestComponent(t)); 639 if (src.hasTeardown()) 640 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 641 return tgt; 642 } 643 644 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent convertTestScriptContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 645 if (src == null || src.isEmpty()) 646 return null; 647 org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent(); 648 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 649 if (src.hasNameElement()) 650 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 651 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 652 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 653 return tgt; 654 } 655 656 public static org.hl7.fhir.r4.model.ContactDetail convertTestScriptContactComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent src) throws FHIRException { 657 if (src == null || src.isEmpty()) 658 return null; 659 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 660 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 661 if (src.hasNameElement()) 662 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 663 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 664 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 665 return tgt; 666 } 667 668 public static org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 669 if (src == null || src.isEmpty()) 670 return null; 671 org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent(); 672 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 673 if (src.hasAutocreateElement()) 674 tgt.setAutocreateElement(Boolean10_40.convertBoolean(src.getAutocreateElement())); 675 if (src.hasAutodeleteElement()) 676 tgt.setAutodeleteElement(Boolean10_40.convertBoolean(src.getAutodeleteElement())); 677 if (src.hasResource()) 678 tgt.setResource(Reference10_40.convertReference(src.getResource())); 679 return tgt; 680 } 681 682 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.r4.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 683 if (src == null || src.isEmpty()) 684 return null; 685 org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent(); 686 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 687 if (src.hasAutocreateElement()) 688 tgt.setAutocreateElement(Boolean10_40.convertBoolean(src.getAutocreateElement())); 689 if (src.hasAutodeleteElement()) 690 tgt.setAutodeleteElement(Boolean10_40.convertBoolean(src.getAutodeleteElement())); 691 if (src.hasResource()) 692 tgt.setResource(Reference10_40.convertReference(src.getResource())); 693 return tgt; 694 } 695 696 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 697 if (src == null || src.isEmpty()) 698 return null; 699 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent(); 700 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 701 if (src.hasRequiredElement()) 702 tgt.setRequiredElement(Boolean10_40.convertBoolean(src.getRequiredElement())); 703 if (src.hasValidatedElement()) 704 tgt.setValidatedElement(Boolean10_40.convertBoolean(src.getValidatedElement())); 705 if (src.hasDescriptionElement()) 706 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 707 if (src.hasDestinationElement()) 708 tgt.setDestinationElement(Integer10_40.convertInteger(src.getDestinationElement())); 709 for (org.hl7.fhir.r4.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 710 if (src.hasCapabilitiesElement()) 711 tgt.setConformance(Canonical10_40.convertCanonicalToReference(src.getCapabilitiesElement())); 712 return tgt; 713 } 714 715 public static org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 716 if (src == null || src.isEmpty()) 717 return null; 718 org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent(); 719 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 720 if (src.hasRequiredElement()) 721 tgt.setRequiredElement(Boolean10_40.convertBoolean(src.getRequiredElement())); 722 if (src.hasValidatedElement()) 723 tgt.setValidatedElement(Boolean10_40.convertBoolean(src.getValidatedElement())); 724 if (src.hasDescriptionElement()) 725 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 726 if (src.hasDestinationElement()) 727 tgt.setDestinationElement(Integer10_40.convertInteger(src.getDestinationElement())); 728 for (org.hl7.fhir.dstu2.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 729 if (src.hasConformance()) 730 tgt.setCapabilitiesElement(Canonical10_40.convertReferenceToCanonical(src.getConformance())); 731 return tgt; 732 } 733 734 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 735 if (src == null || src.isEmpty()) 736 return null; 737 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent(); 738 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 739 for (org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 740 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 741 for (org.hl7.fhir.r4.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 742 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 743 return tgt; 744 } 745 746 public static org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 747 if (src == null || src.isEmpty()) 748 return null; 749 org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptMetadataComponent(); 750 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 751 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 752 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 753 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 754 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 755 return tgt; 756 } 757 758 public static org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 759 if (src == null || src.isEmpty()) 760 return null; 761 org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent(); 762 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 763 if (src.hasUrlElement()) 764 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 765 if (src.hasDescriptionElement()) 766 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 767 return tgt; 768 } 769 770 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.r4.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 771 if (src == null || src.isEmpty()) 772 return null; 773 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent(); 774 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 775 if (src.hasUrlElement()) 776 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 777 if (src.hasDescriptionElement()) 778 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 779 return tgt; 780 } 781 782 public static org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 783 if (src == null || src.isEmpty()) 784 return null; 785 org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent(); 786 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 787 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent t : src.getAction()) 788 tgt.addAction(convertSetupActionComponent(t)); 789 return tgt; 790 } 791 792 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.r4.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 793 if (src == null || src.isEmpty()) 794 return null; 795 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent(); 796 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 797 for (org.hl7.fhir.r4.model.TestScript.SetupActionComponent t : src.getAction()) 798 tgt.addAction(convertSetupActionComponent(t)); 799 return tgt; 800 } 801 802 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 803 if (src == null || src.isEmpty()) 804 return null; 805 org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent(); 806 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 807 for (org.hl7.fhir.r4.model.TestScript.TeardownActionComponent t : src.getAction()) 808 tgt.addAction(convertTeardownActionComponent(t)); 809 return tgt; 810 } 811 812 public static org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 813 if (src == null || src.isEmpty()) 814 return null; 815 org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptTeardownComponent(); 816 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 817 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent t : src.getAction()) 818 tgt.addAction(convertTeardownActionComponent(t)); 819 return tgt; 820 } 821 822 public static org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent src) throws FHIRException { 823 if (src == null || src.isEmpty()) 824 return null; 825 org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent(); 826 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 827 if (src.hasNameElement()) 828 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 829 if (src.hasDescriptionElement()) 830 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 831 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent t : src.getAction()) 832 tgt.addAction(convertTestActionComponent(t)); 833 return tgt; 834 } 835 836 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.r4.model.TestScript.TestScriptTestComponent src) throws FHIRException { 837 if (src == null || src.isEmpty()) 838 return null; 839 org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent(); 840 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 841 if (src.hasNameElement()) 842 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 843 if (src.hasDescriptionElement()) 844 tgt.setDescriptionElement(String10_40.convertString(src.getDescriptionElement())); 845 for (org.hl7.fhir.r4.model.TestScript.TestActionComponent t : src.getAction()) 846 tgt.addAction(convertTestActionComponent(t)); 847 return tgt; 848 } 849 850 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 851 if (src == null || src.isEmpty()) 852 return null; 853 org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent(); 854 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 855 if (src.hasNameElement()) 856 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 857 if (src.hasHeaderFieldElement()) 858 tgt.setHeaderFieldElement(String10_40.convertString(src.getHeaderFieldElement())); 859 if (src.hasPathElement()) 860 tgt.setPathElement(String10_40.convertString(src.getPathElement())); 861 if (src.hasSourceIdElement()) 862 tgt.setSourceIdElement(Id10_40.convertId(src.getSourceIdElement())); 863 return tgt; 864 } 865 866 public static org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 867 if (src == null || src.isEmpty()) 868 return null; 869 org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.r4.model.TestScript.TestScriptVariableComponent(); 870 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 871 if (src.hasNameElement()) 872 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 873 if (src.hasHeaderFieldElement()) 874 tgt.setHeaderFieldElement(String10_40.convertString(src.getHeaderFieldElement())); 875 if (src.hasPathElement()) 876 tgt.setPathElement(String10_40.convertString(src.getPathElement())); 877 if (src.hasSourceIdElement()) 878 tgt.setSourceIdElement(Id10_40.convertId(src.getSourceIdElement())); 879 return tgt; 880 } 881}