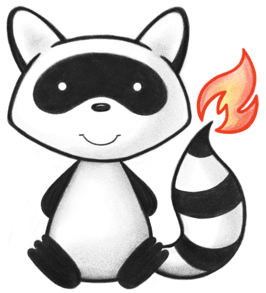
001package org.hl7.fhir.convertors.conv10_40.resources10_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_40; 006import org.hl7.fhir.convertors.context.ConversionContext10_40; 007import org.hl7.fhir.convertors.conv10_40.VersionConvertor_10_40; 008import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.CodeableConcept10_40; 009import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Coding10_40; 010import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.ContactPoint10_40; 011import org.hl7.fhir.convertors.conv10_40.datatypes10_40.complextypes10_40.Identifier10_40; 012import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Boolean10_40; 013import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Code10_40; 014import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.DateTime10_40; 015import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Integer10_40; 016import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.String10_40; 017import org.hl7.fhir.convertors.conv10_40.datatypes10_40.primitivetypes10_40.Uri10_40; 018import org.hl7.fhir.dstu2.model.ValueSet; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r4.model.BooleanType; 021import org.hl7.fhir.r4.model.CodeSystem; 022import org.hl7.fhir.r4.model.CodeSystem.CodeSystemContentMode; 023import org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionComponent; 024import org.hl7.fhir.r4.terminologies.CodeSystemUtilities; 025 026public class ValueSet10_40 { 027 028 public static org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 029 if (src == null || src.isEmpty()) 030 return null; 031 org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent(); 032 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 033 if (src.hasCodeElement()) 034 tgt.setCodeElement(Code10_40.convertCode(src.getCodeElement())); 035 if (src.hasDisplayElement()) 036 tgt.setDisplayElement(String10_40.convertString(src.getDisplayElement())); 037 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent t : src.getDesignation()) 038 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 039 return tgt; 040 } 041 042 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 043 if (src == null || src.isEmpty()) 044 return null; 045 org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent(); 046 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 047 if (src.hasCodeElement()) 048 tgt.setCodeElement(Code10_40.convertCode(src.getCodeElement())); 049 if (src.hasDisplayElement()) 050 tgt.setDisplayElement(String10_40.convertString(src.getDisplayElement())); 051 for (org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 052 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent(); 060 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 061 if (src.hasLanguageElement()) 062 tgt.setLanguageElement(Code10_40.convertCode(src.getLanguageElement())); 063 if (src.hasUse()) 064 tgt.setUse(Coding10_40.convertCoding(src.getUse())); 065 if (src.hasValueElement()) 066 tgt.setValueElement(String10_40.convertString(src.getValueElement())); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 071 if (src == null || src.isEmpty()) 072 return null; 073 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent(); 074 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 075 if (src.hasLanguageElement()) 076 tgt.setLanguageElement(Code10_40.convertCode(src.getLanguageElement())); 077 if (src.hasUse()) 078 tgt.setUse(Coding10_40.convertCoding(src.getUse())); 079 if (src.hasValueElement()) 080 tgt.setValueElement(String10_40.convertString(src.getValueElement())); 081 return tgt; 082 } 083 084 public static org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent src) throws FHIRException { 085 if (src == null || src.isEmpty()) 086 return null; 087 org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent(); 088 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 089 if (src.hasSystemElement()) 090 tgt.setSystemElement(Uri10_40.convertUri(src.getSystemElement())); 091 if (src.hasVersionElement()) 092 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 093 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 094 tgt.addConcept(convertConceptReferenceComponent(t)); 095 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 096 tgt.addFilter(convertConceptSetFilterComponent(t)); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent(); 104 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 105 if (src.hasSystemElement()) 106 tgt.setSystemElement(Uri10_40.convertUri(src.getSystemElement())); 107 if (src.hasVersionElement()) 108 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 109 for (org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 110 tgt.addConcept(convertConceptReferenceComponent(t)); 111 for (org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 112 tgt.addFilter(convertConceptSetFilterComponent(t)); 113 return tgt; 114 } 115 116 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent(); 120 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 121 if (src.hasPropertyElement()) 122 tgt.setPropertyElement(Code10_40.convertCode(src.getPropertyElement())); 123 if (src.hasOp()) 124 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 125 if (src.hasValue()) 126 tgt.setValue(src.getValue()); 127 return tgt; 128 } 129 130 public static org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent(); 134 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 135 if (src.hasPropertyElement()) 136 tgt.setPropertyElement(Code10_40.convertCode(src.getPropertyElement())); 137 if (src.hasOp()) 138 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 139 if (src.hasValue()) 140 tgt.setValue(src.getValue()); 141 return tgt; 142 } 143 144 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ValueSet.FilterOperator> src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ValueSet.FilterOperator> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ValueSet.FilterOperatorEnumFactory()); 148 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 149 switch (src.getValue()) { 150 case EQUAL: 151 tgt.setValue(org.hl7.fhir.r4.model.ValueSet.FilterOperator.EQUAL); 152 break; 153 case ISA: 154 tgt.setValue(org.hl7.fhir.r4.model.ValueSet.FilterOperator.ISA); 155 break; 156 case ISNOTA: 157 tgt.setValue(org.hl7.fhir.r4.model.ValueSet.FilterOperator.ISNOTA); 158 break; 159 case REGEX: 160 tgt.setValue(org.hl7.fhir.r4.model.ValueSet.FilterOperator.REGEX); 161 break; 162 case IN: 163 tgt.setValue(org.hl7.fhir.r4.model.ValueSet.FilterOperator.IN); 164 break; 165 case NOTIN: 166 tgt.setValue(org.hl7.fhir.r4.model.ValueSet.FilterOperator.NOTIN); 167 break; 168 default: 169 tgt.setValue(org.hl7.fhir.r4.model.ValueSet.FilterOperator.NULL); 170 break; 171 } 172 return tgt; 173 } 174 175 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ValueSet.FilterOperator> src) throws FHIRException { 176 if (src == null || src.isEmpty()) 177 return null; 178 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ValueSet.FilterOperator> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ValueSet.FilterOperatorEnumFactory()); 179 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 180 switch (src.getValue()) { 181 case EQUAL: 182 tgt.setValue(org.hl7.fhir.dstu2.model.ValueSet.FilterOperator.EQUAL); 183 break; 184 case ISA: 185 tgt.setValue(org.hl7.fhir.dstu2.model.ValueSet.FilterOperator.ISA); 186 break; 187 case ISNOTA: 188 tgt.setValue(org.hl7.fhir.dstu2.model.ValueSet.FilterOperator.ISNOTA); 189 break; 190 case REGEX: 191 tgt.setValue(org.hl7.fhir.dstu2.model.ValueSet.FilterOperator.REGEX); 192 break; 193 case IN: 194 tgt.setValue(org.hl7.fhir.dstu2.model.ValueSet.FilterOperator.IN); 195 break; 196 case NOTIN: 197 tgt.setValue(org.hl7.fhir.dstu2.model.ValueSet.FilterOperator.NOTIN); 198 break; 199 default: 200 tgt.setValue(org.hl7.fhir.dstu2.model.ValueSet.FilterOperator.NULL); 201 break; 202 } 203 return tgt; 204 } 205 206 public static org.hl7.fhir.r4.model.ValueSet convertValueSet(org.hl7.fhir.dstu2.model.ValueSet src) throws FHIRException { 207 return convertValueSet(src, null); 208 } 209 210 public static org.hl7.fhir.r4.model.ValueSet convertValueSet(org.hl7.fhir.dstu2.model.ValueSet src, BaseAdvisor_10_40 advisor) throws FHIRException { 211 if (src == null || src.isEmpty()) 212 return null; 213 org.hl7.fhir.r4.model.ValueSet tgt = new org.hl7.fhir.r4.model.ValueSet(); 214 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 215 if (src.hasUrlElement()) 216 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 217 if (src.hasIdentifier()) 218 tgt.addIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 219 if (src.hasVersionElement()) 220 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 221 if (src.hasNameElement()) 222 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 223 if (src.hasStatus()) 224 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 225 if (src.hasExperimental()) 226 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 227 if (src.hasPublisherElement()) 228 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 229 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent t : src.getContact()) 230 tgt.addContact(convertValueSetContactComponent(t)); 231 if (src.hasDate()) 232 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 233 if (src.hasDescription()) 234 tgt.setDescription(src.getDescription()); 235 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 236 if (VersionConvertor_10_40.isJurisdiction(t)) 237 tgt.addJurisdiction(CodeableConcept10_40.convertCodeableConcept(t)); 238 else 239 tgt.addUseContext(CodeableConcept10_40.convertCodeableConceptToUsageContext(t)); 240 if (src.hasImmutableElement()) 241 tgt.setImmutableElement(Boolean10_40.convertBoolean(src.getImmutableElement())); 242 if (src.hasRequirements()) 243 tgt.setPurpose(src.getRequirements()); 244 if (src.hasCopyright()) 245 tgt.setCopyright(src.getCopyright()); 246 if (src.hasExtensible()) 247 tgt.addExtension("http://hl7.org/fhir/StructureDefinition/valueset-extensible", new BooleanType(src.getExtensible())); 248 if (src.hasCompose()) { 249 if (src.hasCompose()) 250 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 251 tgt.getCompose().setLockedDate(src.getLockedDate()); 252 } 253 if (src.hasCodeSystem() && advisor != null) { 254 org.hl7.fhir.r4.model.CodeSystem tgtcs = new org.hl7.fhir.r4.model.CodeSystem(); 255 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgtcs); 256 tgtcs.setUrl(src.getCodeSystem().getSystem()); 257 tgtcs.addIdentifier(Identifier10_40.convertIdentifier(src.getIdentifier())); 258 tgtcs.setVersion(src.getCodeSystem().getVersion()); 259 tgtcs.setName(src.getName() + " Code System"); 260 tgtcs.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 261 if (src.hasExperimental()) 262 tgtcs.setExperimental(src.getExperimental()); 263 tgtcs.setPublisher(src.getPublisher()); 264 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent t : src.getContact()) 265 tgtcs.addContact(convertValueSetContactComponent(t)); 266 if (src.hasDate()) 267 tgtcs.setDate(src.getDate()); 268 tgtcs.setDescription(src.getDescription()); 269 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 270 if (VersionConvertor_10_40.isJurisdiction(t)) 271 tgtcs.addJurisdiction(CodeableConcept10_40.convertCodeableConcept(t)); 272 else 273 tgtcs.addUseContext(CodeableConcept10_40.convertCodeableConceptToUsageContext(t)); 274 tgtcs.setPurpose(src.getRequirements()); 275 tgtcs.setCopyright(src.getCopyright()); 276 tgtcs.setContent(CodeSystemContentMode.COMPLETE); 277 tgtcs.setCaseSensitive(src.getCodeSystem().getCaseSensitive()); 278 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent cs : src.getCodeSystem().getConcept()) 279 processConcept(tgtcs.getConcept(), cs, tgtcs); 280 advisor.handleCodeSystem(tgtcs, tgt); 281 tgt.setUserData("r2-cs", tgtcs); 282 tgt.getCompose().addInclude().setSystem(tgtcs.getUrl()); 283 } 284 if (src.hasExpansion()) 285 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 286 return tgt; 287 } 288 289 public static org.hl7.fhir.dstu2.model.ValueSet convertValueSet(org.hl7.fhir.r4.model.ValueSet src, BaseAdvisor_10_40 advisor) throws FHIRException { 290 if (src == null || src.isEmpty()) 291 return null; 292 org.hl7.fhir.dstu2.model.ValueSet tgt = new org.hl7.fhir.dstu2.model.ValueSet(); 293 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyDomainResource(src, tgt); 294 if (src.hasUrlElement()) 295 tgt.setUrlElement(Uri10_40.convertUri(src.getUrlElement())); 296 for (org.hl7.fhir.r4.model.Identifier i : src.getIdentifier()) 297 tgt.setIdentifier(Identifier10_40.convertIdentifier(i)); 298 if (src.hasVersionElement()) 299 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 300 if (src.hasNameElement()) 301 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 302 if (src.hasStatus()) 303 tgt.setStatusElement(Enumerations10_40.convertConformanceResourceStatus(src.getStatusElement())); 304 if (src.hasExperimental()) 305 tgt.setExperimentalElement(Boolean10_40.convertBoolean(src.getExperimentalElement())); 306 if (src.hasPublisherElement()) 307 tgt.setPublisherElement(String10_40.convertString(src.getPublisherElement())); 308 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) tgt.addContact(convertValueSetContactComponent(t)); 309 if (src.hasDate()) 310 tgt.setDateElement(DateTime10_40.convertDateTime(src.getDateElement())); 311 tgt.setLockedDate(src.getCompose().getLockedDate()); 312 if (src.hasDescription()) 313 tgt.setDescription(src.getDescription()); 314 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 315 if (t.hasValueCodeableConcept()) 316 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t.getValueCodeableConcept())); 317 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 318 tgt.addUseContext(CodeableConcept10_40.convertCodeableConcept(t)); 319 if (src.hasImmutableElement()) 320 tgt.setImmutableElement(Boolean10_40.convertBoolean(src.getImmutableElement())); 321 if (src.hasPurpose()) 322 tgt.setRequirements(src.getPurpose()); 323 if (src.hasCopyright()) 324 tgt.setCopyright(src.getCopyright()); 325 if (src.hasExtension("http://hl7.org/fhir/StructureDefinition/valueset-extensible")) 326 tgt.setExtensible(((BooleanType) src.getExtensionByUrl("http://hl7.org/fhir/StructureDefinition/valueset-extensible").getValue()).booleanValue()); 327 org.hl7.fhir.r4.model.CodeSystem srcCS = (CodeSystem) src.getUserData("r2-cs"); 328 if (srcCS == null) 329 srcCS = advisor.getCodeSystem(src); 330 if (srcCS != null) { 331 tgt.getCodeSystem().setSystem(srcCS.getUrl()); 332 tgt.getCodeSystem().setVersion(srcCS.getVersion()); 333 tgt.getCodeSystem().setCaseSensitive(srcCS.getCaseSensitive()); 334 for (org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionComponent cs : srcCS.getConcept()) 335 processConcept(tgt.getCodeSystem().getConcept(), cs, srcCS); 336 } 337 if (src.hasCompose()) 338 tgt.setCompose(convertValueSetComposeComponent(src.getCompose(), srcCS == null ? null : srcCS.getUrl())); 339 if (src.hasExpansion()) 340 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 341 return tgt; 342 } 343 344 public static org.hl7.fhir.dstu2.model.ValueSet convertValueSet(org.hl7.fhir.r4.model.ValueSet src) throws FHIRException { 345 return convertValueSet(src, null); 346 } 347 348 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent src, String noSystem) throws FHIRException { 349 if (src == null || src.isEmpty()) 350 return null; 351 org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent(); 352 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 353 for (org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent t : src.getInclude()) { 354 for (org.hl7.fhir.r4.model.UriType ti : t.getValueSet()) tgt.addImport(ti.getValue()); 355 if (noSystem == null || !t.getSystem().equals(noSystem)) 356 tgt.addInclude(convertConceptSetComponent(t)); 357 } 358 for (org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent t : src.getExclude()) 359 tgt.addExclude(convertConceptSetComponent(t)); 360 return tgt; 361 } 362 363 public static org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 364 if (src == null || src.isEmpty()) 365 return null; 366 org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent(); 367 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 368 for (org.hl7.fhir.dstu2.model.UriType t : src.getImport()) tgt.addInclude().addValueSet(t.getValue()); 369 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent t : src.getInclude()) 370 tgt.addInclude(convertConceptSetComponent(t)); 371 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent t : src.getExclude()) 372 tgt.addExclude(convertConceptSetComponent(t)); 373 return tgt; 374 } 375 376 public static org.hl7.fhir.r4.model.ContactDetail convertValueSetContactComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent src) throws FHIRException { 377 if (src == null || src.isEmpty()) 378 return null; 379 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 380 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 381 if (src.hasNameElement()) 382 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 383 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 384 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 385 return tgt; 386 } 387 388 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent convertValueSetContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 389 if (src == null || src.isEmpty()) 390 return null; 391 org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent(); 392 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 393 if (src.hasNameElement()) 394 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 395 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 396 tgt.addTelecom(ContactPoint10_40.convertContactPoint(t)); 397 return tgt; 398 } 399 400 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 401 if (src == null || src.isEmpty()) 402 return null; 403 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent(); 404 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 405 if (src.hasIdentifierElement()) 406 tgt.setIdentifierElement(Uri10_40.convertUri(src.getIdentifierElement())); 407 if (src.hasTimestampElement()) 408 tgt.setTimestampElement(DateTime10_40.convertDateTime(src.getTimestampElement())); 409 if (src.hasTotalElement()) 410 tgt.setTotalElement(Integer10_40.convertInteger(src.getTotalElement())); 411 if (src.hasOffsetElement()) 412 tgt.setOffsetElement(Integer10_40.convertInteger(src.getOffsetElement())); 413 for (org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 414 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 415 for (org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 416 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 417 return tgt; 418 } 419 420 public static org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 421 if (src == null || src.isEmpty()) 422 return null; 423 org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent(); 424 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 425 if (src.hasIdentifierElement()) 426 tgt.setIdentifierElement(Uri10_40.convertUri(src.getIdentifierElement())); 427 if (src.hasTimestampElement()) 428 tgt.setTimestampElement(DateTime10_40.convertDateTime(src.getTimestampElement())); 429 if (src.hasTotalElement()) 430 tgt.setTotalElement(Integer10_40.convertInteger(src.getTotalElement())); 431 if (src.hasOffsetElement()) 432 tgt.setOffsetElement(Integer10_40.convertInteger(src.getOffsetElement())); 433 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 434 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 435 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 436 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 437 return tgt; 438 } 439 440 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 441 if (src == null || src.isEmpty()) 442 return null; 443 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent(); 444 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 445 if (src.hasSystemElement()) 446 tgt.setSystemElement(Uri10_40.convertUri(src.getSystemElement())); 447 if (src.hasAbstractElement()) 448 tgt.setAbstractElement(Boolean10_40.convertBoolean(src.getAbstractElement())); 449 if (src.hasVersionElement()) 450 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 451 if (src.hasCodeElement()) 452 tgt.setCodeElement(Code10_40.convertCode(src.getCodeElement())); 453 if (src.hasDisplayElement()) 454 tgt.setDisplayElement(String10_40.convertString(src.getDisplayElement())); 455 for (org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 456 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 457 return tgt; 458 } 459 460 public static org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 461 if (src == null || src.isEmpty()) 462 return null; 463 org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent(); 464 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 465 if (src.hasSystemElement()) 466 tgt.setSystemElement(Uri10_40.convertUri(src.getSystemElement())); 467 if (src.hasAbstractElement()) 468 tgt.setAbstractElement(Boolean10_40.convertBoolean(src.getAbstractElement())); 469 if (src.hasVersionElement()) 470 tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 471 if (src.hasCodeElement()) 472 tgt.setCodeElement(Code10_40.convertCode(src.getCodeElement())); 473 if (src.hasDisplayElement()) 474 tgt.setDisplayElement(String10_40.convertString(src.getDisplayElement())); 475 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 476 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 477 return tgt; 478 } 479 480 public static org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 481 if (src == null || src.isEmpty()) 482 return null; 483 org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent(); 484 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 485 if (src.hasNameElement()) 486 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 487 if (src.hasValue()) 488 tgt.setValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getValue())); 489 return tgt; 490 } 491 492 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 493 if (src == null || src.isEmpty()) 494 return null; 495 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent(); 496 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 497 if (src.hasNameElement()) 498 tgt.setNameElement(String10_40.convertString(src.getNameElement())); 499 if (src.hasValue()) 500 tgt.setValue(ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().convertType(src.getValue())); 501 return tgt; 502 } 503 504 static public void processConcept(List<ConceptDefinitionComponent> concepts, org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent cs, CodeSystem tgtcs) throws FHIRException { 505 org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionComponent ct = new org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionComponent(); 506 concepts.add(ct); 507 ct.setCode(cs.getCode()); 508 ct.setDisplay(cs.getDisplay()); 509 ct.setDefinition(cs.getDefinition()); 510 if (cs.getAbstract()) 511 CodeSystemUtilities.setNotSelectable(tgtcs, ct); 512 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent csd : cs.getDesignation()) { 513 org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionDesignationComponent cst = new org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionDesignationComponent(); 514 cst.setLanguage(csd.getLanguage()); 515 cst.setUse(Coding10_40.convertCoding(csd.getUse())); 516 cst.setValue(csd.getValue()); 517 } 518 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent csc : cs.getConcept()) 519 processConcept(ct.getConcept(), csc, tgtcs); 520 } 521 522 static public void processConcept(List<ValueSet.ConceptDefinitionComponent> concepts, ConceptDefinitionComponent cs, CodeSystem srcCS) throws FHIRException { 523 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent ct = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent(); 524 concepts.add(ct); 525 ct.setCode(cs.getCode()); 526 ct.setDisplay(cs.getDisplay()); 527 ct.setDefinition(cs.getDefinition()); 528 if (CodeSystemUtilities.isNotSelectable(srcCS, cs)) 529 ct.setAbstract(true); 530 for (org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionDesignationComponent csd : cs.getDesignation()) { 531 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent cst = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent(); 532 cst.setLanguage(csd.getLanguage()); 533 cst.setUse(Coding10_40.convertCoding(csd.getUse())); 534 cst.setValue(csd.getValue()); 535 } 536 for (ConceptDefinitionComponent csc : cs.getConcept()) processConcept(ct.getConcept(), csc, srcCS); 537 } 538 539 public static ValueSet.ValueSetCodeSystemComponent convertCodeSystem(CodeSystem src) throws FHIRException { 540 if (src == null || src.isEmpty()) return null; 541 ValueSet.ValueSetCodeSystemComponent tgt = new ValueSet.ValueSetCodeSystemComponent(); 542 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyElement(src, tgt); 543 if (src.hasUrlElement()) tgt.setSystemElement(Uri10_40.convertUri(src.getUrlElement())); 544 if (src.hasVersionElement()) tgt.setVersionElement(String10_40.convertString(src.getVersionElement())); 545 if (src.hasCaseSensitiveElement()) 546 tgt.setCaseSensitiveElement(Boolean10_40.convertBoolean(src.getCaseSensitiveElement())); 547 for (ConceptDefinitionComponent cc : src.getConcept()) tgt.addConcept(convertCodeSystemConcept(src, cc)); 548 return tgt; 549 } 550 551 public static ValueSet.ConceptDefinitionComponent convertCodeSystemConcept(CodeSystem cs, ConceptDefinitionComponent src) throws FHIRException { 552 if (src == null || src.isEmpty()) return null; 553 ValueSet.ConceptDefinitionComponent tgt = new ValueSet.ConceptDefinitionComponent(); 554 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 555 tgt.setAbstract(CodeSystemUtilities.isNotSelectable(cs, src)); 556 tgt.setCode(src.getCode()); 557 tgt.setDefinition(src.getDefinition()); 558 tgt.setDisplay(src.getDisplay()); 559 for (ConceptDefinitionComponent cc : src.getConcept()) tgt.addConcept(convertCodeSystemConcept(cs, cc)); 560 for (CodeSystem.ConceptDefinitionDesignationComponent cc : src.getDesignation()) 561 tgt.addDesignation(convertCodeSystemDesignation(cc)); 562 return tgt; 563 } 564 565 public static ValueSet.ConceptDefinitionDesignationComponent convertCodeSystemDesignation(CodeSystem.ConceptDefinitionDesignationComponent src) throws FHIRException { 566 if (src == null || src.isEmpty()) return null; 567 ValueSet.ConceptDefinitionDesignationComponent tgt = new ValueSet.ConceptDefinitionDesignationComponent(); 568 ConversionContext10_40.INSTANCE.getVersionConvertor_10_40().copyBackboneElement(src,tgt); 569 tgt.setUse(Coding10_40.convertCoding(src.getUse())); 570 tgt.setLanguage(src.getLanguage()); 571 tgt.setValue(src.getValue()); 572 return tgt; 573 } 574}