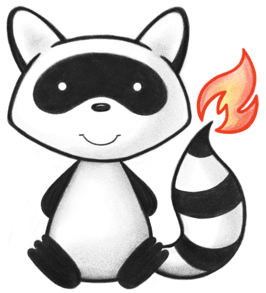
001package org.hl7.fhir.convertors.conv10_50.datatypes10_50; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 008import org.hl7.fhir.dstu2.model.Reference; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r5.model.CanonicalType; 011import org.hl7.fhir.r5.model.Immunization; 012 013public class Reference10_50 { 014 public static org.hl7.fhir.r5.model.Reference convertReference(org.hl7.fhir.dstu2.model.Reference src) throws FHIRException { 015 if (src == null || src.isEmpty()) return null; 016 org.hl7.fhir.r5.model.Reference tgt = new org.hl7.fhir.r5.model.Reference(); 017 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 018 tgt.setReference(src.getReference()); 019 if (src.hasDisplayElement()) tgt.setDisplayElement(String10_50.convertString(src.getDisplayElement())); 020 return tgt; 021 } 022 023 public static org.hl7.fhir.dstu2.model.Reference convertReference(org.hl7.fhir.r5.model.Reference src) throws FHIRException { 024 if (src == null || src.isEmpty()) return null; 025 org.hl7.fhir.dstu2.model.Reference tgt = new org.hl7.fhir.dstu2.model.Reference(); 026 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 027 tgt.setReference(src.getReference()); 028 if (src.hasDisplayElement()) tgt.setDisplayElement(String10_50.convertString(src.getDisplayElement())); 029 return tgt; 030 } 031 032 static public CanonicalType convertReferenceToCanonical(Reference src) throws FHIRException { 033 CanonicalType dst = new CanonicalType(src.getReference()); 034 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, dst); 035 return dst; 036 } 037 038 static public Reference convertCanonicalToReference(CanonicalType src) throws FHIRException { 039 Reference dst = new Reference(src.getValue()); 040 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, dst); 041 return dst; 042 } 043 044 static public org.hl7.fhir.r5.model.Reference getPerformer(List<Immunization.ImmunizationPerformerComponent> practitioner) { 045 for (Immunization.ImmunizationPerformerComponent p : practitioner) { 046 if (CodeableConcept10_50.hasConcept(p.getFunction(), "http://hl7.org/fhir/v2/0443", "AP")) return p.getActor(); 047 } 048 return null; 049 } 050 051 static public org.hl7.fhir.r5.model.Reference getRequester(List<Immunization.ImmunizationPerformerComponent> practitioner) { 052 for (Immunization.ImmunizationPerformerComponent p : practitioner) { 053 if (CodeableConcept10_50.hasConcept(p.getFunction(), "http://hl7.org/fhir/v2/0443", "OP")) return p.getActor(); 054 } 055 return null; 056 } 057}