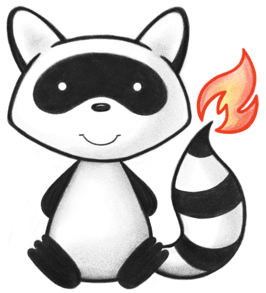
001package org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 005import org.hl7.fhir.dstu2.model.CodeableConcept; 006import org.hl7.fhir.exceptions.FHIRException; 007import org.hl7.fhir.r5.model.CodeableReference; 008 009public class CodeableConcept10_50 { 010 public static org.hl7.fhir.r5.model.CodeableConcept convertCodeableConcept(org.hl7.fhir.dstu2.model.CodeableConcept src) throws FHIRException { 011 if (src == null || src.isEmpty()) return null; 012 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 013 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 014 for (org.hl7.fhir.dstu2.model.Coding t : src.getCoding()) tgt.addCoding(Coding10_50.convertCoding(t)); 015 if (src.hasTextElement()) tgt.setTextElement(String10_50.convertString(src.getTextElement())); 016 return tgt; 017 } 018 019 public static org.hl7.fhir.dstu2.model.CodeableConcept convertCodeableConcept(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 020 if (src == null || src.isEmpty()) return null; 021 org.hl7.fhir.dstu2.model.CodeableConcept tgt = new org.hl7.fhir.dstu2.model.CodeableConcept(); 022 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 023 for (org.hl7.fhir.r5.model.Coding t : src.getCoding()) tgt.addCoding(Coding10_50.convertCoding(t)); 024 if (src.hasTextElement()) tgt.setTextElement(String10_50.convertString(src.getTextElement())); 025 return tgt; 026 } 027 028 static public CodeableReference convertCodeableConceptToCodableReference(CodeableConcept src) { 029 CodeableReference tgt = new CodeableReference(); 030 tgt.setConcept(convertCodeableConcept(src)); 031 return tgt; 032 } 033 034 public static org.hl7.fhir.r5.model.UsageContext convertCodeableConceptToUsageContext(CodeableConcept t) throws FHIRException { 035 org.hl7.fhir.r5.model.UsageContext result = new org.hl7.fhir.r5.model.UsageContext(); 036 result.setValue(convertCodeableConcept(t)); 037 return result; 038 } 039 040 static public boolean hasConcept(org.hl7.fhir.r5.model.CodeableConcept cc, String system, String code) { 041 for (org.hl7.fhir.r5.model.Coding c : cc.getCoding()) { 042 if (system.equals(c.getSystem()) && code.equals(c.getCode())) return true; 043 } 044 return false; 045 } 046 047 static public boolean hasConcept(CodeableConcept cc, String system, String code) { 048 for (org.hl7.fhir.dstu2.model.Coding c : cc.getCoding()) { 049 if (system.equals(c.getSystem()) && code.equals(c.getCode())) return true; 050 } 051 return false; 052 } 053}