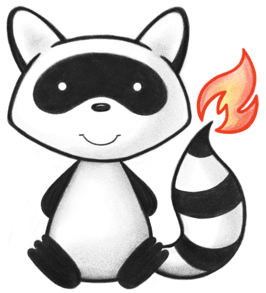
001package org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.PositiveInt10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class ContactPoint10_50 { 009 public static org.hl7.fhir.r5.model.ContactPoint convertContactPoint(org.hl7.fhir.dstu2.model.ContactPoint src) throws FHIRException { 010 if (src == null || src.isEmpty()) return null; 011 org.hl7.fhir.r5.model.ContactPoint tgt = new org.hl7.fhir.r5.model.ContactPoint(); 012 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 013 if (src.hasSystem()) tgt.setSystemElement(convertContactPointSystem(src.getSystemElement())); 014 if (src.hasValueElement()) tgt.setValueElement(String10_50.convertString(src.getValueElement())); 015 if (src.hasUse()) tgt.setUseElement(convertContactPointUse(src.getUseElement())); 016 if (src.hasRankElement()) tgt.setRankElement(PositiveInt10_50.convertPositiveInt(src.getRankElement())); 017 if (src.hasPeriod()) tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 018 return tgt; 019 } 020 021 public static org.hl7.fhir.dstu2.model.ContactPoint convertContactPoint(org.hl7.fhir.r5.model.ContactPoint src) throws FHIRException { 022 if (src == null || src.isEmpty()) return null; 023 org.hl7.fhir.dstu2.model.ContactPoint tgt = new org.hl7.fhir.dstu2.model.ContactPoint(); 024 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 025 if (src.hasSystem()) tgt.setSystemElement(convertContactPointSystem(src.getSystemElement())); 026 if (src.hasValueElement()) tgt.setValueElement(String10_50.convertString(src.getValueElement())); 027 if (src.hasUse()) tgt.setUseElement(convertContactPointUse(src.getUseElement())); 028 if (src.hasRankElement()) tgt.setRankElement(PositiveInt10_50.convertPositiveInt(src.getRankElement())); 029 if (src.hasPeriod()) tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 030 return tgt; 031 } 032 033 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem> convertContactPointSystem(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem> src) throws FHIRException { 034 if (src == null || src.isEmpty()) return null; 035 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ContactPoint.ContactPointSystemEnumFactory()); 036 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 037 if (src.getValue() == null) { 038 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem.NULL); 039 } else { 040 switch (src.getValue()) { 041 case PHONE: 042 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem.PHONE); 043 break; 044 case FAX: 045 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem.FAX); 046 break; 047 case EMAIL: 048 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem.EMAIL); 049 break; 050 case PAGER: 051 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem.PAGER); 052 break; 053 case OTHER: 054 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem.OTHER); 055 break; 056 default: 057 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem.NULL); 058 break; 059 } 060 } 061 return tgt; 062 } 063 064 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem> convertContactPointSystem(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem> src) throws FHIRException { 065 if (src == null || src.isEmpty()) return null; 066 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystemEnumFactory()); 067 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 068 if (src.getValue() == null) { 069 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.NULL); 070 } else { 071 switch (src.getValue()) { 072 case PHONE: 073 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.PHONE); 074 break; 075 case FAX: 076 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.FAX); 077 break; 078 case EMAIL: 079 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.EMAIL); 080 break; 081 case PAGER: 082 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.PAGER); 083 break; 084 case OTHER: 085 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.OTHER); 086 break; 087 case URL: 088 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.OTHER); 089 break; 090 default: 091 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem.NULL); 092 break; 093 } 094 } 095 return tgt; 096 } 097 098 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ContactPoint.ContactPointUse> convertContactPointUse(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse> src) throws FHIRException { 099 if (src == null || src.isEmpty()) return null; 100 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ContactPoint.ContactPointUse> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ContactPoint.ContactPointUseEnumFactory()); 101 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 102 if (src.getValue() == null) { 103 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointUse.NULL); 104 } else { 105 switch (src.getValue()) { 106 case HOME: 107 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointUse.HOME); 108 break; 109 case WORK: 110 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointUse.WORK); 111 break; 112 case TEMP: 113 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointUse.TEMP); 114 break; 115 case OLD: 116 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointUse.OLD); 117 break; 118 case MOBILE: 119 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointUse.MOBILE); 120 break; 121 default: 122 tgt.setValue(org.hl7.fhir.r5.model.ContactPoint.ContactPointUse.NULL); 123 break; 124 } 125 } 126 return tgt; 127 } 128 129 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse> convertContactPointUse(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ContactPoint.ContactPointUse> src) throws FHIRException { 130 if (src == null || src.isEmpty()) return null; 131 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUseEnumFactory()); 132 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 133 if (src.getValue() == null) { 134 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse.NULL); 135 } else { 136 switch (src.getValue()) { 137 case HOME: 138 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse.HOME); 139 break; 140 case WORK: 141 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse.WORK); 142 break; 143 case TEMP: 144 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse.TEMP); 145 break; 146 case OLD: 147 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse.OLD); 148 break; 149 case MOBILE: 150 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse.MOBILE); 151 break; 152 default: 153 tgt.setValue(org.hl7.fhir.dstu2.model.ContactPoint.ContactPointUse.NULL); 154 break; 155 } 156 } 157 return tgt; 158 } 159}