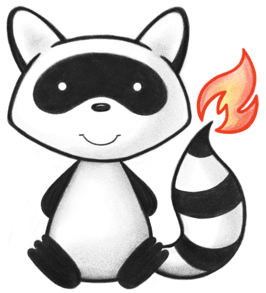
001package org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.dstu2.model.DateType; 005import org.hl7.fhir.exceptions.FHIRException; 006import org.hl7.fhir.r5.model.DateTimeType; 007 008public class Date10_50 { 009 public static org.hl7.fhir.r5.model.DateType convertDate(org.hl7.fhir.dstu2.model.DateType src) throws FHIRException { 010 org.hl7.fhir.r5.model.DateType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.DateType(src.getValueAsString()) : new org.hl7.fhir.r5.model.DateType(); 011 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 012 return tgt; 013 } 014 015 public static org.hl7.fhir.r5.model.DateType convertDate(org.hl7.fhir.dstu2.model.DateTimeType src) throws FHIRException { 016 org.hl7.fhir.r5.model.DateType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.DateType(src.getValueAsString()) : new org.hl7.fhir.r5.model.DateType(); 017 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 018 return tgt; 019 } 020 021 public static org.hl7.fhir.dstu2.model.DateType convertDate(org.hl7.fhir.r5.model.DateType src) throws FHIRException { 022 org.hl7.fhir.dstu2.model.DateType tgt = src.hasValue() ? new org.hl7.fhir.dstu2.model.DateType(src.getValueAsString()) : new org.hl7.fhir.dstu2.model.DateType(); 023 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 024 return tgt; 025 } 026 027 public static org.hl7.fhir.dstu2.model.DateType convertDate(org.hl7.fhir.r5.model.DateTimeType src) throws FHIRException { 028 org.hl7.fhir.dstu2.model.DateType tgt = src.hasValue() ? new org.hl7.fhir.dstu2.model.DateType(src.getValueAsString()) : new org.hl7.fhir.dstu2.model.DateType(); 029 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 030 return tgt; 031 } 032 033 public static DateTimeType convertDatetoDateTime(DateType src) { 034 org.hl7.fhir.r5.model.DateTimeType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.DateTimeType(src.getValueAsString()) : new org.hl7.fhir.r5.model.DateTimeType(); 035 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 036 return tgt; 037 } 038 039 public static DateType convertDateTimeToDate(DateTimeType src) { 040 org.hl7.fhir.dstu2.model.DateType tgt = src.hasValue() ? new org.hl7.fhir.dstu2.model.DateType(src.getValueAsString()) : new org.hl7.fhir.dstu2.model.DateType(); 041 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 042 return tgt; 043 } 044}