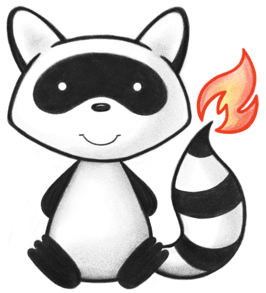
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Instant10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.MarkDown10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.PositiveInt10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 011import org.hl7.fhir.dstu2.model.UnsignedIntType; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.Appointment; 014import org.hl7.fhir.r5.model.BooleanType; 015import org.hl7.fhir.r5.model.CodeableConcept; 016import org.hl7.fhir.r5.model.Enumeration; 017 018public class Appointment10_50 { 019 020 public static org.hl7.fhir.dstu2.model.Appointment convertAppointment(org.hl7.fhir.r5.model.Appointment src) throws FHIRException { 021 if (src == null || src.isEmpty()) 022 return null; 023 org.hl7.fhir.dstu2.model.Appointment tgt = new org.hl7.fhir.dstu2.model.Appointment(); 024 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 025 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 026 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 027 if (src.hasStatus()) 028 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 029 for (org.hl7.fhir.r5.model.CodeableReference t : src.getServiceType()) 030 tgt.setType(CodeableConcept10_50.convertCodeableConcept(t.getConcept())); 031 if (src.hasPriority()) 032 tgt.setPriorityElement(convertAppointmentPriority(src.getPriority())); 033 if (src.hasDescriptionElement()) 034 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 035 if (src.hasStartElement()) 036 tgt.setStartElement(Instant10_50.convertInstant(src.getStartElement())); 037 if (src.hasEndElement()) 038 tgt.setEndElement(Instant10_50.convertInstant(src.getEndElement())); 039 if (src.hasMinutesDurationElement()) 040 tgt.setMinutesDurationElement(PositiveInt10_50.convertPositiveInt(src.getMinutesDurationElement())); 041 for (org.hl7.fhir.r5.model.Reference t : src.getSlot()) tgt.addSlot(Reference10_50.convertReference(t)); 042 if (src.hasNote()) 043 tgt.setCommentElement(String10_50.convertString(src.getNoteFirstRep().getTextElement())); 044 for (org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 045 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 046 return tgt; 047 } 048 049 private static UnsignedIntType convertAppointmentPriority(CodeableConcept src) { 050 UnsignedIntType tgt = new UnsignedIntType(convertAppointmentPriorityFromR5(src)); 051 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 052 return tgt; 053 } 054 055 private static CodeableConcept convertAppointmentPriority(UnsignedIntType src) { 056 CodeableConcept tgt = src.hasValue() ? convertAppointmentPriorityToR5(src.getValue()) : new CodeableConcept(); 057 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 058 return tgt; 059 } 060 061 public static org.hl7.fhir.r5.model.CodeableConcept convertAppointmentPriorityToR5(int priority) { 062 return null; 063 } 064 065 public static int convertAppointmentPriorityFromR5(org.hl7.fhir.r5.model.CodeableConcept priority) { 066 return 0; 067 } 068 069 public static org.hl7.fhir.r5.model.Appointment convertAppointment(org.hl7.fhir.dstu2.model.Appointment src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.r5.model.Appointment tgt = new org.hl7.fhir.r5.model.Appointment(); 073 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 074 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 075 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 076 if (src.hasStatus()) 077 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 078 if (src.hasType()) 079 tgt.addServiceType().setConcept(CodeableConcept10_50.convertCodeableConcept(src.getType())); 080 if (src.hasPriorityElement()) 081 tgt.setPriority(convertAppointmentPriority(src.getPriorityElement())); 082 if (src.hasDescriptionElement()) 083 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 084 if (src.hasStartElement()) 085 tgt.setStartElement(Instant10_50.convertInstant(src.getStartElement())); 086 if (src.hasEndElement()) 087 tgt.setEndElement(Instant10_50.convertInstant(src.getEndElement())); 088 if (src.hasMinutesDurationElement()) 089 tgt.setMinutesDurationElement(PositiveInt10_50.convertPositiveInt(src.getMinutesDurationElement())); 090 for (org.hl7.fhir.dstu2.model.Reference t : src.getSlot()) tgt.addSlot(Reference10_50.convertReference(t)); 091 if (src.hasCommentElement()) 092 tgt.getNoteFirstRep().setTextElement(MarkDown10_50.convertStringToMarkdown(src.getCommentElement())); 093 for (org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 094 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 095 return tgt; 096 } 097 098 099 public static org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 100 if (src == null || src.isEmpty()) 101 return null; 102 org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent(); 103 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 104 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 105 tgt.addType(CodeableConcept10_50.convertCodeableConcept(t)); 106 if (src.hasActor()) 107 tgt.setActor(Reference10_50.convertReference(src.getActor())); 108 if (src.hasRequired()) 109 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 110 if (src.hasStatus()) 111 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 112 return tgt; 113 } 114 115 public static org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.dstu2.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 116 if (src == null || src.isEmpty()) 117 return null; 118 org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent(); 119 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 120 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getType()) 121 tgt.addType(CodeableConcept10_50.convertCodeableConcept(t)); 122 if (src.hasActor()) 123 tgt.setActor(Reference10_50.convertReference(src.getActor())); 124 if (src.hasRequired()) 125 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 126 if (src.hasStatus()) 127 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 128 return tgt; 129 } 130 131 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus> src) throws FHIRException { 132 if (src == null || src.isEmpty()) 133 return null; 134 Enumeration<Appointment.AppointmentStatus> tgt = new Enumeration<>(new Appointment.AppointmentStatusEnumFactory()); 135 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 136 if (src.getValue() == null) { 137 tgt.setValue(null); 138 } else { 139 switch (src.getValue()) { 140 case PROPOSED: 141 tgt.setValue(Appointment.AppointmentStatus.PROPOSED); 142 break; 143 case PENDING: 144 tgt.setValue(Appointment.AppointmentStatus.PENDING); 145 break; 146 case BOOKED: 147 tgt.setValue(Appointment.AppointmentStatus.BOOKED); 148 break; 149 case ARRIVED: 150 tgt.setValue(Appointment.AppointmentStatus.ARRIVED); 151 break; 152 case FULFILLED: 153 tgt.setValue(Appointment.AppointmentStatus.FULFILLED); 154 break; 155 case CANCELLED: 156 tgt.setValue(Appointment.AppointmentStatus.CANCELLED); 157 break; 158 case NOSHOW: 159 tgt.setValue(Appointment.AppointmentStatus.NOSHOW); 160 break; 161 default: 162 tgt.setValue(Appointment.AppointmentStatus.NULL); 163 break; 164 } 165 } 166 return tgt; 167 } 168 169 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.AppointmentStatus> src) throws FHIRException { 170 if (src == null || src.isEmpty()) 171 return null; 172 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Appointment.AppointmentStatusEnumFactory()); 173 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 174 if (src.getValue() == null) { 175 tgt.setValue(null); 176 } else { 177 switch (src.getValue()) { 178 case PROPOSED: 179 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.PROPOSED); 180 break; 181 case PENDING: 182 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.PENDING); 183 break; 184 case BOOKED: 185 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.BOOKED); 186 break; 187 case ARRIVED: 188 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.ARRIVED); 189 break; 190 case FULFILLED: 191 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.FULFILLED); 192 break; 193 case CANCELLED: 194 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.CANCELLED); 195 break; 196 case NOSHOW: 197 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.NOSHOW); 198 break; 199 default: 200 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.AppointmentStatus.NULL); 201 break; 202 } 203 } 204 return tgt; 205 } 206 207 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipantRequired> convertParticipantRequired(org.hl7.fhir.r5.model.BooleanType src) throws FHIRException { 208 if (src == null || src.isEmpty()) 209 return null; 210 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipantRequired> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Appointment.ParticipantRequiredEnumFactory()); 211 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 212 if (src.getValue()) { 213 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.ParticipantRequired.REQUIRED); 214 } else { // case OPTIONAL: 215 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.ParticipantRequired.OPTIONAL); 216 } 217 return tgt; 218 } 219 220 static public org.hl7.fhir.r5.model.BooleanType convertParticipantRequired(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipantRequired> src) throws FHIRException { 221 if (src == null || src.isEmpty()) 222 return null; 223 BooleanType tgt = new BooleanType(); 224 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 225 if (src.getValue() == null) { 226 tgt.setValue(null); 227 } else { 228 switch (src.getValue()) { 229 case REQUIRED: 230 tgt.setValue(true); 231 break; 232 case OPTIONAL: 233 tgt.setValue(false); 234 break; 235 case INFORMATIONONLY: 236 tgt.setValue(false); 237 break; 238 default: 239 break; 240 } 241 } 242 return tgt; 243 } 244 245 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus> src) throws FHIRException { 246 if (src == null || src.isEmpty()) 247 return null; 248 Enumeration<Appointment.ParticipationStatus> tgt = new Enumeration<>(new Appointment.ParticipationStatusEnumFactory()); 249 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 250 if (src.getValue() == null) { 251 tgt.setValue(null); 252 } else { 253 switch (src.getValue()) { 254 case ACCEPTED: 255 tgt.setValue(Appointment.ParticipationStatus.ACCEPTED); 256 break; 257 case DECLINED: 258 tgt.setValue(Appointment.ParticipationStatus.DECLINED); 259 break; 260 case TENTATIVE: 261 tgt.setValue(Appointment.ParticipationStatus.TENTATIVE); 262 break; 263 case NEEDSACTION: 264 tgt.setValue(Appointment.ParticipationStatus.NEEDSACTION); 265 break; 266 default: 267 tgt.setValue(Appointment.ParticipationStatus.NULL); 268 break; 269 } 270 } 271 return tgt; 272 } 273 274 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.ParticipationStatus> src) throws FHIRException { 275 if (src == null || src.isEmpty()) 276 return null; 277 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Appointment.ParticipationStatusEnumFactory()); 278 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 279 if (src.getValue() == null) { 280 tgt.setValue(null); 281 } else { 282 switch (src.getValue()) { 283 case ACCEPTED: 284 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus.ACCEPTED); 285 break; 286 case DECLINED: 287 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus.DECLINED); 288 break; 289 case TENTATIVE: 290 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus.TENTATIVE); 291 break; 292 case NEEDSACTION: 293 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus.NEEDSACTION); 294 break; 295 default: 296 tgt.setValue(org.hl7.fhir.dstu2.model.Appointment.ParticipationStatus.NULL); 297 break; 298 } 299 } 300 return tgt; 301 } 302}