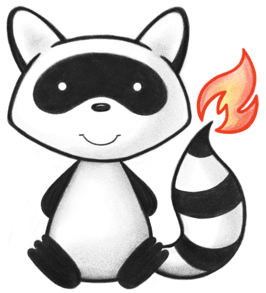
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Coding10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Base64Binary10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.CodeableConcept; 013 014public class AuditEvent10_50 { 015 016 public static org.hl7.fhir.dstu2.model.AuditEvent convertAuditEvent(org.hl7.fhir.r5.model.AuditEvent src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.dstu2.model.AuditEvent tgt = new org.hl7.fhir.dstu2.model.AuditEvent(); 020 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 021 if (src.getCategoryFirstRep().hasCoding()) { 022 tgt.getEvent().setType(Coding10_50.convertCoding(src.getCategoryFirstRep().getCodingFirstRep())); 023 } 024 for (org.hl7.fhir.r5.model.Coding t : src.getCode().getCoding()) tgt.getEvent().addSubtype(Coding10_50.convertCoding(t)); 025 tgt.getEvent().setActionElement(convertAuditEventAction(src.getActionElement())); 026 tgt.getEvent().setDateTime(src.getRecorded()); 027 028 if (src.hasOutcome() && "http://terminology.hl7.org/CodeSystem/audit-event-outcome".equals(src.getOutcome().getCode().getSystem())) 029 tgt.getEvent().getOutcomeElement().setValueAsString(src.getOutcome().getCode().getCode()); 030 if (src.getOutcome().getDetailFirstRep().hasText()) 031 tgt.getEvent().setOutcomeDescElement(String10_50.convertString(src.getOutcome().getDetailFirstRep().getTextElement())); 032 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAuthorization()) 033 for (org.hl7.fhir.r5.model.Coding cc : t.getCoding()) 034 tgt.getEvent().addPurposeOfEvent(Coding10_50.convertCoding(cc)); 035 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent t : src.getAgent()) 036 tgt.addParticipant(convertAuditEventAgentComponent(t)); 037 if (src.hasSource()) 038 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 039 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent t : src.getEntity()) 040 tgt.addObject(convertAuditEventEntityComponent(t)); 041 return tgt; 042 } 043 044 public static org.hl7.fhir.r5.model.AuditEvent convertAuditEvent(org.hl7.fhir.dstu2.model.AuditEvent src) throws FHIRException { 045 if (src == null || src.isEmpty()) 046 return null; 047 org.hl7.fhir.r5.model.AuditEvent tgt = new org.hl7.fhir.r5.model.AuditEvent(); 048 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 049 if (src.hasEvent()) { 050 if (src.hasType()) 051 tgt.getCategoryFirstRep().addCoding(Coding10_50.convertCoding(src.getEvent().getType())); 052 for (org.hl7.fhir.dstu2.model.Coding t : src.getEvent().getSubtype()) 053 tgt.getCode().addCoding(Coding10_50.convertCoding(t)); 054 tgt.setActionElement(convertAuditEventAction(src.getEvent().getActionElement())); 055 tgt.setRecorded(src.getEvent().getDateTime()); 056 057 if (src.getEvent().hasOutcome()) 058 tgt.getOutcome().getCode().setSystem("http://terminology.hl7.org/CodeSystem/audit-event-outcome").setCode(src.getEvent().getOutcome().toCode()); 059 if (src.getEvent().hasOutcomeDesc()) 060 tgt.getOutcome().getDetailFirstRep().setTextElement(String10_50.convertString(src.getEvent().getOutcomeDescElement())); 061 062 for (org.hl7.fhir.dstu2.model.Coding t : src.getEvent().getPurposeOfEvent()) 063 tgt.addAuthorization().addCoding(Coding10_50.convertCoding(t)); 064 } 065 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent t : src.getParticipant()) 066 tgt.addAgent(convertAuditEventAgentComponent(t)); 067 if (src.hasSource()) 068 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 069 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent t : src.getObject()) 070 tgt.addEntity(convertAuditEventEntityComponent(t)); 071 return tgt; 072 } 073 074 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> src) throws FHIRException { 075 if (src == null || src.isEmpty()) 076 return null; 077 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AuditEvent.AuditEventActionEnumFactory()); 078 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 079 switch (src.getValue()) { 080 case C: 081 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.C); 082 break; 083 case R: 084 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.R); 085 break; 086 case U: 087 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.U); 088 break; 089 case D: 090 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.D); 091 break; 092 case E: 093 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.E); 094 break; 095 default: 096 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.NULL); 097 break; 098 } 099 return tgt; 100 } 101 102 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> src) throws FHIRException { 103 if (src == null || src.isEmpty()) 104 return null; 105 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventActionEnumFactory()); 106 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 107 switch (src.getValue()) { 108 case C: 109 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.C); 110 break; 111 case R: 112 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.R); 113 break; 114 case U: 115 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.U); 116 break; 117 case D: 118 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.D); 119 break; 120 case E: 121 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.E); 122 break; 123 default: 124 tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventAction.NULL); 125 break; 126 } 127 return tgt; 128 } 129 130 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent convertAuditEventAgentComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent(); 134 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 135 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getRole()) 136 tgt.addRole(CodeableConcept10_50.convertCodeableConcept(t)); 137 if (src.hasWho()) { 138 if (src.getWho().hasIdentifier()) 139 tgt.setUserId(Identifier10_50.convertIdentifier(src.getWho().getIdentifier())); 140 if (src.getWho().hasReference() || src.getWho().hasDisplay() || src.getWho().hasExtension() || src.getWho().hasId()) 141 tgt.setReference(Reference10_50.convertReference(src.getWho())); 142 } 143// if (src.hasAltIdElement()) 144// tgt.setAltIdElement(String10_50.convertString(src.getAltIdElement())); 145// if (src.hasNameElement()) 146// tgt.setNameElement(String10_50.convertString(src.getNameElement())); 147 if (src.hasRequestorElement()) 148 tgt.setRequestorElement(Boolean10_50.convertBoolean(src.getRequestorElement())); 149 if (src.hasLocation()) 150 tgt.setLocation(Reference10_50.convertReference(src.getLocation())); 151 for (org.hl7.fhir.r5.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 152// if (src.hasMedia()) 153// tgt.setMedia(Coding10_50.convertCoding(src.getMedia())); 154// if (src.hasNetwork()) 155// tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 156 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAuthorization()) 157 for (org.hl7.fhir.r5.model.Coding cc : t.getCoding()) tgt.addPurposeOfUse(Coding10_50.convertCoding(cc)); 158 return tgt; 159 } 160 161 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent convertAuditEventAgentComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantComponent src) throws FHIRException { 162 if (src == null || src.isEmpty()) 163 return null; 164 org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent(); 165 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 166 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getRole()) 167 tgt.addRole(CodeableConcept10_50.convertCodeableConcept(t)); 168 if (src.hasReference()) 169 tgt.setWho(Reference10_50.convertReference(src.getReference())); 170 if (src.hasUserId()) 171 tgt.getWho().setIdentifier(Identifier10_50.convertIdentifier(src.getUserId())); 172// if (src.hasAltIdElement()) 173// tgt.setAltIdElement(String10_50.convertString(src.getAltIdElement())); 174// if (src.hasNameElement()) 175// tgt.setNameElement(String10_50.convertString(src.getNameElement())); 176 if (src.hasRequestorElement()) 177 tgt.setRequestorElement(Boolean10_50.convertBoolean(src.getRequestorElement())); 178 if (src.hasLocation()) 179 tgt.setLocation(Reference10_50.convertReference(src.getLocation())); 180 for (org.hl7.fhir.dstu2.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 181// if (src.hasMedia()) 182// tgt.setMedia(Coding10_50.convertCoding(src.getMedia())); 183// if (src.hasNetwork()) 184// tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 185 for (org.hl7.fhir.dstu2.model.Coding t : src.getPurposeOfUse()) 186 tgt.addAuthorization().addCoding(Coding10_50.convertCoding(t)); 187 return tgt; 188 } 189 190// public static org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent src) throws FHIRException { 191// if (src == null || src.isEmpty()) 192// return null; 193// org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent(); 194// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 195// if (src.hasAddressElement()) 196// tgt.setAddressElement(String10_50.convertString(src.getAddressElement())); 197// if (src.hasType()) 198// tgt.setTypeElement(convertAuditEventParticipantNetworkType(src.getTypeElement())); 199// return tgt; 200// } 201// 202// public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent src) throws FHIRException { 203// if (src == null || src.isEmpty()) 204// return null; 205// org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkComponent(); 206// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 207// if (src.hasAddressElement()) 208// tgt.setAddressElement(String10_50.convertString(src.getAddressElement())); 209// if (src.hasType()) 210// tgt.setTypeElement(convertAuditEventParticipantNetworkType(src.getTypeElement())); 211// return tgt; 212// } 213 214 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent convertAuditEventEntityComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent src) throws FHIRException { 215 if (src == null || src.isEmpty()) 216 return null; 217 org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent(); 218 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 219 if (src.hasIdentifier()) 220 tgt.getWhat().setIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 221 if (src.hasReference()) 222 tgt.setWhat(Reference10_50.convertReference(src.getReference())); 223// if (src.hasType()) 224// tgt.setType(Coding10_50.convertCoding(src.getType())); 225 if (src.hasRole()) 226 tgt.getRole().addCoding(Coding10_50.convertCoding(src.getRole())); 227// if (src.hasLifecycle()) 228// tgt.setLifecycle(Coding10_50.convertCoding(src.getLifecycle())); 229 for (org.hl7.fhir.dstu2.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel().addCoding(Coding10_50.convertCoding(t)); 230// if (src.hasNameElement()) 231// tgt.setNameElement(String10_50.convertString(src.getNameElement())); 232 if (src.hasQueryElement()) 233 tgt.setQueryElement(Base64Binary10_50.convertBase64Binary(src.getQueryElement())); 234 for (org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent t : src.getDetail()) 235 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 236 return tgt; 237 } 238 239 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent convertAuditEventEntityComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent src) throws FHIRException { 240 if (src == null || src.isEmpty()) 241 return null; 242 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectComponent(); 243 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 244 if (src.hasWhat()) { 245 if (src.getWhat().hasIdentifier()) 246 tgt.setIdentifier(Identifier10_50.convertIdentifier(src.getWhat().getIdentifier())); 247 if (src.getWhat().hasReference() || src.getWhat().hasDisplay() || src.getWhat().hasExtension() || src.getWhat().hasId()) 248 tgt.setReference(Reference10_50.convertReference(src.getWhat())); 249 } 250// if (src.hasType()) 251// tgt.setType(Coding10_50.convertCoding(src.getType())); 252 if (src.hasRole()) 253 tgt.setRole(Coding10_50.convertCoding(src.getRole().getCodingFirstRep())); 254// if (src.hasLifecycle()) 255// tgt.setLifecycle(Coding10_50.convertCoding(src.getLifecycle())); 256 for (CodeableConcept t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding10_50.convertCoding(t.getCodingFirstRep())); 257// if (src.hasNameElement()) 258// tgt.setNameElement(String10_50.convertString(src.getNameElement())); 259 if (src.hasQueryElement()) 260 tgt.setQueryElement(Base64Binary10_50.convertBase64Binary(src.getQueryElement())); 261 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent t : src.getDetail()) 262 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 263 return tgt; 264 } 265 266 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent src) throws FHIRException { 267 if (src == null || src.isEmpty()) 268 return null; 269 org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent(); 270 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 271 if (src.hasTypeElement()) 272 tgt.getType().setTextElement(String10_50.convertString(src.getTypeElement())); 273 if (src.hasValue()) 274 tgt.setValue(new org.hl7.fhir.r5.model.Base64BinaryType(src.getValue())); 275 return tgt; 276 } 277 278 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent src) throws FHIRException { 279 if (src == null || src.isEmpty()) 280 return null; 281 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventObjectDetailComponent(); 282 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 283 if (src.getType().hasTextElement()) 284 tgt.setTypeElement(String10_50.convertString(src.getType().getTextElement())); 285 if (src.hasValueStringType()) 286 tgt.setValue(src.getValueStringType().getValue().getBytes()); 287 else if (src.hasValueBase64BinaryType()) 288 tgt.setValue(src.getValueBase64BinaryType().getValue()); 289 return tgt; 290 } 291 292// 293// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> convertAuditEventParticipantNetworkType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> src) throws FHIRException { 294// if (src == null || src.isEmpty()) 295// return null; 296// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkTypeEnumFactory()); 297// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 298// switch (src.getValue()) { 299// case _1: 300// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._1); 301// break; 302// case _2: 303// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._2); 304// break; 305// case _3: 306// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._3); 307// break; 308// case _4: 309// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._4); 310// break; 311// case _5: 312// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._5); 313// break; 314// default: 315// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType.NULL); 316// break; 317// } 318// return tgt; 319// } 320// 321// static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> convertAuditEventParticipantNetworkType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> src) throws FHIRException { 322// if (src == null || src.isEmpty()) 323// return null; 324// org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkTypeEnumFactory()); 325// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 326// switch (src.getValue()) { 327// case _1: 328// tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._1); 329// break; 330// case _2: 331// tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._2); 332// break; 333// case _3: 334// tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._3); 335// break; 336// case _4: 337// tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._4); 338// break; 339// case _5: 340// tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType._5); 341// break; 342// default: 343// tgt.setValue(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventParticipantNetworkType.NULL); 344// break; 345// } 346// return tgt; 347// } 348 349 public static org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 350 if (src == null || src.isEmpty()) 351 return null; 352 org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent(); 353 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 354// if (src.hasSiteElement()) 355// tgt.setSiteElement(String10_50.convertString(src.getSiteElement())); 356 if (src.hasObserver()) 357 tgt.setIdentifier(Identifier10_50.convertIdentifier(src.getObserver().getIdentifier())); 358 for (CodeableConcept t : src.getType()) tgt.addType(Coding10_50.convertCoding(t.getCodingFirstRep())); 359 return tgt; 360 } 361 362 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.dstu2.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 363 if (src == null || src.isEmpty()) 364 return null; 365 org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent(); 366 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 367// if (src.hasSiteElement()) 368// tgt.setSiteElement(String10_50.convertString(src.getSiteElement())); 369 if (src.hasIdentifier()) 370 tgt.getObserver().setIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 371 for (org.hl7.fhir.dstu2.model.Coding t : src.getType()) tgt.addType().addCoding(Coding10_50.convertCoding(t)); 372 return tgt; 373 } 374}